Why Am I Facing an Operand Type Clash: Is My Date Incompatible with Int?
In the world of programming and database management, encountering errors is an inevitable part of the journey. One such error that often leaves developers scratching their heads is the notorious “operand type clash: date is incompatible with int.” This seemingly cryptic message can halt progress and lead to frustration, especially for those who may not be well-versed in data types and their interactions. Understanding this error is crucial, as it not only highlights the importance of data type compatibility but also serves as a gateway to mastering more complex coding challenges.
As we delve into the intricacies of this error, we’ll explore the fundamental concepts of data types, focusing particularly on how dates and integers interact within various programming environments. The clash between these two types can arise in numerous contexts, from SQL queries to programming languages like Python and Java. By dissecting the underlying causes of this error, we can equip ourselves with the knowledge to prevent it in the future and enhance our coding skills.
Moreover, we will discuss best practices for handling data type compatibility, providing insights into how to effectively manage and manipulate data in a way that avoids such conflicts. Whether you’re a seasoned developer or a newcomer to the field, understanding the nuances of operand type clashes will empower you to write cleaner, more efficient code and navigate the complexities
Understanding Operand Type Clash
Operand type clashes typically occur in programming and database contexts when there is an attempt to perform operations on incompatible data types. One common scenario involves dates and integers, leading to the specific error message: “operand type clash date is incompatible with int.”
When you encounter this error, it indicates that a date value is being used in a context where an integer is expected, or vice versa. This mismatch can happen in various situations, such as during comparisons, arithmetic operations, or when inserting or updating records in a database.
Common Causes of the Error
Several factors can contribute to this operand type clash:
- Mismatched Data Types: Using a date in a calculation where an integer is required.
- Incorrect Query Syntax: Writing SQL queries that do not properly convert data types.
- Function Misuse: Applying functions that expect one data type but receiving another.
Resolving Operand Type Clash Issues
To resolve the operand type clash, it is essential to ensure that the data types in your operations or queries are compatible. Here are some strategies:
- Type Conversion: Use explicit conversion functions to ensure data types match. For example, in SQL, you can convert a date to an integer using `CAST` or `CONVERT`.
- Check Data Types: Review the definitions of your database tables or variables to confirm that the expected data types align with your operations.
- Debugging Queries: Break down complex queries into simpler parts to isolate the point where the type clash occurs.
Example of Type Conversion in SQL
To illustrate the type conversion, consider the following SQL snippet:
“`sql
SELECT *
FROM orders
WHERE order_date = CAST(123456 AS DATE);
“`
In this case, attempting to compare an integer (`123456`) directly with a date (`order_date`) will result in an operand type clash. Instead, you should ensure the integer is converted to a date format if it represents a valid date value.
Best Practices to Avoid Type Clashes
To minimize the risk of encountering operand type clashes in the future, adhere to these best practices:
- Consistent Data Types: Maintain consistency in data types across your application and database schema.
- Use Strong Typing: Where applicable, use programming languages that enforce strong typing to catch errors at compile time.
- Regular Testing: Implement unit tests and integration tests to catch type-related errors early in the development process.
Summary Table of Data Type Conversions
Original Data Type | Target Data Type | Conversion Function |
---|---|---|
DATE | INT | CAST(date AS INT) |
INT | DATE | CAST(int AS DATE) |
VARCHAR | DATE | CAST(varchar AS DATE) |
By implementing these strategies and practices, you can effectively manage and prevent operand type clash errors in your applications and databases.
Understanding the Operand Type Clash Error
The error message “operand type clash: date is incompatible with int” typically occurs in SQL databases when there is an attempt to perform operations between incompatible data types. This often arises in scenarios involving date comparisons or calculations where one operand is a date and the other is an integer.
Common Scenarios Leading to the Error
Several situations can lead to this error:
- Date Comparisons: Attempting to compare a date field with an integer value directly.
- Inappropriate Function Usage: Using aggregate functions or calculations that expect a numeric value but receive a date instead.
- Incorrect Casting: Failing to properly cast or convert data types in queries.
Examples of the Error
Consider the following SQL query:
“`sql
SELECT *
FROM orders
WHERE order_date > 20230101;
“`
In this example, `order_date` is a date type, while `20230101` is treated as an integer. The comparison fails due to type mismatch.
Another example is:
“`sql
SELECT COUNT(*)
FROM events
WHERE event_date – 1 > GETDATE();
“`
Here, subtracting an integer from a date type leads to an operand type clash.
