Why Am I Seeing the Error: ‘No Access-Control-Allow-Origin Header Is Present on the Requested Resource’?
In the ever-evolving landscape of web development, the intricacies of cross-origin resource sharing (CORS) often leave developers scratching their heads. One common issue that arises is the dreaded message: “No access-control-allow-origin header is present on the requested resource.” This seemingly cryptic notification can halt progress and frustrate even the most seasoned programmers. Understanding the underlying principles of CORS and the implications of this error is crucial for anyone looking to create seamless web applications that interact with multiple domains.
Overview
At its core, the “no access-control-allow-origin header” error signifies a security measure designed to protect users and their data from malicious activities. When a web application attempts to request resources from a different origin, the browser enforces a same-origin policy that restricts such actions unless explicitly permitted. This policy is vital for maintaining the integrity of web applications, but it can also lead to roadblocks during development, especially when integrating third-party APIs or services.
Navigating the complexities of CORS requires a solid understanding of how headers work and the role they play in facilitating secure communication between different origins. Developers must learn how to configure their servers to include the appropriate access-control headers, ensuring that resources are shared safely and effectively. By delving into
Understanding CORS and Its Impact
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to restrict web pages from making requests to a different domain than the one that served the web page. This is where the “no access-control-allow-origin header” issue often arises. When a web page tries to fetch resources from a different origin, the browser checks the response headers to determine whether the request is allowed.
When a resource is requested from a different origin and the appropriate CORS headers are not present in the response, the browser will block the request. This is a mechanism designed to prevent malicious scripts from accessing sensitive data on other domains.
Key CORS Headers
To facilitate cross-origin requests, servers need to include specific headers in their responses. The most crucial header is the `Access-Control-Allow-Origin` header. Below are some other important CORS headers:
- Access-Control-Allow-Origin: Specifies which origins are allowed to access the resource. A wildcard `*` can be used to allow all origins, but this may not be suitable for sensitive data.
- Access-Control-Allow-Methods: Lists the HTTP methods that are permitted when accessing the resource (e.g., GET, POST).
- Access-Control-Allow-Headers: Specifies which headers can be used during the actual request.
- Access-Control-Expose-Headers: Indicates which headers are safe to expose to the client.
Header | Description |
---|---|
Access-Control-Allow-Origin | Defines which origins can access the resource. |
Access-Control-Allow-Methods | Specifies the allowed HTTP methods. |
Access-Control-Allow-Headers | Lists the allowed request headers. |
Access-Control-Expose-Headers | Indicates which headers can be exposed to the client. |
Common Causes of Missing CORS Headers
Several factors can lead to the absence of CORS headers in responses:
- Server Configuration: The server may not be configured to include CORS headers. This is a common issue with default server settings.
- Backend Framework Limitations: Some backend frameworks may require additional configuration or plugins to enable CORS support.
- API Gateway Restrictions: If an API is accessed through a gateway, it may not pass through the required headers unless explicitly configured to do so.
Troubleshooting CORS Issues
To resolve CORS-related issues effectively, consider the following steps:
- Inspect Network Requests: Use browser developer tools to check the network tab and see the request and response headers.
- Update Server Configuration: Modify the server settings to include the appropriate CORS headers.
- Test with Different Origins: If applicable, test the resource from different origins to ensure that the headers are working as intended.
- Consult Documentation: Refer to the documentation of the web server or framework in use to understand how to enable CORS.
By understanding the role of CORS and the significance of the `Access-Control-Allow-Origin` header, developers can better manage cross-origin requests and mitigate issues related to resource accessibility.
Understanding the Access-Control-Allow-Origin Header
The `Access-Control-Allow-Origin` header is a crucial component of the Cross-Origin Resource Sharing (CORS) protocol. It determines whether a resource can be accessed from a different origin than the one that served the original content. The absence of this header in a response can lead to browser security restrictions, preventing the resource from being accessed by web pages from other domains.
Why No Access-Control-Allow-Origin Header is Present?
There are several reasons why the `Access-Control-Allow-Origin` header may not be present in the response:
- Server Configuration: The server may not be configured to allow CORS. This is common in default configurations where security is prioritized.
- Resource Type: Certain resources, such as images or scripts, may not require CORS headers, depending on how the server handles these requests.
- Request Method: If the request method is not supported (e.g., using `PUT` or `DELETE` on a server that only allows `GET`), the server may not send the appropriate CORS headers.
Implications of Missing Access-Control-Allow-Origin Header
When the `Access-Control-Allow-Origin` header is missing, the following issues may arise:
- Access Denied: Browsers will block requests from different origins, resulting in errors such as “No ‘Access-Control-Allow-Origin’ header is present on the requested resource.”
- Limited Functionality: Applications relying on data from external APIs or domains will fail to retrieve necessary resources, hindering functionality.
- User Experience Impact: Users may encounter unexpected behavior or broken features, leading to frustration and decreased trust in the application.
