How Can I Use a One-Line If Statement in Python?
In the world of Python programming, efficiency and readability often reign supreme, and one of the most elegant features that embodies these principles is the one-line if statement. This compact syntax not only streamlines your code but also enhances its clarity, allowing developers to express complex conditions succinctly. Whether you’re a seasoned coder or a newcomer to Python, mastering this feature can significantly improve your coding style and productivity.
The one-line if statement, also known as a conditional expression or ternary operator, enables you to evaluate conditions and assign values in a single line, reducing the need for verbose multi-line structures. This capability is particularly useful in scenarios where you want to make quick decisions without cluttering your code with excessive indentation or multiple lines. By leveraging this concise syntax, you can write cleaner, more maintainable code that still conveys your intent clearly.
As we delve deeper into the mechanics of one-line if statements, we’ll explore their syntax, practical applications, and best practices. Whether you’re looking to simplify your code or enhance its functionality, understanding how to effectively implement one-line if statements will undoubtedly elevate your Python programming skills. Get ready to unlock a new level of coding efficiency and clarity!
Understanding One-Line If Statements
One-line if statements, also known as conditional expressions or ternary operators, provide a concise way to perform conditional logic in Python. This syntax allows you to evaluate a condition and return a value based on whether that condition is true or , all within a single line of code.
The basic syntax for a one-line if statement in Python is as follows:
“`python
value_if_true if condition else value_if_
“`
This structure allows for a clear and efficient way to handle simple conditional assignments. The condition is evaluated first, and if it returns true, the expression evaluates to `value_if_true`; otherwise, it evaluates to `value_if_`.
Examples of One-Line If Statements
Here are a few practical examples to illustrate the usage of one-line if statements:
– **Basic Example**
Assigning a value based on a condition:
“`python
x = 10
result = “High” if x > 5 else “Low”
“`
– **Using in Functions**
You can also incorporate one-line if statements within functions:
“`python
def check_age(age):
return “Adult” if age >= 18 else “Minor”
“`
- List Comprehensions
One-line if statements can be particularly useful in list comprehensions:
“`python
numbers = [1, 2, 3, 4, 5]
labels = [“Even” if n % 2 == 0 else “Odd” for n in numbers]
“`
Advantages of One-Line If Statements
One-line if statements offer several advantages:
- Conciseness: Reduces the number of lines of code, making scripts shorter and easier to read.
- Clarity: In certain contexts, they can enhance readability by clearly showing the conditional logic in a compact form.
- Efficiency: Simplifies code, particularly in functional programming or when processing lists.
When to Use One-Line If Statements
While one-line if statements can enhance code clarity, they are best used in specific scenarios:
- When the logic is simple and easy to understand at a glance.
- In cases where minimizing lines of code is beneficial without sacrificing readability.
- When integrating into expressions where a full if-else structure may seem excessive.
Conversely, one-line if statements should be avoided in complex conditions or when multiple statements are required for either outcome. In such cases, traditional multi-line if statements enhance readability and maintainability.
Condition | True Case | Case |
---|---|---|
x > 10 | “Greater than 10” | “10 or less” |
y == 0 | “Zero” | “Not Zero” |
In summary, while one-line if statements are a powerful feature in Python, their usage should be balanced with the need for code clarity and maintainability. By understanding when and how to effectively use these conditional expressions, developers can write more efficient and readable Python code.
Using One-Line If Statements in Python
In Python, a one-line if statement is often referred to as a ternary conditional operator. This construct allows you to assign a value based on a condition in a concise manner. The general syntax is as follows:
“`python
value_if_true if condition else value_if_
“`
Example of a One-Line If Statement
Here’s a simple example demonstrating the use of a one-line if statement:
“`python
result = “Even” if number % 2 == 0 else “Odd”
“`
In this case, `result` will be assigned “Even” if `number` is divisible by 2; otherwise, it will be assigned “Odd”.
Use Cases
One-line if statements are particularly useful in various scenarios, including:
– **Variable assignment**: Assigning values based on conditions.
– **Function arguments**: Setting default values based on conditions in function calls.
– **List comprehensions**: Filtering or transforming lists succinctly.
