How to Resolve ‘ModuleNotFoundError: No Module Named ‘ConfigParser’ in Your Python Project?
In the world of Python programming, encountering errors is an inevitable part of the development journey. One such common hurdle is the dreaded `ModuleNotFoundError: No module named ‘configparser’`. This error can be particularly frustrating for both novice and seasoned developers alike, as it interrupts the flow of coding and can lead to confusion about the underlying causes. Understanding this error is essential for anyone working with configuration files in Python, as it not only highlights potential issues in your environment but also serves as a gateway to mastering the intricacies of module management and package installation.
When you see the `ModuleNotFoundError`, it signifies that Python is unable to locate the specified module, in this case, `configparser`. This module plays a crucial role in managing configuration files, allowing developers to read and write settings in a structured format. However, the error may arise for various reasons, such as incorrect installation, version discrepancies, or even simple typos in the code. By unraveling the complexities surrounding this error, you can enhance your troubleshooting skills and ensure a smoother development experience.
As we delve deeper into the nuances of the `ModuleNotFoundError: No module named ‘configparser’`, we will explore the common pitfalls that lead to this issue, effective strategies for resolution
Understanding the Error
The `ModuleNotFoundError: No module named ‘configparser’` typically indicates that Python is unable to locate the `configparser` module, which is crucial for reading configuration files in a structured format. This error is common, especially among users who are transitioning from Python 2 to Python 3, as `configparser` was renamed from `ConfigParser` in Python 2.
Common scenarios that lead to this error include:
- The module is not installed in your current Python environment.
- You are using a version of Python that does not include `configparser` by default (e.g., Python 2).
- There is a misconfiguration in your Python environment or virtual environment.
Installing the ConfigParser Module
For those using Python 3, `configparser` is included in the standard library, and no additional installation is typically required. However, if you are using Python 2, you may need to install it manually via pip. Here’s how to check your Python version and install the required module:
- Check Python Version:
Open your terminal or command prompt and run:
“`bash
python –version
“`
This will display the current version of Python you are using.
- Install the Module (if using Python 2):
If you are using Python 2 and need to install `ConfigParser`, you can do so with the following command:
“`bash
pip install ConfigParser
“`
Using Virtual Environments
To avoid conflicts between different projects, it is recommended to use a virtual environment. This isolates your project’s dependencies and prevents issues like the `ModuleNotFoundError`.
To create and activate a virtual environment, follow these steps:
- Create a Virtual Environment:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Required Packages:
After activating your environment, install the necessary packages:
“`bash
pip install configparser
“`
Common Solutions
If you encounter the `ModuleNotFoundError`, consider the following troubleshooting steps:
- Check Python Version: Ensure you are using the correct version of Python.
- Verify Installation: Use pip to check if `configparser` is installed:
“`bash
pip show configparser
“`
- Reinstall the Module: If it is installed but still not found, try reinstalling it:
“`bash
pip uninstall configparser
pip install configparser
“`
Comparison of ConfigParser and configparser
For clarity, here’s a table comparing the key differences between `ConfigParser` in Python 2 and `configparser` in Python 3:
Feature | Python 2 (ConfigParser) | Python 3 (configparser) |
---|---|---|
Module Name | ConfigParser | configparser |
Case Sensitivity | Case insensitive | Case sensitive |
Default Section | Supported | Supported |
Interpolation | Limited | Enhanced |
By understanding these differences and ensuring the correct environment setup, users can effectively manage configuration files in their Python applications without encountering the `ModuleNotFoundError`.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘configparser’` indicates that Python is unable to locate the `configparser` module. This can occur due to several reasons, including:
- The module is not installed in the current Python environment.
- The code is being run in a Python version that does not support `configparser` as a standalone module.
- There is a typo in the module name or an issue with the import statement.
Python Version Compatibility
The `configparser` module is part of the standard library in Python 3. If you are using Python 2, the module is named `ConfigParser` (with an uppercase ‘C’). To check your Python version, you can run the following command in your terminal:
“`bash
python –version
“`
If you are using Python 2, update your import statement as follows:
“`python
import ConfigParser For Python 2
“`
For Python 3, ensure that you are using:
“`python
import configparser For Python 3
“`
Installing the Module
If you are using Python 3 and still encounter the error, it is possible that the module is not installed or there is an issue with your Python environment. To install `configparser`, run the following command:
“`bash
pip install configparser
“`
This command will install the module in your current environment. If you are using a virtual environment, ensure it is activated before running the command.
