Why Am I Seeing ‘Module Not Found: Can’t Resolve fs’ and How Can I Fix It?
In the world of software development, encountering errors is an inevitable part of the journey. One such frustrating error that many developers face is the infamous “module not found: can’t resolve fs.” This message can halt progress and leave even experienced programmers scratching their heads. The `fs` module, a core component of Node.js, is essential for file system operations, and when it becomes unreachable, it can disrupt the flow of an entire project. Understanding the nuances behind this error is crucial for developers who want to keep their applications running smoothly and efficiently.
This article delves into the intricacies of the “module not found: can’t resolve fs” error, exploring its common causes and practical solutions. We will examine how the structure of your project, the environment in which you’re working, and the dependencies you manage can impact the accessibility of the `fs` module. By shedding light on these factors, we aim to equip you with the knowledge needed to troubleshoot and resolve this issue effectively.
As we navigate through the potential pitfalls that lead to this error, we’ll provide insights into best practices for project organization and dependency management. Whether you’re a seasoned developer or just starting out, understanding the underlying principles of module resolution in Node.js will empower you to tackle this and similar challenges with confidence. Join
Troubleshooting “Module Not Found” Errors in Node.js
Encountering the error “Module not found: Can’t resolve ‘fs'” typically indicates that the code is attempting to import the ‘fs’ module in an environment where it is not available. The ‘fs’ module is a core Node.js module designed for file system operations and is not accessible in client-side JavaScript environments, such as those created with frameworks like React or Angular.
To resolve this issue, consider the following steps:
- Check the Environment: Ensure that the code using the ‘fs’ module is running in a Node.js environment. If your project is a front-end application, you will need to refactor your code to avoid using ‘fs’ directly in the client-side context.
- Conditional Imports: If you must use ‘fs’ in a mixed environment (both client-side and server-side), you can conditionally import the module using dynamic imports or checking the environment:
“`javascript
let fs;
if (typeof window === ”) {
fs = require(‘fs’);
}
“`
- Use Alternative Libraries: For client-side applications, consider using libraries that mimic file system operations without direct access to the Node.js ‘fs’ module. Libraries like `browserify-fs` or `localforage` can provide similar functionalities.
Understanding Module Resolution in Webpack
Webpack manages module resolution and bundling for JavaScript applications, but it has some limitations regarding core Node.js modules. When Webpack encounters an import statement for a module like ‘fs’, it will look for that module in the context of the browser, leading to the “Module not found” error.
To avoid such issues, take note of the following:
- Webpack Configuration: Update your Webpack configuration to ignore certain Node.js modules when building for the web. This can be done by adding the following to your `webpack.config.js`:
“`javascript
module.exports = {
// other configurations…
resolve: {
fallback: {
fs:
}
}
};
“`
- Environment-Specific Code: Structure your code to separate server-side logic from client-side logic. This practice can prevent unnecessary imports and potential errors in your builds.
Error Message | Possible Cause | Solution |
---|---|---|
Module not found: Can’t resolve ‘fs’ | Using ‘fs’ in a client-side context | Refactor code to avoid ‘fs’ in the browser |
Module not found: Can’t resolve ‘path’ | Incorrect import statement | Check for typos and ensure correct module usage |
Module not found: Can’t resolve ‘express’ | Missing dependency in package.json | Run `npm install express` to install |
By understanding the context in which your code runs and adjusting your imports and project configuration, you can effectively resolve these common module resolution errors.
Understanding the ‘fs’ Module
The ‘fs’ module in Node.js provides an API for interacting with the file system. It allows developers to perform actions such as reading, writing, and manipulating files and directories. However, it is important to note that the ‘fs’ module is specific to Node.js and is not available in browser environments.
Common Causes of ‘Module Not Found: Can’t Resolve fs’
Several issues can lead to the error message stating that the module ‘fs’ cannot be resolved. Identifying these causes is crucial for troubleshooting:
- Environment Mismatch:
- Attempting to use ‘fs’ in a frontend JavaScript context (e.g., React, Vue) where Node.js APIs are not accessible.
- Incorrect Configuration:
- Webpack or similar bundlers may be improperly configured, causing them to try to resolve server-side modules in a client-side context.
- Missing Dependencies:
- The project might have missing or improperly installed packages that rely on ‘fs’.
Troubleshooting Steps
To resolve the ‘fs’ module not found error, consider the following troubleshooting steps:
- Check the Environment:
- Ensure that the code utilizing ‘fs’ is intended for a Node.js environment. If using frameworks like React, consider alternative methods to handle file operations.
- Review Webpack Configuration:
- Open the Webpack configuration file (usually `webpack.config.js`).
