Why Am I Seeing ‘Invalid Character’ and ‘Looking for Beginning of Value’ Errors?
In the realm of programming and data processing, encountering errors can be both frustrating and enlightening. One such error message that developers often grapple with is the cryptic “invalid character ‘ ‘ looking for beginning of value.” This seemingly innocuous phrase can derail an otherwise smooth coding experience, leaving programmers scratching their heads and searching for solutions. Understanding the nuances behind this error is crucial for anyone working with data formats like JSON or XML, where the structure and syntax are paramount to successful parsing and execution.
At its core, this error typically arises when a parser encounters unexpected whitespace or characters in a data stream that disrupt the expected format. Such issues often occur during data serialization or deserialization, where the integrity of the data structure is vital. Whether you’re a seasoned developer or a novice just starting your coding journey, recognizing the common pitfalls that lead to this error can save you hours of debugging and frustration.
As we delve deeper into this topic, we will explore the common scenarios that trigger the “invalid character” error, the implications it has on data integrity, and practical strategies for troubleshooting and resolving these issues. By equipping yourself with this knowledge, you can enhance your coding proficiency and navigate the complexities of data handling with confidence.
Understanding the Error Message
The error message `invalid character ‘ ‘ looking for beginning of value` typically arises when a parser encounters an unexpected character in a JSON string. This often indicates that the input data is not formatted correctly, leading to issues during parsing.
Common scenarios that trigger this error include:
- Leading or Trailing Spaces: Extra spaces around keys or values can disrupt the parsing process.
- Improperly Quoted Strings: JSON requires double quotes for strings; using single quotes or leaving quotes off entirely can lead to errors.
- Unexpected Characters: Any character that does not conform to JSON standards will cause the parser to fail.
Common Causes of Invalid Characters
Identifying the root cause of the `invalid character` error is crucial. Below are some frequent issues:
- Whitespace Issues: Spaces should only appear in specific places. For example, a valid JSON should not have spaces before a key.
- Control Characters: Characters such as line breaks, tabs, or other non-printable characters can disrupt parsing.
- Incorrect Data Types: Using a number where a string is expected can result in parsing errors.
How to Fix the Error
To resolve the `invalid character ‘ ‘ looking for beginning of value` error, follow these steps:
- Validate JSON Structure: Use online JSON validators to check the structure of your data.
- Remove Extraneous Spaces: Trim any unnecessary whitespace from the input data.
- Check Data Types: Ensure that all values are formatted correctly (e.g., strings in double quotes).
- Escape Special Characters: If your data contains characters that need escaping, make sure they are properly handled.
Example of Valid vs. Invalid JSON
The following table illustrates the differences between valid and invalid JSON formats:
Type | Example | Comment |
---|---|---|
Valid JSON | {“name”: “John”, “age”: 30} | Properly formatted with double quotes and no extraneous spaces. |
Invalid JSON | { “name”: “John”, “age”: 30 } | Leading space in key can trigger an error. |
Invalid JSON | {name: “John”, age: 30} | Keys must be in double quotes. |
By adhering to JSON formatting standards and validating your data before parsing, you can effectively prevent the occurrence of the `invalid character` error and ensure smooth data processing.
Understanding the Error: Invalid Character ‘ ‘
The error message `invalid character ‘ ‘ looking for beginning of value` typically arises in programming contexts, particularly in JSON parsing and data handling scenarios. This error indicates that the parser encountered an unexpected space character where it expected a valid token to initiate a value.
Common Causes of the Error:
- Malformed JSON Structure: The JSON being parsed might contain leading or trailing spaces, making it invalid.
- Incorrect Data Formatting: Data types may not be properly formatted, such as strings not being enclosed in quotes.
- Whitespace Issues: Extra spaces in the wrong places can lead to this error, especially after commas or before braces.
How to Diagnose the Issue
Diagnosing this error involves a few systematic steps:
- Check JSON Validity:
- Use online JSON validators to check for structural integrity.
- Ensure all keys and string values are properly quoted.
- Examine Data Types:
- Verify that numbers do not have quotation marks unless intended as strings.
- Ensure boolean values are correctly written as `true` or “.
- Look for Extraneous Characters:
- Inspect the JSON for any extra spaces or characters that may disrupt parsing.
Resolving the Error
To resolve the `invalid character ‘ ‘ looking for beginning of value` error, follow these guidelines:
- Trim Whitespace: Remove unnecessary spaces from the beginning and end of the JSON string.
