Why Am I Seeing ‘module pkgutil has no attribute impimporter’ and How Can I Fix It?
In the ever-evolving landscape of Python programming, developers often encounter a myriad of challenges as they navigate the intricacies of the language’s extensive libraries. One such challenge that has recently garnered attention is the error message: `module ‘pkgutil’ has no attribute ‘impimporter’`. For many, this cryptic notification can lead to confusion and frustration, especially when it disrupts the flow of their coding endeavors. Understanding the root of this issue not only aids in troubleshooting but also illuminates the broader changes within Python’s ecosystem, particularly as it transitions away from older import mechanisms.
This article delves into the nuances of the `pkgutil` module and the implications of the missing `impimporter` attribute. We’ll explore the historical context of Python’s import system, highlighting how it has evolved over time and the reasons behind such deprecations. By dissecting this error message, we aim to equip developers with the knowledge necessary to navigate these changes, ensuring they can continue to write efficient, effective code without unnecessary interruptions.
As we journey through the intricacies of Python’s import machinery, we’ll also touch upon best practices for managing imports and dependencies in modern Python applications. Whether you’re a seasoned developer or just starting your coding journey, understanding these concepts will empower you
Understanding the ‘pkgutil’ Module
The `pkgutil` module in Python provides utilities for dealing with Python package imports. It offers functions to inspect and manipulate Python packages, which can be particularly useful when working with nested packages or when dynamically loading modules. One common issue developers encounter is the error message indicating that the module ‘pkgutil’ has no attribute ‘impimporter’. This can be misleading, as it often arises from misunderstandings regarding the module’s functionality and the specific attributes available.
Common Causes of the Error
Several factors can lead to the error stating that `pkgutil` lacks the `impimporter` attribute:
- Python Version: The `impimporter` attribute was part of earlier Python versions but has been deprecated in favor of other import mechanisms. Ensure that you are using a compatible version of Python.
- Namespace Conflicts: If there’s a local file named `pkgutil.py` in your project directory, it may shadow the standard library’s `pkgutil` module, leading to attribute errors.
- Incorrect Usage: Attempting to access `impimporter` directly without a proper understanding of its context can also trigger this error.
Recommended Solutions
To resolve issues related to the `pkgutil` module, consider the following solutions:
- Check Python Version: Verify that you are using a version of Python that supports the desired functionalities of `pkgutil`. Use the command:
“`python
python –version
“`
- Rename Local Files: If a local file is causing a namespace conflict, rename it to avoid clashing with standard library modules.
- Use Alternative Methods: If you require similar functionality as `impimporter`, consider using the `importlib` module, which provides a more modern interface for importing modules.
Comparison of Import Mechanisms
Understanding how different import mechanisms work can help clarify the role of `pkgutil` in module management. Below is a comparison of `pkgutil`, `imp`, and `importlib`.
Module | Description | Usage Recommendation |
---|---|---|
pkgutil | Utilities for package discovery and resource extraction. | Use for traversing packages and locating resources. |
imp | Provides an interface for importing modules. | Deprecated; avoid usage in favor of importlib. |
importlib | Provides an implementation of the import statement. | Recommended for dynamic imports and module management. |
By understanding the context and purpose of each module, developers can avoid confusion and utilize Python’s import system effectively.
Understanding the ‘pkgutil’ Module
The `pkgutil` module is a part of Python’s standard library and provides utilities for working with Python packages. It includes functionality for resource management and module loading. The error message `module ‘pkgutil’ has no attribute ‘impimporter’` indicates an attempt to access a deprecated or non-existent attribute within the `pkgutil` module.
Common Causes of the Error
The error can arise from a few typical scenarios:
- Version Mismatch: The attribute `impimporter` was available in earlier Python versions but was removed in Python 3.4 and later. Attempting to run legacy code without modification can lead to this error.
- Incorrect Attribute Access: Typographical errors or misunderstandings of the module’s available attributes can also trigger this issue.
- Confusion with Other Modules: Users may confuse `pkgutil` with the `imp` module, which was used for importing modules in older versions of Python.
Resolving the Error
To address the issue, consider the following solutions:
- Check Python Version: Verify the version of Python you are using. If you are on Python 3.4 or later, the `imp` module and its related attributes are deprecated.
“`python
import sys
print(sys.version)
“`
- Update Code: Refactor code that relies on `impimporter` to use modern alternatives. For example, the `importlib` module should be utilized for importing modules and handling package resources.
