Is Golang Memory Safe? Exploring the Safety Features of Go Programming
In the ever-evolving landscape of programming languages, Go, commonly known as Golang, has emerged as a powerful contender, particularly in the realms of cloud computing and microservices. As developers seek to create robust, efficient, and scalable applications, the question of memory safety becomes increasingly pertinent. Memory safety refers to the prevention of common programming errors, such as buffer overflows and null pointer dereferences, which can lead to vulnerabilities and crashes. But how does Golang stack up in this critical area? In this article, we will delve into the nuances of Golang’s memory management features, exploring its design philosophy and the mechanisms it employs to ensure safer memory usage.
Golang’s approach to memory safety is rooted in its unique blend of simplicity and efficiency. Unlike languages that require manual memory management, Go incorporates garbage collection, which automatically reclaims memory that is no longer in use. This feature not only reduces the burden on developers but also minimizes the risk of memory leaks and dangling pointers. However, the language does not completely eliminate the potential for memory-related issues, as certain constructs and practices can still lead to unsafe behavior. Understanding these aspects is crucial for developers who wish to harness the full potential of Golang while maintaining safe coding practices.
As we explore the intricacies
Memory Management in Go
Go employs a garbage collection mechanism that automatically manages memory allocation and deallocation, which significantly reduces the risk of memory leaks and dangling pointers. The garbage collector runs concurrently with the application, allowing it to reclaim memory from objects that are no longer in use without requiring explicit programmer intervention. This feature enhances memory safety by ensuring that memory is not accessed after it has been freed.
- Garbage Collection: Go uses a concurrent mark-and-sweep garbage collector.
- Automatic Memory Management: Developers do not need to manually allocate or free memory, minimizing human error.
However, while Go’s memory management features contribute to its safety, there are still scenarios where memory safety can be compromised:
- Pointer Arithmetic: Go allows the use of pointers, which can lead to unsafe memory access if misused.
- C Interoperability: When using cgo to call C libraries, the programmer must be cautious, as C does not enforce the same memory safety guarantees.
Concurrency and Memory Safety
Go’s concurrency model, built around goroutines and channels, enhances memory safety in concurrent programming. The language encourages communication over shared memory, which mitigates race conditions and helps ensure that data is accessed in a safe manner.
To illustrate the interplay between concurrency and memory safety, consider the following characteristics:
Feature | Description |
---|---|
Goroutines | Lightweight threads managed by Go’s runtime, facilitating concurrent execution. |
Channels | A built-in mechanism for safe communication between goroutines, helping to synchronize data access. |
Select Statement | Allows a goroutine to wait on multiple communication operations, promoting safe concurrent handling of data. |
By leveraging these features, developers can write concurrent programs that maintain memory safety, as the language’s design discourages shared mutable state.
Unsafe Package in Go
While Go provides a robust set of memory safety features, it also includes an `unsafe` package. This package allows developers to bypass Go’s type safety and memory safety guarantees when absolutely necessary. It offers functionalities such as pointer manipulation and type conversions that can lead to performance optimizations but comes with significant risks.
- Use Cases for `unsafe`: Situations where maximum performance is critical, or when interfacing directly with hardware or system calls.
- Risks: Utilizing the `unsafe` package can lead to memory corruption, crashes, and security vulnerabilities if not handled with extreme caution.
In summary, while Go is designed to be memory safe through automatic memory management and safe concurrency practices, the presence of the `unsafe` package provides flexibility at the cost of potential risks. Developers should weigh the benefits and drawbacks carefully when considering its use.
Understanding Memory Safety in Go
Go, or Golang, incorporates several features designed to enhance memory safety, although it does not guarantee complete memory safety in all scenarios. The language’s design and architecture promote safe memory handling through the following mechanisms:
- Garbage Collection: Go employs automatic garbage collection to manage memory allocation and deallocation. This reduces the chances of memory leaks and dangling pointers, which are common sources of memory safety issues in languages without garbage collection.
- Strong Typing: Go is a statically typed language, which means that type checks occur at compile time. This helps catch type-related errors before the code is executed, minimizing runtime errors related to memory access.
- No Pointer Arithmetic: Unlike languages such as C or C++, Go does not allow pointer arithmetic. This restriction prevents programmers from inadvertently accessing memory outside of allocated bounds, a common source of vulnerabilities.
- Concurrency Safety: Go’s concurrency model, based on goroutines and channels, facilitates safe concurrent programming. The language’s design avoids shared memory where possible, reducing the risk of race conditions and ensuring that memory access is managed safely across concurrent operations.
