How Can I Use LINQ to Select All Columns from a DataTable?
When working with data in .NET applications, developers often turn to LINQ (Language Integrated Query) for its powerful querying capabilities. One common scenario that arises is the need to select all columns from a DataTable. Whether you’re aggregating data for reporting, filtering records for analysis, or simply displaying information in a user interface, efficiently retrieving all columns can save time and streamline your code. In this article, we will explore the various methods and best practices for using LINQ to select all columns from a DataTable, ensuring you can maximize your data manipulation efforts.
Selecting all columns from a DataTable using LINQ is not only a straightforward task but also an essential skill for any developer working with data in C. The beauty of LINQ lies in its ability to seamlessly integrate with the DataTable structure, allowing for intuitive and expressive queries. By leveraging LINQ, developers can access and manipulate data with ease, transforming complex queries into readable and maintainable code.
In the following sections, we will delve into the different approaches to selecting all columns, highlighting the nuances of each method. From basic queries to more advanced techniques, you will gain insights into how to efficiently handle DataTables and enhance your data processing capabilities. Join us as we uncover the potential of LINQ and empower your development
Using LINQ to Select All Columns from a DataTable
When working with a DataTable in C, you may find the need to extract all the columns for processing or manipulation. LINQ provides a concise way to achieve this by leveraging the `AsEnumerable()` method, which enables you to query DataTable rows as a collection. Below are the steps and examples to effectively select all columns using LINQ.
To select all columns from a DataTable, you can use the following syntax:
“`csharp
var allColumns = dataTable.AsEnumerable()
.Select(row => row.ItemArray);
“`
In this example, `ItemArray` is an array of objects representing all the columns in the current row. This allows you to retrieve every column in a straightforward manner.
Example: Selecting All Columns
Here’s a practical example demonstrating how to select all columns from a DataTable and store them in a list:
“`csharp
DataTable dataTable = new DataTable();
// Assume dataTable is populated with data
var allColumnsList = dataTable.AsEnumerable()
.Select(row => row.ItemArray.ToArray())
.ToList();
“`
This code snippet converts each row into an array and gathers all rows in a list.
Using Anonymous Types for Selected Columns
If you only want to select specific columns, you can use anonymous types in your LINQ query. Here’s how to do it:
“`csharp
var selectedColumns = dataTable.AsEnumerable()
.Select(row => new
{
Column1 = row.Field
Column2 = row.Field
});
“`
This method allows you to define which columns you want to include in the output, improving readability and performance.
Performance Considerations
When selecting all columns from a DataTable using LINQ, consider the following performance aspects:
- Memory Usage: Storing all columns may lead to high memory consumption, especially with large datasets.
- Row Filtering: Apply filtering conditions before selecting to minimize the data processed.
- Deferred Execution: LINQ queries utilize deferred execution, which means the query is not executed until you iterate over the result. This can be beneficial for performance.
Aspect | Consideration |
---|---|
Memory Usage | Be cautious with large DataTables |
Row Filtering | Filter rows before selection to optimize performance |
Deferred Execution | Queries are executed when iterated |
By using these techniques, you can efficiently select all columns from a DataTable while also maintaining good performance in your applications.
Using LINQ to Select All Columns from a DataTable
To retrieve all columns from a DataTable using LINQ, you can leverage the `AsEnumerable()` method, which allows you to work with DataRows in a manner similar to standard LINQ collections. Below is a concise guide on how to effectively perform this operation.
Basic Syntax
The basic syntax for selecting all columns from a DataTable is as follows:
“`csharp
var allColumns = dataTable.AsEnumerable()
.Select(row => row.ItemArray);
“`
In this example, `ItemArray` returns an array of all the values in the row, effectively selecting all columns.
Detailed Example
Here is a complete example demonstrating how to use LINQ to select all columns from a DataTable:
“`csharp
using System;
using System.Data;
using System.Linq;
class Program
{
static void Main()
{
DataTable dataTable = new DataTable();
// Assume dataTable is populated with data
var allColumns = dataTable.AsEnumerable()
.Select(row => new
{
Columns = row.ItemArray
});
foreach (var item in allColumns)
{
Console.WriteLine(string.Join(“, “, item.Columns));
}
}
}
“`
In this example:
- A DataTable named `dataTable` is assumed to be populated with data.
- The LINQ query selects all columns by accessing `ItemArray`.
- The result is printed to the console.
