How Can You Create a JavaScript Extension for Shipping.js in Magento 2?
In the ever-evolving world of eCommerce, providing a seamless and personalized shopping experience is paramount. For businesses utilizing Magento 2, the platform offers a robust framework that allows for extensive customization, particularly in the realm of shipping options. One of the most critical components of this framework is the `shipping.js` file, which governs the shipping methods and their interactions within the checkout process. However, as businesses grow and customer expectations shift, there often arises a need to extend or modify these functionalities to better suit specific requirements. This article will guide you through the process of creating a JavaScript file to extend `shipping.js`, empowering you to enhance your Magento 2 store’s shipping capabilities.
Extending `shipping.js` in Magento 2 provides developers with the flexibility to implement custom logic, integrate third-party shipping services, or adjust the user interface to improve the customer experience. By creating a new JavaScript file, you can seamlessly introduce additional features without disrupting the core functionality of the platform. This not only ensures that your store remains up-to-date with the latest enhancements but also allows for a tailored approach to shipping that can set your business apart from the competition.
As we delve deeper into this topic, we will explore the necessary steps to create and implement your custom JavaScript file, ensuring
Creating a Custom JavaScript File
To extend the functionality of `shipping.js` in Magento 2, the first step is to create a custom JavaScript file. This file will contain the additional logic you want to implement. Follow these steps to set up your custom JS file:
- Create a new directory structure in your theme:
“`
app/design/frontend/Vendor/theme/Magento_Checkout/web/js/view/
“`
- Create a new JavaScript file named `custom-shipping.js` within this directory.
- In your `custom-shipping.js`, you can extend the original `shipping.js`. Here’s an example of how to achieve this:
“`javascript
define([
‘jquery’,
‘Magento_Checkout/js/view/shipping’
], function ($, Shipping) {
‘use strict’;
return Shipping.extend({
initialize: function () {
this._super();
// Your custom logic here
},
customFunction: function () {
// Custom functionality
}
});
});
“`
This code snippet initializes your custom shipping module and allows you to add additional functions.
Registering Your Custom JavaScript File
After creating your custom JavaScript file, you need to register it in the layout XML files to ensure it loads correctly. This is done by creating or modifying the `default.xml` file located in:
“`
app/design/frontend/Vendor/theme/Magento_Checkout/layout/
“`
Add the following code to `default.xml` to include your custom script:
“`xml
This XML configuration tells Magento to load your custom JavaScript file on the checkout page.
Dependencies Management
When extending JavaScript in Magento 2, managing dependencies is crucial. Ensure that your custom file loads after the original `shipping.js` to avoid any conflicts. You can handle dependencies using the `requirejs-config.js` file.
Create or modify the `requirejs-config.js` file in your theme at:
```
app/design/frontend/Vendor/theme/Magento_Checkout/requirejs-config.js
```
Add the following code to ensure your custom script loads correctly:
```javascript
var config = {
map: {
'*': {
'Magento_Checkout/js/view/shipping': 'Vendor_Theme/js/view/custom-shipping'
}
}
};
```
This configuration maps the original `shipping.js` to your custom implementation.
Testing Your Custom Functionality
After making these changes, it’s crucial to test your custom shipping functionality. Here are some steps to ensure everything works as expected:
- Clear the cache using:
```bash
php bin/magento cache:clean
php bin/magento cache:flush
```
- Deploy static content:
```bash
php bin/magento setup:static-content:deploy
```
- Open your Magento 2 checkout page and check the console for any JavaScript errors.
Task | Command |
---|---|
Clear Cache | php bin/magento cache:clean & php bin/magento cache:flush |
Deploy Static Content | php bin/magento setup:static-content:deploy |
By following these steps, you can successfully extend the `shipping.js` functionality in Magento 2, enhancing your checkout process with custom logic tailored to your business needs.
Creating a JavaScript File to Extend `shipping.js` in Magento 2
To extend the existing `shipping.js` file in Magento 2, you will need to follow a specific process that involves creating a new JavaScript file and configuring it properly within the Magento framework. This process allows you to add or override functionality while maintaining the integrity of the core files.