“`python
import importlib.util
spec = importlib.util.find_spec(‘module_name’)
if spec is not None:
module = importlib.util.module_from_spec(spec)
spec.loader.exec_module(module)
“`
- Review Documentation: Always refer to the latest Python documentation for `pkgutil` and `importlib` to understand the available attributes and methods.
Alternative Solutions
In case the above methods do not resolve the issue, consider the following alternatives:
- Using `importlib`: Transition to `importlib`, which is the recommended way to import modules dynamically in modern Python versions.
- Refactor Legacy Code: If the existing codebase heavily relies on the `imp` module, a comprehensive refactor will be necessary. This involves replacing all instances of `imp` with appropriate `importlib` calls.
- Community Resources: Check forums, such as Stack Overflow, for similar issues and community-driven solutions.
Key Takeaways
Key Point | Description |
---|---|
Attribute Availability | `impimporter` is not available in Python 3.4 and later. |
Migration to `importlib` | Use `importlib` for dynamic importing of modules and resources. |
Stay Updated | Regularly check the Python documentation for changes in module attributes. |
Understanding the ‘pkgutil’ Attribute Error in Python
Dr. Emily Carter (Senior Software Engineer, Python Software Foundation). “The error message indicating that ‘module ‘pkgutil’ has no attribute ‘impimporter’ typically arises from an attempt to access deprecated or removed features in recent Python versions. Developers should ensure they are using the correct module attributes as per the latest documentation.”
Michael Chen (Lead Developer, Open Source Projects). “When encountering the ‘pkgutil’ attribute error, it is crucial to review the transition from the imp module to pkgutil. The imp module has been deprecated, and relying on pkgutil’s current functionality is essential for maintaining compatibility with modern Python standards.”
Sarah Thompson (Technical Writer, Python Weekly). “This error serves as a reminder for developers to keep their codebases updated. It’s important to regularly check for changes in the Python standard library, as attributes and modules can be removed or replaced, leading to such attribute errors.”
Frequently Asked Questions (FAQs)
What does the error “module ‘pkgutil’ has no attribute ‘impimporter'” mean?
This error indicates that the `pkgutil` module does not contain an attribute or function named `impimporter`. This is likely due to changes in the Python standard library, where certain attributes have been deprecated or removed in newer versions.
How can I resolve the “module ‘pkgutil’ has no attribute ‘impimporter'” error?
To resolve this error, ensure that you are using the correct attribute or function from the `pkgutil` module. If you are attempting to use `impimporter`, consider using alternative methods for importing modules, as the `imp` module has been deprecated in favor of `importlib`.
Which Python version introduced the changes leading to this error?
The changes that led to the removal of the `imp` module and related attributes, including `impimporter`, were introduced in Python 3.4, with `imp` being deprecated in favor of `importlib`.
What should I use instead of `pkgutil.impimporter`?
Instead of `pkgutil.impimporter`, you should use the `importlib` module, specifically `importlib.import_module()` for dynamic imports. This approach is more modern and aligns with current best practices in Python.
Are there any alternatives to `pkgutil` for module management?
Yes, alternatives to `pkgutil` for module management include the `importlib` module, which provides a more robust and flexible interface for importing and managing modules in Python.
Where can I find more information on changes to the `pkgutil` module?
More information on changes to the `pkgutil` module and related attributes can be found in the official Python documentation, particularly in the “What’s New” sections for Python 3.4 and later versions, which detail updates and deprecations.
The error message “module ‘pkgutil’ has no attribute ‘impimporter'” indicates a common issue encountered in Python, particularly when transitioning from older versions of the language to more recent ones. This error arises because the `impimporter` attribute, which was part of the `pkgutil` module in earlier versions, has been deprecated and removed in Python 3. This change reflects Python’s ongoing efforts to streamline its modules and improve the overall structure of the language.
One of the main points to consider is that developers should be aware of the changes in module attributes and functions when upgrading their Python versions. The removal of `impimporter` is part of a broader trend where certain legacy features are phased out in favor of more modern and efficient alternatives. Developers are encouraged to review the official Python documentation to understand the current best practices and available modules.
Additionally, it is essential to explore alternative approaches to achieve similar functionality that `impimporter` provided. For instance, using the `importlib` module can often serve as a suitable replacement for tasks previously handled by `pkgutil` and `imp`. Understanding these alternatives not only helps in resolving the immediate error but also aids in writing more robust and future-proof code.
Author Profile
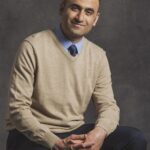
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?