How Can I Find Specific Text in a Stored Procedure in MSSQL?
In the world of database management, Microsoft SQL Server (MSSQL) stands as a powerful tool for handling vast amounts of data with efficiency and precision. As developers and database administrators work with stored procedures—those essential blocks of code that encapsulate complex logic and operations—they often need to search for specific text or patterns within these procedures. Whether it’s for debugging, refactoring, or simply understanding the existing codebase, knowing how to effectively locate text in stored procedures can save valuable time and enhance productivity.
Finding text in stored procedures isn’t just about searching for keywords; it’s about navigating the intricacies of your database’s architecture. With the right techniques, you can uncover valuable insights, identify dependencies, and streamline your workflow. MSSQL provides several methods to facilitate this search, from built-in system views to dynamic management functions. Each approach offers unique advantages, making it essential for users to choose the method that best fits their needs.
As we delve deeper into the topic, we will explore various strategies for locating text within stored procedures, including practical examples and tips for optimizing your search process. Whether you’re a seasoned SQL developer or just starting your journey, mastering these techniques will empower you to manage your stored procedures with confidence and ease. Prepare to unlock the full potential of your MSSQL environment as we
Finding Text in SQL Server Stored Procedures
To locate specific text within SQL Server stored procedures, you can leverage system views and functions that SQL Server provides. The primary system view used for this purpose is `sys.sql_modules`, which contains the definitions of all SQL Server objects, including stored procedures. This can be particularly useful when you need to search for a specific keyword or phrase across multiple stored procedures in your database.
Using T-SQL to Search for Text
You can execute a T-SQL query to search for text within the definition of stored procedures. Here’s a basic example of how to do this:
“`sql
SELECT
OBJECT_NAME(object_id) AS ObjectName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%your_search_text%’
AND OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
In this query:
- `OBJECT_NAME(object_id)` retrieves the name of the stored procedure.
- `definition` contains the actual text of the stored procedure.
- The `LIKE` clause allows you to specify the text you are searching for, using wildcards (`%`) to match any characters before or after the search term.
- The `OBJECTPROPERTY` function filters the results to include only objects that are stored procedures.
Interpreting the Results
When you run the query above, the results will include a list of stored procedures that contain the specified text. This can help you quickly identify which stored procedures may need to be reviewed or modified. Below is an example of how the output might look:
ObjectName | Definition |
---|---|
usp_GetEmployee | CREATE PROCEDURE usp_GetEmployee AS … |
usp_UpdateEmployee | CREATE PROCEDURE usp_UpdateEmployee AS … |
Additional Considerations
When searching for text in stored procedures, consider the following:
- Permissions: Ensure you have the necessary permissions to view the definitions of the stored procedures.
- Performance: Searching through a large number of procedures can be resource-intensive, so use this method judiciously in production environments.
- Text Variations: Be aware of variations in text (e.g., case sensitivity) depending on your SQL Server collation settings.
With these techniques, you can effectively search through stored procedures to find specific text, facilitating better management and maintenance of your SQL Server database.
Searching for Text in Stored Procedures
To find specific text within stored procedures in Microsoft SQL Server, you can utilize system catalog views or built-in functions. This allows you to search for keywords, comments, or any other specific text within the SQL definitions.
Using `sys.sql_modules` and `sys.objects`
The `sys.sql_modules` view contains the definitions of SQL objects, including stored procedures. You can join it with `sys.objects` to filter the results to stored procedures.
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — ‘P’ indicates stored procedures
AND m.definition LIKE ‘%your_search_text%’
“`
Replace `your_search_text` with the text you are searching for. This query returns the names of stored procedures along with their definitions containing the specified text.
Using `OBJECT_DEFINITION` Function
You can also utilize the `OBJECT_DEFINITION` function to get the definition of a specific stored procedure by its name or ID.
“`sql
SELECT
OBJECT_DEFINITION(OBJECT_ID(‘schema.ProcedureName’)) AS ProcedureDefinition
WHERE
OBJECT_DEFINITION(OBJECT_ID(‘schema.ProcedureName’)) LIKE ‘%your_search_text%’
“`
Replace `schema.ProcedureName` with your procedure’s full name and `your_search_text` with the desired text.
Script to Search All Procedures
If you want to search across all stored procedures, you can create a dynamic SQL script that iterates through all stored procedures.
“`sql
DECLARE @ProcedureName NVARCHAR(255)
DECLARE @SQL NVARCHAR(MAX)
DECLARE cursor_procs CURSOR FOR
SELECT name
FROM sys.objects
WHERE type = ‘P’
OPEN cursor_procs
FETCH NEXT FROM cursor_procs INTO @ProcedureName
WHILE @@FETCH_STATUS = 0
BEGIN
SET @SQL = ‘IF OBJECT_ID(”’ + @ProcedureName + ”’) IS NOT NULL
BEGIN
PRINT ”’ + @ProcedureName + ”’
SELECT ”’ + @ProcedureName + ”’ AS ProcedureName,
OBJECT_DEFINITION(OBJECT_ID(”’ + @ProcedureName + ”’)) AS ProcedureDefinition
WHERE
OBJECT_DEFINITION(OBJECT_ID(”’ + @ProcedureName + ”’)) LIKE ”%your_search_text%”
END’
EXEC sp_executesql @SQL
FETCH NEXT FROM cursor_procs INTO @ProcedureName
END
CLOSE cursor_procs
DEALLOCATE cursor_procs
“`
This script prints the names of stored procedures containing the specified text.
