How Can I Use Playwright in Python to Scroll to a Specific Element?
In the fast-evolving world of web automation and testing, Playwright has emerged as a powerful tool that allows developers to interact with web applications seamlessly. With its robust capabilities, Playwright simplifies the process of scripting complex user interactions, making it a favorite among testers and developers alike. One common challenge that arises during automation tasks is the need to scroll to specific elements on a webpage. Whether it’s to ensure visibility for testing or to trigger lazy loading features, mastering the art of scrolling to elements is essential for effective automation.
In this article, we will explore how to efficiently scroll to elements using Playwright with Python. Understanding the techniques and methods available for scrolling can significantly enhance your automation scripts, allowing for smoother interactions and more reliable tests. We will delve into the various approaches you can take, from simple scroll commands to more advanced strategies that ensure your target elements are in view before performing actions on them.
As we navigate through the intricacies of scrolling in Playwright, you’ll discover practical examples and best practices that will empower you to optimize your automation workflows. Whether you’re a seasoned developer or just starting with Playwright, this guide will equip you with the knowledge to tackle scrolling challenges head-on, ensuring your automated tests run flawlessly every time. Get ready to unlock the full potential of Playwright and
Scrolling to an Element in Playwright with Python
To scroll to an element in Playwright using Python, you can use the `scroll_into_view_if_needed` method. This method ensures that the specified element is brought into view, allowing for any interactions or assertions that need to take place.
Here’s how to effectively implement scrolling to an element:
- Ensure that the Playwright environment is set up and the necessary libraries are imported.
- Locate the element you wish to scroll to using a selector.
- Call the `scroll_into_view_if_needed` method on the element handle.
Example Code Snippet:
“`python
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto(“https://example.com”)
Locate the element
element = page.query_selector(“selector-for-element”)
Scroll to the element
element.scroll_into_view_if_needed()
Optionally, perform actions on the element
element.click()
browser.close()
“`
In the above example, replace `”selector-for-element”` with the appropriate CSS selector for your target element. This method is particularly useful in scenarios where the element may be out of the viewport initially.
Handling Dynamic Content Loading
When dealing with dynamically loaded content, such as single-page applications (SPAs), it is crucial to ensure the element is present before attempting to scroll to it. This can be handled using Playwright’s wait mechanisms.
Consider the following strategies:
- Using `wait_for_selector`: This method will pause the execution until the specified element appears in the DOM.
- Timeout Management: Adjusting the timeout can help in scenarios with slower loading times.
Example Code Snippet:
“`python
page.wait_for_selector(“selector-for-element”, timeout=5000)
element = page.query_selector(“selector-for-element”)
element.scroll_into_view_if_needed()
“`
Best Practices for Scrolling
When implementing scrolling in your Playwright scripts, consider the following best practices:
- Avoid Excessive Scrolling: If the target element is not present after a reasonable timeout, consider handling the situation with error logging or retries.
- Use Assertions: After scrolling, it may be prudent to include assertions to confirm the element is interactable.
- Performance Considerations: Minimize the number of scroll operations to enhance script execution performance.
Common Issues and Troubleshooting
While scrolling to elements, you may encounter several common issues. Below is a table summarizing these issues along with possible solutions.
Issue | Solution |
---|---|
Element not found | Ensure the selector is correct and use `wait_for_selector`. |
Element is not interactable | Check if the element is obscured by other elements or not visible. |
Timeout errors | Increase the timeout duration or check for network issues. |
By following these guidelines and understanding the tools provided by Playwright, you can effectively manage scrolling to elements within your automated tests.
Scrolling to an Element with Playwright in Python
In Playwright for Python, scrolling to an element can be accomplished using the built-in functions that allow for element interactions. This process is essential when dealing with dynamic content where elements are not immediately visible in the viewport.
Methods to Scroll to an Element
There are several effective methods to scroll to an element in Playwright:
– **Using the `scrollIntoViewIfNeeded` Method**: This method ensures that an element is scrolled into view if it is not already visible.
“`python
await page.locator(‘selector’).scroll_into_view_if_needed()
“`
– **Using JavaScript Execution**: For more complex scenarios, you can execute JavaScript to scroll the element into view.
“`python
await page.evaluate(“element => element.scrollIntoView()”, await page.query_selector(‘selector’))
“`
- Setting Viewport: Adjusting the viewport size can also help in making elements visible without explicit scrolling.
