Why Am I Seeing ‘java.lang.IllegalArgumentException: Invalid Character Found in Method Name’?
In the world of Java programming, exceptions are an inevitable part of the development process, serving as crucial indicators of underlying issues in code execution. Among these, the `java.lang.IllegalArgumentException` stands out as a common yet perplexing error that developers encounter, particularly when dealing with method names. The message “invalid character found in method name” can strike fear into the hearts of even seasoned programmers, signaling that something has gone awry in their code. Understanding this exception is essential for any Java developer aiming to write clean, efficient, and error-free code.
This article delves into the intricacies of the `IllegalArgumentException`, focusing specifically on the scenarios that lead to the “invalid character” error. We will explore the common pitfalls that can cause this exception to be thrown, shedding light on how method names are parsed and validated within the Java environment. By examining the underlying principles of method naming conventions and the significance of adhering to these rules, we will equip developers with the knowledge they need to troubleshoot and resolve this frustrating issue.
As we journey through the nuances of this exception, we will also discuss best practices for naming methods, offering insights that can help prevent such errors from occurring in the first place. Whether you’re a novice programmer or a seasoned Java expert,
Understanding the Exception
The `java.lang.IllegalArgumentException: invalid character found in method name` typically indicates that the Java compiler has encountered an unexpected character in a method name within your code. This situation can arise from various coding errors, and understanding the underlying causes can help you quickly resolve the issue.
Common sources of this exception include:
- Incorrect Characters: Method names should only consist of letters, digits, underscores, and dollar signs. Any other characters, such as spaces, punctuation, or special symbols, will trigger this exception.
- Copy-Paste Errors: Sometimes, copying code from external sources may inadvertently introduce hidden or non-printable characters.
- Misconfigured IDE Settings: Integrated Development Environments (IDEs) may have settings that affect how method names are parsed or checked, leading to unexpected errors.
Examples of Invalid Method Names
To clarify what constitutes an invalid method name, consider the following examples:
Method Name | Validity |
---|---|
`calculateTotal()` | Valid |
`sumnumbers()` | Invalid |
`get user info()` | Invalid |
`setValue$()` | Valid |
`doSomething@Home()` | Invalid |
In the examples above, the method names `sumnumbers()`, `get user info()`, and `doSomething@Home()` contain invalid characters or spaces, which will lead to the `IllegalArgumentException`.
Troubleshooting Steps
When faced with this exception, follow these troubleshooting steps to identify and fix the issue:
- Check Method Names: Review the names of all your methods for any invalid characters or spaces.
- Look for Invisible Characters: Use a text editor that can display hidden characters to identify any non-printable characters.
- Refactor Code: If you find invalid characters, refactor your method names to comply with Java naming conventions.
- IDE Analysis: Utilize your IDE’s built-in analysis tools to identify any coding standard violations that may not be immediately visible.
Best Practices for Method Naming
Following best practices for naming methods can help prevent this exception from occurring in the first place:
- Use Camel Case: Start with a lowercase letter and use camel case for subsequent words, e.g., `calculateTotalPrice()`.
- Avoid Reserved Keywords: Do not use Java reserved keywords as method names.
- Descriptive Names: Choose names that describe the method’s function clearly, enhancing code readability.
- Consistent Prefixes: Use consistent prefixes for similar methods (e.g., `get`, `set`, `calculate`) to maintain clarity.
By adhering to these guidelines, you can avoid common pitfalls that lead to the `IllegalArgumentException` and improve the overall quality of your Java code.
Understanding the Error
The `java.lang.IllegalArgumentException: invalid character found in method name` typically arises when there is an attempt to invoke a method with an improperly formatted name. This can occur in various scenarios, particularly when using reflection or when dynamically generating method names.
Common Causes
- Invalid Characters: Method names must follow specific naming conventions. They should start with a letter, and can contain letters, digits, underscores, and dollar signs. Any deviation can trigger this error.
- Whitespace: Spaces or other whitespace characters in method names will lead to this exception.
- Special Characters: Characters such as `@`, “, `!`, or any other non-alphanumeric symbols are not allowed in method names.
Example Scenarios
To illustrate how this exception can occur, consider the following examples:
Reflection Usage
When using Java Reflection, ensure that method names are valid:
“`java
Method method = obj.getClass().getMethod(“invalid method name”); // Throws IllegalArgumentException
“`
Dynamic Method Generation
When dynamically generating method names, check the input for validity:
“`java
String methodName = “someMethod@name”; // Invalid due to ‘@’
obj.getClass().getMethod(methodName);
“`
Valid Method Name Examples
Valid Name | Description |
---|---|
`myMethod` | Starts with a letter and contains only letters. |
`method_1` | Contains a letter and an underscore. |
`$specialMethod` | Starts with a dollar sign, valid in Java. |
Invalid Method Name Examples
Invalid Name | Reason |
---|---|
`2ndMethod` | Starts with a digit. |
`method name` | Contains a space. |
`methodname` | Contains a special character. |
Debugging Steps
To resolve this issue, consider the following debugging steps:
- Check Method Name: Verify that the method name adheres to Java’s naming conventions.
