How Can You Easily Convert an Int to a Double in Java?
In the world of programming, data types play a crucial role in how we manipulate and store information. Java, a widely-used programming language, offers a rich set of data types, each serving its unique purpose. Among these, integers and doubles are fundamental, representing whole numbers and decimal values, respectively. However, as developers often encounter scenarios where they need to convert one data type to another, understanding how to seamlessly transition from an `int` to a `double` becomes essential. Whether you’re working on a complex algorithm, performing calculations, or simply trying to ensure precision in your applications, mastering this conversion can enhance your coding efficiency and accuracy.
Converting an integer to a double in Java is a straightforward process, yet it is vital to grasp the underlying principles to avoid potential pitfalls. The language’s type system allows for implicit and explicit conversions, each with its own implications. While an implicit conversion may occur automatically, an explicit cast can provide greater control over how the data is transformed. Understanding these nuances not only helps in writing cleaner code but also aids in optimizing performance, especially in applications that require frequent data type conversions.
As we delve deeper into this topic, we will explore the various methods available for converting integers to doubles in Java. From simple casting techniques to more advanced
Understanding Type Conversion in Java
In Java, type conversion is a fundamental concept that allows developers to convert one data type into another. The process is particularly relevant when dealing with numerical data types, such as converting an integer (`int`) to a double (`double`). This conversion is essential when precision is required, as a double can represent fractional values, unlike an int.
Methods to Convert int to double
There are several straightforward methods to convert an `int` to a `double` in Java. Each method has its use case depending on the context of the code.
- Implicit Conversion: Java automatically converts `int` to `double` when an int is assigned to a double variable.
- Explicit Conversion: You can also explicitly cast an `int` to a `double` using a cast operator.
The following table illustrates these methods:
Method | Description | Example |
---|---|---|
Implicit Conversion | Automatic conversion during assignment. |
|
Explicit Conversion | Using casting to convert int to double. |
|
Code Examples
Here are examples demonstrating both implicit and explicit conversion of an `int` to a `double`:
“`java
public class ConversionExample {
public static void main(String[] args) {
// Implicit conversion
int intValue = 10;
double doubleValueImplicit = intValue; // No cast required
System.out.println(“Implicit conversion: ” + doubleValueImplicit);
// Explicit conversion
double doubleValueExplicit = (double) intValue; // Cast required
System.out.println(“Explicit conversion: ” + doubleValueExplicit);
}
}
“`
The output of the code will be:
“`
Implicit conversion: 10.0
Explicit conversion: 10.0
“`
Both methods yield the same result, showcasing how the integer value is effectively represented as a double.
Performance Considerations
While the conversion from `int` to `double` is generally efficient, it is essential to consider the following factors:
- Performance Overhead: Implicit conversions are typically optimized by the Java compiler, while explicit conversions may introduce slight overhead due to the casting operation.
- Precision: When converting larger integers, it’s crucial to ensure that the resulting double can accurately represent the integer value without loss of precision.
- Use Cases: Use implicit conversion where possible for cleaner code. Reserve explicit conversion for scenarios where clarity is paramount.
By understanding these methods and considerations, developers can effectively manage data type conversions in their Java applications.
Methods to Convert int to double in Java
In Java, converting an `int` to a `double` can be done using several straightforward methods. Here are the primary approaches:
- Implicit Casting
- Explicit Casting
- Using Wrapper Classes
Implicit Casting
Java supports implicit casting, where an `int` can be automatically converted to a `double` without requiring any special syntax. This occurs because a `double` has a larger range and can accommodate the value of an `int`.
“`java
int intValue = 10;
double doubleValue = intValue; // Implicit conversion
“`
In this example, the `int` value of `10` is automatically promoted to a `double`, resulting in `10.0`.
Explicit Casting
Though not necessary for converting `int` to `double`, you can still use explicit casting for clarity. This is done by specifying the type conversion.
“`java
int intValue = 20;
double doubleValue = (double) intValue; // Explicit conversion
“`
This method provides a clear indication of the conversion, making the code more readable for those unfamiliar with implicit casting.
Using Wrapper Classes
Java’s wrapper classes provide another method to convert an `int` to a `double`. This can be particularly useful in situations where you need to perform conversions with objects.
