Why Am I Getting a ModuleNotFoundError: No Module Named ‘yaml’?
In the world of Python programming, encountering errors is an inevitable part of the development journey. Among these, the `ModuleNotFoundError: No module named ‘yaml’` stands out as a common yet perplexing hurdle for many developers. This error typically arises when attempting to utilize the powerful capabilities of the PyYAML library, which is essential for parsing and writing YAML files—an increasingly popular data serialization format. Whether you’re working on a small project or a large application, understanding how to troubleshoot and resolve this error can significantly enhance your coding experience and productivity.
As you delve into the intricacies of Python and its vast ecosystem of libraries, the importance of proper module management becomes evident. The `ModuleNotFoundError` serves as a reminder that even the most robust code can falter if the necessary dependencies are not correctly installed or configured. This article will guide you through the common causes of this error, offering insights into how to effectively address it and ensure your projects run smoothly.
By the end of this exploration, you will not only grasp the nuances of the `ModuleNotFoundError` related to YAML but also gain a deeper appreciation for the best practices in managing Python libraries. Armed with this knowledge, you will be better equipped to navigate the challenges of Python
Understanding the ModuleNotFoundError
The `ModuleNotFoundError` is a common error encountered in Python, indicating that the interpreter cannot find the specified module. This error typically arises from various reasons, including:
- The module is not installed in the current environment.
- There is a typo in the module name.
- The Python environment is not set up correctly.
When dealing with YAML files in Python, the error message `No module named ‘yaml’` signifies that the PyYAML library, which provides support for YAML serialization and deserialization, is missing.
Installing PyYAML
To resolve the `ModuleNotFoundError: No module named ‘yaml’`, you need to ensure that the PyYAML library is installed. This can be accomplished using the following methods:
- Using pip (Python’s package manager):
“`bash
pip install pyyaml
“`
- If you are using a virtual environment, ensure that the environment is activated before running the pip command.
Here is a simple command line table for installation methods:
Method | Command |
---|---|
Install via pip | pip install pyyaml |
Install via conda | conda install pyyaml |
Verifying the Installation
Once installed, it is prudent to verify that PyYAML is correctly installed and accessible. You can do this by attempting to import the module in a Python shell or script:
“`python
import yaml
print(yaml.__version__)
“`
If no error is raised, and the version number is printed, the installation is successful.
Troubleshooting Common Issues
If the error persists after installation, consider the following troubleshooting steps:
- Check Python Version: Ensure that the PyYAML installation corresponds to the correct version of Python you are using. Running multiple Python versions can lead to confusion regarding where the module is installed.
- Virtual Environment Activation: Confirm that your virtual environment is activated if you are working within one.
- IDE Configuration: Some Integrated Development Environments (IDEs) may be configured to use a specific interpreter. Verify that your IDE points to the correct Python environment where PyYAML is installed.
By systematically addressing these areas, you can effectively resolve the `ModuleNotFoundError` related to the YAML module in Python.
Understanding the Error
The error message “ModuleNotFoundError: No module named ‘yaml'” indicates that Python cannot locate the PyYAML library, which is required for YAML file operations. YAML (YAML Ain’t Markup Language) is commonly used for configuration files and data exchange.
Common Causes
- Missing Library: The PyYAML library is not installed.
- Virtual Environment Issues: The library is installed in a different environment than the one being used.
- Python Version Conflicts: The installed version of PyYAML is incompatible with the current Python version.
Installing PyYAML
To resolve the error, you need to install the PyYAML library. Follow the steps below based on your environment:
Using pip
For most Python installations, the following command works:
“`bash
pip install pyyaml
“`
Using pip with Virtual Environments
If you are using a virtual environment, ensure it is activated before installing:
“`bash
Activate your virtual environment
source /path/to/venv/bin/activate On macOS/Linux
.\path\to\venv\Scripts\activate On Windows
Then install PyYAML
pip install pyyaml
“`
Installing with Conda
If you are using Anaconda or Miniconda, use:
“`bash
conda install pyyaml
“`
Verifying Installation
After installation, verify that PyYAML is correctly installed:
“`python
import yaml
print(yaml.__version__)
“`
If no error is raised and the version number is displayed, the installation was successful.
