How Can I Use PowerShell to Create a Folder Only If It Doesn’t Already Exist?
In the world of system administration and automation, PowerShell stands out as a powerful tool that streamlines tasks and enhances productivity. One common yet essential task that many users encounter is the need to create folders for organizing files and projects. However, what happens when you attempt to create a folder that already exists? This is where PowerShell’s versatility shines, allowing you to efficiently manage your file system without the risk of errors or duplicates. In this article, we will explore how to leverage PowerShell to create folders only when they do not already exist, ensuring a clean and organized workspace.
Understanding how to conditionally create folders in PowerShell can save you time and prevent unnecessary clutter in your directories. By utilizing simple commands and logic, you can check for the existence of a folder before attempting to create it. This not only helps maintain an orderly file structure but also reduces the chances of script failures due to conflicts. Whether you are a seasoned administrator or a newcomer to PowerShell, mastering this technique can enhance your scripting skills and improve your overall workflow.
As we delve deeper into this topic, we will uncover various methods and best practices for implementing folder creation in PowerShell. You’ll learn how to write scripts that are not only efficient but also robust enough to handle different scenarios. By the end of
Creating a Folder with PowerShell
PowerShell provides a straightforward method to create folders, ensuring that they are created only if they do not already exist. This is particularly useful in scripting and automation tasks where you want to avoid errors due to duplicate folder creation.
To create a folder in PowerShell, you can use the `New-Item` cmdlet along with a conditional check to verify if the folder already exists. The following command demonstrates this process:
“`powershell
$folderPath = “C:\YourFolderName”
if (-Not (Test-Path $folderPath)) {
New-Item -ItemType Directory -Path $folderPath
}
“`
In this example, `$folderPath` is set to the desired folder location. The `Test-Path` cmdlet checks whether the folder exists. If it does not exist (`-Not`), the `New-Item` cmdlet creates the folder.
Explanation of Key Components
- Test-Path: This cmdlet checks if the specified path exists in the file system. It returns `$true` if the path exists and `$` otherwise.
- New-Item: This cmdlet is used to create new items, including files and folders. The `-ItemType Directory` parameter specifies that a folder should be created.
- Conditional Logic: The use of `if` statements allows for decision-making within scripts, enabling the execution of commands based on the existence of the folder.
Example Usage
Here are some practical examples of how to create folders based on different conditions:
Scenario | Command Example | |
---|---|---|
Create a folder in a specific path | `$folderPath = “C:\NewFolder”; if (-Not (Test-Path $folderPath)) { New-Item -ItemType Directory -Path $folderPath }` | |
Create nested folders | `$parentFolder = “C:\ParentFolder”; $childFolder = “C:\ParentFolder\ChildFolder”; if (-Not (Test-Path $parentFolder)) { New-Item -ItemType Directory -Path $parentFolder }; if (-Not (Test-Path $childFolder)) { New-Item -ItemType Directory -Path $childFolder }` | |
Create multiple folders | `@(“Folder1”, “Folder2”, “Folder3”) | ForEach { $folderPath = “C:\$$_”; if (-Not (Test-Path $folderPath)) { New-Item -ItemType Directory -Path $folderPath }}` |
Handling Errors and Logging
When automating folder creation, it’s essential to handle potential errors and log actions for troubleshooting purposes. Here’s how to enhance the script:
“`powershell
$folderPath = “C:\YourFolderName”
try {
if (-Not (Test-Path $folderPath)) {
New-Item -ItemType Directory -Path $folderPath -ErrorAction Stop
Write-Host “Folder created successfully: $folderPath”
} else {
Write-Host “Folder already exists: $folderPath”
}
} catch {
Write-Host “Error creating folder: $_”
}
“`
In this script:
- A `try` block is used to catch any errors during folder creation.
- The `-ErrorAction Stop` parameter forces the script to enter the `catch` block if an error occurs.
- Logging messages provide feedback on the operation’s success or failure, which is invaluable for debugging.
By utilizing these techniques, you can efficiently manage folder creation in PowerShell scripts while ensuring your scripts run smoothly and predictably.
Creating a Folder if It Does Not Exist
To create a folder in PowerShell only if it does not already exist, you can use the `Test-Path` cmdlet in combination with the `New-Item` cmdlet. This approach ensures that your script does not attempt to create a folder that is already present, thus avoiding unnecessary errors.
PowerShell Command Syntax
Here is the basic syntax to create a folder if it does not exist:
“`powershell
$folderPath = “C:\Path\To\Your\Folder”
if (-not (Test-Path -Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory
}
“`
Explanation of Each Component
- $folderPath: This variable holds the path of the folder you want to create. Modify this path according to your needs.
- Test-Path: This cmdlet checks if the specified path exists. It returns `True` if the path exists and “ otherwise.
- -not: This operator negates the result of the `Test-Path` cmdlet, allowing the script to proceed to create the folder only when the path does not exist.
- New-Item: This cmdlet creates a new item. Here, it is used to create a directory specified by the `-Path` parameter.
- -ItemType Directory: This specifies that the item being created is a directory.