Common Troubleshooting Steps
To resolve the `ModuleNotFoundError`, consider the following troubleshooting steps:
- Verify the Python Environment: Ensure you are operating in the correct Python environment where `configparser` is installed.
- Check for Typos: Confirm that there are no typographical errors in your import statement.
- Reinstall the Module: If the module is installed but still not found, try uninstalling and reinstalling it:
“`bash
pip uninstall configparser
pip install configparser
“`
- Use Virtual Environments: If you are not already using a virtual environment, consider creating one. This helps manage dependencies effectively:
“`bash
python -m venv myenv
source myenv/bin/activate On Unix or MacOS
myenv\Scripts\activate On Windows
“`
Then install your required packages within this environment.
Conclusion of Troubleshooting
Following the steps outlined above should help resolve the `ModuleNotFoundError: No module named ‘configparser’`. If the issue persists, consider checking the official Python documentation or community forums for additional support.
Understanding the `ModuleNotFoundError` in Python
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “The error ‘ModuleNotFoundError: No module named ‘configparser” typically arises when the Python interpreter cannot locate the specified module. This often occurs due to a missing installation or an incorrect Python environment setup. It is crucial to ensure that the module is installed in the environment you are currently using.”
James Liu (Python Developer, Open Source Advocate). “When encountering the ‘ModuleNotFoundError’ for ‘configparser’, one should first verify the Python version. The ‘configparser’ module is included in Python 3, but if you are using Python 2, you need to install the backport as ‘ConfigParser’. Always check your version compatibility before proceeding.”
Sarah Thompson (Technical Support Specialist, CodeHelp). “Resolving ‘ModuleNotFoundError: No module named ‘configparser” can often be as simple as running ‘pip install configparser’ in your command line. However, if the error persists, ensure that your PYTHONPATH is set correctly and that you are not in a virtual environment that lacks the module.”
Frequently Asked Questions (FAQs)
What does the error “modulenotfounderror: no module named ‘configparser'” indicate?
This error indicates that Python cannot find the `configparser` module, which is typically used for handling configuration files. This may occur if the module is not installed or if there is a typo in the import statement.
How can I resolve the “modulenotfounderror: no module named ‘configparser'” error?
To resolve this error, ensure that you are using Python 3, as `configparser` is included in the standard library. If you are using Python 2, you should use `ConfigParser` instead. Additionally, verify that your Python environment is correctly set up.
Is the ‘configparser’ module available in all Python versions?
The `configparser` module is available in Python 3.x as part of the standard library. In Python 2.x, it is named `ConfigParser` with a capital ‘C’ and ‘P’.
How do I install the ‘configparser’ module if I’m using Python 2?
If you are using Python 2 and need to install `configparser`, you can use the following command: `pip install configparser`. This will install the backport of the module for Python 2 environments.
What should I check if I still encounter the error after installation?
If the error persists after installation, check your Python environment and ensure that you are executing the script with the correct Python interpreter. Additionally, verify that there are no conflicting module names in your project.
Can I use ‘configparser’ in a virtual environment?
Yes, you can use `configparser` in a virtual environment. Ensure that you activate the virtual environment before running your script, and install any required packages within that environment to avoid conflicts.
The error message “ModuleNotFoundError: No module named ‘configparser'” typically indicates that the Python interpreter cannot locate the specified module in the current environment. This issue often arises when a user attempts to import the ‘configparser’ module in a Python 2 environment, where it is named ‘ConfigParser’ instead. It is crucial for users to be aware of the differences in module naming conventions between Python 2 and Python 3 to avoid such errors.
To resolve this error, users should first verify the version of Python they are using. If they are working in a Python 3 environment, the ‘configparser’ module should be available by default. However, if they are using Python 2, they need to adjust their import statement to ‘import ConfigParser’ instead. Additionally, users should ensure that their Python environment is properly set up and that there are no conflicting installations that could lead to module resolution issues.
Another important takeaway is the significance of virtual environments in Python development. By utilizing virtual environments, users can create isolated spaces for their projects, which helps manage dependencies and avoid conflicts between packages. This practice can significantly reduce the likelihood of encountering module-related errors, including the ‘ModuleNotFoundError’ for ‘config
Author Profile
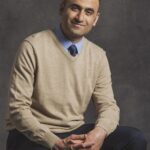
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?