How Can I Run Another PowerShell Script from Within a PowerShell Script?
In the realm of Windows automation and system administration, PowerShell stands out as a powerful tool that streamlines complex tasks and enhances productivity. As users delve deeper into the capabilities of this scripting language, they often encounter scenarios where executing one PowerShell script from another becomes essential. Whether you’re orchestrating a series of automated tasks, managing configurations, or integrating various scripts for a cohesive workflow, understanding how to run a PowerShell script within another can significantly elevate your scripting game.
PowerShell’s versatility allows for seamless script execution, enabling users to harness the power of modular scripting. By calling one script from another, you can create more organized, maintainable, and reusable code. This practice not only simplifies the management of your scripts but also enhances collaboration among team members who may be working on different components of a larger automation project. As we explore the methods and best practices for executing scripts within scripts, you’ll discover how to leverage PowerShell’s capabilities to streamline your workflows.
In this article, we will delve into the various techniques for running PowerShell scripts from within other scripts, examining the nuances of script paths, parameter passing, and error handling. Whether you’re a seasoned PowerShell user or just beginning your journey, mastering this skill will empower you to build more efficient and effective automation solutions
Methods to Run a PowerShell Script from Another PowerShell Script
Running a PowerShell script from another script can be achieved using various methods, each suited for different scenarios. Below are the most common methods to accomplish this task.
Using the Call Operator
The call operator (`&`) allows you to execute another script within your current script’s context. This method is straightforward and preserves the current scope.
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
This command runs `Script.ps1`, and any variables or functions defined in it will be available in the calling script after execution.
Using the Dot Sourcing Method
Dot sourcing is another method that executes a script in the current scope, allowing access to its variables and functions directly.
“`powershell
. “C:\Path\To\Your\Script.ps1”
“`
The space before the path is crucial, as it tells PowerShell to run the script in the current session instead of a new one.
Using the Start-Process Cmdlet
For scenarios where you want to run the script in a new process or with different credentials, `Start-Process` is the preferred method. This method does not share the current session’s variables or functions.
“`powershell
Start-Process -FilePath “powershell.exe” -ArgumentList “-File C:\Path\To\Your\Script.ps1”
“`
This command opens a new PowerShell process to execute the specified script.
Passing Arguments to Scripts
When invoking scripts, you may need to pass arguments. Both the call operator and dot sourcing allow you to do this easily.
“`powershell
& “C:\Path\To\Your\Script.ps1” -Argument1 Value1 -Argument2 Value2
“`
In the receiving script, you can access these arguments using the `$args` automatic variable or by defining parameters:
“`powershell
param (
[string]$Argument1,
[string]$Argument2
)
“`
Handling Script Execution Policies
Before running scripts, ensure that your PowerShell execution policy allows script execution. You can check the current policy using:
“`powershell
Get-ExecutionPolicy
“`
If necessary, change the policy with:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Be cautious when modifying execution policies, as they can affect system security.
Common Errors and Troubleshooting
While executing scripts, you may encounter several common issues. Here’s a summary of potential problems and solutions:
Error | Possible Cause | Solution |
---|---|---|
File Not Found | Incorrect path to the script | Verify the script path is correct |
Execution Policy Error | Script execution policy restrictions | Change the execution policy |
Permission Denied | Lack of required permissions | Run PowerShell as an administrator |
By understanding these methods and considerations, you can effectively run one PowerShell script from another, enhancing your scripting capabilities and automating tasks efficiently.
Executing a PowerShell Script from Another PowerShell Script
To run one PowerShell script from another, you can utilize the `&` operator, which is the call operator in PowerShell. This operator allows you to execute a script or command directly. Below are the steps and examples to achieve this.
Using the Call Operator
The simplest method to execute another PowerShell script is by using the call operator `&`. Here’s how you can do it:
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
In this example, replace `C:\Path\To\Your\Script.ps1` with the actual path to your script.
Passing Parameters
If your script requires parameters, you can pass them after the script path:
“`powershell
& “C:\Path\To\Your\Script.ps1” -Parameter1 “Value1” -Parameter2 “Value2”
“`
Ensure that the parameters are defined in the called script to accept these values.
Using `Start-Process` Cmdlet
Another method to run a script is by using the `Start-Process` cmdlet. This is particularly useful if you want to run the script in a new PowerShell window or need to redirect output.
