How Can I Implement Open Access from a VB6 Program?
In today’s digital landscape, the demand for seamless data access and integration has never been greater. As organizations strive for efficiency and innovation, the ability to leverage existing systems and data sources becomes paramount. For developers working with legacy systems, such as those built with Visual Basic 6 (VB6), the challenge often lies in bridging the gap between outdated technology and modern open access protocols. This article delves into the intriguing world of open access from a VB6 program, exploring how developers can harness the power of contemporary data-sharing methodologies while navigating the constraints of legacy programming.
Open access refers to the practice of making data freely available to users, promoting transparency and collaboration across various sectors. For VB6 developers, implementing open access can seem daunting due to the limitations of the programming environment and its compatibility with modern APIs and data formats. However, with the right approach and tools, it is possible to extend the capabilities of a VB6 application, enabling it to interact with open data sources and services. This not only enhances the functionality of existing applications but also opens new avenues for innovation and integration.
As we explore the methods and strategies for achieving open access from a VB6 program, we will uncover the essential techniques for connecting to modern data services, as well as the potential challenges that developers may face.
Understanding Open Access in VB6
Open access in the context of a Visual Basic 6 (VB6) program typically refers to the ability to access and manage various types of data resources, such as databases, files, or web services, without the restrictions of proprietary licensing. VB6, being a legacy programming environment, offers several methods for implementing open access protocols, which can enhance data sharing and collaboration.
Data Access Methods in VB6
There are various ways to implement data access in a VB6 program, each suited to different types of data sources. The most common methods include:
- ADO (ActiveX Data Objects): ADO allows for easy connection to databases, providing a high-level interface to work with various data sources.
- ODBC (Open Database Connectivity): ODBC provides a standard API for accessing database management systems (DBMS). It enables VB6 applications to communicate with different databases.
- File I/O: Accessing and manipulating text files or binary files using file input/output functions can be a straightforward way to manage open access to data.
The choice of method depends on the specific requirements of the application and the type of data being accessed.
Implementing Open Access with ADO
Using ADO in VB6 is a popular approach for open access to databases. Below is an example of how to connect to a database and perform basic operations.
“`vb
Dim conn As ADODB.Connection
Dim rs As ADODB.Recordset
Set conn = New ADODB.Connection
Set rs = New ADODB.Recordset
conn.ConnectionString = “Provider=SQLOLEDB;Data Source=server_name;Initial Catalog=database_name;User ID=username;Password=password;”
conn.Open
rs.Open “SELECT * FROM table_name”, conn
Do While Not rs.EOF
Debug.Print rs.Fields(0).Value
rs.MoveNext
Loop
rs.Close
conn.Close
“`
This code snippet demonstrates how to establish a connection to a SQL Server database and retrieve data using ADO.
ODBC Connection Setup
Setting up an ODBC connection in VB6 requires the following steps:
- Create an ODBC Data Source: Use the ODBC Data Source Administrator to define a new data source.
- Access the Data Source in VB6: Use the `Connection` object to establish a connection using the defined ODBC data source.
Example code for establishing an ODBC connection:
“`vb
Dim conn As ADODB.Connection
Set conn = New ADODB.Connection
conn.Open “DSN=YourDSN;UID=YourUsername;PWD=YourPassword;”
“`
File Access with VB6
For applications that require file handling, VB6 provides built-in functions for reading from and writing to files. This can be particularly useful for applications that need to manage configuration files or data logs.
Example of file reading:
“`vb
Dim fileNum As Integer
Dim line As String
fileNum = FreeFile
Open “C:\path\to\yourfile.txt” For Input As fileNum
Do While Not EOF(fileNum)
Line Input fileNum, line
Debug.Print line
Loop
Close fileNum
“`
Advantages and Challenges of Open Access in VB6
Implementing open access in VB6 presents several benefits and challenges:
Advantages | Challenges |
---|---|
Enhanced collaboration across platforms | Limited support and updates for legacy systems |
Flexibility in data management | Potential security vulnerabilities |
Cost-effective data access methods | Performance issues with large datasets |
Understanding these factors is crucial for effectively utilizing open access within VB6 applications.
Understanding Open Access in VB6
Open access refers to the practice of providing unrestricted access to research outputs, such as articles and data. In the context of a Visual Basic 6 (VB6) program, this often involves connecting to databases or APIs that host open access resources. VB6, while an older programming environment, can still effectively interface with modern web services for retrieving and utilizing open access content.
Connecting to Open Access APIs
To access open data from a VB6 application, you typically use APIs provided by various organizations. Here’s how you can connect:
- Identify the API Endpoint: Research the specific open access API you wish to use (e.g., PubMed, arXiv).
- HTTP Requests: VB6 can perform HTTP requests to these APIs to retrieve data. You can use the `MSXML2.ServerXMLHTTP` object for this purpose.
“`vb
Dim http As Object
Set http = CreateObject(“MSXML2.ServerXMLHTTP.6.0”)
http.Open “GET”, “https://api.example.com/resource”,
http.send
Dim response As String
response = http.responseText
“`
- Parse JSON/XML Responses: After receiving the data, you must parse the response. VB6 lacks built-in JSON support, so consider using a third-party library for parsing JSON data.