- Ensure that the `target` property is set to `node` if your application is a Node.js server application.
- Example:
“`javascript
module.exports = {
target: ‘node’,
// other configuration options
};
“`
- Install Missing Dependencies:
- Run the following commands to install any missing dependencies:
“`bash
npm install fs –save
“`
- Use Alternative Solutions:
- For frontend applications, consider using browser-compatible libraries like `browserify-fs` or `file-saver` for file handling.
Configuration Example
Here’s an example of a simple Webpack configuration that correctly targets Node.js:
“`javascript
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
target: ‘node’, // Ensure the target is set to node
resolve: {
fallback: {
fs: , // Disable fs for client-side code
},
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: ‘babel-loader’,
},
},
],
},
};
“`
Alternative Methods for File Handling in Frontend
If you need to handle files on the frontend, consider the following alternatives:
- File API: Utilize the HTML5 File API for file uploads and manipulation.
- IndexedDB: For storage of files in the browser.
- Third-party Libraries:
- FileSaver.js: For saving files on the client-side.
- axios: For making requests to upload or download files.
Conclusion of Troubleshooting
By following these steps and understanding the context in which the ‘fs’ module is utilized, you can effectively resolve the ‘module not found: can’t resolve fs’ error. Proper configuration and usage of the appropriate tools will facilitate a smoother development experience.
Resolving the ‘Module Not Found: Can’t Resolve FS’ Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘module not found: can’t resolve fs’ error typically arises in environments like React or other front-end frameworks where the ‘fs’ module, which is a Node.js core module, is not available. Developers should ensure they are not attempting to use Node.js specific modules in the browser context.”
Michael Chen (Full Stack Developer, CodeCraft Solutions). “To effectively troubleshoot this error, it is crucial to verify your project setup. If you are using a bundler like Webpack, ensure that your configuration is correctly set up to handle Node.js modules, or consider using alternatives like ‘browserify’ for front-end applications.”
Sarah Thompson (Technical Lead, DevOps Experts). “In many cases, the error can be mitigated by refactoring your code to avoid direct imports of Node.js modules in client-side code. Utilizing environment variables or alternative libraries that provide similar functionalities can often resolve the issue without compromising application performance.”
Frequently Asked Questions (FAQs)
What does the error “module not found: can’t resolve fs” mean?
This error indicates that the Node.js `fs` (file system) module cannot be found in your project. This typically occurs in environments that do not support Node.js modules, such as browser-based applications.
Why am I encountering this error in a React application?
React applications run in the browser, which does not have access to Node.js core modules like `fs`. Attempting to use `fs` in a React app will result in this error since it is not available in the browser environment.
How can I resolve the “module not found: can’t resolve fs” error?
To resolve this error, you should avoid using the `fs` module in your client-side code. If you need file system access, consider using server-side code or libraries designed for the browser, such as the File API.
Are there any alternatives to the `fs` module for front-end applications?
Yes, alternatives include using the File API for file uploads and downloads, or libraries like `browserify-fs` or `memfs` which simulate file system operations in the browser environment.
Can I use `fs` in a server-side rendered application?
Yes, if you are using server-side rendering with frameworks like Next.js, you can use the `fs` module in your server-side code. Ensure that the code accessing `fs` is not executed on the client-side.
What should I do if I need to access files in a web application?
Use the File API to handle file uploads and downloads in the browser. For server-side file operations, implement an API endpoint that interacts with the `fs` module and communicates with the client-side application.
The error message “module not found: can’t resolve fs” typically arises in JavaScript environments, especially when working with Node.js or frameworks like React. This issue indicates that the code is attempting to access the ‘fs’ (file system) module, which is native to Node.js but not available in browser environments. Understanding the context in which this error occurs is crucial for effective troubleshooting.
One of the primary reasons for encountering this error is the attempt to use Node.js-specific modules in a client-side application. Developers must recognize that while Node.js provides a rich set of modules for server-side programming, these modules are not accessible in the browser. Consequently, alternatives must be sought, such as using browser-compatible libraries or APIs that can fulfill similar functionalities without relying on the ‘fs’ module.
Another key takeaway is the importance of environment awareness when developing applications. Developers should ensure that they are using the correct tools and libraries for their intended environment, whether it be server-side or client-side. Additionally, utilizing build tools like Webpack or Babel can help manage dependencies and resolve issues related to module compatibility, thereby reducing the likelihood of encountering such errors.
addressing the “module not found: can’t resolve fs” error requires
Author Profile
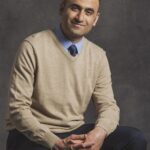
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?