How Can You Populate a SQL Query Using Form Input in JavaScript?
In the world of web development, the seamless integration of user input and database interactions is crucial for creating dynamic and responsive applications. One of the most common challenges developers face is how to effectively populate a SQL query using form input in JavaScript. This task not only requires a solid understanding of SQL syntax but also a keen awareness of security practices to prevent vulnerabilities like SQL injection. As web applications become increasingly complex, mastering this skill is essential for developers looking to build robust and secure data-driven solutions.
At its core, the process of populating a SQL query with form input involves capturing user data from HTML forms and then dynamically constructing a SQL statement that can be executed against a database. This interplay between the front-end and back-end is fundamental to many applications, whether they are simple contact forms or complex data management systems. Understanding how to properly handle user input and translate it into SQL commands can significantly enhance the functionality and user experience of your application.
Moreover, it’s vital to consider the implications of user-generated data on database security. Developers must implement best practices, such as parameterized queries and prepared statements, to safeguard against potential attacks. By exploring the techniques and strategies for populating SQL queries with form input in JavaScript, you will not only improve your coding skills but also ensure that your
Understanding the Basics of SQL Query Population
To effectively populate a SQL query using form input in JavaScript, it’s essential to grasp the basic principles of SQL syntax and how to manipulate form data. This process typically involves gathering user input from HTML forms, validating that input, and then dynamically constructing a SQL query that can be executed against a database.
When populating a SQL query, consider the following key steps:
- Collect User Input: Use JavaScript to extract data from form fields.
- Validate Input: Ensure the data is sanitized to prevent SQL injection attacks.
- Construct the Query: Dynamically build the SQL statement using the validated input.
Collecting Input from HTML Forms
HTML forms are the primary means of collecting user input. To retrieve data, you will typically use JavaScript’s `document.getElementById` or `querySelector` methods. Below is a simple example of an HTML form:
“`html
“`
You can collect input from this form with the following JavaScript code:
“`javascript
document.getElementById(‘userForm’).addEventListener(‘submit’, function(event) {
event.preventDefault();
var username = document.getElementById(‘username’).value;
var email = document.getElementById(’email’).value;
// Validate and use these values to construct the SQL query
});
“`
Validating Input to Prevent SQL Injection
Input validation is crucial for security. Always sanitize user input before using it in SQL queries. Here are some common validation practices:
- Type Checking: Ensure data types match expected values (e.g., strings for usernames).
- Length Checking: Limit the length of input fields to avoid overflow.
- Whitelist Approach: Use a whitelist of acceptable values when possible.
You can implement basic validation in JavaScript as follows:
“`javascript
function validateInput(username, email) {
var usernameRegex = /^[a-zA-Z0-9]{3,20}$/; // Example regex for username
var emailRegex = /^[^@\s]+@[^@\s]+\.[^@\s]+$/; // Simple email validation
return usernameRegex.test(username) && emailRegex.test(email);
}
“`
Constructing the SQL Query
Once you have validated the input, you can construct your SQL query. However, it’s imperative to use parameterized queries or prepared statements to prevent SQL injection. Here’s an example of how to construct a simple SQL insert statement:
“`javascript
if (validateInput(username, email)) {
var sqlQuery = `INSERT INTO users (username, email) VALUES (?, ?)`;
// Use a database library to execute the query with parameters
}
“`
Example of Using a Database Library
Using libraries like `mysql` in Node.js allows you to execute SQL queries securely. Here’s how you might incorporate user input into a database operation:
“`javascript
var mysql = require(‘mysql’);
var connection = mysql.createConnection({
host: ‘localhost’,
user: ‘user’,
password: ‘password’,
database: ‘database’
});
connection.connect();
var sqlQuery = ‘INSERT INTO users (username, email) VALUES (?, ?)’;
connection.query(sqlQuery, [username, email], function (error, results) {
if (error) throw error;
console.log(‘User added: ‘, results.insertId);
});
connection.end();
“`
Summary of Important Points
When populating SQL queries using form input in JavaScript, it is essential to:
- Collect and validate input effectively.
- Use prepared statements to secure against SQL injection.
- Utilize a database library that supports parameterized queries.
Action | Best Practice |
---|---|
Input Collection | Use form controls and JavaScript DOM methods |
Input Validation | Implement regex checks and length restrictions |
SQL Query Construction | Use parameterized queries to mitigate risks |
Using JavaScript to Populate SQL Queries
When collecting user input through forms, it’s essential to securely populate SQL queries to prevent SQL injection attacks. Below are the steps to effectively achieve this while maintaining security.
Form Input Handling
To begin, gather user input from HTML forms using JavaScript. This can be done using the `document.getElementById()` or `document.querySelector()` methods to access form elements.
