Is ‘Not Equal To’ in Python the Same as in Other Programming Languages?
In the world of programming, the nuances of syntax can often lead to confusion, especially for those new to coding. One common point of contention arises with the concept of inequality in Python. While many languages utilize a variety of symbols to denote “not equal to,” Python has its own unique approach that can be both intuitive and perplexing for developers. Understanding how Python handles inequality is crucial for writing effective code and avoiding common pitfalls. This article delves into the intricacies of the “not equal to” operator in Python, exploring its syntax, functionality, and the implications of its use in various programming scenarios.
Overview
At its core, the “not equal to” operator in Python serves a fundamental purpose: it allows developers to compare values and determine if they differ. This operator is essential in conditional statements, loops, and functions, where making decisions based on the equality or inequality of values is a routine necessity. However, Python’s implementation of this operator, represented by `!=`, can sometimes lead to unexpected behaviors, especially when dealing with different data types or complex objects.
Moreover, understanding the context in which the “not equal to” operator is employed can significantly impact the efficiency and readability of code. As developers navigate through comparisons, they must also consider how Python
Understanding Inequality in Python
In Python, the concept of inequality is represented by the `!=` operator. This operator allows developers to compare two values and determine if they are not equal. When the values on either side of the `!=` operator differ, the expression evaluates to `True`; otherwise, it evaluates to “.
For example:
“`python
a = 5
b = 10
result = a != b This will be True
“`
It’s important to note that Python also provides the `is not` operator, which serves a different purpose. While `!=` checks for value equality, `is not` checks for identity, meaning it determines whether two references point to different objects in memory.
Key Differences Between `!=` and `is not`
Understanding the distinction between these two operators is crucial for effective programming in Python. Here are the primary differences:
- Purpose:
- `!=` checks for value inequality.
- `is not` checks for object identity.
- Usage:
- `!=` can be used with any data type.
- `is not` is primarily used with objects, especially in cases involving singleton objects like `None`.
- Return Type:
- Both operators return a Boolean value (`True` or “).
Examples of Usage
To illustrate these differences, consider the following examples:
“`python
Using !=
x = [1, 2, 3]
y = [1, 2, 3]
print(x != y) Outputs: True, as the lists are different objects
Using is not
print(x is not y) Outputs: True, as x and y are different objects in memory
Using with None
z = None
print(z is not None) Outputs:
“`
When to Use Each Operator
Choosing the right operator depends on the context of the comparison:
Operator | When to Use | Example |
---|---|---|
`!=` | When you care about the value | `if a != b:` |
`is not` | When you care about the object identity | `if obj is not None:` |
Common Pitfalls
When working with these operators, developers often encounter several common pitfalls:
- Immutable vs. Mutable Types: Be cautious with mutable types (like lists or dictionaries) when using `is not`, as two different instances can have the same content but are not the same object.
- Singletons: Use `is not` for comparisons against singletons (e.g., `None`, `True`, “) to avoid unexpected behavior.
- Overriding Equality: If a class overrides the equality operator (`__eq__`), the behavior of `!=` will be affected, whereas `is not` will still check for object identity.
In summary, while both `!=` and `is not` serve to evaluate inequality, understanding their distinct roles is vital for writing robust and error-free Python code.
Understanding the Concept of “Not Equal To” in Python
In Python, the notion of inequality is expressed using the `!=` operator. This operator is fundamental in performing comparisons between values, determining whether they are not equal to one another. Below are key points regarding its functionality:
- Basic Syntax: The syntax for using the `!=` operator is straightforward. You simply place it between two values or variables you wish to compare.
“`python
a = 5
b = 10
if a != b:
print(“a is not equal to b”)
“`
- Comparison Types: The `!=` operator can compare various data types, including:
- Integers
- Floats
- Strings
- Lists and Tuples
- Custom Objects (if the `__ne__` method is defined)
- Return Values: The operator returns a Boolean value:
- `True` if the values are not equal.
- “ if the values are equal.
Detailed Examples of Using “Not Equal To”
To illustrate the use of the `!=` operator, consider the following examples that cover different data types:
“`python
Integer comparison
x = 7
y = 8
print(x != y) Output: True
String comparison
str1 = “hello”
str2 = “world”
print(str1 != str2) Output: True
List comparison
list1 = [1, 2, 3]
list2 = [1, 2, 4]
print(list1 != list2) Output: True
Tuple comparison
tuple1 = (1, 2)
tuple2 = (1, 2, 3)
print(tuple1 != tuple2) Output: True
“`
Common Pitfalls When Using “Not Equal To”
While using the `!=` operator is straightforward, there are some common pitfalls to be aware of:
- Type Comparison: Comparing different data types can lead to unexpected results. For instance, comparing an integer to a string will always evaluate to `True`.
