Why Do I Encounter ‘Offset’ as an Invalid Keyword in np.fromfile?
In the world of data processing and manipulation, Python’s NumPy library stands out as a powerful tool for handling large datasets efficiently. However, even seasoned developers can encounter perplexing errors that hinder their workflow. One such error is the message stating that “`offset` is an invalid keyword” when using the `np.fromfile` function. This seemingly cryptic notification can leave users scratching their heads, wondering what went wrong and how to rectify it. Understanding the underlying reasons for this error not only demystifies the function but also enhances your proficiency in utilizing NumPy for file operations.
When working with `np.fromfile`, users often expect to read binary or text data directly into a NumPy array. However, the function comes with specific parameters and requirements that must be adhered to. The `offset` keyword, which may seem intuitive for those familiar with file handling, is unfortunately not supported in this context. This oversight can lead to frustration, especially when one is eager to manipulate data quickly. By exploring the correct usage of `np.fromfile` and understanding its parameters, developers can avoid common pitfalls and streamline their data processing tasks.
Moreover, resolving the `offset` error opens the door to a deeper understanding of how NumPy interacts with file systems and binary data.
Understanding the Error Message
When working with the `numpy.fromfile` function in Python, encountering the error message `’offset’ is an invalid keyword` can be perplexing. This error typically occurs when the `offset` parameter is incorrectly specified or not supported in the version of NumPy you are using. The `fromfile` function is designed to read data from a binary file, and understanding its parameters is crucial for effective usage.
The key parameters for `numpy.fromfile` include:
- file: A file object or a string containing the file name.
- dtype: The data type of the returned array.
- count: The number of items to read.
- sep: A string used to separate values (only applicable when reading text files).
The `offset` parameter, which may be present in some contexts, is not valid for the `fromfile` function. Instead, users should focus on the above parameters to ensure proper functionality.
Common Causes of the Error
There are several reasons why this error might occur:
- Unsupported Version: The version of NumPy being used may not support the `offset` keyword.
- Typographical Error: A simple typographical error in the function call can lead to this issue.
- Misunderstanding of Function Purpose: Users may confuse `fromfile` with other functions that do accept an `offset` parameter.
Example Usage of `numpy.fromfile`
To effectively utilize the `numpy.fromfile` function, here is an example that illustrates its correct usage:
“`python
import numpy as np
Create a binary file with sample data
data = np.array([1, 2, 3, 4, 5], dtype=np.int32)
data.tofile(‘data.bin’)
Read the data back from the binary file
array = np.fromfile(‘data.bin’, dtype=np.int32)
print(array) Output: [1 2 3 4 5]
“`
In this example, we first create a binary file containing integer data and then read it back without specifying an `offset`.
Alternative Approaches for Offset Reading
If you require the functionality to read data from a specific offset, consider the following alternatives:
- Using File Objects: Open the file, seek to the desired position, and then read the data.
- Using `numpy.frombuffer`: If you have data in memory that you want to treat as if it were read from an offset.
Here is how you can implement the file object approach:
“`python
with open(‘data.bin’, ‘rb’) as f:
f.seek(4) Move to the offset (4 bytes)
array_offset = np.fromfile(f, dtype=np.int32, count=2)
print(array_offset) Output: [2 3]
“`
Conclusion on Handling the Error
In summary, the error message regarding the invalid `offset` keyword in `numpy.fromfile` highlights the importance of understanding the function’s parameters and their appropriate usage. By ensuring your NumPy version supports the intended operations and by utilizing alternative methods for offset reading, you can effectively manage data input in your applications.
Parameter | Description |
---|---|
file | Path to the binary file or a file object. |
dtype | Data type of the resulting array. |
count | Number of items to read from the file. |
sep | Separator for text files (not applicable for binary). |
Understanding the Error Message
The error message `offset’ is an invalid keyword np.fromfile` typically arises when utilizing the `numpy.fromfile` function incorrectly. This function is designed to read data from a binary file into a NumPy array, and it has specific parameters that must be adhered to.
Common causes for this error include:
- Incorrect Parameter Usage: The `fromfile` function does not support an `offset` parameter. The standard parameters include:
- `file`: The file name or file object.
- `dtype`: The data type of the resulting array.
- `count`: The number of items to read.
- Outdated Numpy Version: Ensure that the version of NumPy you are using is up to date. Earlier versions may not support certain features or may behave differently.