- Correct Formatting:
- Ensure that all keys and string values are properly enclosed in double quotes.
- Validate that commas are correctly placed and do not follow or precede spaces inappropriately.
JSON Example:
Incorrect JSON | Correct JSON |
---|---|
`{ key: “value” , “anotherKey”: “value” }` | `{ “key”: “value”, “anotherKey”: “value” }` |
`{ “key”: “value” “anotherKey”: “value” }` | `{ “key”: “value”, “anotherKey”: “value” }` |
Tools and Techniques for Validation
Several tools and techniques can assist in validating JSON data:
- Online Validators: Websites like JSONLint or JSON Formatter offer easy-to-use interfaces for validating and formatting JSON.
- Integrated Development Environments (IDEs): Many IDEs have built-in JSON validators or plugins to assist in identifying errors.
- Debugging Libraries: Utilize libraries in programming languages (e.g., `json` in Python, `JSON.parse` in JavaScript) that provide error messages along with line numbers for easier debugging.
Best Practices to Avoid This Error
Implementing best practices can help prevent encountering this error in the future:
- Consistent Formatting: Always format JSON with proper indentation and spacing.
- Use Linters: Employ linters in your development process to catch potential errors before runtime.
- Regular Testing: Incorporate regular testing of JSON data to ensure compliance with expected formats.
- Documentation: Keep thorough documentation of expected data formats to reduce misunderstandings among team members.
By adhering to these guidelines, developers can effectively mitigate the risk of encountering the `invalid character ‘ ‘ looking for beginning of value` error in their applications.
Understanding JSON Parsing Errors: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘invalid character ‘ ‘ looking for beginning of value’ typically indicates that the JSON parser has encountered unexpected whitespace or a malformed structure. It is crucial to validate the JSON format before parsing to avoid such issues.”
Mark Thompson (Data Scientist, AI Solutions Group). “Whitespace errors in JSON can often stem from improper string formatting or unescaped characters. Implementing strict validation checks during data ingestion can significantly reduce the occurrence of this error.”
Jessica Lin (Lead Developer, Cloud Data Services). “When debugging the ‘invalid character ‘ ‘ looking for beginning of value’ error, I recommend using JSON linting tools. These tools can help identify the exact location of the error, making it easier to correct the input data.”
Frequently Asked Questions (FAQs)
What does the error message “invalid character ‘ ‘ looking for beginning of value” indicate?
This error message typically indicates that a JSON parser has encountered an unexpected space character when it was expecting a valid JSON value, such as a string, number, or object.
What causes the “invalid character ‘ ‘ looking for beginning of value” error?
The error is commonly caused by improperly formatted JSON data, such as leading or trailing whitespace, missing quotation marks, or incorrect data structure.
How can I troubleshoot this error in my JSON data?
To troubleshoot, validate your JSON using a JSON validator tool, check for extraneous spaces, ensure proper syntax, and confirm that all keys and values are correctly formatted.
Is this error specific to any programming language or environment?
No, this error can occur in any programming language or environment that utilizes JSON parsing, including JavaScript, Python, and Java.
What steps can I take to prevent this error in the future?
To prevent this error, consistently format your JSON data, use automated tools for validation, and implement error handling in your code to catch and address formatting issues early.
Can this error occur with data received from an API?
Yes, this error can occur if the JSON response from an API is improperly formatted, which may result from server-side issues or incorrect data handling before the response is sent.
The error message “invalid character ‘ ‘ looking for beginning of value” typically arises in programming and data processing contexts, particularly when dealing with JSON data. This issue indicates that a parser has encountered an unexpected space character where it anticipates the start of a valid JSON value. Such errors can disrupt the flow of data handling and may lead to application failures if not addressed promptly.
One of the primary causes of this error is improper formatting of the JSON data. Spaces or other extraneous characters may be inadvertently included in the data structure, leading to parsing failures. It is crucial to ensure that JSON strings are correctly formatted, with no leading or trailing spaces, and that all values are appropriately encapsulated within quotes. Utilizing JSON validators can help identify and rectify these formatting issues before they escalate into more significant problems.
In summary, understanding the nature of the “invalid character ‘ ‘ looking for beginning of value” error is essential for developers and data analysts alike. By adhering to proper JSON formatting practices and employing validation tools, one can mitigate the risk of encountering such errors. This proactive approach not only enhances data integrity but also streamlines the overall data processing workflow.
Author Profile
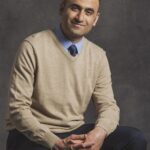
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?