“`python
print(5 != “5”) Output: True
“`
- Floating Point Precision: When comparing floating-point numbers, be cautious of precision issues. Small differences in representation can lead to unexpected `True` results.
“`python
a = 0.1 + 0.2
b = 0.3
print(a != b) Output: True (due to floating-point precision)
“`
Comparison in Complex Data Structures
When using the `!=` operator with complex data structures, such as dictionaries or sets, it is essential to understand how Python evaluates equality:
Data Structure | Behavior of `!=` |
---|---|
Dictionary | Compares keys and values. Two dictionaries are not equal if they have different keys or values. |
Set | Compares the unique elements. Two sets are not equal if their contents differ. |
Custom Objects | Requires implementation of the `__ne__` method for meaningful comparison. |
“`python
Dictionary comparison
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘a’: 1, ‘b’: 3}
print(dict1 != dict2) Output: True
Set comparison
set1 = {1, 2, 3}
set2 = {1, 2, 4}
print(set1 != set2) Output: True
“`
Performance Considerations
In performance-sensitive applications, consider the following:
- Time Complexity: The time complexity of the `!=` operator varies based on the data structure being compared. For example, comparing two lists is `O(n)` in the worst case.
- Short-Circuit Evaluation: In logical expressions, Python evaluates conditions in order and short-circuits as soon as it determines the outcome. This can improve performance in certain scenarios.
Using the `!=` operator in Python is a powerful tool for controlling the flow of logic in your applications, and understanding its nuances can enhance your programming proficiency.
Understanding Inequality in Python Programming
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “In Python, the ‘is not equal to’ operator is represented by ‘!=’. This operator is crucial for comparing values, and understanding its application is fundamental for effective coding in Python.”
Michael Rodriguez (Lead Python Developer, CodeCraft Solutions). “When working with conditional statements in Python, using ‘!=’ allows developers to create more dynamic and responsive applications. It’s essential to grasp how this operator interacts with different data types.”
Sarah Patel (Python Educator, LearnPythonToday). “Many beginners confuse ‘!=’ with ‘is not’, which can lead to logical errors in their code. Clarifying these distinctions is vital for anyone looking to master Python programming.”
Frequently Asked Questions (FAQs)
What does “is not equal to” mean in Python?
In Python, “is not equal to” is represented by the operator `!=`. It is used to compare two values or expressions, returning `True` if they are not equal and “ if they are equal.
How does “is not equal to” differ from “is not” in Python?
The `!=` operator checks for value inequality, while the `is not` keyword checks for identity, determining if two references point to different objects in memory.
Can you provide an example of “is not equal to” in Python?
Certainly. For example, `5 != 3` evaluates to `True`, while `5 != 5` evaluates to “, indicating that the first comparison is true and the second is .
Is “is not equal to” case-sensitive in Python?
Yes, string comparisons using `!=` are case-sensitive. For example, `”Hello” != “hello”` returns `True` because the capitalization differs.
What data types can be compared using “is not equal to” in Python?
The `!=` operator can be used to compare various data types, including integers, floats, strings, lists, tuples, and custom objects, as long as the data types support comparison.
Are there any performance considerations when using “is not equal to” in Python?
Generally, the performance impact of using `!=` is minimal for basic data types. However, for complex objects or large data structures, the implementation of the comparison method can affect performance.
In Python, the concept of “not equal to” is represented by the operator `!=`. This operator is fundamental in programming, allowing developers to compare two values and determine if they are different. The use of `!=` is prevalent in conditional statements, loops, and functions, making it an essential tool for implementing logic in Python code. Understanding how to effectively use this operator is crucial for writing accurate and efficient programs.
Moreover, Python’s flexibility with data types enhances the functionality of the `!=` operator. It can be used to compare integers, floats, strings, lists, and other data types, enabling developers to create dynamic and versatile applications. This versatility is one of the reasons why Python is favored for various programming tasks, from simple scripts to complex web applications.
Additionally, it is important to note that Python also provides the `is not` operator, which serves a different purpose. While `!=` checks for value inequality, `is not` checks for identity, determining whether two references point to different objects in memory. This distinction is vital for developers to understand, as it can affect the behavior of their code, particularly when dealing with mutable and immutable data types.
In summary, the “not equal
Author Profile
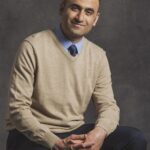
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?