Step-by-Step Guide to Create and Extend `shipping.js`
- Create Your Custom Module
Begin by setting up a custom module if you do not already have one. This module will house your new JavaScript file. The structure should look like this:
```
app/code/Vendor/Module/
├── registration.php
├── etc
│ └── module.xml
└── view
└── frontend
└── requirejs-config.js
└── web
└── js
└── custom-shipping.js
```
- Define the Module
In `registration.php`, register your module:
```php
```
- Create `custom-shipping.js`
In your custom JavaScript file, extend the core `shipping.js` functionality:
```javascript
define([
'jquery',
'Magento_Checkout/js/view/shipping'
], function ($, Shipping) {
'use strict';
return Shipping.extend({
initialize: function () {
this._super();
// Add your custom code here
},
customFunction: function () {
// Your custom logic
}
});
});
```
- Configure RequireJS
In `requirejs-config.js`, map your custom JavaScript file to override the default `shipping.js`:
```javascript
var config = {
map: {
'*': {
'Magento_Checkout/js/view/shipping': 'Vendor_Module/js/custom-shipping'
}
}
};
```
- Deploy Static Content
After making the changes, deploy the static content to ensure that your new JavaScript file is recognized:
```
php bin/magento setup:static-content:deploy -f
```
- Clear Cache
Finally, clear the cache to see the changes take effect:
```
php bin/magento cache:clean
php bin/magento cache:flush
```
Testing Your Extension
To verify that your customizations are working:
- Open the Frontend: Navigate to the checkout page where shipping options are displayed.
- Check Console for Errors: Use the browser's developer tools to ensure there are no JavaScript errors.
- Test Functionality: Interact with the shipping options to confirm that your custom code is executing as expected.
By following these steps, you can effectively extend the functionality of `shipping.js` in Magento 2, allowing for enhanced control over the shipping options presented to customers during checkout.
Expert Insights on Extending Shipping.js in Magento 2
Jessica Lin (Senior Magento Developer, eCommerce Innovations). "Extending shipping.js in Magento 2 requires a solid understanding of the Knockout.js framework, as it is heavily utilized in Magento's frontend architecture. Developers should ensure that they properly override the existing shipping.js file to maintain compatibility with future updates."
Michael Thompson (Lead Software Engineer, Magento Solutions Group). "When creating a JavaScript extension for shipping.js, it's crucial to follow Magento's best practices for module development. This includes using the RequireJS framework for dependency management and ensuring that your custom scripts are properly registered in the module's configuration."
Sarah Patel (eCommerce Architect, Digital Commerce Experts). "Testing is an essential part of extending shipping.js. I recommend setting up a robust testing environment to validate your changes. Utilize both unit tests and integration tests to ensure that your modifications do not introduce any regressions in the shipping functionality."
Frequently Asked Questions (FAQs)
How can I create a custom JavaScript file to extend shipping.js in Magento 2?
To create a custom JavaScript file that extends shipping.js, you need to create a new module in your Magento 2 installation. Within your module, create a `view/frontend/web/js` directory and add your custom JavaScript file. Use the `define` function to extend the original shipping.js and include your custom logic.
What is the purpose of extending shipping.js in Magento 2?
Extending shipping.js allows developers to customize the shipping methods and functionalities in the checkout process. This can include adding new shipping options, modifying existing behaviors, or integrating third-party shipping services.
Where do I register my custom JavaScript file in Magento 2?
You should register your custom JavaScript file in the `requirejs-config.js` file of your module. This file allows you to configure RequireJS to load your custom script as an extension of shipping.js, ensuring that it is executed appropriately during the checkout process.
Do I need to enable my module after creating it to see the changes?
Yes, after creating your module and adding your custom JavaScript file, you must enable the module using the command line. Use the command `php bin/magento module:enable Your_Module_Name`, followed by `php bin/magento setup:upgrade` to apply the changes.
How can I test if my custom JavaScript is working correctly in the checkout?
To test your custom JavaScript, you can open the checkout page in your browser and use the developer tools (F12) to check for any console errors. Additionally, you can add console logs in your custom script to verify that it is being executed and that your changes are functioning as intended.
What should I do if my customizations are not reflecting on the frontend?
If your customizations are not reflecting, ensure that you have cleared the cache using `php bin/magento cache:clean` and `php bin/magento cache:flush`. Additionally, check for any JavaScript errors in the console that may prevent your script from executing.
In Magento 2, extending the functionality of existing JavaScript files, such as shipping.js, is a common requirement for developers looking to customize the checkout process. This involves creating a new JavaScript file that leverages the existing shipping.js functionality while allowing for additional features or modifications. By following the proper steps to set up the new JS file and ensuring it properly extends the original, developers can enhance the user experience and tailor the checkout flow to meet specific business needs.
One of the key insights from this discussion is the importance of understanding the Magento 2 JavaScript architecture, particularly the use of RequireJS for module loading. Developers should be familiar with how to define dependencies and utilize the extend functionality effectively. This knowledge is crucial for ensuring that the new JavaScript functionality integrates seamlessly with the existing codebase and does not introduce conflicts or errors.
Additionally, leveraging Magento's built-in mechanisms for overriding and extending JavaScript files allows for a more maintainable and upgrade-friendly approach. This practice not only helps in preserving customizations during upgrades but also enhances collaboration among team members by adhering to established coding standards. Overall, mastering the extension of shipping.js and similar files is a valuable skill for Magento 2 developers aiming to create a more dynamic and responsive
Author Profile
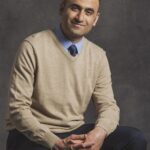
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?