How Can I Use Laravel 11 to Dump and Die All Request Data?
In the world of web development, debugging is an essential skill that can make or break a project. Laravel, a robust PHP framework, offers a plethora of tools to streamline this process, with the `dd()` (dump and die) function being one of the most popular among developers. As Laravel continues to evolve, with the latest version being Laravel 11, understanding how to effectively utilize `dd()` can significantly enhance your debugging experience. This article delves into the nuances of using `$request` with `dd()` to inspect all aspects of incoming data, ensuring you have the insights needed to address any issues that may arise in your applications.
When working with forms and APIs, the `$request` object in Laravel is your gateway to accessing user input and other request data. However, simply dumping the `$request` object may not always provide the clarity you need. By leveraging `dd()` in conjunction with the `$request` object, developers can gain a comprehensive view of the entire request lifecycle, including headers, query parameters, and payloads. This approach not only aids in identifying errors but also fosters a deeper understanding of how data flows through your application.
As we explore the best practices for using `dd()` with `$request`, we’ll uncover tips and techniques that can help
Understanding the dd() Function in Laravel 11
The `dd()` function in Laravel is a powerful debugging tool that stands for “dump and die.” It allows developers to quickly inspect variables or request data while halting the execution of the script. When you use `dd($request)`, it outputs the contents of the `$request` object, making it easier to debug issues related to incoming HTTP requests.
The `$request` object in Laravel encapsulates all the data from the client’s request, including:
- Input data (from forms)
- Query parameters
- Headers
- Cookies
- Files
By dumping the entire `$request` object, you can gain insights into what data is being sent to your application, facilitating easier troubleshooting.
Dumping All Request Data
To dump all request data effectively, you can use the `all()` method provided by the `$request` object. This method returns an array of all input data. You can combine it with `dd()` to output everything clearly.
“`php
dd($request->all());
“`
This command will display all the input data sent with the request, which is particularly useful for POST requests where multiple parameters are often involved.
Key Components of the Request Object
The `$request` object can be explored further to access specific components, such as:
– **Input Data**: Accessed via `$request->input(‘key’)` or `$request->all()`.
– **Headers**: Retrieved using `$request->headers`.
– **Method**: The HTTP method used in the request can be checked with `$request->method()`.
– **URL**: The current URL can be obtained using `$request->url()`.
Here’s a brief table summarizing the most common methods used with the `$request` object:
Method | Description |
---|---|
input($key) |
Retrieve a specific input value. |
all() |
Get all input data as an array. |
headers |
Access all headers of the request. |
method() |
Get the request method (GET, POST, etc.). |
url() |
Get the current URL of the request. |
This detailed exploration of the `$request` object with the `dd()` function enhances your debugging capabilities and ensures that you can effectively track down issues in your Laravel applications.
Understanding the `dd()` Function in Laravel 11
The `dd()` function in Laravel, which stands for “dump and die,” is a powerful tool for debugging. It outputs the contents of a variable and stops the execution of the script. When working with requests, developers often need to inspect the entire request object or specific parameters.
To utilize `dd()` effectively with the `$request` object in Laravel 11, consider the following:
- Basic Usage:
- To dump the entire request object:
“`php
dd($request);
“`
- This will provide a comprehensive view of all the data passed in the request, including query parameters, form data, cookies, and more.
Dumping Specific Data from the Request
When debugging, it may be more efficient to inspect specific parts of the request. Laravel provides methods to access these segments easily.
– **Accessing Input Data**:
- To dump all input data:
“`php
dd($request->all());
“`
- To dump a specific input field:
“`php
dd($request->input(‘field_name’));
“`
– **Accessing Query Parameters**:
- To dump all query parameters:
“`php
dd($request->query());
“`
– **Accessing Headers**:
- To dump request headers:
“`php
dd($request->headers->all());
“`
Using `dump()` for Continuous Execution
In scenarios where you want to examine the request without terminating the script, use the `dump()` function. This will allow the script to continue execution after outputting the data.
– **Example**:
“`php
dump($request->all());
// Continue with additional logic…
“`
This is particularly useful for debugging within middleware or when you need to validate data before proceeding.
Common Use Cases for `dd($request)`
Here are some common scenarios where `dd($request)` can be particularly beneficial:
- Verifying Form Submissions:
- Check if all expected fields are present and correctly populated.
- Debugging API Requests:
- Ensure that incoming requests to your API endpoints contain the necessary data.
