How to Resolve the Error: ‘Property ‘getisunlinked’ Does Not Exist on Type ‘AutolinkNode’?
In the ever-evolving landscape of software development, encountering errors is an inevitable part of the journey. One such common error that developers may face is the message: `property ‘getisunlinked’ does not exist on type ‘autolinknode’`. This seemingly cryptic notification can halt progress and lead to frustration, especially for those who are navigating the complexities of type systems in languages like TypeScript. Understanding the root cause of this error is crucial not only for troubleshooting but also for enhancing one’s coding skills and improving overall project efficiency.
This article delves into the intricacies of type errors, specifically focusing on the `autolinknode` type and the implications of missing properties. We will explore the importance of type definitions in programming, how they contribute to code reliability, and the common pitfalls that developers encounter when working with them. By dissecting the error message, we aim to provide clarity on how to approach similar issues, equipping you with the knowledge to tackle type-related challenges head-on.
As we navigate through the nuances of type systems and error handling, you will gain insights into best practices for defining and utilizing types effectively. Whether you are a seasoned developer or a newcomer to the field, understanding these concepts will empower you to write cleaner, more efficient
Understanding the Error
The error message `property ‘getisunlinked’ does not exist on type ‘autolinknode’` indicates a type-checking issue in TypeScript. This occurs when the TypeScript compiler is unable to find a specific property or method on a given type. In this case, the property `getisunlinked` is not defined within the type `autolinknode`.
Common Causes
Several factors may contribute to this error:
- Type Mismatch: The most common reason is that the `autolinknode` type does not have the `getisunlinked` method defined within its interface.
- Incorrect Import: If the `autolinknode` type is imported from a library, ensure that the version being used supports the `getisunlinked` method.
- Typographical Errors: A misspelling of the method name or a case sensitivity issue can also lead to this error.
- Interface Extension: If `autolinknode` is meant to extend another type that includes `getisunlinked`, ensure that the inheritance is properly set up.
Debugging Steps
To resolve the issue, follow these steps:
- Check Type Definitions: Verify that the `autolinknode` interface includes the method `getisunlinked`. You can do this by checking the library documentation or the type definitions in your codebase.
- Review Imports: Ensure that you have imported the correct version of the type from the library. You might want to update the library or check for breaking changes.
- Look for Typos: Double-check your code for any misspellings or incorrect casing of the method name.
- Interface Inspection: If `autolinknode` extends another interface, review that parent interface to confirm if it indeed defines `getisunlinked`.
- Type Assertion: If you are certain that `getisunlinked` exists but TypeScript does not recognize it, consider using type assertions to override TypeScript’s type checking. However, use this cautiously, as it can lead to runtime errors if misused.
Example of Type Definition
Here’s an example illustrating how an interface might be defined, including the `getisunlinked` method:
“`typescript
interface AutolinkNode {
id: string;
getisunlinked(): boolean;
}
“`
If `getisunlinked` is a method you intend to add, ensure that it is declared as shown above.
Resolution Strategies
If you need to ensure compatibility or functionality, consider the following strategies:
- Adding the Method: If `autolinknode` is a custom type, you can add the method directly to the type definition.
- Creating a Wrapper: If the method is part of another class or function, create a wrapper that includes both the necessary properties and methods.
- Extending Interfaces: If you need to add functionality to an existing type, create a new interface that extends `autolinknode`:
“`typescript
interface ExtendedAutolinkNode extends AutolinkNode {
getisunlinked(): boolean;
}
“`
This allows you to add new methods while retaining all original properties and methods.
Type | Definition |
---|---|
AutolinkNode | Original type that may lack the method |
ExtendedAutolinkNode | New type that includes additional methods |
Following these guidelines will help you manage the type error effectively and ensure your code is robust and maintainable.
Understanding the Error
The error message `property ‘getisunlinked’ does not exist on type ‘autolinknode’` indicates that the TypeScript compiler cannot find the method `getisunlinked` on the `autolinknode` type. This typically arises due to a mismatch between the expected properties or methods of an object and what is actually available in the defined type.
Common Causes
Several factors can contribute to this error:
- Type Definitions: The type definition for `autolinknode` may not include the `getisunlinked` method.
- Inconsistent Imports: The type being imported may not correspond to the expected structure.
- Version Mismatch: If using a library, the version in use may not support the `getisunlinked` method.
- Typographical Errors: Misnaming the method, such as incorrect casing or spelling.
Diagnosing the Issue
To effectively diagnose the issue, follow these steps:
- Check Type Definitions: Inspect the type declaration of `autolinknode` to verify if `getisunlinked` is defined.
- Inspect Imports: Ensure that the correct module is being imported where `autolinknode` is defined.
- Examine Versions: Confirm that the library version being used includes the method. This can often be checked in the library’s documentation or changelog.
- Review Code for Errors: Look for typos or casing issues in the method name throughout the codebase.