Filtering and Projection
In scenarios where filtering or projecting specific data is required, you can modify the LINQ query. For instance, if you want to select only specific columns while still retrieving all data:
“`csharp
var selectedColumns = dataTable.AsEnumerable()
.Select(row => new
{
Column1 = row.Field
Column2 = row.Field
});
“`
This approach allows you to specify the data type for each column explicitly using the `Field
Performance Considerations
When working with large DataTables, consider the following performance aspects:
- Deferred Execution: LINQ queries are not executed until the data is enumerated, which can save processing time if further filtering is applied.
- Memory Usage: Using `ItemArray` creates a new array for each row, which can increase memory usage. If you only need a few columns, prefer selecting only those.
- Type Safety: Using `Field
()` ensures type safety and can prevent runtime errors.
Common Use Cases
The following are scenarios where selecting all columns from a DataTable can be beneficial:
- Data Export: Preparing data for export to formats like CSV or Excel.
- Reporting: Generating reports that require all data from a DataTable.
- Data Transformation: Transforming data into another format or structure for further processing.
By employing LINQ effectively, you can streamline operations on DataTables while maintaining clarity and conciseness in your code.
Expert Insights on Selecting All Columns from a DataTable Using LINQ
Dr. Emily Carter (Data Science Consultant, Tech Innovations Inc.). “When working with DataTables in LINQ, selecting all columns can be efficiently achieved using the ‘AsEnumerable()’ method followed by ‘Select()’. This approach allows developers to manipulate and retrieve data without losing the flexibility of the original DataTable structure.”
Michael Thompson (Senior Software Engineer, Data Solutions Corp.). “Utilizing LINQ to select all columns from a DataTable can streamline data processing tasks. By leveraging the ‘Select’ method, you can create a new collection that includes all columns, which is particularly useful for data transformation and analysis in .NET applications.”
Jessica Lin (Lead Developer, Analytics Group). “Incorporating LINQ with DataTables enhances data manipulation capabilities. To select all columns, one can use ‘DataTable.AsEnumerable().Select(row => row.ItemArray)’. This method preserves the integrity of the data while providing a straightforward way to access all columns in a clean format.”
Frequently Asked Questions (FAQs)
What is LINQ and how does it relate to DataTables?
LINQ, or Language Integrated Query, is a feature in .NET that allows developers to query collections in a concise and readable manner. When working with DataTables, LINQ can be used to perform queries on the data contained within the table.
How can I select all columns from a DataTable using LINQ?
To select all columns from a DataTable using LINQ, you can use the `AsEnumerable()` method followed by a `Select` statement that returns each row. For example: `var result = dataTable.AsEnumerable().Select(row => row.ItemArray);`
Is it possible to filter rows while selecting all columns in a DataTable?
Yes, you can filter rows while selecting all columns by using the `Where` clause in conjunction with the `Select` statement. For instance: `var result = dataTable.AsEnumerable().Where(row => row.Field
Can I convert the result of a LINQ query on a DataTable back to a DataTable?
Yes, you can convert the result of a LINQ query back to a DataTable by creating a new DataTable and importing the rows. You can use `CopyToDataTable()` method after filtering and selecting the desired rows.
What are the performance implications of using LINQ with large DataTables?
Using LINQ with large DataTables can lead to performance overhead due to the creation of enumerators and the execution of queries. It is advisable to optimize queries and consider alternative methods for very large datasets, such as using SQL directly or optimizing the DataTable structure.
Are there any limitations to using LINQ with DataTables?
Yes, limitations include the inability to directly use LINQ to modify the DataTable structure or enforce constraints. Additionally, LINQ queries may not support all types of data operations that can be performed directly on DataTables.
In the context of working with DataTables in .NET, utilizing LINQ to select all columns from a DataTable is a common requirement for developers. LINQ (Language Integrated Query) provides a powerful and expressive way to query data, allowing for efficient data manipulation and retrieval. By employing the `AsEnumerable()` method on a DataTable, developers can convert the DataTable into an enumerable collection, which can then be queried using LINQ syntax. This approach facilitates the selection of all columns from the DataTable, enabling developers to retrieve data in a streamlined manner.
One of the key advantages of using LINQ to select all columns is the ability to maintain readability and conciseness in code. Instead of writing complex loops or data access code, developers can leverage LINQ’s fluent syntax to achieve the same results with fewer lines of code. This not only enhances code maintainability but also improves performance by optimizing the data retrieval process. Additionally, LINQ supports various query operations, allowing for further filtering, sorting, and grouping of data as needed.
In summary, employing LINQ to select all columns from a DataTable is an effective strategy for developers working within the .NET framework. It simplifies data access, enhances code clarity, and provides
Author Profile
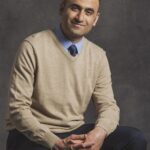
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?