- Log Method Names: If method names are generated dynamically, log them to identify any invalid characters before invocation.
- Use Regular Expressions: Implement validation using regex to ensure that method names meet the criteria:
“`java
String regex = “^[a-zA-Z_$][a-zA-Z\\d_$]*$”;
if (!methodName.matches(regex)) {
throw new IllegalArgumentException(“Invalid method name: ” + methodName);
}
“`
Best Practices
To avoid encountering this exception in the future, adhere to these best practices:
- Follow Naming Conventions: Always use valid Java naming conventions for method names.
- Validate Input: If method names are provided as input, validate them before attempting to use reflection.
- Error Handling: Implement robust error handling to catch and respond to `IllegalArgumentException` gracefully, providing informative error messages.
By applying these practices, developers can minimize the risk of encountering `java.lang.IllegalArgumentException: invalid character found in method name` and ensure smoother operation of Java applications.
Understanding the Causes of java.lang.IllegalArgumentException in Java Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘java.lang.IllegalArgumentException: invalid character found in method name’ typically arises when developers inadvertently include special characters or whitespace in method names. It is crucial to adhere to Java’s naming conventions, which only allow alphanumeric characters and underscores.”
Michael Chen (Java Development Consultant, CodeCraft Solutions). “This exception serves as a reminder of the importance of code validation. Implementing static analysis tools can help catch these errors early in the development process, thereby preventing runtime exceptions that can disrupt application flow.”
Sarah Thompson (Lead Java Architect, Future Tech Labs). “When encountering this exception, it is advisable to review the method definitions thoroughly. Often, a simple oversight in naming can lead to significant debugging time, which can be mitigated by adhering to best practices in code reviews.”
Frequently Asked Questions (FAQs)
What does the error `java.lang.IllegalArgumentException: invalid character found in method name` mean?
This error indicates that a method name in your Java code contains characters that are not allowed according to Java’s naming conventions, such as spaces, special symbols, or starting with a number.
What are the valid characters for method names in Java?
Valid characters for method names in Java include letters (a-z, A-Z), digits (0-9), underscores (_), and dollar signs ($). However, method names cannot start with a digit and should not contain spaces or special characters.
How can I fix the `java.lang.IllegalArgumentException: invalid character found in method name` error?
To resolve this error, review the method names in your code and ensure they comply with Java’s naming conventions. Rename any methods that contain invalid characters.
Are there specific tools to help identify invalid method names in Java?
Yes, integrated development environments (IDEs) like IntelliJ IDEA or Eclipse provide real-time syntax checking and will highlight invalid method names, making it easier to identify and correct errors.
Can this error occur in frameworks or libraries that use reflection?
Yes, this error can occur in frameworks or libraries that utilize reflection to invoke methods. If the method names passed to these frameworks contain invalid characters, it will result in this exception.
Is there a difference between method name restrictions in Java and other programming languages?
Yes, different programming languages have varying rules for method naming. For instance, Python allows underscores and does not restrict method names as strictly as Java, while languages like Chave similar conventions to Java.
The `java.lang.IllegalArgumentException: invalid character found in method name` error typically arises in Java when a method name contains characters that are not permissible according to the Java Language Specification. This exception indicates that the method name violates the naming conventions established for Java identifiers, which must begin with a letter, underscore, or dollar sign, and can only contain letters, digits, underscores, or dollar signs thereafter. Understanding these conventions is crucial for developers to avoid such errors in their code.
Common causes of this exception include accidental inclusion of special characters, spaces, or punctuation in method names. Such mistakes can occur during manual coding or when generating code dynamically. To resolve this issue, developers should carefully review their method declarations and ensure that they adhere to the proper naming conventions. Utilizing IDE features that highlight syntax errors can also aid in identifying and correcting these issues before compilation.
In summary, the `IllegalArgumentException` related to invalid characters in method names serves as a reminder of the importance of following Java’s naming conventions. By maintaining proper naming practices, developers can enhance code readability and maintainability while minimizing the likelihood of encountering such exceptions. Adopting a disciplined approach to naming methods will ultimately contribute to more robust and error-free Java applications.
Author Profile
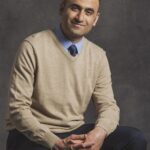
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?