Is JavaScript Pass by Reference or Pass by Value? Unpacking the Confusion!
JavaScript is a versatile and widely-used programming language that powers everything from dynamic web applications to server-side development. As developers delve into its intricacies, one question often arises: Is JavaScript pass by reference? This seemingly simple inquiry opens the door to a deeper understanding of how JavaScript handles data, memory, and variable manipulation. Whether you’re a seasoned programmer or just starting your coding journey, grasping the nuances of how JavaScript manages variable passing can significantly impact the way you write and debug your code.
At its core, the concept of passing variables in programming languages can be categorized into two main types: pass by value and pass by reference. In JavaScript, understanding these distinctions is crucial for effective coding. While primitive data types, such as numbers and strings, are passed by value, complex data types like objects and arrays behave differently. This duality can lead to confusion, especially for those accustomed to languages that strictly adhere to one method of passing variables.
As we explore this topic further, we’ll uncover how JavaScript’s approach to variable passing affects memory allocation, performance, and the behavior of your code. By the end of this discussion, you’ll not only have a clearer understanding of whether JavaScript is truly pass by reference, but you’ll also gain insights into best practices for managing
Understanding JavaScript’s Parameter Passing
In JavaScript, the way parameters are passed to functions can often lead to confusion, particularly when distinguishing between pass-by-value and pass-by-reference. To clarify, JavaScript utilizes a combination of both methods, depending on the data type involved.
Primitive Types vs. Reference Types
JavaScript has two main categories of data types: primitive types and reference types.
- Primitive Types: These include `number`, `string`, `boolean`, `undefined`, `null`, and `symbol`. When a primitive type is passed to a function, a copy of the value is created. Therefore, changes made to the parameter inside the function do not affect the original variable.
- Reference Types: These encompass objects, arrays, and functions. When a reference type is passed, a reference to the original object is provided, meaning that any modifications made to the parameter inside the function will affect the original object.
Data Type | Pass Type | Example Behavior |
---|---|---|
Primitive | Pass by Value | Original value unchanged |
Reference | Pass by Reference | Original object modified |
Examples of Parameter Passing
To illustrate how JavaScript handles these two types of passing, consider the following code examples:
Example 1: Primitive Type
javascript
function changeValue(val) {
val = 10;
}
let num = 5;
changeValue(num);
console.log(num); // Output: 5
In this example, the value of `num` remains unchanged because `val` receives a copy of `num`.
Example 2: Reference Type
javascript
function changeObject(obj) {
obj.key = ‘new value’;
}
let myObject = { key: ‘initial value’ };
changeObject(myObject);
console.log(myObject.key); // Output: ‘new value’
Here, the `obj` parameter refers to the same object as `myObject`, thus modifying `obj` also alters `myObject`.
Implications of Pass-by-Reference
Understanding that objects are passed by reference has several implications for developers:
- Mutability: Since objects can be altered directly, it’s essential to be cautious when passing mutable objects to functions to avoid unintended side effects.
- Memory Management: Passing large objects can be more efficient as only the reference is passed rather than a full copy of the object.
- Functional Programming: In functional programming paradigms, maintaining immutability is often preferred. Developers can utilize techniques such as cloning objects to avoid unwanted modifications.
JavaScript’s Passing Mechanism
JavaScript’s approach to parameter passing, combining pass-by-value for primitive types and pass-by-reference for objects, allows for flexibility but also requires careful handling to prevent unintended side effects in code. Understanding these nuances is crucial for effective JavaScript programming.
Understanding Pass by Value vs. Pass by Reference
In JavaScript, the concept of passing arguments to functions can be categorized into two main types: pass by value and pass by reference. Understanding these concepts is crucial for effectively managing variable interactions within your code.
- Pass by Value: This occurs when a copy of the variable’s value is passed to the function. Any modifications made to the parameter within the function do not affect the original variable outside the function.
- Example:
javascript
function modifyValue(x) {
x = 10;
}
let a = 5;
modifyValue(a);
console.log(a); // Outputs: 5
- Pass by Reference: This takes place when a reference to the actual variable is passed, allowing the function to modify the original variable. In JavaScript, this applies primarily to objects and arrays.
- Example:
javascript
function modifyObject(obj) {
obj.key = ‘new value’;
}
let myObj = { key: ‘initial value’ };
modifyObject(myObj);
console.log(myObj.key); // Outputs: ‘new value’
JavaScript’s Behavior with Primitive Types
JavaScript has distinct treatment for primitive types and reference types:
Type | Passed By | Behavior |
---|---|---|
Number | Value | Changes do not reflect outside the function. |
String | Value | Strings are immutable; changes do not reflect. |
Boolean | Value | Same as above; no effect on the original. |
Undefined | Value | No effect; remains undefined outside. |
Null | Value | Treated as a value; no changes outside. |
When primitives are passed to functions, they are copied, ensuring the original values remain unchanged.