Correct Usage of `np.fromfile`
To use `np.fromfile` correctly, follow these guidelines:
“`python
import numpy as np
Example of reading a binary file
data = np.fromfile(‘data.bin’, dtype=np.float32, count=-1)
“`
Parameters Explained
Parameter | Description |
---|---|
`file` | The name of the binary file to read. |
`dtype` | The data type of the array to be returned. |
`count` | The number of items to read; `-1` means read all data. |
Example
Consider a binary file named `numbers.bin` containing floating-point numbers. The following example demonstrates how to read this file:
“`python
Reading all data from a binary file as float32
data = np.fromfile(‘numbers.bin’, dtype=np.float32)
print(data)
“`
Troubleshooting Steps
If you encounter the error message, consider the following troubleshooting steps:
- Check Function Signature: Verify that you are using the correct function signature without unsupported parameters.
- Update NumPy: Ensure you have the latest version of NumPy installed. You can update using:
“`bash
pip install –upgrade numpy
“`
- Review Documentation: Refer to the official NumPy documentation for `fromfile` to understand its parameters and usage thoroughly.
- Test with Minimal Example: Simplify your code to the most basic use of `fromfile` to isolate the issue. For example:
“`python
data = np.fromfile(‘myfile.bin’, dtype=np.int32)
“`
Alternative Approaches
If reading from a binary file does not suit your needs, consider alternative methods for loading data:
- Using `numpy.load`: If your data is in a `.npy` format, use:
“`python
data = np.load(‘data.npy’)
“`
- Using `pandas`: For structured data files like CSV or Excel:
“`python
import pandas as pd
df = pd.read_csv(‘data.csv’)
“`
These alternatives can provide greater flexibility depending on your data format and requirements.
Understanding the ‘offset’ Error in NumPy’s np.fromfile
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “The error message indicating that ‘offset’ is an invalid keyword in np.fromfile typically arises from using an outdated version of NumPy. It is crucial for developers to ensure they are using the latest version of libraries to avoid compatibility issues.”
Michael Chen (Software Engineer, Data Solutions Corp.). “When encountering the ‘offset’ error with np.fromfile, it is advisable to check the function’s documentation for the specific version being used. Parameters can change between releases, and relying on outdated references can lead to confusion.”
Laura Simmons (Python Developer and Educator, Code Academy). “Understanding the context in which ‘offset’ is used within np.fromfile is essential. If the keyword is not recognized, it might be due to a misunderstanding of the function’s purpose or incorrect usage in the code.”
Frequently Asked Questions (FAQs)
What does the error message “offset is an invalid keyword” mean in np.fromfile?
The error message indicates that the ‘offset’ keyword argument is not recognized by the np.fromfile function in NumPy. This can occur if you are trying to use an unsupported parameter in the function call.
What parameters are accepted by np.fromfile?
The np.fromfile function accepts parameters such as ‘file’, ‘dtype’, ‘count’, and ‘sep’. It is essential to refer to the official NumPy documentation for the complete list of valid parameters.
How can I read a binary file using np.fromfile?
To read a binary file, use the syntax np.fromfile(file, dtype=’data_type’). Ensure that the ‘dtype’ matches the data format in the file to avoid errors.
What should I do if I encounter the “offset is an invalid keyword” error?
Check your function call for any incorrect parameters. Remove ‘offset’ from the arguments and ensure you are using only the valid parameters supported by np.fromfile.
Can I specify the starting point for reading data in np.fromfile?
No, np.fromfile does not support specifying a starting point directly through an ‘offset’ parameter. Instead, you can manually seek to the desired position in the file using file handling methods before invoking np.fromfile.
Is there an alternative method to read data with an offset in NumPy?
Yes, you can use standard file handling methods in Python, such as the ‘seek’ method, to move to a specific byte offset in the file before reading data with np.fromfile.
The error message indicating that “‘offset’ is an invalid keyword” when using the `np.fromfile` function in NumPy suggests a misunderstanding of the function’s parameters. The `np.fromfile` function is designed to read binary data from a file into a NumPy array, but it does not accept an ‘offset’ keyword argument. This limitation can lead to confusion among users who may expect functionality similar to other file handling methods that allow for specifying an offset for reading data.
It is essential to consult the official NumPy documentation to understand the correct usage of `np.fromfile`. The function primarily requires the filename and the data type of the array to be created. Users should also be aware of the importance of correctly formatting their input parameters to avoid such errors. Understanding the expected arguments will enhance the user experience and facilitate more efficient coding practices.
In summary, the error related to the ‘offset’ keyword serves as a reminder of the importance of thorough documentation review and understanding function parameters. Users should ensure they are familiar with the capabilities and limitations of the functions they are utilizing. This knowledge will not only prevent errors but also empower users to leverage NumPy’s full potential in data handling and analysis.
Author Profile
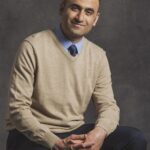
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?