Solutions and Best Practices
To resolve and prevent the operand type clash error, consider the following approaches:
– **Use Proper Date Formats**: Ensure that all date comparisons use date literals or functions. For example, use `CAST` or `CONVERT` to ensure type compatibility.
“`sql
SELECT *
FROM orders
WHERE order_date > CAST(‘2023-01-01’ AS DATE);
“`
– **Perform Type Casting**: Explicitly cast integers to dates if necessary.
“`sql
SELECT *
FROM events
WHERE event_date > CAST(20230101 AS DATE);
“`
– **Review Function Requirements**: Check the documentation for SQL functions to confirm that the correct data types are being used.
– **Employ Date Functions**: Utilize built-in date functions that return date types to avoid type conflicts.
“`sql
SELECT COUNT(*)
FROM events
WHERE event_date > DATEADD(DAY, -1, GETDATE());
“`
Preventive Measures
Implement the following strategies to mitigate the risk of encountering this error:
- Data Type Awareness: Always be aware of the data types being used in your queries.
- Testing and Validation: Test queries thoroughly in a development environment before deploying to production.
- Code Reviews: Encourage code reviews to catch potential type clashes early in the development process.
Understanding and addressing the “operand type clash: date is incompatible with int” error is crucial for smooth database operations. By using proper data types and functions, you can ensure that your SQL queries execute without type-related issues.
Understanding Operand Type Clash: Insights from Database Experts
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “The operand type clash error, specifically regarding the incompatibility of date and int types, often arises when developers attempt to perform arithmetic operations on date fields without proper conversion. It is essential to ensure that data types align correctly to avoid runtime errors.”
Mark Thompson (Senior Software Engineer, Data Solutions Corp.). “When encountering the ‘operand type clash date is incompatible with int’ error, it is crucial to examine the SQL queries for implicit conversions. Utilizing explicit type casting can often resolve these issues and enhance the clarity of the code.”
Linda Patel (Database Consultant, OptimizeDB). “This error typically indicates a misunderstanding of how different data types interact within SQL operations. Developers should familiarize themselves with the implications of data types in their queries, particularly when dealing with date functions and integer comparisons.”
Frequently Asked Questions (FAQs)
What does “operand type clash date is incompatible with int” mean?
This error indicates that there is a type mismatch in an operation, where a date type is being compared or used with an integer type, which is not allowed in many programming languages and databases.
How can I resolve the operand type clash error?
To resolve this error, ensure that both operands in the operation are of compatible types. Convert the date to an integer format (e.g., a timestamp) or the integer to a date format, depending on the context of the operation.
What are common scenarios that lead to this error?
Common scenarios include attempting to perform arithmetic operations between date fields and integer values, or using date fields in conditional statements where integers are expected.
Is this error specific to any programming language or database?
While the error message may vary, the concept of operand type clash is common across many programming languages and databases, including SQL, Python, and Java. Each may have its own specific error message.
Can I prevent this error from occurring in my code?
Yes, you can prevent this error by implementing type checks and validations before performing operations. Use explicit type conversion functions to ensure compatibility between different data types.
What tools can help identify and fix type clashes in code?
Many integrated development environments (IDEs) and static analysis tools can help identify type clashes. Tools like linters and type checkers (e.g., TypeScript for JavaScript, MyPy for Python) can provide warnings and suggestions for resolving such issues.
The error message “operand type clash: date is incompatible with int” typically arises in database operations, particularly within SQL queries. This error indicates that there is an attempt to perform an operation that involves incompatible data types, specifically trying to compare or manipulate a date type with an integer type. Such type clashes can occur in various scenarios, including conditional statements, mathematical operations, or when passing parameters to functions that expect specific data types.
To resolve this issue, it is essential to ensure that the data types being used in the operation are compatible. This may involve converting the date type to an integer format or vice versa, depending on the context of the operation. For instance, if the goal is to compare a date with a numeric value representing a timestamp, appropriate conversion functions should be utilized to align the data types correctly. Understanding the underlying data types and their expected formats is crucial for preventing such errors.
In summary, the operand type clash error serves as a reminder of the importance of data type compatibility in programming and database management. Developers and database administrators should be vigilant about the data types they are working with and implement proper type conversions when necessary. By doing so, they can avoid runtime errors and ensure smoother execution of their queries and operations.
Author Profile
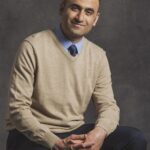
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?