JavaScript’s Behavior with Reference Types
In contrast, reference types include objects and arrays, which are passed by reference. This allows for direct manipulation of the original data structure.
Type | Passed By | Behavior |
---|---|---|
Object | Reference | Modifications affect the original object. |
Array | Reference | Changes reflect in the original array. |
- Example with Array:
javascript
function modifyArray(arr) {
arr.push(4);
}
let numbers = [1, 2, 3];
modifyArray(numbers);
console.log(numbers); // Outputs: [1, 2, 3, 4]
Implications for Developers
Understanding the distinction between pass by value and pass by reference is essential for developers to avoid unintended side effects in their code. Key considerations include:
- Immutable Data: Always treat primitive types as immutable to prevent unexpected changes.
- Object Mutability: Be cautious when passing objects or arrays; unintended changes can lead to bugs.
- Function Parameters: Clearly document function parameters to indicate whether they are expected to be modified.
By mastering these concepts, developers can write more predictable and maintainable JavaScript code.
Understanding JavaScript’s Pass-by-Value vs. Pass-by-Reference
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). JavaScript primarily uses a pass-by-value mechanism for primitive data types, such as numbers and strings. However, when it comes to objects and arrays, it behaves like pass-by-reference, meaning that the reference to the object is passed, not the object itself. This distinction is crucial for developers to understand how data is manipulated within their applications.
Michael Chen (JavaScript Framework Specialist, CodeCraft Academy). It is a common misconception that JavaScript is purely pass-by-reference. In reality, the language’s handling of data types leads to a nuanced behavior where primitives are passed by value, while objects are passed by reference. This can lead to unexpected results if developers are not careful with how they manage object references in their code.
Sarah Thompson (Lead Developer, Web Solutions Group). Understanding whether JavaScript is pass-by-reference or pass-by-value is essential for debugging and optimizing code. While objects are passed by reference, any changes made to a primitive type in a function do not affect the original variable outside that function. This behavior highlights the importance of recognizing the type of data being handled in JavaScript.
Frequently Asked Questions (FAQs)
Is JavaScript pass by reference or pass by value?
JavaScript primarily uses pass by value for primitive types (e.g., numbers, strings, booleans) and pass by reference for objects and arrays. When an object is passed to a function, a reference to that object is passed, allowing modifications to the original object.
What happens when you pass an object to a function in JavaScript?
When you pass an object to a function, the function receives a reference to the original object. Any changes made to the object within the function affect the original object outside the function.
Can you demonstrate pass by reference with an example?
Certainly. For example, if you have an object `const obj = { name: ‘Alice’ };` and pass it to a function that modifies `obj.name`, the change will reflect in the original object since the reference was passed.
Are arrays treated differently than objects in JavaScript?
No, arrays are also objects in JavaScript. Therefore, when you pass an array to a function, it is passed by reference, allowing the function to modify the original array.
What are the implications of pass by reference in JavaScript?
The implications include potential side effects, where a function can unintentionally alter the state of objects or arrays passed to it. This behavior necessitates careful management of data to avoid unexpected changes.
How can I prevent unintentional modifications to an object in JavaScript?
To prevent unintentional modifications, you can create a shallow copy of the object using methods like `Object.assign()` or the spread operator `{…originalObject}`. This way, the function operates on a copy rather than the original object.
JavaScript is primarily a pass-by-value language, but it exhibits behaviors that can sometimes create confusion regarding pass-by-reference. When dealing with primitive data types, such as numbers, strings, and booleans, JavaScript passes a copy of the value to functions. This means that modifications made to the parameter within the function do not affect the original variable outside the function.
In contrast, when working with objects and arrays, JavaScript passes a reference to the value rather than the actual value itself. This means that if an object is modified within a function, the changes will be reflected in the original object outside the function. However, it is crucial to understand that the reference itself is passed by value, meaning that reassigning the parameter to a new object will not affect the original object.
Key takeaways from this discussion include the importance of recognizing the distinction between primitive types and reference types in JavaScript. Understanding this difference is essential for effective programming and avoiding unintended side effects. Additionally, developers should be mindful of how object mutations can impact the state of their applications, especially in larger codebases where data integrity is critical.
Author Profile
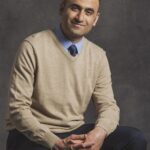
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?