How Can You Make an ASPX Checkbox Invisible?
In the world of web development, creating a seamless user experience is paramount. As developers, we often find ourselves needing to control the visibility of various elements on a webpage, including ASP.NET controls like the `aspx:CheckBox`. Whether you’re looking to streamline your interface, enhance user interaction, or simply hide options that are not relevant at a given moment, knowing how to manipulate the visibility of these controls can be a game-changer. In this article, we’ll explore effective strategies for making an `aspx:CheckBox` invisible, ensuring your web applications remain clean and user-friendly.
Overview
The ability to show or hide elements dynamically is a powerful feature in web applications. For ASP.NET developers, the `aspx:CheckBox` control is a common component that may need to be hidden under certain conditions. This could be due to user permissions, specific application states, or simply to reduce clutter on the page. Understanding how to manage the visibility of this control not only improves the aesthetic of your application but also enhances functionality by guiding users toward the most relevant options.
In this article, we will delve into various methods for altering the visibility of an `aspx:CheckBox`. From utilizing server-side logic to employing client-side techniques, we will cover
Using CSS to Hide an ASPX CheckBox
To make an ASPX CheckBox not visible, one effective method is to utilize CSS. By applying a CSS rule, you can set the display property of the CheckBox to none. This method allows the CheckBox to remain in the DOM but not be rendered in the user interface.
“`html
“`
In this example, the CheckBox will not be displayed on the page due to the `hidden-checkbox` class that sets `display: none;`. This approach is beneficial when you want to dynamically control the visibility of the CheckBox through JavaScript or server-side logic.
Setting Visibility in Code-Behind
Another approach to hide an ASPX CheckBox is by manipulating its `Visible` property in the code-behind file. This property can be set to “ to remove the CheckBox from the rendered output entirely.
“`csharp
protected void Page_Load(object sender, EventArgs e)
{
CheckBox1.Visible = ; // Hides the CheckBox
}
“`
This method is straightforward and ensures that the CheckBox is not part of the page’s lifecycle, which can help optimize performance in scenarios where the CheckBox is not needed.
Using JavaScript for Dynamic Visibility Control
JavaScript can provide a dynamic way to control the visibility of an ASPX CheckBox. You may want to hide or show the CheckBox based on user interaction, such as a button click. Here’s how you can implement this:
“`html
“`
In this example, clicking the button will toggle the visibility of the CheckBox. The use of `<%= CheckBox1.ClientID %>` ensures that the correct client-side ID is referenced.
Comparison of Methods to Hide ASPX CheckBox
Method | Advantages | Disadvantages |
---|---|---|
CSS | Easy to implement, keeps element in DOM | Still loads in the DOM, affecting performance |
Code-Behind | Completely removes from page lifecycle | Requires post-back to change visibility |
JavaScript | Dynamic control without post-backs | Requires client-side scripting support |
Each method has its own advantages and disadvantages, making it important to choose the right one based on the specific requirements of your application.
Hiding an ASPX CheckBox
To make an ASPX CheckBox control not visible in an ASP.NET Web Forms application, you can use various methods depending on your requirements. Below are the primary approaches to achieve this.
Using the Visible Property
The simplest way to hide a CheckBox is to set its `Visible` property to “. This method completely removes the CheckBox from the page rendering.
“`aspx
“`
Alternatively, you can set this property programmatically in your code-behind:
“`csharp
protected void Page_Load(object sender, EventArgs e)
{
CheckBox1.Visible = ; // Hides the CheckBox
}
“`
Using CSS for Hiding
If you want to keep the CheckBox in the control tree but not display it to users, you can apply CSS to set its display to `none`. This approach is beneficial when you might want to show the CheckBox later without requiring a postback.
“`aspx
“`
And then define the CSS class in your stylesheet:
“`css
.hiddenCheckbox {
display: none;
}
“`
Conditional Visibility
To conditionally hide a CheckBox based on certain logic, you can use server-side code to set the visibility dynamically. For example:
“`csharp
protected void Page_Load(object sender, EventArgs e)
{
if (SomeCondition)
{
CheckBox1.Visible = true; // Show if condition is met
}
else
{
CheckBox1.Visible = ; // Hide if condition is not met
}
}
“`
JavaScript for Client-Side Control
You can also utilize JavaScript to hide the CheckBox after the page has loaded. This method allows for dynamic interaction without needing a server postback.
