How Can I Read a File Line by Line in PowerShell?
In the world of automation and system administration, PowerShell stands out as a powerful tool for managing and manipulating data. One common task that many administrators and developers encounter is reading files, especially when dealing with large datasets or configuration files. The ability to read a file line by line can be invaluable, allowing for efficient processing and analysis of information without overwhelming the system’s memory. Whether you’re looking to extract specific data, perform batch modifications, or simply analyze logs, mastering this technique can significantly enhance your PowerShell skills.
Reading a file line by line in PowerShell is not just about accessing the content; it’s about leveraging the flexibility and efficiency of the language to handle data dynamically. PowerShell provides several cmdlets and methods that allow users to iterate through files seamlessly, making it easy to implement custom logic for each line. This approach is particularly useful when dealing with structured data formats, where each line may represent a distinct record or command.
As you delve deeper into the intricacies of reading files in PowerShell, you’ll discover various techniques and best practices that can streamline your workflows. From using simple loops to employing advanced filtering and processing methods, you’ll learn how to harness the full potential of PowerShell to manipulate text files effectively. Whether you’re a seasoned scripter or just starting out, understanding how
Reading Files Line by Line in PowerShell
PowerShell provides a straightforward approach to read files line by line, which can be particularly useful for processing large files or extracting specific information from text files. The `Get-Content` cmdlet is the primary tool for this purpose.
To read a file line by line, the command structure is as follows:
“`powershell
Get-Content -Path “C:\Path\To\Your\File.txt”
“`
This command retrieves the contents of the specified file and outputs it to the console, displaying each line sequentially. However, for more advanced scenarios, such as filtering or processing each line, you can leverage the pipeline feature of PowerShell.
Using the Pipeline for Processing
When you want to perform operations on each line of the file, you can pipe the output of `Get-Content` to other cmdlets. Here are some examples:
- Filtering Lines: To read only lines that contain a specific keyword:
“`powershell
Get-Content -Path “C:\Path\To\Your\File.txt” | Where-Object { $_ -match “keyword” }
“`
- Counting Lines: To count the total number of lines in a file:
“`powershell
(Get-Content -Path “C:\Path\To\Your\File.txt”).Count
“`
- Processing Lines: To transform or manipulate each line, you can use a loop:
“`powershell
Get-Content -Path “C:\Path\To\Your\File.txt” | ForEach-Object {
Process each line here
Write-Host “Processing line: $_”
}
“`
Performance Considerations
When working with large files, it’s essential to consider the performance implications. By default, `Get-Content` loads the entire file into memory, which can lead to high memory usage. To mitigate this, you can use the `-ReadCount` parameter to limit the number of lines read into memory at once.
- Example of Using -ReadCount:
“`powershell
Get-Content -Path “C:\Path\To\Your\File.txt” -ReadCount 100 | ForEach-Object {
Process lines in batches
}
“`
This method allows you to handle larger files more efficiently by processing them in smaller chunks.
Advanced Techniques
For more complex scenarios, such as reading files with specific encoding or handling errors, additional parameters can be utilized:
- Specifying Encoding:
“`powershell
Get-Content -Path “C:\Path\To\Your\File.txt” -Encoding UTF8
“`
- Error Handling: To handle cases where the file may not exist:
“`powershell
try {
Get-Content -Path “C:\Path\To\Your\File.txt”
} catch {
Write-Host “Error: File not found.”
}
“`
Table of Common Cmdlets for File Reading
Cmdlet | Description |
---|---|
Get-Content | Reads the content of a file line by line. |
Where-Object | Filters objects based on specified criteria. |
ForEach-Object | Processes each item in a collection. |
Measure-Object | Performs calculations on property values of objects. |
Reading Files Line by Line in PowerShell
To read a file line by line in PowerShell, you can utilize several methods. Below are some of the most common approaches:
Using Get-Content
The `Get-Content` cmdlet is the most straightforward way to read a file line by line. This cmdlet retrieves the content of the file and outputs it as an array of lines.
“`powershell
$lines = Get-Content -Path “C:\path\to\your\file.txt”
foreach ($line in $lines) {
Write-Host $line
}
“`
- Parameters:
- `-Path`: Specify the path of the file you want to read.
This method reads the entire file into memory, which is suitable for smaller files.
Using StreamReader for Large Files
For larger files, using `System.IO.StreamReader` is more efficient as it reads the file line by line without loading the entire content into memory.
“`powershell
$reader = [System.IO.StreamReader]::new(“C:\path\to\your\file.txt”)
while (-not $reader.EndOfStream) {
$line = $reader.ReadLine()
Write-Host $line
}
$reader.Close()
“`
- Benefits:
- Reduces memory usage.
- Suitable for very large files.