Limitations and Considerations
Despite these features, certain limitations exist that developers should be aware of:
Aspect | Explanation |
---|---|
Nil Pointers | Go allows the use of nil pointers, which can lead to runtime panics if dereferenced without checking. |
Slice and Map Behavior | Slices and maps in Go are reference types. Modifying them can affect other references, potentially leading to unexpected behavior. |
Unsafe Package | Go provides an `unsafe` package that allows low-level memory manipulation. This can lead to memory safety violations if used improperly. |
External Libraries | Using third-party libraries may introduce memory safety risks, as those libraries may not adhere to Go’s safety principles. |
Best Practices for Ensuring Memory Safety in Go
To maximize memory safety while programming in Go, developers should consider the following best practices:
- Check for Nil Values: Always verify if pointers or interfaces are nil before dereferencing them.
- Use `defer` for Cleanup: Utilize the `defer` statement to ensure that resources are released properly, especially when dealing with external resources like file handles or network connections.
- Avoid the Unsafe Package: Limit the use of the `unsafe` package unless absolutely necessary, as it bypasses Go’s safety guarantees.
- Leverage the Type System: Utilize Go’s strong typing to define clear interfaces and avoid type assertions wherever possible.
- Conduct Regular Code Reviews: Collaborate with peers to review code for potential memory safety issues and ensure adherence to best practices.
Implementing these practices can significantly improve memory safety in Go applications, making them more robust and less prone to common memory-related errors.
Evaluating Memory Safety in Go Programming
Dr. Emily Carter (Computer Science Professor, Tech University). “Go, or Golang, incorporates several features that enhance memory safety, such as garbage collection and strong typing. However, it is not entirely memory-safe, as developers can still inadvertently introduce memory leaks or race conditions if they misuse goroutines or pointers.”
Michael Chen (Senior Software Engineer, Cloud Solutions Inc.). “While Go provides a robust framework for managing memory through its built-in features, it is essential to understand that memory safety largely depends on the developer’s discipline. Proper usage of channels and synchronization primitives is crucial to avoid unsafe memory access.”
Lisa Patel (Security Analyst, Secure Code Initiative). “In the context of memory safety, Go is a significant improvement over languages like C or C++. However, developers must remain vigilant. The absence of certain safety checks means that while Go minimizes risks, it does not eliminate them entirely.”
Frequently Asked Questions (FAQs)
Is Golang memory safe?
Golang, or Go, is designed with memory safety in mind. It employs garbage collection and prevents common memory-related issues such as buffer overflows and null pointer dereferences, making it safer than languages that allow manual memory management.
What features contribute to Golang’s memory safety?
Golang includes features such as garbage collection, strong typing, and built-in data structures that manage memory automatically. These features help reduce the likelihood of memory leaks and other unsafe memory operations.
Can developers still introduce memory safety issues in Golang?
While Golang minimizes memory safety issues, developers can still introduce problems through the use of unsafe packages, which allow low-level memory manipulation. Caution is advised when using these features.
How does Golang handle concurrency and memory safety?
Golang uses goroutines and channels for concurrency, which helps ensure memory safety by avoiding shared memory access issues. This design encourages safe communication between goroutines without the need for explicit locks.
Are there any limitations to Golang’s memory safety?
Golang’s memory safety mechanisms may introduce performance overhead due to garbage collection. Additionally, certain low-level operations may require the use of unsafe packages, which can compromise safety if not handled properly.
How does Golang compare to other languages in terms of memory safety?
Compared to languages like C and C++, Golang provides a higher level of memory safety due to its automatic memory management and strong type system. However, languages like Rust offer even stricter guarantees through ownership and borrowing principles.
Golang, or Go, is designed with memory safety in mind, incorporating features that help prevent common programming errors related to memory management. Its garbage collection system automatically handles memory allocation and deallocation, reducing the risk of memory leaks and dangling pointers. This built-in feature allows developers to focus on writing efficient code without the burden of manual memory management, which is often a source of vulnerabilities in other programming languages.
Moreover, Go employs strong typing and a strict compile-time checking mechanism that helps catch potential memory-related issues before the code is executed. The language’s design encourages the use of safe concurrency patterns, which further enhances memory safety by preventing data races and ensuring that shared data is accessed in a controlled manner. These characteristics make Go a compelling choice for developers looking to build robust and secure applications.
However, it is important to note that while Go significantly mitigates memory safety issues, it does not eliminate them entirely. Developers must still adhere to best practices and be vigilant about how they manage pointers and slices. Understanding the language’s memory model and utilizing its features effectively is crucial to maintaining memory safety in Go applications.
Author Profile
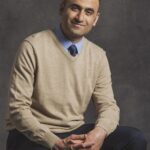
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?