List Comprehensions with One-Line If Statements
Using one-line if statements in list comprehensions allows for efficient data manipulation. For example, to create a list of even numbers from another list:
“`python
even_numbers = [num for num in numbers if num % 2 == 0]
“`
Alternatively, to assign a default value when filtering:
“`python
status = [“Even” if num % 2 == 0 else “Odd” for num in numbers]
“`
Conditional Expressions in Functions
You can also use one-line if statements within functions to streamline your code. For instance:
“`python
def check_age(age):
return “Adult” if age >= 18 else “Minor”
“`
This function succinctly returns “Adult” or “Minor” based on the input age.
Limitations and Best Practices
While one-line if statements enhance readability for simple conditions, they can lead to reduced clarity in more complex scenarios. Consider the following points:
- Readability: Use one-line if statements for simple conditions; for more complex logic, prefer full if statements.
- Nested Conditions: Avoid deep nesting; it reduces readability.
- Debugging: One-line statements can make debugging harder. Use traditional if statements when necessary.
Summary of Syntax and Use
Context | Syntax Example |
---|---|
Variable Assignment | `result = “Yes” if condition else “No”` |
List Comprehensions | `[x if condition else y for x in iterable]` |
Function Return Values | `return “Success” if condition else “Failure”` |
The one-line if statement is a powerful feature in Python, enabling concise and expressive code. However, it is essential to use it judiciously to maintain code readability and clarity.
Understanding One-Line If Statements in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “One-line if statements, also known as conditional expressions, enhance code readability and conciseness. They allow developers to execute conditional logic in a single line, making the code cleaner and easier to maintain.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Utilizing one-line if statements can significantly reduce the number of lines in your code, but it’s crucial to ensure that the logic remains clear. Overusing this feature can lead to confusion, especially for those new to Python.”
Sarah Thompson (Python Educator, LearnPythonNow). “Teaching one-line if statements is essential for beginners. It introduces them to the power of Python’s syntax while emphasizing the importance of writing readable code. Proper use can lead to elegant solutions in programming.”
Frequently Asked Questions (FAQs)
What is a one line if statement in Python?
A one line if statement in Python, also known as a conditional expression or ternary operator, allows you to evaluate a condition and return a value based on that condition in a single line of code.
How is a one line if statement structured in Python?
The structure of a one line if statement in Python is: `value_if_true if condition else value_if_`. This format evaluates the condition and returns the corresponding value based on whether the condition is true or .
Can you provide an example of a one line if statement?
Certainly. An example would be: `result = “Pass” if score >= 60 else “Fail”`, which assigns “Pass” to `result` if `score` is 60 or higher, otherwise it assigns “Fail”.
Are one line if statements suitable for complex conditions?
One line if statements are best suited for simple conditions. For complex logic, using traditional multi-line if statements enhances readability and maintainability of the code.
What are the advantages of using one line if statements?
The advantages include conciseness and improved readability for straightforward conditions, allowing for quicker coding and less clutter in the codebase.
Are there any limitations to using one line if statements?
Yes, limitations include reduced clarity for complex conditions and potential difficulty in debugging. They should be used judiciously to maintain code readability.
In Python, a one-line if statement, often referred to as a conditional expression or ternary operator, allows developers to write concise conditional logic. The syntax follows the format: `value_if_true if condition else value_if_`. This structure enables the execution of a simple conditional operation in a single line, enhancing code readability and reducing verbosity.
Using one-line if statements can significantly streamline code, especially in scenarios where simple conditions need to be evaluated. They are particularly useful in list comprehensions, lambda functions, and when initializing variables based on conditions. However, it is essential to use them judiciously, as overly complex one-liners can lead to reduced code clarity and maintainability.
In summary, one-line if statements in Python serve as a powerful tool for writing succinct and efficient code. They provide a clear and elegant way to handle conditional logic, making them a valuable addition to any Python programmer’s toolkit. Nevertheless, maintaining a balance between brevity and clarity is crucial to ensure that the code remains understandable for future modifications and collaborations.
Author Profile
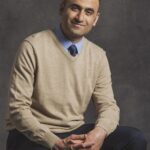
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?