How Can I Resolve the ‘Invalid Value Type for Attribute ‘factorybeanobjecttype’: java.lang.String’ Error?
In the ever-evolving landscape of software development, the intricacies of configuration and dependency management can often lead to perplexing challenges. One such conundrum that developers encounter is the error message: `invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string?`. This seemingly cryptic notification can halt progress and provoke frustration, particularly when the underlying issue is not immediately apparent. Understanding the nuances of this error is crucial for developers who aim to streamline their applications and enhance their productivity.
At its core, this error typically arises within the context of frameworks that utilize the FactoryBean pattern, such as Spring. When a developer attempts to define a bean with an incorrect type or value, the framework raises this alert to signal a mismatch between expected and provided data types. This situation can stem from various factors, including misconfigured XML files, incorrect annotations, or even simple typographical errors. As a result, grasping the underlying principles of FactoryBeans and the importance of type safety is essential for effective troubleshooting.
Navigating this error requires a blend of technical acumen and a methodical approach to problem-solving. By dissecting the configuration files and understanding the expected value types, developers can not only resolve the immediate issue but also fortify their applications against similar pitfalls in the future.
Understanding the Error Message
The error message `invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string?` typically occurs in Java applications, particularly those utilizing Spring Framework’s factory beans. This error indicates that there is a mismatch between the expected type of an attribute and the actual type being provided.
In Spring, factory beans are used to encapsulate the logic of creating objects. Each factory bean can have various attributes defined in its configuration. The `factorybeanobjecttype` attribute specifies the type of object that the factory will create. If a string is provided where an object type is expected, the application throws this error.
Common Causes
Several factors can lead to this error:
- Incorrect Configuration: The configuration file (usually XML or Java-based) may have an incorrect type specified for the `factorybeanobjecttype`.
- Type Mismatch: There may be a type mismatch between the expected Java class and the string provided in the configuration.
- Dependency Injection Issues: Issues in dependency injection where beans are not correctly defined or wired can also lead to this error.
Resolving the Error
To resolve the error, consider the following steps:
- Review Configuration: Check the configuration file for the factory bean. Ensure that the `factorybeanobjecttype` attribute correctly references a valid Java class.
- Validate Types: Confirm that the type specified aligns with the class being instantiated. The type must be a fully qualified class name.
- Check Bean Definitions: Make sure that all required beans are properly defined and that their dependencies are correctly injected.
Example Configuration
Here’s an example of a correct configuration that avoids the `invalid value type for attribute ‘factorybeanobjecttype’` error:
“`xml
“`
In this example, the `factorybeanobjecttype` is set to the fully qualified name of the class `MyObject`, which is what the factory bean is expected to produce.
Best Practices
To minimize the risk of encountering this error in the future, adhere to the following best practices:
- Use Type Safety: Where possible, use type-safe methods to define beans, such as Java-based configuration instead of XML.
- Leverage IDE Features: Utilize IDE features that can validate configurations and type references, helping catch errors early.
- Consistent Naming Conventions: Maintain consistent naming conventions for classes and ensure that they match the configurations.
Commonly Used Attributes
Here is a table summarizing commonly used attributes in factory beans and their expected types:
Attribute | Expected Type | Description |
---|---|---|
factorybeanobjecttype | Class Name (String) | The type of object the factory creates. |
singleton | Boolean | Indicates if the bean is a singleton. |
prototype | Boolean | Indicates if the bean is a prototype. |
By following these guidelines and understanding the intricacies of factory beans, developers can effectively manage and troubleshoot issues related to object creation in Spring applications.
Understanding the Error Message
The error message `invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string` typically arises in Java applications, particularly those using the Spring Framework. This indicates that a configuration issue has occurred regarding the expected type of an attribute within a factory bean.
Common Causes of the Error
- Type Mismatch: The attribute `factorybeanobjecttype` expects a specific type, usually a class or interface. Providing a string instead leads to this error.
- Incorrect XML Configuration: If you are using XML-based configuration, the attribute may be defined incorrectly, often due to typos or incorrect nesting.
- Java Configuration Issues: In Java-based configuration, ensure that the object type is defined correctly in the method signatures.
Key Attributes to Check
When troubleshooting this error, focus on the following attributes in your configuration:
Attribute | Description |
---|---|
`factory-bean` | The bean that creates the object. |
`factory-method` | The method on the factory bean to invoke. |
`factory-bean-object-type` | The expected type of the object produced. |
Resolution Steps
Addressing this issue involves several steps to ensure that your configuration aligns with expected types.
