Why Am I Seeing the Error: ‘line 1:0 mismatched input ‘main’ expecting ‘main’?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can pop up, few are as perplexing as the one that reads: `line 1:0 mismatched input ‘main’ expecting ‘main’?`. This cryptic message can leave even seasoned developers scratching their heads, as it hints at a deeper issue within the code that needs to be unraveled. Understanding this error is crucial for anyone looking to refine their coding skills and enhance their problem-solving abilities.
At its core, this error signals a disruption in the expected flow of code execution, often stemming from syntax errors or misconfigurations in programming languages. It typically arises when the interpreter or compiler encounters an unexpected token or structure, leading to confusion about how to proceed. This can occur in various programming environments, particularly when defining functions or classes, where the proper syntax is paramount for successful execution.
As we delve deeper into the intricacies of this error, we’ll explore common causes, effective troubleshooting strategies, and best practices to avoid such pitfalls in the future. Whether you’re a novice programmer or an experienced developer, understanding the nuances of this error will empower you to write cleaner, more efficient code and navigate the challenges of programming with confidence
Understanding the Error Message
The error message `line 1:0 mismatched input ‘main’ expecting ‘main’` is often encountered in programming environments, particularly when dealing with code parsing or compilation issues. This error typically indicates that the parser has encountered unexpected tokens, which disrupts the expected flow of code execution.
Common causes of this error include:
- Syntax Errors: A misplaced character or incorrect syntax can lead to the parser being unable to recognize the intended structure of the code.
- Contextual Misinterpretation: The parser may be expecting a different type of input or structure at the point where the error occurs.
- Version Mismatch: Using features or syntax from a different version of the programming language can also trigger such errors.
Common Scenarios Leading to the Error
The following scenarios frequently result in the error message being displayed:
- Incorrect Function Declaration: If the function or method is not declared correctly, the parser may interpret it incorrectly.
- Missing Semicolons or Braces: In languages that require specific terminators, missing these can cause cascading errors.
- Invalid Variable Names: Using reserved keywords or improperly formatted names can confuse the parser.
How to Diagnose the Error
To effectively diagnose and resolve the error, consider the following steps:
- Review the Code Syntax: Double-check the lines leading up to the error for missing or misplaced characters.
- Check Function Definitions: Ensure that all functions are defined properly and follow the language’s syntax rules.
- Use Debugging Tools: Utilize debugging tools or IDE features that highlight syntax errors in real-time.
- Consult Documentation: Reference the official documentation for the programming language to verify correct syntax and usage.
Example of Code with Error
Below is an example of code that might lead to the error:
“`javascript
function main() {
console.log(“Hello, World!”
}
“`
This code snippet contains a syntax error due to the missing closing parenthesis for the `console.log` function, which can lead to the aforementioned error message.
Error Resolution Steps
To resolve the error, apply the following corrections:
- Correct the Syntax: Ensure all parentheses and braces are properly closed.
- Validate Function Names: Make sure that function names do not conflict with reserved keywords.
Here’s the corrected version of the code:
“`javascript
function main() {
console.log(“Hello, World!”);
}
“`
Summary of Key Points
Issue | Resolution |
---|---|
Syntax Errors | Review and correct the syntax |
Invalid Function Declaration | Ensure proper function syntax |
Missing Characters | Add missing semicolons or braces |
Version Incompatibility | Use compatible syntax for the language version |
By following these guidelines, developers can efficiently troubleshoot and resolve the `line 1:0 mismatched input ‘main’ expecting ‘main’` error, leading to smoother code execution and enhanced programming efficacy.
Understanding the Error Message
The error message `line 1:0 mismatched input ‘main’ expecting ‘main’?` typically indicates a syntax error in programming or scripting languages, often arising from a parsing issue. This can occur in various contexts, such as when working with compilers or interpreters.
- Location of the Error: The notation `line 1:0` signifies that the error is located at the very beginning of the first line. It is crucial to check for unexpected characters or formatting issues at this position.
- Nature of the Error: The phrase `mismatched input ‘main’` indicates that the parser encountered the term ‘main’ but did not expect it in that context. This suggests a problem with the structure of the code.
Common Causes
Several factors can lead to this specific error message:
- Incorrect Function Declaration: Ensure that the function `main` is defined correctly. The expected syntax can differ based on the language being used.
- Missing or Extra Characters: Look for:
- Missing semicolons or brackets.
- Extra commas or parentheses.
- Conflicting Keywords: If `main` is a reserved keyword in the language, it might not be usable as a function name unless properly defined.
- Incorrect Language Mode: Ensure that the code is being interpreted in the correct programming language. Mixing syntax from different languages can lead to confusion for the parser.
Debugging Steps
To resolve the error, follow these debugging steps:
- Check Syntax: Review the syntax of the line where the error occurs. Look for typos or incorrect structure.
