Why Am I Seeing the ‘pip install error: cannot combine ‘–user’ and ‘–target’?’ and How Can I Fix It?
When working with Python’s package manager, pip, you may encounter a variety of errors that can halt your development progress. One such error that can be particularly perplexing is the message: “can not combine ‘–user’ and ‘–target’.” This error arises from the complexities of package installation options and can leave even seasoned developers scratching their heads. Understanding the nuances of pip’s command-line arguments is crucial for seamless package management and can save you time and frustration in your coding journey.
At its core, this error stems from conflicting installation directives that pip cannot reconcile. The `–user` flag is designed to install packages in the user’s home directory, while the `–target` option specifies a custom directory for installation. When these two options are used together, pip is unable to determine the correct installation path, leading to the error message that interrupts your workflow. This situation often arises in environments where multiple Python versions or virtual environments are in play, making it essential to grasp the implications of each installation method.
Navigating pip’s installation options effectively can empower you to manage dependencies and packages with confidence. By understanding the underlying principles of how pip interprets these flags and the contexts in which they are applicable, you can avoid common pitfalls and streamline your development process. In the following sections,
Understanding the Error
When you encounter the error message `pip install error: can not combine ‘–user’ and ‘–target’`, it indicates a conflict in the command-line arguments being used with the `pip` package installer. This error typically arises when attempting to specify multiple installation options that are incompatible with one another.
The `–user` flag is used to install packages into the user’s home directory, while the `–target` option specifies a custom directory where the package should be installed. These two options cannot be combined because they direct `pip` to install packages in two different locations, leading to ambiguity about the desired installation path.
Common Scenarios Leading to the Error
The error can occur in several scenarios:
- Using both flags in a single command: If you inadvertently include both `–user` and `–target` in your `pip install` command.
Example:
“`bash
pip install –user –target=/path/to/directory package_name
“`
- Script or Environment Issues: Sometimes, scripts or environment configurations may implicitly add one of the flags, leading to the error when you run an installation command.
How to Resolve the Error
To fix the issue, you need to decide between the two installation methods and modify your command accordingly. Here’s how to address it:
- Choose User Installation: If you want to install the package for your user only, remove the `–target` option.
Correct command:
“`bash
pip install –user package_name
“`
- Choose Target Installation: If you want to install the package in a specific directory, omit the `–user` flag.
Correct command:
“`bash
pip install –target=/path/to/directory package_name
“`
Best Practices for Using pip
To avoid similar errors in the future, consider the following best practices:
- Be Consistent with Installation Methods: Stick to either user installations or target installations for a specific project or environment.
- Use Virtual Environments: Create virtual environments using `venv` or `virtualenv` to avoid package conflicts and manage dependencies more effectively. This allows you to install packages without needing `–user` or `–target`.
Example to create a virtual environment:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use myenv\Scripts\activate
“`
- Review Command Options: Before executing `pip install`, double-check the options to ensure they are compatible.
Installation Method | Command Example | Use Case |
---|---|---|
User Installation | pip install --user package_name |
Install for the current user, without admin rights |
Target Installation | pip install --target=/path/to/directory package_name |
Install to a specified directory |
Virtual Environment | python -m venv myenv |
Isolated environment for project dependencies |
Understanding the Error
The error message `can not combine ‘–user’ and ‘–target’` occurs when trying to use both the `–user` and `–target` options in a single `pip install` command. This situation arises because these two options serve different purposes and are mutually exclusive.
- `–user`: Installs a package to the user’s home directory. This is useful when you do not have administrative privileges or wish to avoid affecting the global Python environment.
- `–target`: Specifies a custom directory for installation. This option is often used for portable environments or when managing dependencies in specific locations.
Using both options together creates a conflict as `pip` does not know which installation method to prioritize.
Common Scenarios Leading to the Error
Several situations may lead to encountering this error:
- Misconfigured Environment: A user may inadvertently set environment variables or configurations that lead to combining these flags.
- Copy-Paste Errors: Users might copy commands from different sources that include both flags without understanding their implications.
- Script Issues: Automated scripts that manage package installations may erroneously include both options due to conditional logic flaws.
How to Resolve the Error
To resolve this error, you should decide between using `–user` or `–target` based on your specific needs. Here are steps to correct the command:
- Decide on the Installation Method:
- If you want to install the package just for your user account, use `–user`.
- If you need to specify a particular directory for installation, use `–target`.
- Modify the Command:
- For user installation:
“`bash
pip install –user package_name
“`
- For target directory installation:
“`bash
pip install –target /path/to/directory package_name
“`
- Verify Environment:
- Ensure there are no configurations that could automatically apply conflicting options.