Using ForEach-Object with Get-Content
Another efficient way to read files line by line is by piping the output of `Get-Content` directly into a `ForEach-Object` block.
“`powershell
Get-Content -Path “C:\path\to\your\file.txt” | ForEach-Object {
Write-Host $_
}
“`
- Advantages:
- Streamlined syntax.
- Processes each line as it is read, which is memory efficient.
Reading Specific Lines
If you need to read specific lines from a file, you can combine the `Get-Content` cmdlet with array indexing.
“`powershell
$lines = Get-Content -Path “C:\path\to\your\file.txt”
$specificLines = $lines[0..4] Reads the first five lines
foreach ($line in $specificLines) {
Write-Host $line
}
“`
- Note: PowerShell uses zero-based indexing.
Handling Errors
When working with file operations, it is essential to handle potential errors, such as missing files or access issues. You can use a try-catch block to manage these exceptions.
“`powershell
try {
$lines = Get-Content -Path “C:\path\to\your\file.txt”
foreach ($line in $lines) {
Write-Host $line
}
} catch {
Write-Host “Error reading file: $_”
}
“`
- Try-Catch: This structure allows for graceful error handling, ensuring that your script does not terminate unexpectedly.
By employing these methods, you can efficiently read files line by line in PowerShell, taking into consideration the file size and resource management. Each technique serves a specific purpose, allowing for flexibility based on your requirements.
Expert Insights on Reading Files Line by Line in PowerShell
Maria Chen (Senior Systems Administrator, Tech Solutions Inc.). “Reading files line by line in PowerShell is an efficient way to process large text files without consuming excessive memory. Utilizing the `Get-Content` cmdlet with the `-ReadCount` parameter allows for optimal performance when handling extensive datasets.”
James O’Reilly (PowerShell Expert and Author, Scripting Mastery). “When scripting in PowerShell, the `foreach` loop combined with `Get-Content` is the most straightforward method for line-by-line processing. This approach not only enhances readability but also simplifies error handling during file operations.”
Linda Patel (IT Consultant and PowerShell Trainer, CodeCraft Academy). “For advanced users, employing `Get-Content` with a pipeline can significantly streamline the processing of file contents. This method allows for chaining other cmdlets, enabling powerful data manipulation directly from the command line.”
Frequently Asked Questions (FAQs)
How can I read a file line by line in PowerShell?
You can read a file line by line in PowerShell using the `Get-Content` cmdlet. For example, use `Get-Content -Path “C:\path\to\your\file.txt”` to read the file.
What is the purpose of the `-ReadCount` parameter in `Get-Content`?
The `-ReadCount` parameter specifies the number of lines to read at a time. Setting it to `1` will read the file line by line, while a higher number will read multiple lines in one go.
Can I process each line as I read it in PowerShell?
Yes, you can use a `foreach` loop to process each line as you read it. For example:
“`powershell
foreach ($line in Get-Content -Path “C:\path\to\your\file.txt”) {
Process $line here
}
“`
Is it possible to read a large file without loading it entirely into memory?
Yes, using `Get-Content` with the `-ReadCount` parameter set to a higher number or using `-Tail` can help manage memory usage by reading the file in chunks.
What should I do if the file is too large and takes too long to read?
Consider using the `-Tail` parameter to read only the last few lines of the file, or implement streaming techniques with `Get-Content` to read it in smaller, manageable portions.
Can I filter lines while reading a file in PowerShell?
Yes, you can filter lines using the `Where-Object` cmdlet. For example:
“`powershell
Get-Content -Path “C:\path\to\your\file.txt” | Where-Object { $_ -like “*searchTerm*” }
“`
This command reads the file and filters lines containing “searchTerm”.
In summary, reading a file line by line in PowerShell is a straightforward process that can be accomplished using several methods. The most common approaches include using the `Get-Content` cmdlet, which reads the content of a file into an array of strings, with each string representing a line. This method is efficient for processing large files, as it allows for streaming the content rather than loading the entire file into memory at once.
Another effective technique involves utilizing a `foreach` loop in conjunction with `Get-Content`, enabling users to process each line individually. This approach is particularly useful for applying specific operations or transformations to each line of the file, making it a versatile option for file manipulation tasks. Additionally, using the `-ReadCount` parameter can optimize performance by controlling how many lines are read at a time.
Key takeaways from the discussion include the importance of understanding the various methods available for reading files in PowerShell, as well as the benefits of choosing the right approach based on the size of the file and the desired operations. By leveraging these techniques, users can efficiently manage file contents and automate tasks, ultimately enhancing productivity in their scripting endeavors.
Author Profile
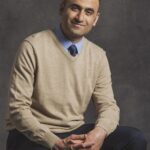
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?