How to Resolve the ‘ModuleNotFoundError: No Module Named ‘yaml” in Python?
In the world of programming, encountering errors is an inevitable part of the journey. One such error that can leave developers scratching their heads is the infamous `ModuleNotFoundError: No module named ‘yaml’`. This error often arises when working with YAML files in Python, a popular format for configuration files and data serialization. Whether you’re a seasoned developer or a newcomer to the Python ecosystem, understanding this error is crucial for smooth project execution and effective debugging.
As you dive into the intricacies of Python’s module system, you’ll discover that the `ModuleNotFoundError` serves as a crucial indicator of missing dependencies in your environment. The specific mention of ‘yaml’ points to the PyYAML library, a widely used package that allows for seamless interaction with YAML data. This article will guide you through the common causes of this error, helping you identify why your Python environment may be lacking the necessary module and how to resolve the issue effectively.
From installation mishaps to environment misconfigurations, the reasons behind this error can vary significantly. By exploring the underlying principles of Python’s package management, we will equip you with the knowledge to troubleshoot and prevent similar issues in the future. Get ready to unlock the secrets of YAML integration in Python and ensure your projects run smoothly without
Understanding the Error
The `ModuleNotFoundError: No module named ‘yaml’` error indicates that Python cannot locate the PyYAML library, which is necessary for working with YAML files. This issue typically arises in environments where the library is not installed or when Python is unable to find it due to configuration issues.
Common causes of this error include:
- The PyYAML library is not installed in the current Python environment.
- The Python interpreter being used is different from the one where PyYAML was installed.
- There are virtual environments in use, and the library has not been installed in the active virtual environment.
Installing PyYAML
To resolve the error, the PyYAML library must be installed. This can be accomplished using the Python package manager, pip. The following command can be executed in the terminal or command prompt:
“`bash
pip install pyyaml
“`
For specific Python versions, you may need to specify the version of pip. For example, if using Python 3, you might use:
“`bash
pip3 install pyyaml
“`
In some cases, you may also require administrative privileges to install packages, which can be done using `sudo` on Linux or macOS:
“`bash
sudo pip install pyyaml
“`
Verifying Installation
After installation, it is prudent to verify that PyYAML is correctly installed. You can do this by attempting to import the library in a Python shell or script:
“`python
import yaml
“`
If this command executes without errors, the installation was successful. If the error persists, consider the following troubleshooting steps.
Troubleshooting Steps
If you continue to experience issues after installation, consider the following:
- Check Python Version: Ensure you are running the correct version of Python. You can check the version with:
“`bash
python –version
“`
- Check Environment: If using virtual environments, ensure you have activated the correct one:
“`bash
source /path/to/your/venv/bin/activate On Linux or macOS
.\path\to\your\venv\Scripts\activate On Windows
“`
- Reinstall PyYAML: If issues persist, you may try uninstalling and then reinstalling PyYAML:
“`bash
pip uninstall pyyaml
pip install pyyaml
“`
- Inspect `PYTHONPATH`: If you have custom paths for Python packages, ensure that the `PYTHONPATH` environment variable is set correctly.
Common Use Cases for PyYAML
PyYAML is widely used for various applications, including:
- Configuration files for applications.
- Serialization and deserialization of Python objects to/from YAML format.
- Data exchange between different programming languages.
The following table summarizes some key features of PyYAML:
Feature | Description |
---|---|
YAML Parsing | Read YAML files and convert them into Python data structures. |
YAML Dumping | Convert Python objects into YAML format for easy readability. |
Support for Custom Python Objects | Allows serialization of custom classes and data types. |
Utilizing PyYAML effectively enhances the functionality of Python applications, particularly in configuration management and data interchange.
Understanding the Error
The error `ModuleNotFoundError: No module named ‘yaml’` indicates that Python is unable to locate the PyYAML package, which is necessary for handling YAML files. This error typically arises in the following scenarios:
- The PyYAML module is not installed in your Python environment.
- The module is installed in a different environment (e.g., a virtual environment).
- There are issues with your Python installation or environment setup.
Installing PyYAML
To resolve the `ModuleNotFoundError`, you need to install the PyYAML package. Follow these steps based on your environment:
Using pip:
- Open your terminal or command prompt.
- Run the following command:
“`bash
pip install pyyaml
“`
Using conda (if you are using Anaconda):
- Open your Anaconda Prompt.
- Execute the following command:
“`bash
conda install pyyaml
“`
Verifying Installation:
After installation, you can verify if PyYAML is correctly installed:
“`bash
pip show pyyaml
“`
This command should display details about the PyYAML installation, confirming its presence.
