How to Fix the ModuleNotFoundError: No Module Named ‘keras.src.engine’?
In the dynamic world of machine learning and deep learning, Keras has emerged as a go-to library for developers and researchers alike. Its user-friendly interface and powerful capabilities make it a staple in building neural networks. However, as with any evolving software, users often encounter challenges that can hinder their progress. One such common obstacle is the dreaded `ModuleNotFoundError: No module named ‘keras.src.engine’`. This error can be frustrating, especially for those eager to dive into their projects. In this article, we will unravel the intricacies behind this error, exploring its causes and providing actionable solutions to get you back on track.
When you encounter the `ModuleNotFoundError`, it typically indicates that Python cannot locate the specified module within your environment. This can stem from a variety of issues, including version mismatches, improper installations, or changes in the library’s structure. As Keras continues to evolve, certain modules may be relocated or deprecated, leading to confusion among users who rely on previous documentation or code snippets. Understanding the root causes of this error is essential for troubleshooting effectively and ensuring a smooth development experience.
Moreover, resolving this error not only enhances your coding skills but also deepens your understanding of how Keras and its underlying components function. By familiarizing
Understanding the Error
The error `ModuleNotFoundError: No module named ‘keras.src.engine’` indicates that Python is unable to locate the specified module within the Keras library. This issue can arise due to several reasons, primarily related to installation and version compatibility.
Common causes for this error include:
- Incorrect Installation: Keras may not be installed correctly or could be missing altogether.
- Version Mismatch: The installed version of Keras might not support the structure used in your code.
- Environment Issues: The Python environment may not have access to the installed packages, particularly when using virtual environments.
Troubleshooting Steps
To resolve the `ModuleNotFoundError`, follow these troubleshooting steps:
- Verify Installation: Ensure that Keras is installed in your Python environment. You can check this by running:
“`bash
pip show keras
“`
- Install or Upgrade Keras: If Keras is not installed or is outdated, you can install or upgrade it using the following command:
“`bash
pip install –upgrade keras
“`
- Check Python Environment: Make sure you are using the correct Python environment where Keras is installed. If you are using a virtual environment, activate it before running your scripts.
- Import Statement Review: Ensure that your import statements are correct. For example:
“`python
from keras import models
“`
- Consult Documentation: Refer to the official Keras documentation for the version you are using to ensure you are following the correct module structure.
Common Solutions
Here are some common solutions to address the `ModuleNotFoundError` related to Keras:
- Reinstall Keras: If you suspect a corrupt installation, uninstall and reinstall Keras:
“`bash
pip uninstall keras
pip install keras
“`
- Check for Typos: Ensure that there are no typos in your import statements or anywhere in your code that references Keras.
- Use Compatible Versions: Sometimes, libraries like TensorFlow and Keras need to be compatible. Ensure that you are using versions of TensorFlow and Keras that are known to work together.
Package | Version | Compatibility |
---|---|---|
Keras | 2.10.0 | Compatible with TensorFlow 2.10.0 |
Keras | 2.9.0 | Compatible with TensorFlow 2.9.0 |
By following these steps, you should be able to resolve the `ModuleNotFoundError` and ensure that Keras is correctly integrated into your Python projects.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘keras.src.engine’` typically indicates that Python is unable to locate the specified module, often due to changes in the library structure or installation issues. This error can arise in various scenarios, particularly when working with Keras and TensorFlow.
Common Causes
Several factors may contribute to this error:
- Library Version Mismatch: The version of Keras or TensorFlow you are using may not have the `keras.src.engine` module.
- Improper Installation: Keras may not be installed correctly, or it may be missing dependencies.
- Incorrect Import Statements: The way you are importing Keras modules may be outdated or incorrect.
Troubleshooting Steps
To resolve the `ModuleNotFoundError`, consider the following troubleshooting steps:
- Check Installed Versions:
- Use the following commands in your terminal:
“`bash
pip show keras
pip show tensorflow
“`
- Ensure that you have compatible versions. Keras is now integrated with TensorFlow, so using TensorFlow 2.x is recommended.
