How Can I Use Laravel Faker to Generate Random Dates?
In the world of web development, creating realistic and meaningful test data is crucial for building robust applications. Laravel, a popular PHP framework, offers a powerful tool known as Faker, which simplifies the process of generating fake data for testing and seeding databases. Among the various types of data Faker can produce, random dates play a vital role in simulating real-world scenarios. Whether you’re developing a blog, an e-commerce platform, or a social networking site, understanding how to effectively utilize the Laravel Faker generator to create random dates can enhance your application’s testing phase, ensuring that it behaves as expected under various conditions.
Faker’s ability to generate random dates opens up a myriad of possibilities for developers. From setting up user registration dates to creating timestamps for posts and transactions, the flexibility of random date generation can help mimic real-life data patterns. This ensures that your application can handle a variety of date-related functionalities, such as sorting, filtering, and displaying records. Moreover, utilizing Faker not only saves time but also allows developers to focus on other critical aspects of their projects without getting bogged down by the tedious task of manually creating test data.
As we delve deeper into the topic, we will explore the various methods and techniques for generating random dates using Laravel’s Faker library. From understanding
Generating Random Dates with Laravel Faker
Using Laravel’s Faker library to generate random dates is a straightforward process that can greatly enhance the testing and seeding of your database. Faker provides a variety of methods to create dates, including past and future dates, which can be tailored to fit your needs.
Basic Syntax for Random Dates
To generate a random date using Faker, you can utilize the `dateTime` and `dateTimeBetween` methods. Here’s how each method works:
- `dateTime()`: Generates a random date and time.
- `dateTimeBetween($startDate, $endDate)`: Generates a random date and time between two specified dates.
Here is a basic example of how to use these methods:
“`php
$faker = Faker\Factory::create();
$randomDate = $faker->dateTime(); // Generates a random date
$randomDateBetween = $faker->dateTimeBetween(‘2000-01-01’, ‘2023-12-31’); // Generates a random date between two dates
“`
Generating Specific Date Formats
Faker allows you to format the date in various ways. You can use the `format` method to specify how the date should appear. For example:
“`php
$formattedDate = $faker->dateTime()->format(‘Y-m-d’); // Outputs date in YYYY-MM-DD format
“`
You can replace `’Y-m-d’` with any valid PHP date format string to achieve your desired output.
Examples of Random Date Generation
Here are some practical examples of generating random dates using Laravel Faker:
“`php
$faker = Faker\Factory::create();
// Random past date
$pastDate = $faker->dateTimeBetween(‘-1 year’, ‘now’);
// Random future date
$futureDate = $faker->dateTimeBetween(‘now’, ‘+1 year’);
// Random date in a specific range
$customRangeDate = $faker->dateTimeBetween(‘2020-01-01’, ‘2020-12-31’);
“`
Using Random Dates in Database Seeding
When seeding your database with random data, including random dates can provide more realistic entries. Here’s an example of how you might use random dates in a database seeder:
“`php
use Illuminate\Database\Seeder;
use App\Models\YourModel;
class YourModelSeeder extends Seeder
{
public function run()
{
$faker = Faker\Factory::create();
foreach (range(1, 50) as $index) {
YourModel::create([
‘date_field’ => $faker->dateTimeBetween(‘-1 year’, ‘now’),
// Other fields…
]);
}
}
}
“`
Table of Date Methods
The following table outlines some of the key methods available in the Faker library for generating random dates:
Method | Description |
---|---|
dateTime() | Generates a random date and time. |
dateTimeBetween($startDate, $endDate) | Generates a random date and time between two specified dates. |
date() | Generates a random date in YYYY-MM-DD format. |
dateTimeThisYear() | Generates a random date within the current year. |
By leveraging these methods, you can effectively create realistic and varied date entries in your Laravel applications, enhancing the quality of your data during testing and development.
Generating Random Dates with Laravel Faker
Laravel provides an excellent tool for generating fake data through its Faker library, which is particularly useful for testing and seeding databases. One of the common requirements is to generate random dates. The Faker library allows you to easily create these random dates in various formats.
Using Faker to Generate Random Dates
To generate random dates, you can use the `dateTime`, `dateTimeBetween`, or `dateTimeThisDecade` methods provided by the Faker library. Each method serves a different purpose based on your requirements.
Commonly Used Methods:
- `dateTime()`: Generates a random date and time.
- `dateTimeBetween($startDate, $endDate)`: Generates a random date and time between two specified dates.
- `dateTimeThisDecade($max = ‘now’)`: Generates a random date and time within the current decade.
