How Can I Use Kubectl to Watch All Resources of a CRD in Golang?
In the dynamic world of Kubernetes, managing custom resources can often feel like an intricate dance of coordination and observation. As developers and operators, we strive to maintain a seamless flow of information about the state of our applications and their underlying resources. Enter `kubectl watch`, a powerful command that allows us to monitor changes in real-time. But what if you want to take this a step further and keep an eye on all resources of a Custom Resource Definition (CRD) in Golang? This article will guide you through the process, empowering you to harness the full potential of Kubernetes and Golang together.
Understanding how to effectively watch and manage CRDs is crucial for any Kubernetes user looking to extend the platform’s capabilities. With `kubectl`, you can observe changes in resource states, but when it comes to CRDs, the approach requires a bit more finesse. By leveraging the capabilities of Golang, you can create custom controllers that not only watch for changes but also react to them, ensuring your applications are always in sync with the desired state. This synergy between `kubectl` and Golang opens up a world of possibilities for automation and efficiency, making it an essential skill for modern cloud-native development.
As we delve into the intricacies of watching all resources associated with a CR
Watching Custom Resource Definitions (CRDs) with Kubectl
To effectively monitor all resources of a Custom Resource Definition (CRD) in Kubernetes using `kubectl`, you can utilize the `–watch` flag, which allows real-time observation of changes. This functionality is essential for developers and operators who need to be promptly notified of any state changes in their applications.
When using `kubectl` to watch CRDs, ensure that the CRDs are properly defined and registered within your Kubernetes cluster. Once confirmed, you can execute the following command to watch all instances of a specific CRD:
“`bash
kubectl get
“`
Replace `
Watching All CRD Resources
If you want to watch all resources defined under a specific CRD, you can extend the `kubectl` command. However, as of now, there is no direct `kubectl` command to watch all CRD instances simultaneously in a single command. Instead, you can use a script to achieve this.
Consider the following steps:
- List All CRDs: First, list all CRDs in your cluster:
“`bash
kubectl get crd
“`
- Watch Resources for Each CRD: Then, iterate through each CRD and watch its resources. Here’s a sample script in bash:
“`bash
for crd in $(kubectl get crd -o jsonpath='{.items[*].metadata.name}’); do
echo “Watching $crd”
kubectl get $crd –watch &
done
wait
“`
This script runs the watch command for each CRD in the background, allowing you to monitor all resources concurrently.
Using Go Client for Watching CRDs
For more advanced use cases, developers can leverage the Kubernetes Go client to create a custom application that watches CRD resources. The following steps outline how to set up a watcher using Go:
- Initialize Your Go Module:
“`bash
go mod init your-module-name
“`
- Import Required Packages:
Ensure you have the Kubernetes client-go and other dependencies:
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/apimachinery/pkg/watch”
“k8s.io/apimachinery/pkg/apis/meta/v1”
)
“`
- Set Up the Client:
“`go
kubeconfig := “path/to/kubeconfig”
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
panic(err.Error())
}
clientset, err := kubernetes.NewForConfig(config)
“`
- Watch the CRD:
“`go
watcher, err := clientset.CustomResourceDefinitions().Watch(context.TODO(), v1.ListOptions{})
if err != nil {
panic(err.Error())
}
for event := range watcher.ResultChan() {
fmt.Printf(“Event Type: %s, Object: %v\n”, event.Type, event.Object)
}
“`
This code snippet sets up a watcher for CRD events, printing the type of event and the object details to the console.
Key Considerations
When implementing a watch on CRDs, keep the following in mind:
- Performance: Continuously watching resources can lead to performance overhead, particularly in clusters with many resources.
- Error Handling: Ensure that your application handles errors gracefully, especially when dealing with network issues or API rate limits.
- Resource Cleanup: If you are spawning multiple watch processes, ensure proper cleanup to avoid resource leaks.
Aspect | Consideration |
---|---|
Performance | Monitor resource usage to avoid bottlenecks. |
Error Handling | Implement retries and backoff strategies. |
Resource Cleanup | Close watchers when no longer needed. |
By following these guidelines, you can effectively monitor CRD resources in your Kubernetes environment, ensuring that you remain informed about the state and health of your applications.
Watching All Resources of a Custom Resource Definition (CRD) in Kubernetes
To effectively watch all resources of a Custom Resource Definition (CRD) in Kubernetes using `kubectl`, you can leverage the power of the Kubernetes API, which provides mechanisms to monitor changes in the state of resources. Below are steps and considerations for implementing this using Go.
Setting Up the Environment
Before you start, ensure that you have the following prerequisites:
- Go installed on your machine.
- Kubectl configured to access your Kubernetes cluster.
- Client-go library for interacting with Kubernetes APIs.
To install the client-go library, run:
“`bash
go get k8s.io/client-go@latest
“`
Implementing Watch Functionality
You can create a simple Go application that watches all resources of a CRD. Below is a step-by-step guide to implement this.