Checking Python Environment
If you continue to experience issues, it is essential to ensure that you are operating in the correct Python environment. Here are steps to verify your environment:
- Check active environment: You can check which Python environment is currently active using:
“`bash
which python
“`
or
“`bash
python -m site
“`
- Switch environments: If you are using virtual environments, activate the intended environment with:
“`bash
source
“`
Common Troubleshooting Steps
If the error persists after installation, consider the following troubleshooting steps:
- Reinstall PyYAML: Sometimes, a fresh installation can resolve underlying issues. Uninstall PyYAML and reinstall it:
“`bash
pip uninstall pyyaml
pip install pyyaml
“`
- Upgrade pip: An outdated version of pip may cause installation issues. Upgrade pip using:
“`bash
pip install –upgrade pip
“`
- Check Python version compatibility: Ensure that you are using a compatible version of Python. PyYAML supports Python 2.7 and Python 3.5 and above.
Using Virtual Environments
Utilizing virtual environments can help manage dependencies and avoid conflicts. Here’s how to create and use a virtual environment:
- Creating a virtual environment:
“`bash
python -m venv myenv
“`
- Activating the virtual environment:
“`bash
source myenv/bin/activate On macOS/Linux
myenv\Scripts\activate On Windows
“`
- Installing PyYAML within the virtual environment:
“`bash
pip install pyyaml
“`
This method ensures that all dependencies, including PyYAML, are isolated and easily manageable.
Alternative Libraries
If you encounter persistent issues with PyYAML, consider using alternative libraries that offer YAML parsing capabilities:
Library | Description |
---|---|
rueml | A simple YAML parser and emitter for Python. |
oyaml | An ordered YAML parser that maintains order of keys. |
PySyck | An alternative YAML parser, although less common. |
Each of these libraries has its own installation and usage instructions, which can be found in their respective documentation.
Resolving the ‘ModuleNotFoundError’ in Python
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “The ‘ModuleNotFoundError: No module named ‘yaml” typically indicates that the PyYAML library is not installed in your Python environment. To resolve this, you should run ‘pip install PyYAML’ in your terminal or command prompt to install the necessary module.”
Michael Thompson (Python Developer, CodeCraft Academy). “It’s crucial to ensure that you are using the correct Python environment. If you have multiple versions of Python installed, the module might be installed in a different version than the one you are currently using. Utilize ‘pip list’ to check installed packages in your active environment.”
Linda Zhao (Data Scientist, AI Innovations). “In some cases, this error can arise from a virtual environment not being activated. Always ensure that your virtual environment is activated before running your Python scripts, as this ensures that the correct dependencies are loaded.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No module named ‘yaml'” indicate?
This error indicates that the Python interpreter cannot find the PyYAML module in your environment. This typically occurs when the module is not installed or is installed in a different Python environment.
How can I resolve the “ModuleNotFoundError: No module named ‘yaml'” error?
To resolve this error, you should install the PyYAML package using pip. You can do this by running the command `pip install pyyaml` in your terminal or command prompt.
Is PyYAML included in the standard Python library?
No, PyYAML is not included in the standard Python library. It is an external library that must be installed separately to work with YAML files in Python.
What should I do if I have multiple Python versions installed?
If you have multiple Python versions, ensure that you are installing PyYAML for the correct version. Use `pip` associated with that specific Python version, for example, `python3 -m pip install pyyaml` for Python 3.
Can I use a virtual environment to avoid this error?
Yes, using a virtual environment is a recommended practice. It allows you to create isolated environments for your projects, ensuring that dependencies like PyYAML do not conflict with other projects.
What are some common uses of the PyYAML module?
PyYAML is commonly used for parsing and writing YAML files, which are often utilized for configuration files, data serialization, and data exchange between languages.
The error message “ModuleNotFoundError: No module named ‘yaml'” typically indicates that the PyYAML library, which is necessary for working with YAML files in Python, is not installed in the current Python environment. This error can arise in various scenarios, such as when a script attempts to import the PyYAML module without it being available, or when the environment is misconfigured. It is essential for developers and users to ensure that all required libraries are properly installed to avoid such issues.
To resolve this error, users can install the PyYAML module using the package manager pip. The command `pip install pyyaml` can be executed in the terminal or command prompt. It is also advisable to verify that the installation is performed in the correct Python environment, especially when using virtual environments or multiple Python installations. This ensures that the module is accessible to the script that requires it.
Furthermore, users should be aware of the importance of maintaining an organized project structure and environment management. Utilizing virtual environments can help isolate dependencies and prevent conflicts between different projects. Regularly checking for updates and ensuring that all necessary libraries are installed can significantly reduce the likelihood of encountering similar module-related errors in the future.
Author Profile
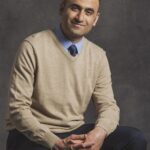
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?