“`html
“`
JavaScript code to capture the input:
“`javascript
document.getElementById(‘userForm’).onsubmit = function(event) {
event.preventDefault(); // Prevent the default form submission
const name = document.getElementById(‘name’).value;
const email = document.getElementById(’email’).value;
// Call function to execute SQL query
executeSQLQuery(name, email);
};
“`
Building SQL Queries
When constructing SQL queries, it is crucial to use parameterized queries or prepared statements to prevent SQL injection. The following example demonstrates how to create a query using placeholders:
“`javascript
function executeSQLQuery(name, email) {
const sqlQuery = “INSERT INTO users (name, email) VALUES (?, ?)”;
const params = [name, email];
// Function to execute the query using a database connection
database.execute(sqlQuery, params)
.then(result => {
console.log(“User added successfully:”, result);
})
.catch(error => {
console.error(“Error adding user:”, error);
});
}
“`
Using AJAX for Server Communication
To send the populated SQL query to the server without refreshing the page, utilize AJAX. Below is an example using the Fetch API:
“`javascript
function executeSQLQuery(name, email) {
const sqlQuery = “INSERT INTO users (name, email) VALUES (?, ?)”;
const params = { name, email };
fetch(‘/api/execute-query’, {
method: ‘POST’,
headers: {
‘Content-Type’: ‘application/json’,
},
body: JSON.stringify({ sqlQuery, params })
})
.then(response => response.json())
.then(data => {
console.log(“User added successfully:”, data);
})
.catch(error => {
console.error(“Error adding user:”, error);
});
}
“`
Server-side Implementation
On the server side, use a language like Node.js with an SQL library such as `mysql` or `pg` for PostgreSQL. Here’s an example of how to handle the request:
“`javascript
const express = require(‘express’);
const mysql = require(‘mysql’);
const bodyParser = require(‘body-parser’);
const app = express();
app.use(bodyParser.json());
const connection = mysql.createConnection({
host: ‘localhost’,
user: ‘root’,
password: ”,
database: ‘mydb’
});
app.post(‘/api/execute-query’, (req, res) => {
const { sqlQuery, params } = req.body;
connection.query(sqlQuery, params, (error, results) => {
if (error) {
return res.status(500).send(error);
}
res.status(200).send(results);
});
});
app.listen(3000, () => {
console.log(‘Server running on port 3000’);
});
“`
Security Best Practices
When working with SQL queries, follow these best practices to enhance security:
- Always use parameterized queries or prepared statements.
- Validate and sanitize all user inputs.
- Implement proper error handling to avoid exposing database details.
- Use HTTPS to encrypt data transmitted between the client and server.
Following these guidelines will help ensure that your application remains secure while effectively handling user input for SQL queries.
Expert Insights on Populating SQL Queries with JavaScript Form Inputs
Dr. Emily Carter (Senior Database Architect, Tech Innovations Inc.). “When populating SQL queries using JavaScript form inputs, it is crucial to ensure that user inputs are properly sanitized to prevent SQL injection attacks. Utilizing prepared statements or parameterized queries is a best practice that enhances security and maintains data integrity.”
Michael Tran (Lead Front-End Developer, Web Solutions Group). “Incorporating form inputs into SQL queries requires a seamless integration between the front-end and back-end. Using AJAX to send form data to the server allows for a dynamic user experience while keeping the application responsive. This method also enables real-time validation of inputs before they reach the database.”
Sarah Kim (Full Stack Developer, CodeCraft Labs). “It is essential to adopt a structured approach when creating SQL queries with JavaScript. Leveraging libraries such as Sequelize or Knex can simplify the process of building queries and managing database interactions, allowing developers to focus on application logic rather than the intricacies of SQL syntax.”
Frequently Asked Questions (FAQs)
How can I safely populate a SQL query using form input in JavaScript?
To safely populate a SQL query using form input, use parameterized queries or prepared statements. This approach helps prevent SQL injection attacks by ensuring that user input is treated as data rather than executable code.
What libraries can help with SQL queries in JavaScript?
Libraries such as Sequelize, Knex.js, and TypeORM are popular choices for building SQL queries in JavaScript. They provide ORM capabilities and support for parameterized queries, simplifying database interactions.
Can I use raw SQL queries with user input in JavaScript?
Yes, you can use raw SQL queries, but it is crucial to sanitize and validate user input to avoid SQL injection vulnerabilities. Always prefer parameterized queries over direct string interpolation.
What is the best practice for handling form input before using it in SQL queries?
Best practices include validating and sanitizing input, using server-side validation, and employing parameterized queries. This ensures that only valid and safe data is processed in SQL queries.
How do I implement input validation for a form in JavaScript?
Input validation can be implemented using HTML5 attributes like `required`, `minlength`, or `pattern`, along with JavaScript functions to check input values before submission. This enhances security and user experience.
What are the risks of directly inserting form input into SQL queries?
Directly inserting form input into SQL queries poses significant risks, primarily SQL injection attacks. Malicious users can manipulate input to execute arbitrary SQL commands, potentially compromising database integrity and security.
Populating a SQL query using form input in JavaScript involves several key steps that ensure data is collected, validated, and safely integrated into a SQL statement. The process typically begins with creating an HTML form that captures user inputs. JavaScript then plays a crucial role in handling these inputs, allowing developers to manipulate the data before it is sent to the server. This interaction is vital for creating dynamic web applications that respond to user actions.
One of the most critical aspects of this process is ensuring the security of the SQL queries. Developers must implement measures to prevent SQL injection attacks, which can occur when user inputs are directly inserted into SQL statements without proper validation or sanitization. Techniques such as prepared statements or parameterized queries are essential in mitigating these risks, thereby enhancing the security of the application.
Moreover, effective error handling and user feedback mechanisms should be integrated into the JavaScript code. This ensures that users are informed of any issues with their input, such as missing fields or incorrect data formats. Providing clear feedback not only improves user experience but also aids in maintaining data integrity within the database.
In summary, populating a SQL query using form input in JavaScript requires a careful blend of user interface design, data validation,
Author Profile
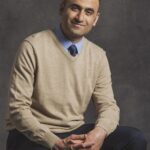
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?