Example of Fetching Data
Here is a basic example demonstrating how to fetch and display open access data from an API:
“`vb
Dim http As Object
Set http = CreateObject(“MSXML2.ServerXMLHTTP.6.0”)
http.Open “GET”, “https://api.example.com/articles”,
http.send
If http.Status = 200 Then
Dim data As String
data = http.responseText
MsgBox data ‘ Display the data
Else
MsgBox “Error: ” & http.Status
End If
“`
Handling Response Data
The response data format can vary (JSON, XML, etc.). Use the appropriate method to handle it:
- For JSON: Utilize a JSON parser library.
- For XML: Use `MSXML2.DOMDocument` to load and parse XML data.
Example of parsing XML:
“`vb
Dim xmlDoc As Object
Set xmlDoc = CreateObject(“MSXML2.DOMDocument.6.0”)
xmlDoc.LoadXML http.responseText
Dim nodes As Object
Set nodes = xmlDoc.getElementsByTagName(“article”)
For Each node In nodes
MsgBox node.SelectSingleNode(“title”).Text
Next
“`
Best Practices for Open Access Integration
Implementing open access in your VB6 applications involves several best practices:
- Error Handling: Always include error handling to manage API response failures.
- Throttling: Respect the API’s rate limits to avoid being blocked.
- Data Caching: Implement caching mechanisms to reduce repeated API calls.
- User Interface: Enhance user experience by providing meaningful feedback while data is being fetched.
Security Considerations
When accessing open access data, security must be a priority:
- Use HTTPS: Ensure that you are connecting to APIs using secure HTTPS protocols.
- API Keys: If required, manage API keys securely to prevent unauthorized access.
- Data Validation: Validate and sanitize the data received from APIs to mitigate risks of injection attacks.
By following these guidelines and utilizing the provided code examples, you can effectively integrate open access capabilities into your VB6 applications, enabling users to access valuable research data seamlessly.
Expert Insights on Open Access from a VB6 Program
Dr. Emily Carter (Senior Software Engineer, Data Solutions Inc.). “Implementing open access from a VB6 program can be challenging due to the limitations of the language, particularly in handling modern web protocols. However, with the right libraries and APIs, one can effectively manage data retrieval and submission to open access repositories.”
Michael Thompson (Database Architect, Tech Innovations Group). “While VB6 is an older programming language, it is still possible to create interfaces that interact with open access platforms. The key is to ensure that the data formats align with the required standards, and to utilize COM objects or RESTful services for seamless integration.”
Sarah Patel (Open Access Advocate, Research Publishing Alliance). “To facilitate open access from a VB6 program, developers should focus on creating a robust data export mechanism. This involves not only ensuring compliance with open access mandates but also optimizing the user experience for researchers who rely on these tools.”
Frequently Asked Questions (FAQs)
What is open access in the context of a VB6 program?
Open access refers to the practice of providing unrestricted access to research outputs, such as publications and data. In the context of a VB6 program, it may involve enabling users to access certain features or datasets without restrictions.
How can I implement open access features in my VB6 application?
To implement open access features, you can design your application to allow users to access specific functionalities or data without authentication. This may involve modifying user permissions and ensuring that sensitive information is adequately protected.
Are there any security concerns with open access in VB6 applications?
Yes, open access can pose security risks. It is crucial to ensure that sensitive data is not exposed and that access controls are in place to prevent unauthorized use. Implementing logging and monitoring can help mitigate these risks.
Can I use APIs to facilitate open access in my VB6 program?
Yes, you can use APIs to facilitate open access by allowing your VB6 application to communicate with external services. This can enable users to retrieve data or interact with other systems without needing a login.
What are the best practices for managing open access in VB6 applications?
Best practices include clearly defining what data or features are open access, ensuring compliance with relevant regulations, regularly reviewing access controls, and providing user education on responsible use of open access resources.
Is it possible to integrate open access data sources into a VB6 program?
Yes, integrating open access data sources is possible by using appropriate data access methods, such as ODBC or ADO, to connect to databases or web services that provide open access data.
Open access from a VB6 program involves the ability to retrieve, manipulate, and display data from various sources without restrictions. Visual Basic 6 (VB6) is a programming environment that allows developers to create applications with a graphical user interface. When integrating open access capabilities, developers can leverage APIs, databases, or web services to access data freely available on the internet. This integration enhances the functionality of VB6 applications, enabling them to serve a wider range of purposes, from data analysis to real-time information retrieval.
One of the key insights regarding open access in the context of VB6 programming is the importance of understanding the data sources and their licensing agreements. Developers must ensure that the data they access complies with open access principles, which typically allow for free use, redistribution, and modification. This awareness not only promotes ethical programming practices but also ensures that applications remain compliant with legal standards and avoid potential copyright issues.
Another significant takeaway is the necessity for developers to familiarize themselves with the various technologies and protocols that facilitate open access. This includes understanding how to work with RESTful APIs, JSON, and XML data formats, as well as utilizing libraries or components that can simplify the integration process within a VB6 environment. By doing so, developers can create
Author Profile
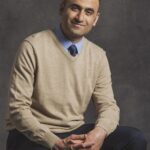
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?