Best Practices to Avoid the Error
To prevent future occurrences of this error, consider adopting the following best practices:
- Understand Options: Familiarize yourself with `pip` options and their purposes.
- Check Command Syntax: Always review your `pip` command syntax before execution.
- Utilize Virtual Environments: Use virtual environments (e.g., `venv` or `conda`) to manage dependencies cleanly without conflicting with global installations.
- Documentation Reference: Regularly consult the official `pip` documentation for the latest practices and updates regarding installation commands.
Example Commands
The following table summarizes examples of valid `pip install` commands without conflicts:
Command Type | Command Example |
---|---|
User Installation | `pip install –user requests` |
Target Directory Installation | `pip install –target /custom/path requests` |
By adhering to these guidelines and understanding the implications of each option, users can effectively manage their Python package installations without encountering the `–user` and `–target` conflict.
Resolving the ‘pip install’ User and Target Conflict
Dr. Emily Carter (Python Package Management Specialist, Tech Solutions Inc.). “The error message regarding the combination of ‘–user’ and ‘–target’ typically arises when users attempt to specify multiple installation paths for a package. It is crucial to understand that the ‘–user’ flag installs packages in the user’s home directory, while the ‘–target’ flag specifies a different directory. Using both simultaneously is not supported, and users should choose one method based on their installation needs.”
James Liu (Senior Software Engineer, Open Source Foundation). “When encountering the ‘can not combine ‘–user’ and ‘–target’?’ error, developers should evaluate their project requirements. If the intention is to install a package globally for all users, the ‘–user’ option should be omitted. Conversely, if a specific directory installation is necessary, the ‘–user’ flag should not be used. Clarity in installation paths can prevent such conflicts.”
Sarah Thompson (DevOps Engineer, Cloud Innovations). “This error often confuses new developers, but it highlights the importance of understanding Python’s package management system. I recommend reviewing the documentation for ‘pip’ to grasp the implications of both flags. Additionally, using virtual environments can help avoid such conflicts by providing isolated spaces for package installations without the need for user or target specifications.”
Frequently Asked Questions (FAQs)
What does the error “can not combine ‘–user’ and ‘–target'” mean?
This error occurs when you attempt to use both the `–user` and `–target` options simultaneously in a `pip install` command. These options are mutually exclusive, as `–user` installs packages in the user’s site-packages directory, while `–target` specifies a custom directory for installation.
How can I resolve the “can not combine ‘–user’ and ‘–target'” error?
To resolve this error, choose either the `–user` option or the `–target` option, but not both. If you want to install packages for your user account, use `–user`. If you prefer a specific directory, use `–target` without `–user`.
What is the purpose of the `–user` option in pip?
The `–user` option allows users to install Python packages in their home directory, specifically in the user site-packages directory. This avoids the need for administrative privileges and does not interfere with system-wide packages.
When should I use the `–target` option in pip?
The `–target` option is useful when you want to install packages in a specific directory other than the default site-packages. This is particularly beneficial for applications that require isolated environments or when deploying applications with specific dependencies.
Can I use virtual environments to avoid this error?
Yes, using virtual environments is an effective way to avoid this error. By creating a virtual environment, you can manage dependencies in isolation without needing to specify `–user` or `–target`, thus preventing any conflicts.
What are the implications of using `–user` and `–target` incorrectly?
Using `–user` and `–target` together will result in a failure to install the desired package, as pip cannot determine the correct installation path. This may lead to confusion and additional troubleshooting steps to rectify the installation process.
The error message “can not combine ‘–user’ and ‘–target'” occurs when using the pip package installer in Python. This message indicates a conflict in the command-line options provided to pip. Specifically, the ‘–user’ flag is used to install packages for the current user, while the ‘–target’ option specifies a directory to which the package should be installed. These two options are mutually exclusive, and attempting to use them together results in this error.
To resolve this issue, users must choose one of the two options based on their installation needs. If the intention is to install a package for the current user without requiring administrative privileges, the ‘–user’ flag should be used alone. Conversely, if the goal is to install the package to a specific directory, the ‘–target’ option should be utilized without the ‘–user’ flag. Understanding the context in which each option is appropriate can prevent this error from occurring.
Additionally, it is important for users to familiarize themselves with the pip documentation to understand the implications of the various command-line options available. Properly managing package installations can enhance the development environment and reduce conflicts. By adhering to the guidelines provided by pip, users can effectively manage their Python packages and avoid common pitfalls associated with installation errors.
Author Profile
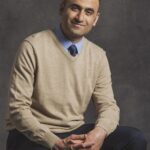
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?