- Examining Middleware Behavior:
- Inspect the request as it passes through middleware to ensure proper modifications.
Alternatives to `dd()` in Laravel 11
While `dd()` is highly useful, there are alternatives you might consider based on your debugging needs:
Function | Description |
---|---|
`dump()` | Outputs data without halting execution. |
`logger()` | Logs data to the Laravel log files for later inspection. |
`Log::info()` | Similar to logger, but allows for more structured logging. |
Each of these alternatives provides a different approach to debugging, helping you choose the best fit for your specific situation.
Best Practices for Debugging Requests
When debugging requests in Laravel, keep these best practices in mind:
- Clear Output: Use `dd()` or `dump()` judiciously to avoid overwhelming output.
- Remove Debugging Code: Always ensure to comment out or remove debugging statements from production code.
- Leverage Laravel’s Built-in Tools: Utilize tools like Laravel Telescope for more advanced debugging capabilities.
By following these guidelines, you can enhance your debugging process and effectively manage request data within Laravel 11.
Expert Insights on Laravel 11’s Debugging Techniques
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Using `dd($request)` in Laravel 11 is a powerful way to inspect incoming requests. However, developers should consider using `dd($request->all())` to capture all input data, including query parameters and form data, which provides a comprehensive view of the request payload.”
Michael Chen (Web Development Consultant, CodeCraft Solutions). “While `dd($request)` is useful for quick debugging, it often omits crucial data. By opting for `dd($request->all())`, developers can avoid missing out on important context that could lead to more effective debugging and issue resolution.”
Lisa Thompson (Laravel Community Advocate, Open Source Projects). “In Laravel 11, the `dd()` function is a staple for debugging. However, it’s important to remember that `dd($request->all())` not only displays the data but also helps in understanding the structure of the input, which is essential for developing robust applications.”
Frequently Asked Questions (FAQs)
What does `dd($request)` do in Laravel?
`dd($request)` is a Laravel helper function that stands for “dump and die.” It outputs the contents of the `$request` object and halts the execution of the script, allowing developers to inspect the request data being sent to the server.
How can I use `dd` to inspect all request data in Laravel 11?
To inspect all request data, you can use `dd($request->all())`. This will dump all input data from the request, including query parameters, form data, and uploaded files, providing a comprehensive overview of what is being submitted.
Is there a difference between `dd($request)` and `dd($request->all())`?
Yes, `dd($request)` will display the entire request object, including metadata and headers, while `dd($request->all())` specifically shows only the input data sent with the request, making it easier to focus on user-submitted data.
Can I use `dump()` instead of `dd()` for debugging requests?
Yes, you can use `dump($request)` instead of `dd($request)`. The `dump()` function outputs the data without stopping the script execution, allowing you to continue running the application after inspecting the request data.
What are some alternatives to `dd()` for logging request data in Laravel?
Alternatives include using Laravel’s built-in logging capabilities with `Log::info($request->all())`, which logs the request data to the log files without interrupting the application flow. Additionally, you can use `Log::debug()` for more detailed logging.
How can I format the output of `dd($request->all())` for better readability?
You can format the output by using the `json_encode()` function with the `JSON_PRETTY_PRINT` option, like this: `dd(json_encode($request->all(), JSON_PRETTY_PRINT));` This will provide a more structured and readable format for the dumped data.
In Laravel 11, the `dd()` function is a powerful debugging tool that allows developers to dump and die, effectively halting the execution of the script while displaying the contents of a variable. When working with HTTP requests, utilizing `$request` with `dd()` can provide developers with a comprehensive overview of all incoming data. This includes not only the request parameters but also headers, files, and other relevant information, making it an essential function for debugging and understanding the structure of incoming requests.
One of the key takeaways from using `dd($request)` is the ability to quickly inspect the entirety of the request object. This can be particularly useful in scenarios where a developer needs to troubleshoot issues related to data being sent from forms or APIs. By dumping the request object, developers can ensure that they are receiving the expected data format and values, thus facilitating easier debugging and validation of request handling logic.
Moreover, leveraging `dd()` in Laravel 11 encourages best practices in debugging by allowing developers to isolate and examine specific parts of the request. This targeted approach not only enhances the efficiency of the debugging process but also aids in maintaining clean and readable code. As developers become more familiar with the structure of the request object, they can make informed
Author Profile
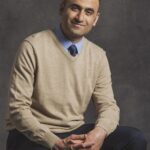
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?