Why Are Napi::FunctionReference::New Calls Triggering the Wrong Constructor from Other Modules?
In the world of Rust and Node.js integration, the `napi` crate serves as a powerful bridge, allowing developers to harness the performance and safety of Rust while leveraging the vast ecosystem of JavaScript. However, as with any complex system, challenges can arise, particularly when it comes to managing function references and constructors across different modules. One such challenge is the perplexing behavior observed when `napi::functionreference::new` seems to invoke the wrong constructor from other modules, leading to confusion and potential bugs in applications.
This article delves into the intricacies of using `napi::functionreference` and the nuances of constructor calls in multi-module environments. We will explore how Rust’s strict type system and JavaScript’s dynamic nature can sometimes clash, resulting in unexpected behavior that can derail even the most carefully crafted code. By understanding the underlying mechanics of `napi` and the common pitfalls developers face, you can better navigate this landscape and ensure your Rust and Node.js applications function as intended.
As we unpack this topic, we will examine the typical scenarios where these constructor call issues arise, along with practical strategies for debugging and resolving them. Whether you’re a seasoned Rustacean or new to the world of Node.js bindings, this exploration will equip you with the insights
Understanding `napi::functionreference::new` Behavior
When utilizing `napi::functionreference::new` in the N-API (Node.js API), it’s crucial to grasp how it interacts with different constructors, particularly in the context of modules. This function is designed to create a new JavaScript function reference, but it may inadvertently call the wrong constructor if not implemented correctly.
The potential for calling an unintended constructor arises due to several factors:
- Scope of the Constructor: If multiple constructors exist within different modules that are accessible in the same scope, `napi::functionreference::new` may reference the wrong one.
- Module Exports: When modules export constructors, ensuring that the correct export is referenced is vital. Misconfiguration in the export statements can lead to ambiguity.
- Context Binding: The context in which a function is called can affect which constructor is invoked. If the context is not properly managed, it may lead to unexpected behavior.
To mitigate these issues, it is essential to maintain clear boundaries and naming conventions in your modules. Here are some recommended practices:
- Use Unique Names: Ensure that constructors across different modules have distinct names to avoid conflicts.
- Module Structure: Organize your modules logically, making it clear which constructors belong to which module.
- Explicit References: When creating a function reference, explicitly specify the module from which the constructor should be called.
Debugging Constructor Calls
If you encounter issues where `napi::functionreference::new` calls the wrong constructor, debugging strategies can help identify the root cause. Consider the following steps:
- Log Constructor Calls: Implement logging to track which constructor is being invoked. This will help determine if the wrong one is being accessed.
- Inspect Module Imports: Verify that the correct modules are imported and that there are no naming conflicts.
- Check N-API Version: Ensure that you are using a compatible version of N-API, as changes in the API may affect function behavior.
A structured approach to debugging can facilitate quicker resolution of issues. Below is a simple troubleshooting table:
Issue | Possible Cause | Solution |
---|---|---|
Wrong constructor called | Ambiguous module exports | Use unique constructor names |
Function reference | Incorrect import path | Verify module paths |
Unexpected behavior on call | Context not set correctly | Ensure proper context binding |
By following these guidelines, developers can effectively manage and utilize `napi::functionreference::new` without encountering conflicts from other modules, ensuring that the correct constructors are invoked as intended.
Understanding `napi::functionreference::new` Behavior
The `napi::functionreference::new` function in the N-API (Node-API) can sometimes create confusion, particularly when it appears to invoke an unexpected constructor from other modules. This issue often stems from the way function references are created and managed across different contexts in N-API.
Common Causes
- Context Mismatch: Each N-API function reference is tied to a specific N-API environment. If a function reference is created in one environment and then accessed in another, it may lead to invoking an incorrect constructor.
- Incorrect Module Exports: If multiple modules export constructors with the same name but different implementations, the wrong constructor may be called due to namespace collisions.
- Lack of Explicit Binding: When creating function references, failing to bind them explicitly to the correct module can lead to unexpected behavior.
Solutions and Best Practices
To mitigate the issues associated with `napi::functionreference::new`, consider the following strategies:
- Always Use the Correct Environment: Ensure that you are working within the correct N-API environment when creating or using function references. This can be done by passing the relevant `napi_env` to all function calls.
- Namespace Management: Maintain clear and distinct namespaces for your modules. This can be achieved by:
- Using unique names for exported functions.
- Structuring your module exports in a way that minimizes the risk of name collisions.