- Import Necessary Packages:
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/apimachinery/pkg/watch”
“k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/client-go/kubernetes/scheme”
)
“`
- Create a Kubernetes Client:
Set up the Kubernetes client to interact with the cluster:
“`go
config, err := clientcmd.BuildConfigFromFlags(“”, “/path/to/kubeconfig”)
if err != nil {
panic(err.Error())
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err.Error())
}
“`
- Watch Custom Resources:
Assuming you have a CRD named `MyResource`, you can set up a watch:
“`go
watcher, err := clientset.RESTClient().
Get().
Resource(“myresources”).
Namespace(“default”).
Watch(context.TODO())
if err != nil {
panic(err.Error())
}
defer watcher.Stop()
for event := range watcher.ResultChan() {
switch event.Type {
case watch.Added:
fmt.Printf(“Added: %s\n”, event.Object)
case watch.Modified:
fmt.Printf(“Modified: %s\n”, event.Object)
case watch.Deleted:
fmt.Printf(“Deleted: %s\n”, event.Object)
}
}
“`
Considerations for Watching Resources
- Namespace Scope: Adjust the namespace in the watch call based on your requirements; use `””` for all namespaces.
- Error Handling: Implement robust error handling to manage connection issues or API errors.
- Resource Limits: Be cautious of API server limits on the number of watch requests. Ensure your application efficiently manages these resources.
- Testing: Verify the implementation by creating, modifying, and deleting instances of your CRD in the specified namespace.
Example CRD Definition
A sample CRD definition for `MyResource` might look like this:
“`yaml
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: myresources.example.com
spec:
group: example.com
names:
kind: MyResource
listKind: MyResourceList
plural: myresources
singular: myresource
scope: Namespaced
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
field1:
type: string
field2:
type: integer
“`
By following these guidelines and examples, you can effectively watch all resources of a CRD in your Kubernetes environment using Go.
Expert Insights on Monitoring Custom Resource Definitions with Kubectl
Dr. Emily Chen (Kubernetes Architect, Cloud Innovations Inc.). “Utilizing `kubectl` to watch all resources of a Custom Resource Definition (CRD) in Golang can significantly enhance your observability. By leveraging the `–watch` flag, developers can monitor changes in real-time, allowing for quicker responses to state changes in their Kubernetes clusters.”
James Patel (Senior DevOps Engineer, Tech Solutions Group). “To effectively watch all resources of a CRD using `kubectl`, one must ensure that the CRD is properly defined and registered within the Kubernetes API. This enables seamless integration with `kubectl` commands, facilitating ongoing monitoring and management of the resources.”
Linda Garcia (Kubernetes Consultant, Agile Cloud Services). “Implementing a watch on CRDs can be further enhanced by creating custom controllers in Golang. This allows for more complex logic and automated responses to events, making your Kubernetes environment more resilient and responsive to changes.”
Frequently Asked Questions (FAQs)
How can I watch all resources of a Custom Resource Definition (CRD) using kubectl?
You can watch all resources of a CRD by using the command `kubectl get
Is it possible to watch multiple CRDs simultaneously with kubectl?
Yes, you can watch multiple CRDs by running separate `kubectl get
What flags can enhance the kubectl watch command for CRDs?
You can use flags like `-n
Can I use kubectl watch with labels or annotations for CRDs?
Yes, you can use the `-l
What programming considerations are there when implementing a watcher for CRDs in Golang?
When implementing a watcher in Golang, you should utilize the client-go library, specifically the `watch` package, to handle events and manage resource updates efficiently.
Are there performance implications when watching all resources of a CRD?
Yes, watching all resources can lead to increased load on the API server and network traffic, especially with a high number of resources. It’s advisable to implement filtering to minimize performance overhead.
In the context of Kubernetes, the ability to watch all resources of a Custom Resource Definition (CRD) using `kubectl` is a significant feature that enhances the management and monitoring of custom resources. By leveraging the `kubectl` command-line tool, developers and operators can observe real-time changes to CRDs, facilitating better responsiveness to modifications in the cluster. This functionality is particularly useful when working with resources defined through Golang, as it allows for seamless integration and interaction with Kubernetes APIs.
Implementing a watch on CRDs can be accomplished through the use of the `kubectl get` command with the `–watch` flag, which enables users to track changes in resource states continuously. This capability is essential for applications that require immediate feedback on resource changes, such as monitoring systems, controllers, or operators built in Golang. Additionally, utilizing client-go libraries in Golang can further enhance the ability to programmatically watch and respond to changes in CRDs, allowing for more sophisticated handling of resource events.
Key takeaways from this discussion include the importance of real-time monitoring in Kubernetes environments, especially for custom resources. The integration of `kubectl` with Golang for watching CRDs not only streamlines operations but also empowers developers
Author Profile
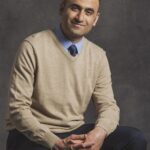
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?