Example Scenarios
Below are various scenarios in which this PowerShell command can be employed:
Scenario | Description |
---|---|
Backup Folder Creation | Create a backup folder if it does not exist before copying files. |
Log Directory Setup | Ensure that a directory for logs is created at the start of a script. |
Project Structure | Automatically generate project directories for development environments. |
Alternative Method Using `mkdir`
An alternative and more concise method to achieve the same result is by using the `mkdir` alias for the `New-Item` cmdlet. This command will not throw an error if the folder already exists:
“`powershell
$folderPath = “C:\Path\To\Your\Folder”
mkdir $folderPath
“`
This approach is useful for quick folder creation, as it simplifies the command but does not explicitly check for existence before creating the folder.
Using Try-Catch for Error Handling
For more robust error handling, consider using a `try-catch` block:
“`powershell
$folderPath = “C:\Path\To\Your\Folder”
try {
if (-not (Test-Path -Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory
Write-Host “Folder created successfully.”
} else {
Write-Host “Folder already exists.”
}
} catch {
Write-Error “An error occurred: $_”
}
“`
This method provides feedback on the operation and handles unexpected errors gracefully.
Utilizing PowerShell to create a folder only if it does not exist is a straightforward yet powerful task. By employing the `Test-Path` and `New-Item` cmdlets, or the `mkdir` alias, users can efficiently manage directory structures in their scripts. Error handling further enhances the reliability of these operations, making PowerShell an invaluable tool for system administration and automation tasks.
Expert Insights on Creating Folders in PowerShell
Jessica Tran (Senior Systems Administrator, Tech Solutions Inc.). “Using PowerShell to create a folder only if it does not already exist is a best practice that enhances script efficiency. The command ‘If (-Not (Test-Path -Path ‘C:\YourFolder’)) { New-Item -ItemType Directory -Path ‘C:\YourFolder’ }’ allows for this conditional creation, ensuring that scripts do not fail due to existing directories.”
Michael Chen (PowerShell Expert and Author, Scripting Mastery). “Incorporating error handling when creating folders in PowerShell is crucial. By using ‘Try-Catch’ blocks alongside the folder creation logic, administrators can manage exceptions effectively, thus maintaining the robustness of their scripts while ensuring that the desired folder structure is in place.”
Linda Patel (IT Automation Specialist, FutureTech Innovations). “The use of PowerShell for folder management not only simplifies tasks but also improves automation workflows. Implementing a script that checks for folder existence before creation can significantly reduce redundancy and streamline operations in larger environments.”
Frequently Asked Questions (FAQs)
How can I create a folder in PowerShell if it does not exist?
You can use the `New-Item` cmdlet with the `-ItemType Directory` parameter. The command is: `New-Item -Path “C:\Path\To\Folder” -ItemType Directory -Force`. The `-Force` parameter will ensure that no error is thrown if the folder already exists.
What is the command to check if a folder exists in PowerShell?
You can use the `Test-Path` cmdlet to check for the existence of a folder. The command is: `Test-Path “C:\Path\To\Folder”`. It returns `True` if the folder exists and “ otherwise.
Can I create multiple folders at once using PowerShell?
Yes, you can create multiple folders by using a loop or by specifying an array of folder paths. For example:
“`powershell
$folders = “C:\Path\To\Folder1”, “C:\Path\To\Folder2”
foreach ($folder in $folders) {
New-Item -Path $folder -ItemType Directory -Force
}
“`
Is there a way to create a folder with a timestamp in its name using PowerShell?
Yes, you can include a timestamp in the folder name by using the `Get-Date` cmdlet. For example:
“`powershell
$timestamp = Get-Date -Format “yyyyMMdd_HHmmss”
New-Item -Path “C:\Path\To\Folder_$timestamp” -ItemType Directory -Force
“`
What happens if I try to create a folder that already exists in PowerShell?
If you use the `-Force` parameter with the `New-Item` cmdlet, PowerShell will not throw an error if the folder already exists. Without `-Force`, an error will be generated indicating that the folder already exists.
Can I create a folder in a remote location using PowerShell?
Yes, you can create a folder in a remote location using PowerShell’s `Invoke-Command` cmdlet. For example:
“`powershell
Invoke-Command -ComputerName “RemotePC” -ScriptBlock { New-Item -Path “C:\Path\To\Folder” -ItemType Directory -Force }
“`
Ensure you
In summary, the process of creating a folder using PowerShell if it does not already exist is straightforward and efficient. The cmdlet `New-Item` can be utilized in conjunction with the `-Force` parameter to ensure that the folder is created without errors, even if it already exists. This approach minimizes unnecessary interruptions in scripts and automates the folder creation process seamlessly.
Additionally, using the `Test-Path` cmdlet can enhance the script’s functionality by checking for the existence of the folder before attempting to create it. This method provides a clear logical flow, allowing for better error handling and resource management. By implementing these techniques, users can maintain organized file systems and streamline their workflows effectively.
Overall, leveraging PowerShell for folder management not only simplifies tasks but also empowers users to automate repetitive actions, ultimately saving time and reducing the likelihood of human error. Mastery of these commands can significantly improve productivity and efficiency in various IT and administrative tasks.
Author Profile
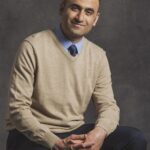
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?