Verify Configuration
- XML Configuration:
- Check the `
` definition in your XML file. - Ensure the `factory-bean` and `factory-method` are correctly specified.
- Confirm that `factorybeanobjecttype` references a valid Java class.
- Java Configuration:
- Inspect the `@Bean` method annotations to ensure the return type matches the expected object type.
- Use generics where applicable to provide type safety.
Example of Correct Configuration
XML Example:
“`xml
“`
Java Example:
“`java
@Bean
public MyBean myBean() {
return myFactory.createInstance();
}
“`
Debugging Techniques
If the error persists after reviewing your configurations, consider employing the following debugging techniques:
- Enable Debug Logging: Increase the logging level in your application to capture detailed information about bean creation.
- Use a Bean Definition Registry: Inspect the bean definitions at runtime to confirm their types.
- Unit Tests: Write tests to isolate the factory bean and ensure it returns the expected type.
Best Practices
To avoid encountering this error in the future, implement these best practices:
- Type Safety: Always specify the expected types in your factory methods.
- Clear Documentation: Maintain documentation for configuration files, ensuring all developers understand the expected types and structure.
- Consistent Naming Conventions: Use consistent and descriptive naming for beans and attributes to reduce the chances of misconfiguration.
Following these guidelines will help maintain robust configurations and reduce the likelihood of encountering the `invalid value type for attribute ‘factorybeanobjecttype’` error.
Understanding the ‘Invalid Value Type’ Error in Java Applications
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string’ typically arises when a Spring configuration expects a specific object type but receives a string instead. It is crucial to ensure that the configuration aligns with the expected data types to prevent runtime exceptions.”
Michael Chen (Java Framework Specialist, CodeCraft Solutions). “This error often indicates a misconfiguration in the Spring context. Developers should verify the bean definitions and ensure that the attributes are correctly defined to match the expected types. A thorough review of the XML or Java configuration can often resolve this issue.”
Sarah Johnson (Lead Application Architect, FutureTech Systems). “When encountering the ‘invalid value type’ error, it is essential to consider the context in which the factory bean is being used. Understanding the expected input types and ensuring that the correct data is provided will help mitigate this common issue in Java applications.”
Frequently Asked Questions (FAQs)
What does the error ‘invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string’ mean?
This error indicates that a string value has been provided for an attribute that expects a different data type, typically an object type that is not compatible with a string.
How can I resolve the ‘invalid value type for attribute ‘factorybeanobjecttype’ error?
To resolve this error, ensure that the value assigned to the ‘factorybeanobjecttype’ attribute matches the expected data type. Check your configuration files or code to verify the correct object type is being used.
What types of values are acceptable for ‘factorybeanobjecttype’?
The ‘factorybeanobjecttype’ attribute typically accepts class types or object references rather than string literals. Make sure to provide a valid class type that corresponds to the expected bean type.
Is this error related to Spring Framework?
Yes, this error commonly occurs in the Spring Framework context when configuring beans, particularly when using FactoryBeans or similar constructs where type mismatches can arise.
Can this error occur due to incorrect bean definitions?
Yes, incorrect bean definitions or misconfigurations in your Spring XML or Java configuration can lead to this error. Review your bean definitions for any discrepancies in type declarations.
What tools can help diagnose this issue in a Spring application?
Utilizing tools such as Spring Boot DevTools, IDE debugging features, and logging frameworks can help diagnose and trace the source of the error in your Spring application.
The error message “invalid value type for attribute ‘factorybeanobjecttype’: java.lang.string” typically indicates a mismatch between the expected data type and the actual data type provided for a specific attribute in a configuration file, often within a Spring application context. This error arises when a string is passed where a different type, such as a class type or bean reference, is expected. Understanding the context in which this error occurs is crucial for effective troubleshooting.
To resolve this issue, developers should carefully review the configuration files, such as XML or Java-based configuration, to ensure that the attributes are correctly defined with the appropriate types. It is essential to verify that the value assigned to the ‘factorybeanobjecttype’ attribute aligns with the expected data type specified by the framework. Additionally, consulting the relevant documentation can provide clarity on the correct usage of the attribute and its expected values.
Furthermore, this error highlights the importance of type safety and validation in configuration settings. It serves as a reminder to developers to implement rigorous checks and balances when defining attributes in their applications. By adhering to best practices and leveraging tools that can validate configurations before runtime, developers can minimize the risk of encountering such type-related errors in the future.
Author Profile
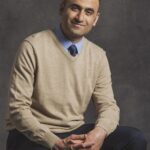
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?