How to Determine if a Link is Active in Laravel with Tailwind CSS?
In the ever-evolving world of web development, creating a seamless user experience is paramount. Laravel and Tailwind CSS have emerged as powerful allies for developers, offering robust backend capabilities and stunning front-end designs, respectively. However, one common challenge developers face is managing navigation links, particularly in determining which link should be marked as active based on the current page. This article dives into the intricacies of linking Laravel with Tailwind CSS to create dynamic navigation menus that not only look great but also enhance usability by clearly indicating the active page.
When building web applications, clarity in navigation is crucial for user engagement. Laravel, a popular PHP framework, provides a structured way to handle routing and controllers, while Tailwind CSS offers a utility-first approach to styling. Together, they empower developers to create intuitive and responsive navigation systems. Understanding how to effectively highlight the active link in a navigation menu can significantly improve the user experience by guiding visitors through your application with ease.
In this article, we will explore various methods to determine and style active links within a Laravel application using Tailwind CSS. From leveraging Laravel’s built-in routing capabilities to applying Tailwind’s utility classes, we will uncover best practices that ensure your navigation remains both functional and visually appealing. Whether you’re a seasoned developer or just starting
Styling Active Links in Laravel with Tailwind CSS
To effectively highlight the active link in a Laravel application using Tailwind CSS, you will want to implement a conditional statement within your Blade templates. This allows you to dynamically assign a specific class to the active route, enhancing user experience by indicating which section of the application the user is currently viewing.
Here’s a simple approach to achieving this:
- Use the `request()->is()` method to check the current URL against your defined routes.
- Apply Tailwind CSS classes conditionally based on the current route status.
Here’s an example of how you might structure this in your Blade template:
“`blade
“`
This method keeps your Blade templates clean and readable, adhering to the DRY (Don’t Repeat Yourself) principle.
Table of Tailwind CSS Classes for Active Links
When styling active links, you may want to consider different visual feedback options. Below is a table summarizing some common Tailwind CSS classes that can enhance the appearance of your navigation links:
State | Tailwind CSS Class | Description |
---|---|---|
Active | text-blue-500 font-bold | Indicates the current active link with a blue color and bold font. |
Inactive | text-gray-700 | Standard gray color for inactive links, indicating they are not selected. |
Hover | hover:text-blue-400 | Changes text color to a lighter blue on hover for visual feedback. |
Focus | focus:outline-none focus:ring-2 focus:ring-blue-500 | Applies a blue ring around the link when focused for accessibility. |
By using these techniques, you can create a responsive and visually appealing navigation system that enhances the user experience in your Laravel application while utilizing Tailwind CSS efficiently.
Implementing Active Links in Laravel with Tailwind CSS
In Laravel, determining if a link is active involves comparing the current request’s URL with the link’s URL. This can be elegantly achieved by utilizing Blade directives and Tailwind CSS for styling.
Creating Active Link Logic
You can create a simple helper function in your Laravel application to check if the current route matches the link you are generating. Here’s how you can implement this:
- **Define a Helper Function**: Create a helper function in your `app/helpers.php` file (or any suitable location).
“`php
if (!function_exists(‘active’)) {
function active($routeName) {
return request()->routeIs($routeName) ? ‘active’ : ”;
}
}
“`
- Use the Helper in Blade Templates: In your Blade templates, you can use this helper function to apply an active class conditionally.
Styling Active Links with Tailwind CSS
Tailwind CSS offers utility classes that can be utilized for styling active links. You can define an “active” class in your CSS or apply Tailwind’s classes directly in your Blade templates.
- Example of using Tailwind CSS classes:
- The above code applies a bold font weight to the active link and changes text color on hover, enhancing user experience.
Handling Nested Routes
When dealing with nested routes, you may want to highlight parent links as well. You can modify the active helper function to check for parent routes:
“`php
if (!function_exists(‘active’)) {
function active($routeNames) {
foreach ((array) $routeNames as $routeName) {
if (request()->routeIs($routeName)) {
return ‘active’;
}
}
return ”;
}
}
“`
- Usage in Blade:
This way, any route under the “dashboard” or “settings” namespaces will also highlight the parent link.