“`aspx
“`
Comparison of Methods
Method | Description | Use Case |
---|---|---|
Visible Property | Completely removes the CheckBox from rendering | When you do not need the CheckBox at all |
CSS | Hides the CheckBox but keeps it in the control tree | When you might need to show it later |
Conditional Logic | Dynamically sets visibility based on conditions | When visibility needs to change based on logic |
JavaScript | Hides CheckBox on the client-side after page load | For dynamic UI changes without a postback |
Each method has its unique advantages, and the choice largely depends on the specific requirements of your application and how you want the user experience to be managed. Be sure to consider performance and usability when deciding on the best approach to hide your ASPX CheckBox.
Strategies for Hiding ASPX Checkboxes Effectively
Jessica Lee (Senior Web Developer, Tech Innovations Inc.). “To make an ASPX checkbox not visible, you can set its `Visible` property to “ in the code-behind file. This approach ensures that the checkbox is not rendered in the HTML output, effectively removing it from the user interface.”
Michael Chen (UI/UX Designer, Creative Solutions Group). “Hiding an ASPX checkbox can also be achieved through CSS. By applying a style rule that sets `display: none;` to the checkbox, you can ensure it remains in the DOM but is not visible to the user, which can be useful for maintaining form structure.”
Laura Patel (Software Engineer, Web Dynamics). “In scenarios where you need to conditionally hide a checkbox based on user actions, leveraging JavaScript to manipulate the checkbox’s visibility dynamically is effective. This method allows for a more interactive user experience while keeping the checkbox accessible for future use.”
Frequently Asked Questions (FAQs)
How can I make an ASPX checkbox not visible on the page?
You can make an ASPX checkbox not visible by setting its `Visible` property to “ in your code-behind file. For example: `CheckBox1.Visible = ;`.
Is there a way to hide the checkbox using CSS?
Yes, you can hide the checkbox using CSS by applying a style rule that sets `display: none;` to the checkbox’s ID or class. For example: `CheckBox1 { display: none; }`.
Can I conditionally hide an ASPX checkbox based on user input?
Yes, you can conditionally hide an ASPX checkbox by using server-side logic in your code-behind file. For instance, based on a user action, you can set the `Visible` property accordingly.
What happens to the checkbox when it is set to not visible?
When an ASPX checkbox is set to not visible, it will not be rendered in the HTML output, and it will not occupy any space on the page. This means users cannot interact with it.
Are there any performance implications of hiding checkboxes in ASPX?
Generally, hiding checkboxes using the `Visible` property does not have significant performance implications. However, if you have many controls being hidden or shown dynamically, it may affect rendering time slightly.
Can I use JavaScript to hide an ASPX checkbox after the page loads?
Yes, you can use JavaScript or jQuery to hide an ASPX checkbox after the page loads by selecting the checkbox and setting its CSS `display` property to `none`. For example: `document.getElementById(‘CheckBox1’).style.display = ‘none’;`.
In summary, making an ASP.NET Checkbox control not visible can be achieved through various methods, primarily by manipulating the properties of the Checkbox control itself. The most straightforward approach is to set the `Visible` property of the Checkbox to “. This effectively removes the Checkbox from the rendered page, ensuring that it does not occupy any space in the layout.
Another method involves using CSS to control the visibility of the Checkbox. By applying styles such as `display: none;` or `visibility: hidden;`, developers can hide the Checkbox while still maintaining its presence in the HTML structure. This flexibility allows for more dynamic user interfaces where elements can be shown or hidden based on user interactions or specific conditions.
It is important to consider the implications of hiding UI elements. Hiding a Checkbox may affect user experience and accessibility. Therefore, developers should ensure that the rationale for hiding the Checkbox is clear and that it aligns with the overall design and functionality of the application. Proper documentation and comments in the code can also help other developers understand the reasoning behind such decisions.
Author Profile
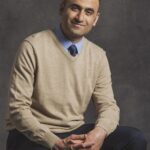
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?