“`powershell
Start-Process powershell -ArgumentList “-File `C:\Path\To\Your\Script.ps1`”
“`
Benefits of Using `Start-Process`
- Runs the script in a separate process.
- Can specify window styles (hidden, minimized, etc.).
- Useful for long-running scripts.
Running Scripts in the Current Session
If you want the called script to run in the same session and inherit the context (variables, functions), use the call operator as shown earlier.
Important Considerations
- Execution Policy: Ensure that your PowerShell execution policy allows running scripts. You can check it using:
“`powershell
Get-ExecutionPolicy
“`
If needed, you can change it temporarily with:
“`powershell
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope Process
“`
- Script Location: If the script is not in the current directory, always provide the full path.
Script with Error Handling
Incorporating error handling is crucial when executing scripts. You can use `try` and `catch` blocks to manage errors effectively.
“`powershell
try {
& “C:\Path\To\Your\Script.ps1”
} catch {
Write-Host “An error occurred: $_”
}
“`
This ensures that if the called script fails, your main script can handle the exception gracefully.
Conclusion on Script Execution
Executing scripts from other scripts in PowerShell is straightforward using the call operator or `Start-Process`. By understanding how to pass parameters and handle errors, you can create robust automation solutions.
Expert Insights on Running PowerShell Scripts
Jessica Lin (Senior Systems Administrator, Tech Solutions Inc.). “Running another PowerShell script from within a PowerShell session is a straightforward process. Utilizing the `&` call operator allows for executing scripts seamlessly, which is crucial for automating complex tasks in enterprise environments.”
Mark Thompson (PowerShell Automation Specialist, SysAdmin Magazine). “When invoking a secondary PowerShell script, it is essential to consider the execution policy settings. Ensuring that the policy allows for script execution can prevent unnecessary roadblocks during automation workflows.”
Dr. Emily Carter (Cybersecurity Analyst, SecureTech Labs). “Incorporating error handling when running another PowerShell script is vital. Using `try/catch` blocks can help manage exceptions gracefully, allowing for robust and reliable script execution in security-sensitive environments.”
Frequently Asked Questions (FAQs)
How can I run another PowerShell script from within a PowerShell script?
You can run another PowerShell script by using the `&` (call) operator followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What is the difference between using the call operator and dot-sourcing?
The call operator (`&`) runs the script in a separate scope, while dot-sourcing (`. “C:\Path\To\YourScript.ps1″`) runs the script in the current scope, allowing any variables or functions defined in the script to be accessible afterward.
Can I pass parameters to the script I am calling?
Yes, you can pass parameters by including them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What should I do if the script I want to run is in a different directory?
You can specify the full path to the script when using the call operator or dot-sourcing. Alternatively, you can change the current directory using `Set-Location` before executing the script.
Are there any security considerations when running scripts from another script?
Yes, ensure that the scripts you are calling are from trusted sources to avoid executing malicious code. Additionally, consider setting the execution policy appropriately using `Set-ExecutionPolicy`.
Can I run PowerShell scripts in parallel?
Yes, you can run PowerShell scripts in parallel using jobs, runspaces, or the `Start-Job` cmdlet. This allows multiple scripts to execute simultaneously without waiting for each other to finish.
In summary, executing one PowerShell script from another is a straightforward process that enhances the modularity and reusability of scripts. By using the `&` call operator or the `Invoke-Expression` cmdlet, users can seamlessly run external scripts, allowing for more complex automation tasks. This capability not only streamlines workflows but also encourages the organization of scripts into manageable components.
Additionally, leveraging parameters when calling another script can significantly increase the flexibility of the scripts involved. By passing arguments, users can customize the behavior of the invoked script, making it adaptable to various scenarios. This feature is particularly beneficial in environments where scripts need to be reused with different inputs or configurations.
Furthermore, understanding the execution policies in PowerShell is crucial when running scripts. Users must ensure that the appropriate execution policy is set to allow the execution of scripts, which can be managed through the `Set-ExecutionPolicy` cmdlet. This consideration is vital for maintaining security while utilizing the powerful capabilities of PowerShell scripting.
the ability to run another PowerShell script from within a script is a fundamental skill that enhances automation capabilities. By mastering this technique, users can create more efficient and organized scripts that can be easily maintained and adapted
Author Profile
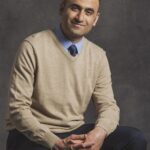
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?