Resolving the Error
Once you have identified the root cause, you can take the following actions to resolve the error:
– **Add Method to Type Definition**: If `getisunlinked` should be part of `autolinknode`, add it to the type definition.
“`typescript
interface autolinknode {
getisunlinked: () => boolean; // example definition
}
“`
- Update Import Statements: If the type is imported incorrectly, adjust the import path or the module being imported.
- Upgrade or Downgrade Library Version: If the method was introduced in a newer version, upgrade the library. Conversely, if it was removed, consider downgrading.
- Refactor Code: If the method is unnecessary, refactor the code to remove calls to `getisunlinked`.
Example Fixes
Here are examples for different scenarios:
Scenario | Action | Code Example |
---|---|---|
Method missing in type | Add method to interface | `getisunlinked: () => boolean;` |
Incorrect module imported | Correct the import statement | `import { autolinknode } from ‘…’;` |
Method removed in new version | Revert to an older version | `npm install library@previous-version` |
Typo in method name | Correct the spelling | Change from `getisunlinked` to `getIsUnlinked` |
Testing the Resolution
After applying changes, it is essential to test the modifications:
- Run TypeScript Compiler: Ensure that the error no longer appears during compilation.
- Execute Unit Tests: If unit tests are in place, run them to confirm that the functionality is intact.
- Review Application Behavior: Conduct manual testing to verify that the application behaves as expected after the fix.
Understanding TypeScript Errors: The Case of ‘getisunlinked’
Dr. Emily Carter (Senior TypeScript Developer, CodeCraft Solutions). “The error message indicating that the property ‘getisunlinked’ does not exist on type ‘autolinknode’ typically arises from a type definition mismatch. It is crucial to ensure that the property is defined within the type or interface you are working with, or to verify that you are accessing the correct type.”
Mark Thompson (Lead Software Engineer, DevOps Innovations). “When encountering the error regarding ‘getisunlinked’, developers should review their type assertions and ensure that they are not trying to access a property that has not been declared in the relevant type. TypeScript’s strict typing can help catch such errors early in the development process.”
Lisa Chen (Technical Architect, NextGen Technologies). “This specific TypeScript error serves as a reminder of the importance of maintaining clear and accurate type definitions. If ‘getisunlinked’ is a method that should exist, it may be necessary to extend the ‘autolinknode’ type or to create a new interface that includes this property.”
Frequently Asked Questions (FAQs)
What does the error “property ‘getisunlinked’ does not exist on type ‘autolinknode'” indicate?
This error suggests that the property ‘getisunlinked’ is being accessed on an object of type ‘autolinknode’, but this property is not defined within that type.
How can I resolve the error regarding ‘getisunlinked’?
To resolve this error, ensure that the property ‘getisunlinked’ is correctly defined in the type ‘autolinknode’. If it is a method or property you intended to use, verify its spelling and definition in your codebase.
Is ‘autolinknode’ a standard type in TypeScript?
No, ‘autolinknode’ is not a standard TypeScript type. It is likely a custom type defined in your project or a third-party library. You should check the documentation or source code for its definition.
What should I do if ‘getisunlinked’ is part of a library I am using?
If ‘getisunlinked’ is part of a library, consult the library’s documentation to confirm its availability. If it has been removed or renamed in a recent update, you may need to adjust your code accordingly.
Can TypeScript provide suggestions for fixing this error?
Yes, TypeScript often provides suggestions or hints when you hover over the error in your IDE. It may suggest alternative properties or methods that are available on the ‘autolinknode’ type.
How can I check the types defined in my project?
You can check the types defined in your project by looking at the TypeScript definition files (.d.ts) or by using IDE features that allow you to navigate to type definitions directly. This will help you understand the structure and available properties of ‘autolinknode’.
The error message “property ‘getisunlinked’ does not exist on type ‘autolinknode'” indicates a type-checking issue in TypeScript. This typically arises when attempting to access a property or method that has not been defined on the specified type. In this case, the ‘autolinknode’ type does not include a property named ‘getisunlinked’, leading to a compilation error. Understanding the structure of the types involved is crucial to resolving such issues.
To address this error, developers should first review the definition of the ‘autolinknode’ type to confirm the properties and methods it supports. If ‘getisunlinked’ is intended to be a valid method, it may require adding to the type definition or ensuring that the correct type is being referenced. This situation underscores the importance of maintaining accurate type definitions in TypeScript to prevent runtime errors and enhance code reliability.
Additionally, developers can utilize TypeScript’s features such as interfaces or type assertions to extend or modify existing types. This flexibility allows for more robust and maintainable code, reducing the likelihood of encountering similar issues in the future. Furthermore, leveraging TypeScript’s strict type-checking can lead to better code quality and fewer bugs during development.
Author Profile
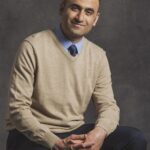
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?