“`java
int intValue = 30;
Double doubleValue = Double.valueOf(intValue); // Using wrapper class
“`
Here, the `Double.valueOf()` method takes an `int` and returns a `Double` object, which can be useful in collections or APIs that require objects.
Comparison of Methods
Method | Syntax | Returns | Notes |
---|---|---|---|
Implicit Casting | `doubleValue = intValue;` | Primitive `double` | Automatic conversion, no additional syntax. |
Explicit Casting | `doubleValue = (double) intValue;` | Primitive `double` | Clearer, but not necessary for `int` to `double`. |
Wrapper Class | `Double doubleValue = Double.valueOf(intValue);` | `Double` object | Useful for working with objects instead of primitives. |
Best Practices
When converting an `int` to a `double`, consider the following best practices:
- Use implicit casting for simplicity unless clarity is a priority.
- Use explicit casting if the code may be read by less experienced developers or if it enhances understanding.
- Opt for wrapper classes when working with APIs that require objects, such as collections.
- Always be mindful of the performance implications when using wrapper classes due to boxing and unboxing.
These conversion methods are efficient and integral to Java programming, allowing developers to handle numerical data effectively across various applications.
Expert Insights on Converting Integers to Doubles in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting an integer to a double in Java, it is essential to utilize the implicit type conversion that Java provides. This allows for a straightforward transition without loss of data, as the double type can represent all integer values accurately.”
James Liu (Java Development Consultant, CodeCrafters). “While converting int to double in Java is generally safe, developers should be aware of the potential for precision loss in more complex calculations. It is advisable to perform such conversions in a controlled manner to maintain numerical integrity.”
Sarah Thompson (Lead Java Architect, FutureTech Solutions). “Using explicit casting is often recommended for clarity, even though Java performs automatic conversion. This practice not only enhances code readability but also mitigates potential errors during arithmetic operations involving mixed data types.”
Frequently Asked Questions (FAQs)
How do you convert an int to a double in Java?
You can convert an int to a double in Java by simply assigning the int value to a double variable. Java performs an implicit type conversion. For example: `int num = 5; double dNum = num;`.
Is explicit casting necessary when converting int to double in Java?
No, explicit casting is not necessary when converting an int to a double in Java, as the conversion is done implicitly. However, if you were converting from double to int, explicit casting would be required.
What happens to the value when converting an int to a double?
When converting an int to a double, the integer value is preserved, and it is represented in floating-point format. For example, the int value `5` becomes `5.0` as a double.
Can you convert an array of ints to an array of doubles in Java?
Yes, you can convert an array of ints to an array of doubles by iterating through the int array and assigning each value to a new double array. For example:
“`java
int[] intArray = {1, 2, 3};
double[] doubleArray = new double[intArray.length];
for (int i = 0; i < intArray.length; i++) {
doubleArray[i] = intArray[i];
}
```
Are there performance implications when converting int to double in Java?
Yes, there can be performance implications due to the additional memory overhead and processing required for floating-point arithmetic. However, for most applications, this overhead is negligible.
Does converting an int to a double affect precision?
No, converting an int to a double does not affect precision, as all integer values can be accurately represented in double format. However, when converting from double to int, precision may be lost due to truncation.
In Java, converting an integer to a double is a straightforward process that can be accomplished in several ways. The most common method involves using type casting, where the integer is explicitly cast to a double type. This conversion is essential in scenarios where arithmetic operations require a higher precision that doubles provide compared to integers. Additionally, Java’s automatic type promotion during arithmetic operations can also facilitate this conversion without the need for explicit casting.
Another approach to convert an integer to a double is by using the Double.valueOf() method, which creates a Double object from the integer. This method is particularly useful when dealing with collections or APIs that require objects instead of primitive types. It is important to note that while both methods effectively convert the integer to a double, the choice between them may depend on the specific requirements of the application and performance considerations.
Overall, understanding how to convert integers to doubles in Java is a fundamental skill for developers. It not only aids in performing calculations with greater precision but also enhances the ability to work with various data types seamlessly. Mastering these conversion techniques is crucial for writing efficient and effective Java code.
Author Profile
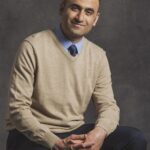
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?