- Upgrade or Reinstall Keras and TensorFlow:
- Upgrade both libraries to ensure you have the latest versions:
“`bash
pip install –upgrade keras tensorflow
“`
- If issues persist, consider reinstalling them:
“`bash
pip uninstall keras tensorflow
pip install keras tensorflow
“`
- Check Import Statements:
- Make sure to use the correct import syntax. For example:
“`python
from tensorflow import keras
“`
- Avoid using `import keras` directly, as it may lead to confusion with the standalone Keras package.
- Verify Environment:
- Ensure you are working in the correct Python environment where Keras and TensorFlow are installed, especially if using virtual environments.
Example of Correct Usage
Here’s an example of how to properly import and use Keras with TensorFlow:
“`python
import tensorflow as tf
Example model creation
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(64, activation=’relu’, input_shape=(32,)),
tf.keras.layers.Dense(10, activation=’softmax’)
])
“`
Additional Resources
For further assistance, consider exploring the following resources:
Resource | Description |
---|---|
Keras Documentation | Official documentation for Keras. |
TensorFlow Documentation | Guidance on using TensorFlow with Keras. |
GitHub Issues | Community discussions and solutions. |
Utilizing these resources can provide deeper insights into resolving module-related issues and enhance your understanding of Keras and TensorFlow integration.
Resolving the `ModuleNotFoundError` in Keras: Expert Insights
Dr. Emily Chen (Machine Learning Researcher, AI Innovations Lab). “The error `modulenotfounderror: no module named ‘keras.src.engine’` typically arises when there is a version mismatch between TensorFlow and Keras. It is crucial to ensure that you are using compatible versions of both libraries, as Keras has undergone significant changes in its structure in recent updates.”
Mark Thompson (Senior Software Engineer, Deep Learning Solutions). “To address this error, I recommend checking your installation of Keras. If you are using TensorFlow 2.x, Keras is included within the TensorFlow package. Therefore, importing Keras directly from TensorFlow, such as `from tensorflow import keras`, can often resolve the issue.”
Dr. Sarah Patel (Data Scientist, Tech Research Institute). “In some cases, this error may also indicate that the Keras library is not installed in your current Python environment. Utilizing package managers like pip or conda to reinstall Keras can help eliminate this error, ensuring that all dependencies are correctly set up.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘keras.src.engine'” indicate?
This error indicates that Python is unable to locate the specified module within the Keras package. This often occurs due to an incorrect installation or an outdated version of Keras.
How can I resolve the “ModuleNotFoundError” for Keras?
To resolve this error, ensure that Keras is properly installed in your Python environment. You can reinstall Keras using pip with the command `pip install keras –upgrade` to get the latest version.
Is ‘keras.src.engine’ a valid module in Keras?
As of the latest versions, ‘keras.src.engine’ may not exist as a standalone module. Keras has undergone restructuring, so it is advisable to refer to the official Keras documentation for the correct module paths.
What should I do if I am using TensorFlow’s Keras?
If you are using TensorFlow’s Keras, you should import Keras from TensorFlow directly using `from tensorflow import keras`. This ensures compatibility with TensorFlow’s integrated version of Keras.
Could this error be related to a virtual environment issue?
Yes, this error can occur if Keras is not installed in the active virtual environment. Ensure that you have activated the correct environment and that Keras is installed within it.
How can I check the installed version of Keras?
You can check the installed version of Keras by running the command `pip show keras` in your terminal. This will display the version along with other package details.
The error message “ModuleNotFoundError: No module named ‘keras.src.engine'” typically indicates that the Python interpreter cannot locate the specified module within the Keras library. This issue often arises due to several common factors, including incorrect installation of the Keras package, version incompatibilities, or changes in the library’s structure across different versions. Users may encounter this error when attempting to import specific components from Keras, particularly if they are using an outdated or improperly configured environment.
To resolve this error, users are advised to ensure that they have the latest version of Keras installed. This can be achieved by using package management tools like pip to update Keras. Additionally, it is essential to verify that the correct environment is activated, especially when using virtual environments or Anaconda. Users should also check the official Keras documentation or GitHub repository for any changes to the module structure that may have occurred in recent updates, as these changes can lead to the unavailability of certain modules or paths.
Moreover, it is beneficial for users to familiarize themselves with the Keras API and its evolving architecture. Understanding the current framework and its components can help prevent such errors in the future. Engaging with community forums or resources can also provide insights and solutions
Author Profile
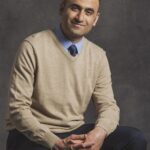
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?