How to Resolve the Oracle Error ORA-01422: Why Does an Exact Fetch Return More Rows Than Expected?
In the realm of database management, encountering errors can be a frustrating yet enlightening experience. Among the myriad of Oracle error codes, `ORA-01422: exact fetch returns more than requested number of rows` stands out as a common yet perplexing issue for developers and database administrators alike. This error serves as a crucial reminder of the importance of data integrity and the need for precise query handling in SQL. As we delve into the intricacies of this error, we will explore its causes, implications, and effective strategies for resolution, empowering you to navigate the complexities of Oracle databases with confidence.
Overview
The `ORA-01422` error typically arises when a SQL query that is expected to return a single row instead returns multiple rows, leading to a mismatch between the anticipated and actual results. This situation often occurs in scenarios where developers rely on single-row fetches, such as when using cursors or PL/SQL blocks, without adequately accounting for the possibility of multiple matches in the underlying data. Understanding the context in which this error occurs is essential for diagnosing and addressing the root cause effectively.
Resolving the `ORA-01422` error involves a careful examination of the SQL query and the data it interacts with. By analyzing the conditions and constraints applied to the query
Understanding ORA-01422
The Oracle error code ORA-01422 indicates that an exact fetch operation has returned more rows than requested. This error typically occurs in scenarios where a SQL query is expected to return a single row but instead returns multiple rows. The implications of this error can significantly affect application functionality and data integrity.
Common Causes
Several factors can lead to the ORA-01422 error, including:
- Improper WHERE Clause: When the conditions in the WHERE clause are not restrictive enough, they can lead to multiple rows being returned.
- Data Anomalies: Duplicate records in the underlying data can cause queries designed to fetch unique results to return multiple rows.
- Incorrect Use of SELECT INTO: This error often arises when using the SELECT INTO statement in PL/SQL. If the query returns more than one row, the error is raised.
Example Scenario
Consider the following SQL statement that attempts to fetch a single employee’s details based on their employee ID:
“`sql
DECLARE
v_employee_name VARCHAR2(100);
BEGIN
SELECT employee_name INTO v_employee_name
FROM employees
WHERE department_id = 10;
END;
“`
If the `employees` table contains multiple records for department_id 10, executing this block will result in the ORA-01422 error.
Solutions to Resolve ORA-01422
To address this error, several approaches can be taken:
- Refine the Query: Ensure that the WHERE clause uniquely identifies a single record.
- Use Aggregation: If appropriate, you can use aggregate functions (e.g., MAX, MIN) to reduce the result set to a single row.
- Implement Exception Handling: Use PL/SQL exception handling to gracefully manage situations where multiple rows are returned.
Example of Handling the Error
Here is an example that incorporates exception handling to manage the ORA-01422 error:
“`sql
DECLARE
v_employee_name VARCHAR2(100);
BEGIN
SELECT employee_name INTO v_employee_name
FROM employees
WHERE department_id = 10;
EXCEPTION
WHEN TOO_MANY_ROWS THEN
DBMS_OUTPUT.PUT_LINE(‘Error: More than one employee found for the specified department.’);
END;
“`
Best Practices to Avoid ORA-01422
To minimize the occurrence of this error, consider the following best practices:
- Design Unique Constraints: Ensure that your database schema enforces uniqueness where necessary.
- Regular Data Cleaning: Periodically review and clean data to remove duplicates.
- Use ROW_NUMBER(): For complex queries where multiple rows may be valid, consider using the ROW_NUMBER() analytic function to limit results.
Action | Description |
---|---|
Refine Query | Narrow the search criteria to ensure a single result is returned. |
Aggregate Data | Use aggregation to ensure the result set is condensed to one row. |
Implement Handling | Use exception handling to manage scenarios where multiple rows are returned. |
Understanding ORA-01422 Error
The ORA-01422 error occurs in Oracle databases when an SQL query that is expected to return a single row fetches multiple rows instead. This often arises when using a cursor or a SELECT statement that is intended to return a single result but encounters multiple matching records.
Common Causes
Several scenarios can lead to the ORA-01422 error:
- Improper Use of SELECT INTO: When using the `SELECT INTO` statement, if the query returns more than one row, the error occurs.
- Filters and Joins: Queries that do not sufficiently filter data or have joins that introduce additional rows can lead to multiple results being returned.
- Dynamic Queries: In dynamic SQL, if the conditions change unexpectedly, the result set may include more rows than anticipated.
Example Scenarios
To illustrate how this error can manifest, consider the following examples:
- **Using SELECT INTO**:
“`sql
DECLARE
v_employee_name employees.name%TYPE;
BEGIN
SELECT name INTO v_employee_name
FROM employees
WHERE department_id = 10; — Assumes multiple employees in department 10
END;
“`
In this case, if multiple employees belong to department 10, the error will occur.
- **Aggregating Data**:
If an aggregate function is not used correctly, for instance:
“`sql
SELECT name
FROM employees
WHERE salary > 50000;
“`
If multiple employees meet the criteria, and you expect only one name, the error arises.