How to Resolve Missing Access-Control-Allow-Origin Header Issues
To resolve issues related to the missing `Access-Control-Allow-Origin` header, consider the following approaches:
– **Server Configuration Changes**: Modify the server settings to include the `Access-Control-Allow-Origin` header in responses. This can typically be done in the server’s configuration files or application code.
For example, in an Express.js application, you can add:
“`javascript
app.use((req, res, next) => {
res.header(“Access-Control-Allow-Origin”, “*”);
next();
});
“`
- Specify Allowed Origins: Instead of allowing all origins (`*`), specify allowed origins for enhanced security:
“`javascript
res.header(“Access-Control-Allow-Origin”, “https://example.com”);
“`
- Use Middleware: Many web frameworks provide middleware to handle CORS, simplifying the process of adding necessary headers.
- Check Preflight Requests: For complex requests, ensure that your server correctly handles OPTIONS preflight requests, which are sent by browsers to check permissions.
Testing CORS Configuration
To verify the correct implementation of CORS headers, use the following methods:
Method | Description |
---|---|
Browser Dev Tools | Inspect network requests to check response headers. |
cURL | Send requests using cURL to see response headers: `curl -I -X OPTIONS https://example.com/resource` |
Postman | Use Postman to simulate cross-origin requests and observe headers. |
By properly configuring the `Access-Control-Allow-Origin` header and understanding its implications, developers can ensure that their applications function correctly across different domains while maintaining security protocols.
Understanding CORS and the Absence of Access-Control-Allow-Origin Header
Dr. Emily Carter (Web Security Analyst, CyberSafe Solutions). “The absence of the Access-Control-Allow-Origin header indicates that the server is not configured to allow cross-origin requests. This can lead to significant security vulnerabilities, as it may expose sensitive data to unauthorized domains.”
Mark Thompson (Lead Developer, OpenWeb Technologies). “When developers encounter the ‘no Access-Control-Allow-Origin header is present on the requested resource’ error, it often signifies a misconfiguration in the server settings. Properly setting this header is crucial for enabling secure cross-origin resource sharing.”
Lisa Chen (Frontend Engineer, Tech Innovations Inc.). “Understanding the implications of the missing Access-Control-Allow-Origin header is essential for modern web applications. It not only affects API accessibility but also impacts user experience and the overall functionality of web services.”
Frequently Asked Questions (FAQs)
What does it mean when there is no access-control-allow-origin header present on the requested resource?
The absence of the `Access-Control-Allow-Origin` header indicates that the server does not permit cross-origin requests from the requesting domain. This can lead to the browser blocking the response to protect user data and privacy.
Why is the access-control-allow-origin header important?
This header is crucial for implementing Cross-Origin Resource Sharing (CORS), which allows servers to specify which origins can access their resources. It helps prevent unauthorized access and enhances security in web applications.
How can I resolve the issue of missing access-control-allow-origin header?
To resolve this issue, you need to configure the server to include the `Access-Control-Allow-Origin` header in its response. This can typically be done by modifying server settings or adding middleware in your application.
What are the potential security implications of allowing all origins with access-control-allow-origin?
Allowing all origins (using `*`) can expose your application to security risks, such as data theft or unauthorized actions. It is advisable to specify trusted origins to mitigate these risks.
Can I bypass the access-control-allow-origin restriction in my web application?
Bypassing this restriction is generally not recommended, as it compromises security. However, during development, you can use browser extensions or proxy servers to test APIs without CORS restrictions, but these should not be used in production environments.
What should I do if I control the server and still encounter this issue?
If you control the server, ensure that the server-side code correctly sets the `Access-Control-Allow-Origin` header for the desired routes. Additionally, verify that there are no server misconfigurations or middleware issues affecting the response headers.
The absence of the “Access-Control-Allow-Origin” header on a requested resource signifies a potential issue with Cross-Origin Resource Sharing (CORS). CORS is a security feature implemented by web browsers to prevent malicious websites from accessing sensitive data from another domain without permission. When a resource does not include this header, it indicates that the server has not explicitly allowed cross-origin requests from the requesting domain, leading to blocked access and potential functionality issues for web applications.
This situation often arises in scenarios where developers are working with APIs or integrating third-party services. Without the proper CORS configuration, clients attempting to fetch resources from different origins will encounter errors, hindering the user experience. It is crucial for developers to understand the implications of CORS and to configure their servers correctly to include the “Access-Control-Allow-Origin” header where necessary, thereby allowing legitimate cross-origin requests while maintaining security protocols.
addressing the absence of the “Access-Control-Allow-Origin” header is vital for ensuring seamless interactions between web applications and external resources. Developers should prioritize implementing appropriate CORS policies to facilitate cross-origin requests while safeguarding against unauthorized access. By doing so, they can enhance the functionality and reliability of their web applications, ultimately leading to improved user satisfaction and engagement.
Author Profile
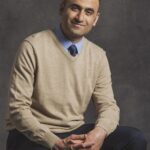
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?