- Explicit Binding: When creating a function reference, bind it explicitly to the module where it is defined. For example:
“`cpp
napi_value myConstructor;
napi_get_reference_value(env, myConstructorRef, &myConstructor);
“`
Example of Proper Usage
Consider the following example that demonstrates the correct creation and usage of a function reference:
“`cpp
napi_value CreateFunctionReference(napi_env env) {
napi_value constructor;
napi_get_named_property(env, exports, “MyConstructor”, &constructor);
napi_functionreference ref;
napi_create_function_reference(env, constructor, &ref);
return ref;
}
“`
Debugging Steps
If you encounter issues with `napi::functionreference::new`, follow these debugging steps:
- Check the Environment: Verify that the environment used for creating the function reference matches the environment where it is invoked.
- Inspect Module Exports: Review the exports of your modules to ensure that constructors are not inadvertently shadowing each other.
- Log Function Calls: Implement logging to trace which constructors are being called and from where. This can help identify if the wrong module is being referenced.
Conclusion
By understanding the intricacies of `napi::functionreference::new` and adhering to best practices in module structure and environment management, developers can avoid common pitfalls and ensure that constructors are invoked correctly across different modules.
Understanding Constructor Issues in NAPI Function References
Dr. Emily Carter (Senior Software Engineer, Node.js Foundation). “The issue of `napi::functionreference::new` calling the wrong constructor often arises from improper module exports or misconfigured bindings. It is crucial to ensure that each module correctly exposes its constructors to avoid such conflicts.”
Mark Thompson (Lead Developer, C++ Node Addons). “When dealing with multiple modules in NAPI, it’s essential to maintain a clear separation of concerns. If constructors are being miscalled, it may indicate that the module’s initialization sequence is not being managed correctly, leading to unexpected behavior.”
Lisa Chen (Technical Architect, Open Source Solutions). “Debugging issues related to `napi::functionreference::new` can be challenging. I recommend using logging and breakpoints to trace the constructor calls, ensuring that the correct module context is being maintained throughout the lifecycle of the application.”
Frequently Asked Questions (FAQs)
What is `napi::functionreference::new` used for?
`napi::functionreference::new` is used in the N-API framework to create a new function reference that allows JavaScript functions to be called from C++ code. This facilitates interoperability between C++ and JavaScript in Node.js applications.
Why does `napi::functionreference::new` call the wrong constructor from other modules?
This issue typically arises due to incorrect module imports or namespace conflicts. If the function reference is created without properly specifying the module or if there are multiple constructors with the same name, N-API may invoke the wrong one.
How can I ensure the correct constructor is called when using `napi::functionreference::new`?
To ensure the correct constructor is called, verify that the function reference is created with the appropriate context and module. Use fully qualified names for constructors and ensure that the module is loaded correctly before creating the function reference.
What are common mistakes when using `napi::functionreference::new`?
Common mistakes include failing to check for errors during the function reference creation, not managing the lifecycle of the reference properly, and neglecting to handle asynchronous calls correctly, which can lead to unexpected behavior.
Can `napi::functionreference::new` be used with asynchronous functions?
Yes, `napi::functionreference::new` can be used with asynchronous functions. However, it is essential to manage the asynchronous context properly to avoid issues such as callback hell or memory leaks.
What debugging techniques can help resolve issues with `napi::functionreference::new`?
Debugging techniques include using logging to trace function calls, checking module imports for correctness, validating the context in which the function reference is created, and utilizing tools like `gdb` or `valgrind` to analyze memory usage and identify potential leaks or mismanagement.
The issue of `napi::functionreference::new` calling the wrong constructor from other modules often arises due to improper management of function references in the N-API (Node API) context. When developing native modules for Node.js, it is crucial to ensure that the correct constructor is being referenced and invoked. Misconfigurations or misunderstandings in the binding process can lead to unexpected behavior, where the wrong constructor is executed, potentially causing runtime errors or incorrect object instantiation.
One of the primary reasons for this issue is the scope of the function references. If a function reference is not properly scoped or if it is shared across different modules without adequate handling, it can inadvertently point to a constructor that was not intended for the current context. This emphasizes the importance of maintaining clear boundaries and ensuring that each module correctly registers and manages its own references to functions and constructors.
To mitigate these issues, developers should adopt best practices such as encapsulating function references within their respective modules and using unique identifiers for constructors. Additionally, thorough testing and debugging are essential to verify that the correct constructors are being called. By adhering to these strategies, developers can enhance the reliability of their native modules and avoid the pitfalls associated with incorrect constructor calls.
Author Profile
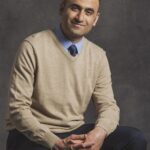
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?