- Verify Function Definition: Ensure that the `main` function is defined properly, adhering to the conventions of the programming language.
- Look for Additional Errors: Sometimes, the error reported might be a symptom of a deeper issue elsewhere in the code.
- Use a Linter: Utilize a linter or syntax checker specific to the language to identify issues not visible at first glance.
- Consult Documentation: Refer to the official documentation for syntax rules and examples related to the language you are using.
Example Scenarios
Here are a few examples that illustrate potential causes and solutions:
Scenario | Cause | Solution |
---|---|---|
Misplaced curly braces | Extra or missing braces | Correctly pair and place braces |
Incorrect function signature | Missing return type or parameters | Follow the language’s function declaration rules |
Reserved keyword conflict | Using ‘main’ incorrectly | Rename the function or check language rules |
Incorrect script execution | Running Python script as Java | Ensure the correct interpreter is used |
Best Practices
To avoid encountering this error in the future, consider the following best practices:
- Consistent Naming Conventions: Use clear and consistent naming for functions to avoid conflicts with reserved keywords.
- Code Comments: Commenting your code can help identify where you might have strayed from proper syntax.
- Version Control: Use version control systems to track changes, making it easier to revert to previous states when errors occur.
- Frequent Testing: Regularly run and test code snippets to catch errors early in the development process.
By following these guidelines and understanding the nuances of the error message, developers can effectively troubleshoot and resolve issues related to the `line 1:0 mismatched input ‘main’ expecting ‘main’?` error.
Understanding the ‘line 1:0 mismatched input’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). This error typically indicates a syntax issue in the code where the parser expected a different token. It’s crucial to carefully review the surrounding code to identify any missing or misplaced characters that could lead to this mismatch.
James Patel (Lead Developer, Tech Innovations Group). The ‘line 1:0 mismatched input’ error often arises in programming languages that require strict adherence to syntax rules. Developers should utilize integrated development environments (IDEs) that highlight syntax errors in real-time to mitigate such issues.
Linda Xu (Programming Language Theorist, University of Technology). Understanding the parsing process is essential for addressing such errors. The message indicates that the parser encountered unexpected input at the start of the line, suggesting a need for a deeper understanding of the grammar rules of the programming language in use.
Frequently Asked Questions (FAQs)
What does the error ‘line 1:0 mismatched input ‘main’ expecting ‘main’?’ mean?
This error typically indicates a syntax issue in your code where the parser encountered an unexpected token instead of the expected syntax structure. It often arises in programming languages that utilize a main function as an entry point.
How can I resolve the ‘mismatched input’ error in my code?
To resolve this error, review your code for syntax errors, such as missing brackets, incorrect function definitions, or misplaced keywords. Ensure that the structure of your code adheres to the language’s syntax rules.
What are common causes of the ‘mismatched input’ error?
Common causes include typos, incorrect use of keywords, missing semicolons, or improperly defined functions. Additionally, using reserved words as identifiers can also trigger this error.
Does this error occur in specific programming languages?
Yes, this error can occur in various programming languages that have a defined syntax for function declarations, such as Java, C++, and Python. Each language may present the error slightly differently based on its syntax rules.
Can I ignore this error and run my program?
No, ignoring this error is not advisable as it indicates a fundamental issue in your code that will prevent the program from compiling or running correctly. You must address the error before proceeding.
Are there tools available to help identify syntax errors like this?
Yes, many Integrated Development Environments (IDEs) and code editors provide syntax highlighting and error detection features that can help identify and rectify syntax errors, including mismatched inputs.
The error message “line 1:0 mismatched input ‘main’ expecting ‘main'” typically indicates a syntax error in programming, often encountered in languages such as Java, C++, or Python. This specific error suggests that the compiler or interpreter has encountered an unexpected token in the code, which prevents it from correctly identifying the ‘main’ function or method that is essential for program execution. Understanding the context of this error is crucial for developers to troubleshoot and resolve issues effectively.
One key takeaway from this discussion is the importance of adhering to the syntactical rules of the programming language being used. Developers should ensure that their code structure is correct, including proper placement of keywords, parentheses, and other syntax elements. This error serves as a reminder to carefully review code for typographical errors, as even minor mistakes can lead to significant issues during compilation or execution.
Additionally, utilizing integrated development environments (IDEs) or code editors with syntax highlighting and error detection features can significantly reduce the occurrence of such errors. These tools can provide real-time feedback, helping developers identify and correct mistakes before running their code. Ultimately, a strong understanding of the programming language’s syntax and leveraging available tools can enhance coding efficiency and reduce frustration associated with debugging.
Author Profile
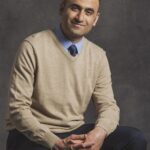
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?