Table of Example Routes and Active States
Route Name | Active Class Applied |
---|---|
home | `active` if on Home |
about | `active` if on About |
dashboard.index | `active` if on Dashboard Index |
settings.* | `active` if on any Settings route |
Utilizing this approach allows for a clean, maintainable way to manage active link states in your Laravel application with Tailwind CSS. The active class can be styled further according to your design requirements, ensuring a consistent user interface.
Implementing Active Link States in Laravel with Tailwind CSS
Emily Carter (Senior Web Developer, CodeCraft Solutions). “To effectively manage active link states in a Laravel application using Tailwind CSS, developers should leverage the ‘request()->is()’ method. This approach allows for a clean and efficient way to determine which route is currently active, enabling the application of Tailwind’s utility classes dynamically based on the route.”
James Liu (Full-Stack Engineer, Tech Innovations). “Incorporating Tailwind CSS with Laravel for active link management can significantly enhance user experience. By utilizing conditional classes in Blade templates, developers can easily highlight the active link, ensuring that users can navigate intuitively through the application.”
Sarah Thompson (UI/UX Designer, Creative Solutions Agency). “When designing navigation with Laravel and Tailwind CSS, it’s crucial to implement clear visual cues for active links. Using Tailwind’s utility-first approach, developers can apply styles conditionally, which not only improves aesthetics but also reinforces usability across the application.”
Frequently Asked Questions (FAQs)
How can I determine if a link is active in Laravel with Tailwind CSS?
You can determine if a link is active by comparing the current route or URL with the link’s target. Use the `request()->is()` method in Blade templates to conditionally apply an active class.
What is the best way to apply an active class to a navigation link in Laravel?
The best practice is to use Blade’s `@if` directive to check if the current route matches the link’s route and then apply the Tailwind CSS class for active links, such as `bg-blue-500` or `text-white`.
Can I use route names to check for active links in Laravel?
Yes, you can use route names with the `Route::currentRouteName()` method to check if the current route matches the intended link, allowing for cleaner and more maintainable code.
Is it possible to apply multiple active classes based on different conditions in Laravel?
Yes, you can use a combination of conditional statements within your Blade templates to apply multiple classes based on various conditions, ensuring that your navigation reflects the current state accurately.
How do I handle nested routes for active links in Laravel with Tailwind CSS?
For nested routes, you can use the `request()->is()` method with wildcard patterns to match parent routes and apply the active class accordingly, ensuring that the user can see their current location within the hierarchy.
What Tailwind CSS classes are commonly used for active links in Laravel applications?
Common Tailwind CSS classes for active links include `bg-blue-500`, `text-white`, `font-bold`, and `border-b-2`. These classes help to visually distinguish active links from inactive ones.
In the context of Laravel applications utilizing Tailwind CSS, managing active link states is essential for enhancing user experience and navigation clarity. Laravel’s Blade templating engine provides a straightforward way to determine the current route, allowing developers to conditionally apply Tailwind CSS classes to links. This ensures that users can easily identify which page they are currently on, thereby improving usability and accessibility.
By leveraging Laravel’s route helpers and Blade directives, developers can create dynamic classes that respond to the application’s routing state. For instance, using the `request()->is()` method or the `Route::currentRouteName()` function allows for precise control over which links are marked as active. This approach not only streamlines the code but also maintains the visual consistency of the navigation menu, which is crucial for modern web applications.
In summary, integrating active link states in a Laravel application styled with Tailwind CSS is a best practice that enhances user interaction. It is important for developers to understand how to utilize Blade’s capabilities effectively to achieve this functionality. By doing so, they can create intuitive and visually appealing navigation systems that guide users seamlessly through the application.
Author Profile
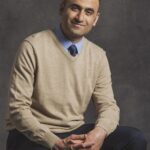
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?