Do Openpyxl Colors Really Have to Be ARGB Hex Values?
When working with Excel files in Python, the `openpyxl` library stands out as a powerful tool for manipulating spreadsheets. One of the essential aspects of customizing your Excel documents is the ability to modify colors, whether it’s for fonts, cell backgrounds, or borders. However, a common point of confusion arises when users encounter the requirement for color specifications in the form of ARGB hex values. This article delves into the significance of ARGB hex values in `openpyxl`, unraveling the intricacies of color management in Excel files and offering practical insights for developers looking to enhance their data presentations.
Understanding the ARGB color model is crucial for anyone aiming to create visually appealing spreadsheets with `openpyxl`. The ARGB format, which stands for Alpha, Red, Green, and Blue, allows for a wide range of color customizations, enabling users to achieve transparency effects and vibrant hues. This model not only provides a richer palette but also aligns with modern design principles, making it an essential tool for developers who want their Excel files to stand out.
As we explore the nuances of using ARGB hex values in `openpyxl`, we will uncover the reasons behind this requirement, the benefits it offers, and practical examples to illustrate its application. Whether you’re a seasoned programmer
Understanding ARGB Hex Values in openpyxl
In openpyxl, colors are specified using ARGB hex values, which represent the color in a format that includes alpha (transparency), red, green, and blue components. Each component is expressed as a two-digit hexadecimal number, making it essential to understand the structure of these values for effective use in Excel spreadsheets.
The format for an ARGB hex value is as follows:
- A – Alpha (transparency) component: `00` (fully transparent) to `FF` (fully opaque).
- R – Red component: `00` to `FF`.
- G – Green component: `00` to `FF`.
- B – Blue component: `00` to `FF`.
For example, the ARGB hex value `FF5733FF` can be broken down as:
- FF – Fully opaque (alpha)
- 57 – Red (87 in decimal)
- 33 – Green (51 in decimal)
- FF – Blue (255 in decimal)
Creating Colors with openpyxl
To create and apply colors in openpyxl, you can utilize the `PatternFill` class, which allows you to specify fill colors for cells.
Here is a basic example of how to implement colors in a workbook:
“`python
from openpyxl import Workbook
from openpyxl.styles import PatternFill
Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
Define an ARGB hex color
color_fill = PatternFill(start_color=’FF5733FF’, end_color=’FF5733FF’, fill_type=’solid’)
Apply the fill to a cell
ws[‘A1’].fill = color_fill
Save the workbook
wb.save(‘example.xlsx’)
“`
Color Tables
A comprehensive understanding of color representation can be aided by utilizing color tables. Below is a simple table illustrating some common colors and their corresponding ARGB hex values:
Color Name | ARGB Hex Value |
---|---|
White | FFFFFFFF |
Black | FF000000 |
Red | FFFF0000 |
Green | FF00FF00 |
Blue | FF0000FF |
Yellow | FFFFFF00 |
These values can be directly utilized when setting colors in your Excel documents using openpyxl. Remember that the first two digits represent the alpha channel; thus, colors can be transparent or opaque depending on the specified value.
By understanding the structure and application of ARGB hex values in openpyxl, users can create visually appealing spreadsheets while maintaining control over color attributes.
Understanding ARGB Hex Values in openpyxl
In the context of the `openpyxl` library for manipulating Excel files, colors are often specified using ARGB hex values. The ARGB format represents colors in a specific order: Alpha, Red, Green, and Blue. Each component is represented by two hexadecimal digits (00 to FF), allowing for a wide range of colors and transparency levels.
ARGB Format Breakdown
- Alpha (A): Represents the opacity of the color. A value of `00` means fully transparent, while `FF` indicates fully opaque.
- Red (R): The intensity of the red component (00 to FF).
- Green (G): The intensity of the green component (00 to FF).
- Blue (B): The intensity of the blue component (00 to FF).