Troubleshooting Tips
If you continue to encounter the error after installation, consider the following:
- Check Python Path: Ensure the script is executed in the environment where PyYAML is installed.
- Reinstall PyYAML: Sometimes, reinstalling can resolve underlying issues:
“`bash
pip uninstall pyyaml
pip install pyyaml
“`
- Check for Typos: Ensure there are no misspellings in the import statement:
“`python
import yaml Correct
“`
Additional Resources
- PyYAML Documentation: [PyYAML Documentation](https://pyyaml.org/wiki/PyYAML)
- Python Virtual Environments: [Virtual Environments Documentation](https://docs.python.org/3/tutorial/venv.html)
By following these instructions and tips, you should be able to resolve the “ModuleNotFoundError: No module named ‘yaml'” issue effectively.
Understanding the ModuleNotFoundError: Insights from Python Experts
Dr. Emily Chen (Senior Software Engineer, Python Development Group). “The error ‘ModuleNotFoundError: No module named yaml’ typically indicates that the PyYAML library is not installed in your Python environment. It is crucial to ensure that you are using the correct Python interpreter and that the library is installed using pip.”
Mark Thompson (Python Educator and Author). “When encountering this error, first verify that you have installed the PyYAML package. You can do this by running ‘pip show pyyaml’ in your terminal. If it is not found, you can install it using ‘pip install pyyaml’.”
Sarah Patel (Data Scientist, Tech Innovations Inc.). “This error can also arise if you are working within a virtual environment that does not have PyYAML installed. Always check your environment setup and ensure that all necessary dependencies are included.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘yaml'” indicate?
This error indicates that the Python interpreter cannot find the PyYAML library, which is necessary for handling YAML files in your project.
How can I install the PyYAML module to resolve this error?
You can install the PyYAML module using pip by running the command `pip install pyyaml` in your terminal or command prompt.
What should I do if I have installed PyYAML but still encounter the error?
Ensure that you are using the correct Python environment where PyYAML is installed. You can check the installed packages using `pip list` and confirm the active environment with `which python` or `python -m site`.
Is PyYAML compatible with both Python 2 and Python 3?
Yes, PyYAML is compatible with both Python 2 and Python 3. However, it is recommended to use Python 3 as Python 2 has reached its end of life.
Are there any alternatives to PyYAML for handling YAML files in Python?
Yes, alternatives include libraries such as `rueml` and `oyaml`, which also provide YAML parsing capabilities. However, PyYAML is the most widely used.
How can I verify that PyYAML is installed correctly?
You can verify the installation by running a simple Python script that imports the module:
“`python
import yaml
print(yaml.__version__)
“`
If no error occurs and the version prints, PyYAML is installed correctly.
The error message “ModuleNotFoundError: No module named ‘yaml'” typically indicates that the Python interpreter is unable to locate the PyYAML library, which is essential for working with YAML files in Python. This issue often arises when the library is not installed in the current Python environment or when there is a misconfiguration in the environment itself. Users may encounter this error when attempting to import the yaml module in their scripts, leading to interruptions in their workflow.
To resolve this error, users should first ensure that PyYAML is installed. This can be done using package management tools like pip. Running the command `pip install pyyaml` in the terminal or command prompt will install the module if it is not already present. Additionally, users should verify that they are operating in the correct virtual environment or Python version where PyYAML is installed. This step is crucial, especially in projects that utilize multiple environments or dependencies.
Moreover, it is essential to maintain an organized development environment. Regularly checking for installed packages and their versions can prevent such errors from occurring. Utilizing virtual environments can help isolate project dependencies, reducing the risk of conflicts between different packages. In summary, addressing the “ModuleNotFoundError: No module named ‘yaml
Author Profile
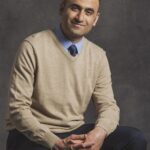
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?