“`python
await page.set_viewport_size({“width”: 1200, “height”: 800})
“`
Example of Scrolling to an Element
Here is a practical example that demonstrates how to scroll to an element and perform an action afterward:
“`python
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto(‘https://example.com’)
Scroll to the desired element
element = page.locator(‘selector’)
element.scroll_into_view_if_needed()
Perform an action on the element
element.click()
browser.close()
“`
Tips for Scrolling
- Visibility Check: Always ensure that the element is interactable after scrolling.
- Wait for Load: Use appropriate wait mechanisms (like `page.wait_for_selector`) to ensure that the element is fully loaded before attempting to scroll.
- Dynamic Content: Be aware that dynamically loaded content might require additional waits or checks after scrolling.
Handling Scrolling in Infinite Scroll Scenarios
When dealing with infinite scrolling, where more content loads as you scroll down, consider implementing a loop:
“`python
async def scroll_and_load_more(page):
last_height = await page.evaluate(“document.body.scrollHeight”)
while True:
await page.evaluate(“window.scrollTo(0, document.body.scrollHeight);”)
await page.wait_for_timeout(2000) Wait for new content to load
new_height = await page.evaluate(“document.body.scrollHeight”)
if new_height == last_height:
break
last_height = new_height
“`
This function continuously scrolls down until no new content loads, effectively managing infinite scrolling scenarios.
Understanding how to scroll to elements effectively in Playwright can enhance your web automation tasks significantly. Employing the right methods and handling different scenarios will ensure successful interactions with dynamic web pages.
Expert Insights on Scrolling to Elements with Playwright in Python
Dr. Emily Carter (Senior Software Engineer, Automation Insights). “When utilizing Playwright with Python, scrolling to an element can be efficiently achieved by using the `scrollIntoView` method. This method ensures that the target element is brought into the viewport, allowing for seamless interaction during automated testing.”
Mark Thompson (Lead Developer Advocate, Testing Technologies Inc.). “In Playwright, it is crucial to ensure that the element is not only scrolled into view but also visible and interactable. I recommend combining `scrollIntoView` with assertions to confirm the element’s visibility, which enhances the reliability of your tests.”
Lisa Chen (Automation Testing Specialist, QA World). “To effectively scroll to an element in Playwright using Python, leveraging the built-in `locator` methods is essential. These methods allow for precise targeting of elements, making the scrolling process both straightforward and efficient in your test scripts.”
Frequently Asked Questions (FAQs)
How can I scroll to an element using Playwright in Python?
To scroll to an element in Playwright, use the `scrollIntoView()` method on the element handle. This method ensures the element is brought into the viewport.
What is the syntax for scrolling to an element in Playwright with Python?
The syntax is as follows:
“`python
await element_handle.scroll_into_view_if_needed()
“`
This command will scroll the element into view if it is not already visible.
Can I scroll to an element that is not immediately visible on the page?
Yes, Playwright can scroll to elements that are not visible. The `scrollIntoView()` method will automatically scroll the page until the element is visible.
Is there a built-in delay when scrolling to an element in Playwright?
No, the `scrollIntoView()` method executes immediately without a built-in delay. However, you can implement a custom delay if necessary using `asyncio.sleep()`.
What happens if the element is not found when trying to scroll to it?
If the element is not found, Playwright will raise a `TimeoutError`. It is advisable to ensure that the element exists before attempting to scroll.
Can I customize the scrolling behavior in Playwright?
Yes, you can customize scrolling behavior by using JavaScript to modify the scrolling options. You can use `page.evaluate()` to execute custom scripts for more control over the scrolling action.
In summary, using Playwright with Python to scroll to an element involves leveraging the powerful automation capabilities of the Playwright library. This process typically includes identifying the target element on the webpage and executing a scroll action to bring that element into view. Playwright provides various methods to interact with web elements, including the ability to scroll programmatically, which is essential for testing scenarios where elements may not be immediately visible in the viewport.
One of the key insights is the importance of ensuring that the element is interactable after scrolling. Playwright offers built-in wait mechanisms that can help confirm that the element is ready for interaction, thereby enhancing the reliability of your tests. By using methods such as `scrollIntoViewIfNeeded()`, developers can efficiently manage the visibility of elements, ensuring that user interactions are accurately simulated in automated tests.
Moreover, it is crucial to consider the overall structure of the webpage when scrolling to elements. Dynamic content, lazy loading, and other factors can affect how elements are displayed. Understanding these aspects can lead to more effective test scripts and better handling of various web environments. Ultimately, mastering the scrolling techniques in Playwright will contribute to more robust and effective web automation testing strategies.
Author Profile
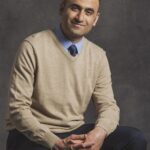
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?