How Can You Resolve Java Net ConnectException: Connection Timed Out Issues?
In the world of Java programming, few errors can be as frustrating as the dreaded `java.net.ConnectException: Connection timed out`. This seemingly cryptic message can halt your application in its tracks, leaving developers scratching their heads and searching for answers. Whether you’re a seasoned programmer or just starting your journey in Java, understanding the nuances of this error is crucial for building robust networked applications. In this article, we will delve into the intricacies of connection timeouts, exploring their causes, implications, and the best practices to troubleshoot and resolve them effectively.
Overview
At its core, a `ConnectException` signals that your Java application is struggling to establish a connection to a remote server or service. This timeout can occur for various reasons, including network issues, server unavailability, or incorrect configurations. As applications become increasingly reliant on network communication, recognizing the telltale signs of a connection timeout is essential for maintaining seamless user experiences and operational efficiency.
Understanding the underlying factors that contribute to connection timeouts can empower developers to implement proactive measures. By mastering the art of diagnosing and resolving these issues, you can enhance your application’s resilience and ensure that it communicates effectively with external systems. Join us as we unravel the complexities of `java.net.ConnectException` and equip you with
Understanding Connection Timeout Errors
A `java.net.ConnectException` indicating a “connection timed out” typically occurs when a Java application attempts to establish a connection to a server but fails to do so within a specified time frame. This error is commonly associated with network issues, server unavailability, or incorrect connection parameters.
Several factors can lead to this type of error:
- Server Unavailability: The target server may be down or not reachable due to maintenance or unexpected outages.
- Network Issues: Problems such as routing issues, firewall restrictions, or network congestion can prevent successful connections.
- Incorrect Configuration: Misconfigured hostnames or port numbers can lead to connection attempts directed at the wrong address.
- Timeout Settings: The timeout settings in the Java application may be too low, causing premature termination of connection attempts.
Troubleshooting Steps
To effectively troubleshoot a `ConnectException`, follow these systematic steps:
- Check Server Status: Verify whether the target server is running and accessible from your network.
- Ping the Server: Use the command line to ping the server’s IP address or hostname to confirm connectivity.
- Review Network Configuration: Ensure that there are no firewall rules or network policies blocking the connection.
- Examine Application Configuration: Double-check that the hostname and port are correctly specified in your application settings.
- Adjust Timeout Settings: Consider increasing the timeout settings in your code to allow for longer connection attempts.
Sample Code for Connection Handling
Here is a simple example of how to handle connections in Java while implementing a timeout:
“`java
import java.net.InetSocketAddress;
import java.net.Socket;
public class ConnectionExample {
public static void main(String[] args) {
String host = “example.com”;
int port = 80;
int timeout = 5000; // 5 seconds
try (Socket socket = new Socket()) {
socket.connect(new InetSocketAddress(host, port), timeout);
System.out.println(“Connection successful!”);
} catch (java.net.ConnectException e) {
System.err.println(“Connection timed out: ” + e.getMessage());
} catch (Exception e) {
System.err.println(“An error occurred: ” + e.getMessage());
}
}
}
“`
Common Solutions
If you encounter a connection timeout, consider the following solutions:
Issue | Solution |
---|---|
Server Down | Contact server administrator or check server status. |
Network Restrictions | Review firewall settings and network policies. |
Incorrect Host/Port | Verify the connection details in your application. |
Low Timeout Value | Increase the timeout duration in your code. |
By following these troubleshooting steps and solutions, developers can effectively manage and resolve `ConnectException` issues in their Java applications, ensuring smoother network interactions.
Understanding Connection Timeout Exceptions
A `java.net.ConnectException` indicating a connection timed out is a common issue encountered when a Java application attempts to connect to a remote server. This exception typically signifies that the connection could not be established within a specified timeout duration.
Causes of Connection Timeout Exceptions
Several factors can lead to a connection timeout:
- Network Configuration Issues: Firewalls, routers, or proxies might block the connection.
- Server Unavailability: The target server may be down or unreachable.
- DNS Resolution Failures: The domain name may not resolve correctly to an IP address.
- Incorrect Endpoint: The URL or IP address used for the connection might be incorrect.
- Heavy Network Traffic: High traffic can delay packets, resulting in timeouts.
- Misconfigured Client: Timeout settings on the client side may be too short.
Troubleshooting Steps
To resolve a `ConnectException` with a timeout, consider the following steps:
- Verify Server Status: Ensure the target server is operational by pinging it or using tools like `curl`.
- Check Network Connectivity: Confirm that your network is functioning properly and that you can access the internet.