Example Usage
Here’s how you can implement these methods in your Laravel application:
“`php
use Faker\Factory as Faker;
$faker = Faker::create();
// Generate a random date and time
$randomDateTime = $faker->dateTime();
echo $randomDateTime->format(‘Y-m-d H:i:s’);
// Generate a random date and time between two dates
$startDate = ‘2020-01-01 00:00:00’;
$endDate = ‘2023-12-31 23:59:59’;
$randomDateBetween = $faker->dateTimeBetween($startDate, $endDate);
echo $randomDateBetween->format(‘Y-m-d H:i:s’);
// Generate a random date and time this decade
$randomDateThisDecade = $faker->dateTimeThisDecade();
echo $randomDateThisDecade->format(‘Y-m-d H:i:s’);
“`
Formatting Dates
The generated dates can be formatted using PHP’s `DateTime` class methods. Here are some common formats:
Format | Example |
---|---|
`Y-m-d` | 2023-10-15 |
`d/m/Y` | 15/10/2023 |
`F j, Y` | October 15, 2023 |
`Y-m-d H:i:s` | 2023-10-15 14:30:00 |
You can adjust the format as per your needs by using the `format()` method on the `DateTime` object returned by Faker.
Seeding Random Dates in Database
When seeding your database, you can incorporate random dates in your seeders. Here’s an example of how to insert random dates into a database table:
“`php
use Illuminate\Database\Seeder;
use App\Models\YourModel;
use Faker\Factory as Faker;
class YourTableSeeder extends Seeder
{
public function run()
{
$faker = Faker::create();
foreach (range(1, 50) as $index) {
YourModel::create([
‘date_field’ => $faker->dateTimeBetween(‘2020-01-01’, ‘2023-12-31’),
// other fields…
]);
}
}
}
“`
This code snippet demonstrates how to generate and insert random dates into the `date_field` of your model. Each time you run the seeder, new random dates will be populated in your database.
Utilizing the Laravel Faker library to generate random dates is straightforward and immensely beneficial for testing and database seeding. By employing the various methods available, you can tailor the random date generation to meet your specific requirements effectively.
Expert Insights on Using Laravel Faker for Generating Random Dates
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing the Laravel Faker library for generating random dates is an excellent way to populate your database with realistic data. It allows developers to create a variety of date formats and ranges, which can be crucial for testing applications under different scenarios.”
James Thompson (Lead Developer, CodeCraft Solutions). “When working with Laravel Faker, the ability to generate random dates can significantly enhance the quality of your test data. By leveraging the `dateTimeBetween` method, developers can specify a range that mimics real-world data, ensuring that the application behaves as expected in production environments.”
Linda Martinez (Data Scientist, FutureTech Labs). “Incorporating random date generation through Laravel Faker not only streamlines the testing process but also helps in identifying potential edge cases. By generating dates across various time frames, developers can observe how their applications handle date-related functionality, which is essential for robust software development.”
Frequently Asked Questions (FAQs)
What is Laravel Faker?
Laravel Faker is a library used for generating fake data in Laravel applications. It allows developers to create realistic test data for various fields, such as names, addresses, and dates.
How can I generate a random date using Laravel Faker?
To generate a random date, you can use the `dateTime` or `dateTimeBetween` methods provided by the Faker library. For example, `$faker->dateTimeBetween($startDate, $endDate)` generates a random date between the specified start and end dates.
Can I specify a date format when using Laravel Faker?
Yes, you can format the date generated by Faker using PHP’s `date` function. For instance, you can use `$faker->date(‘Y-m-d’)` to generate a date in the ‘YYYY-MM-DD’ format.
Is it possible to generate a random date within a specific year?
Yes, you can generate a random date within a specific year by setting the start and end dates accordingly. For example, `$faker->dateTimeBetween(‘2023-01-01’, ‘2023-12-31’)` will generate a random date within the year 2023.
How do I use Laravel Faker in my seeder files?
In your seeder files, you can use the Faker instance by importing it and then calling its methods to generate random data. For example, use `$faker = \Faker\Factory::create();` and then call `$faker->dateTime()` to generate a random date.
Can Laravel Faker generate dates in the past or future?
Yes, Laravel Faker can generate dates in both the past and the future. By using methods like `dateTimeBetween`, you can specify a range that includes past dates (e.g., `$faker->dateTimeBetween(‘-1 year’, ‘now’)`) or future dates (e.g., `$faker->dateTimeBetween(‘now’, ‘+1 year’)`).
In summary, the Laravel Faker generator is a powerful tool for creating fake data for testing and seeding databases in Laravel applications. One of its many features includes the ability to generate random dates, which can be particularly useful for simulating real-world scenarios in development and testing environments. The Faker library provides a straightforward API that allows developers to easily generate dates within specified ranges or formats, enhancing the realism of the data used in applications.
Key takeaways from the discussion include the importance of utilizing the Faker generator to streamline the process of data creation, thereby saving time and effort during development. The ability to customize the generated dates adds flexibility, allowing developers to create data that closely mirrors the requirements of their specific applications. Additionally, understanding how to use Faker effectively can lead to more robust testing practices, as it enables the generation of diverse datasets that can uncover potential issues before deployment.
Overall, leveraging the Laravel Faker generator for random date generation not only improves the efficiency of the development process but also enhances the quality of the application by ensuring that it is tested against a wide variety of data scenarios. As developers continue to seek efficient ways to manage data in their applications, tools like Faker remain indispensable in the Laravel ecosystem.
Author Profile
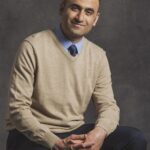
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?