Considerations for Searching
When searching for text in stored procedures, consider the following:
- Case Sensitivity: The search may be case-sensitive based on the collation settings of your database.
- Performance: Searching large databases with many stored procedures can be resource-intensive. Optimize queries where possible.
- Dynamic SQL: Be cautious when executing dynamic SQL to avoid SQL injection vulnerabilities.
Using SQL Server Management Studio (SSMS)
In SQL Server Management Studio, you can also find text within stored procedures using the following steps:
- Open SSMS and connect to your database.
- Right-click on the database and select “View Dependencies”.
- In the Object Explorer, expand the “Programmability” node.
- Right-click on “Stored Procedures” and select “Filter” -> “Filter Settings”.
- Enter the text you want to search for in the filter criteria.
This method provides a user-friendly interface to find stored procedures containing specific text without writing queries.
Utilizing the various methods described allows for efficient searching of text within stored procedures in SQL Server, catering to different user preferences and scenarios.
Expert Insights on Finding Text in MSSQL Stored Procedures
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “To effectively find text within stored procedures in MSSQL, utilizing the system views such as sys.sql_modules and sys.objects is essential. These views allow you to query the definition of stored procedures directly, enabling you to search for specific text patterns with SQL’s LIKE operator.”
Mark Thompson (Senior SQL Developer, Data Dynamics). “I recommend leveraging the built-in functionality of SQL Server Management Studio (SSMS) for a more user-friendly approach. By using the ‘Find in Files’ feature, developers can quickly locate text across all stored procedures, making the process efficient and straightforward.”
Linda Garcia (Database Consultant, Optimal Data Solutions). “For large databases, a script that iterates through the stored procedures and checks for specific text can be invaluable. This method not only identifies the procedures containing the text but also provides insights into potential refactoring opportunities.”
Frequently Asked Questions (FAQs)
How can I search for specific text in a stored procedure in MSSQL?
You can search for specific text in a stored procedure by querying the `sys.sql_modules` or `sys.objects` system views. Use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%your_text%’;
“`
Is there a way to find all stored procedures containing a certain keyword?
Yes, you can find all stored procedures containing a certain keyword by using the same approach as above, adjusting the `LIKE` clause to include your keyword. For example:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%keyword%’;
“`
Can I use SQL Server Management Studio (SSMS) to find text in stored procedures?
Yes, you can use SSMS to find text in stored procedures. Open the “Object Explorer,” right-click on the database, select “Find,” and then choose “Find in Database.” Enter your search text and specify the object type as “Stored Procedures.”
What permissions are required to search text in stored procedures?
To search text in stored procedures, you need at least the `VIEW DEFINITION` permission on the database. This permission allows you to access the metadata and definitions of the stored procedures.
Are there any third-party tools that can assist in finding text in stored procedures?
Yes, several third-party tools, such as Redgate SQL Search and ApexSQL Search, can assist in finding text in stored procedures. These tools provide enhanced search capabilities and user-friendly interfaces for navigating database objects.
How can I automate the process of finding text in stored procedures?
You can automate the process by creating a SQL script or stored procedure that encapsulates the search logic. Schedule this script to run at specified intervals using SQL Server Agent, or integrate it into your CI/CD pipeline for regular checks.
In Microsoft SQL Server (MSSQL), finding specific text within stored procedures can be essential for database management and code maintenance. This task can be accomplished using system catalog views, particularly the `sys.sql_modules` and `sys.objects` views. By querying these views, users can search for specific keywords or phrases within the definition of stored procedures, allowing for efficient identification and analysis of code segments that may require updates or refactoring.
One effective approach to locate text in stored procedures involves using a SQL query that combines these system views. For instance, a query can be constructed to filter stored procedures based on the presence of a specific string in their definition. This method not only aids in code review but also assists in ensuring compliance with coding standards and practices. Additionally, it can help in identifying deprecated functions or potential security vulnerabilities that need addressing.
Furthermore, leveraging tools such as SQL Server Management Studio (SSMS) can enhance the search process. SSMS provides a user-friendly interface that allows developers to perform text searches across multiple objects, including stored procedures. This functionality can significantly streamline the process of managing and updating database code, ultimately leading to improved performance and maintainability of the database systems.
Author Profile
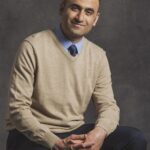
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?