Prevention Strategies
To avoid the ORA-01422 error, consider the following strategies:
- Use Aggregate Functions: If applicable, utilize functions such as `MAX`, `MIN`, or `COUNT` to ensure a single result is returned.
- Limit Results: Implement `ROWNUM` or `FETCH FIRST 1 ROWS ONLY` to restrict the number of rows returned.
- Check Data Integrity: Regularly review and clean up data to ensure that queries are not unexpectedly returning multiple rows.
- Use Exception Handling: Implement exception handling in PL/SQL to manage potential errors gracefully.
Handling the Error
When encountering the ORA-01422 error, developers can take the following actions:
- Debugging Queries: Analyze the SQL query to identify why multiple rows are returned. Utilize tools such as SQL*Plus or SQL Developer to run the query in isolation.
- Modify Logic: Adjust the logic of the query to ensure it aligns with the intended outcome. This may involve refining WHERE clauses or restructuring joins.
- Implementing Cursors: If the application logic requires handling multiple rows, consider using cursors instead of `SELECT INTO`.
Conclusion on Error Handling
Efficient error handling is crucial in database applications. By understanding the causes and implementing preventive measures, developers can minimize the occurrence of the ORA-01422 error and enhance the robustness of their database interactions.
Understanding the ora-01422 Error in Oracle Databases
Dr. Emily Chen (Database Administrator, Oracle Solutions Inc.). “The ora-01422 error typically arises when a query that is expected to return a single row actually returns multiple rows. This situation often indicates a flaw in the logic of the SQL query, necessitating a review of the WHERE clause or the use of aggregate functions to ensure that only one row is returned.”
Michael Thompson (Senior SQL Developer, Data Insights Corp.). “When encountering the ora-01422 error, it is essential to analyze the underlying data and the structure of the query. Implementing a DISTINCT clause or refining the selection criteria can help mitigate this issue, ensuring that the query aligns with the intended outcome of returning a single result.”
Lisa Patel (Data Analyst, Tech Innovations Group). “In my experience, the ora-01422 error serves as a critical reminder of the importance of data integrity and query precision. Developers should consider using PL/SQL blocks with exception handling to gracefully manage scenarios where multiple rows are unexpectedly returned, thereby enhancing the robustness of their applications.”
Frequently Asked Questions (FAQs)
What does the error ORA-01422 mean?
The error ORA-01422 indicates that a query expected to return a single row has instead returned multiple rows. This typically occurs when using a SELECT statement with a single-row fetch mechanism, such as in PL/SQL or when using a cursor.
What causes the ORA-01422 error?
The ORA-01422 error is caused by executing a query that retrieves more than one row when the application logic expects only one. This can happen due to incorrect WHERE clause conditions or data inconsistencies in the database.
How can I resolve the ORA-01422 error?
To resolve the ORA-01422 error, ensure that your query is designed to return only one row. This can be achieved by refining the WHERE clause, using aggregate functions, or applying DISTINCT if appropriate. Alternatively, consider using a cursor or PL/SQL block that can handle multiple rows.
Can I ignore the ORA-01422 error?
Ignoring the ORA-01422 error is not advisable, as it indicates a logical flaw in your query or application logic. Addressing the issue is essential to ensure the integrity of your data retrieval processes and to avoid unexpected application behavior.
Are there any best practices to avoid ORA-01422?
Best practices to avoid the ORA-01422 error include thoroughly validating your query logic, using LIMIT or ROWNUM to restrict the number of returned rows, and implementing error handling in your PL/SQL code to manage situations where multiple rows may be returned.
What should I do if I need multiple rows but still receive ORA-01422?
If you need to retrieve multiple rows, modify your code to use a collection or cursor that can handle multiple results. Ensure that your logic accommodates the expected number of rows, and avoid using single-row fetch methods in such cases.
The Oracle error code ORA-01422 indicates that a query intended to return a single row has instead returned multiple rows. This situation typically arises when a SELECT statement using a cursor or a single-row fetch mechanism encounters more data than it was designed to handle. Such errors can disrupt application functionality and lead to unexpected behavior, necessitating a thorough understanding of the underlying causes and appropriate resolutions.
One of the primary reasons for encountering ORA-01422 is the lack of constraints in the SQL query that would limit the result set to a single row. This can occur when using aggregate functions without proper grouping, failing to include a WHERE clause, or misconfiguring JOIN operations. To prevent this error, developers should ensure that their queries are explicitly designed to return a single row, utilizing techniques such as adding appropriate filters or using aggregate functions correctly.
In resolving ORA-01422, it is crucial to analyze the query structure and the data being queried. Implementing error handling mechanisms, such as exception blocks in PL/SQL, can help manage situations where multiple rows are returned. Additionally, reviewing the logic of the application that generates the SQL queries may reveal opportunities for optimization and refinement, thus reducing the risk of encountering this error in the future.
Author Profile
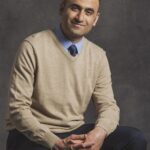
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?