- Inspect Firewalls: Review firewall settings on both the client and server sides to ensure that the necessary ports are open.
- DNS Settings: Check the DNS settings to ensure that domain names are resolving correctly.
- Adjust Timeout Settings: Increase the timeout duration in your connection settings. For example, in Java, you can set it using `URLConnection.setConnectTimeout(int timeout)`.
Example Code Snippet
Below is an example of how to set a timeout in a Java application:
“`java
import java.net.HttpURLConnection;
import java.net.URL;
public class ConnectionExample {
public static void main(String[] args) {
try {
URL url = new URL(“http://example.com”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000); // Timeout in milliseconds
connection.connect();
System.out.println(“Connection established successfully.”);
} catch (java.net.ConnectException e) {
System.out.println(“Connection timed out: ” + e.getMessage());
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Configuration Recommendations
For optimal connectivity, consider the following configuration practices:
Configuration Element | Recommendation |
---|---|
Connection Timeout | Set to a reasonable duration (e.g., 5000 ms) based on expected response time. |
Retries | Implement retry logic with exponential backoff to handle transient issues. |
Logging | Enable detailed logging to capture connection attempts and failures for analysis. |
By following these practices and troubleshooting steps, you can effectively manage and mitigate `java.net.ConnectException` occurrences in your Java applications.
Understanding Java Network Connection Issues
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A `java.net.ConnectException: Connection timed out` error typically indicates that the client is unable to establish a connection to the server within the specified timeout period. This can be due to various reasons, including network issues, server unavailability, or incorrect configuration settings.”
Michael Tran (Network Architect, Global Tech Solutions). “When encountering a connection timeout in Java, it is crucial to examine both the client and server configurations. Firewalls, proxy settings, and incorrect IP addresses can all contribute to this issue. A thorough network diagnosis is often necessary to pinpoint the root cause.”
Sarah Johnson (Java Development Specialist, CodeCraft Labs). “To mitigate the chances of a `ConnectException`, developers should implement retry logic and increase the timeout settings where appropriate. Additionally, utilizing tools like Wireshark can help analyze network traffic to identify potential bottlenecks.”
Frequently Asked Questions (FAQs)
What does “java.net.ConnectException: Connection timed out” mean?
This exception indicates that a connection attempt to a specified host and port has failed due to a timeout. It typically occurs when the server is unreachable or not responding within the expected timeframe.
What are common causes of a connection timeout in Java?
Common causes include network issues, incorrect server addresses, firewall settings blocking the connection, server overload, or the server being down.
How can I troubleshoot a “Connection timed out” error in Java?
To troubleshoot, check the server address and port for correctness, verify network connectivity, review firewall rules, and ensure the server is operational. Additionally, consider increasing the timeout duration in your code.
Can a proxy server affect the connection timeout in Java applications?
Yes, if a proxy server is misconfigured or down, it can lead to connection timeouts. Ensure that the proxy settings are correct and that the proxy server is functioning properly.
Is it possible to handle a “Connection timed out” exception in Java?
Yes, you can handle this exception using a try-catch block in your code. This allows you to implement custom logic, such as retrying the connection or logging the error for further analysis.
What is the default timeout duration for socket connections in Java?
The default timeout duration for socket connections in Java is typically set to zero, meaning it will wait indefinitely. However, you can specify a timeout using the `setSoTimeout(int timeout)` method on a socket.
In summary, the “java.net.ConnectException: Connection timed out” error is a common issue encountered in Java applications when attempting to establish a network connection. This exception typically indicates that the application is unable to reach the specified server or service within a designated timeout period. Several factors can contribute to this problem, including network configuration issues, firewall restrictions, incorrect server addresses, or server unavailability. Understanding these potential causes is essential for troubleshooting and resolving the error effectively.
Key takeaways from the discussion include the importance of verifying network connectivity and ensuring that the target server is operational. Developers should check their application’s configuration settings, including the server address and port number, to confirm they are correct. Additionally, examining firewall settings and network policies can help identify any restrictions that may be blocking the connection. Implementing proper exception handling in the code can also provide more informative error messages, aiding in quicker diagnosis and resolution of connectivity issues.
Ultimately, addressing the “java.net.ConnectException: Connection timed out” error requires a systematic approach to identify and rectify the underlying causes. By being proactive in network management and maintaining clear communication with network administrators, developers can minimize the occurrence of such connectivity issues and enhance the reliability of their Java applications.
Author Profile
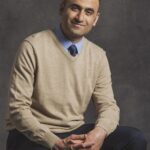
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?