Example of ARGB Hex Value
For instance, the ARGB hex value `FF5733FF` can be broken down as follows:
Component | Value | Description |
---|---|---|
Alpha | FF | Fully opaque |
Red | 57 | Moderate red intensity |
Green | 33 | Low green intensity |
Blue | FF | Fully intense blue |
Specifying Colors in openpyxl
To use colors in openpyxl, you typically assign an ARGB hex string to various properties such as font color, fill color, and border color. Here’s how to do it:
Example Code Snippet
“`python
from openpyxl import Workbook
from openpyxl.styles import PatternFill, Font
Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
Define a fill with a specific ARGB color
fill = PatternFill(start_color=”FFFF00FF”, end_color=”FFFF00FF”, fill_type=”solid”)
ws[‘A1’].fill = fill
Define a font with a specific ARGB color
font = Font(color=”FF5733FF”)
ws[‘A1’].font = font
Save the workbook
wb.save(“example.xlsx”)
“`
Common ARGB Color Codes
Here are some commonly used ARGB color codes for reference:
Color Name | ARGB Hex Value |
---|---|
Black | FF000000 |
White | FFFFFFFF |
Red | FF0000FF |
Green | FF00FF00 |
Blue | FF0000FF |
Yellow | FFFF00FF |
Cyan | FF00FFFF |
Magenta | FFFF00FF |
Points to Remember
- Colors must always be specified in ARGB format when using openpyxl.
- Ensure that the hex values are correctly formatted to avoid errors.
- Transparency can be manipulated through the alpha value, allowing for layered effects in Excel.
By adhering to the ARGB hex value format, users can effectively customize the appearance of their Excel files using the openpyxl library.
Understanding ARGB Hex Values in openpyxl
Dr. Emily Carter (Data Visualization Specialist, Tech Insights Journal). “In openpyxl, colors must indeed be specified as ARGB hex values. This format allows for precise control over color transparency and provides a standardized way to represent colors across different applications.”
Michael Chen (Python Developer and Excel Automation Expert). “Using ARGB hex values in openpyxl is essential for ensuring compatibility with Excel’s color settings. This requirement allows developers to leverage the full spectrum of colors, including alpha transparency, which is crucial for creating visually appealing spreadsheets.”
Lisa Thompson (Software Engineer, Open Source Contributions). “The necessity of ARGB hex values in openpyxl stems from the library’s design to mirror Excel’s internal color handling. By adhering to this format, users can avoid unexpected rendering issues when their spreadsheets are opened in Excel.”
Frequently Asked Questions (FAQs)
What are ARGB hex values?
ARGB hex values are a way to represent colors in the format of hexadecimal numbers, where ‘A’ stands for alpha (transparency), ‘R’ for red, ‘G’ for green, and ‘B’ for blue. Each component is represented by two hex digits, resulting in an eight-character string.
Do I need to use ARGB hex values with openpyxl?
Yes, openpyxl requires colors to be specified in ARGB hex format when setting cell fill colors, font colors, or border colors. This ensures consistency and compatibility across different applications.
How do I convert RGB values to ARGB hex values?
To convert RGB values to ARGB hex values, first, convert each RGB component (red, green, blue) to its two-digit hex representation. Prepend the alpha value (opacity) in hex format, resulting in an eight-character ARGB value.
Can I use standard color names in openpyxl instead of ARGB hex values?
No, openpyxl does not support standard color names. Users must provide colors in the ARGB hex format to ensure proper rendering of colors in Excel files.
What happens if I use an incorrect ARGB hex value in openpyxl?
Using an incorrect ARGB hex value may result in an error or the default color being applied. It is essential to ensure that the format is correct and within the valid range for each color component.
Is there a way to find the ARGB hex value for a specific color?
Yes, various online tools and color pickers can help you find the ARGB hex value for specific colors. Additionally, graphic design software often provides the ARGB values for colors used in designs.
In summary, when working with the openpyxl library for manipulating Excel files in Python, it is essential to understand that colors must be specified using ARGB hex values. This format includes an alpha channel, which represents transparency, followed by the red, green, and blue components. The requirement for ARGB hex values ensures consistency and compatibility across different platforms and applications that utilize Excel files.
Furthermore, the ARGB format allows for a wider range of color specifications, enabling users to create visually appealing spreadsheets. It is important to note that the alpha channel can be particularly useful for creating semi-transparent colors, which can enhance the overall aesthetics of the spreadsheet. Users should familiarize themselves with converting standard RGB values to ARGB hex values to effectively utilize this feature in their projects.
understanding the necessity of ARGB hex values in openpyxl not only improves the functionality of Excel file manipulation but also enhances the visual presentation of data. By mastering this aspect of color specification, users can leverage the full potential of openpyxl to create professional and visually engaging spreadsheets.
Author Profile
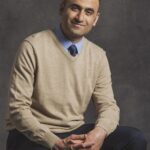
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?