How to Navigate and Retrieve Values from Nested Data Structures in Python?
In the world of programming, data structures serve as the backbone of efficient information storage and retrieval. Among the various types of data structures, nested data structures stand out for their ability to encapsulate complex relationships and hierarchies. Whether you’re working with JSON objects, dictionaries, or lists in Python, understanding how to navigate these intricate arrangements is essential for any developer. In this article, we will delve into the art of recording, pathing, and retrieving values from nested data structures, empowering you to manipulate and access data with finesse.
Navigating nested data structures in Python can initially seem daunting due to their layered complexity. These structures allow you to store data in a way that reflects real-world relationships, making them invaluable for tasks ranging from data analysis to web development. However, the challenge lies in efficiently accessing specific values buried within these layers. By mastering the techniques for traversing these structures, you can unlock a wealth of information and streamline your data handling processes.
In this exploration, we will cover the fundamental concepts of nested data structures, including how to define them and the various methods available for traversing through their layers. We’ll also discuss the importance of understanding the paths to specific values, which can significantly enhance your coding efficiency and accuracy. Whether you’re a seasoned programmer or just starting your
Understanding Nested Data Structures in Python
Nested data structures in Python allow for the organization of complex data in a hierarchical format. These structures can include lists, dictionaries, tuples, and sets, each capable of containing other nested structures. This flexibility makes Python particularly suitable for handling data that is inherently structured, such as JSON or XML.
To effectively retrieve values from nested data structures, one must understand how to navigate through these layers. Typically, nested data structures are accessed using a combination of indexing and keys.
Accessing Values in Nested Data Structures
When working with nested dictionaries and lists, the access methods differ slightly. Here’s how to retrieve values from both types:
- Dictionaries: Use the key associated with the value you want to retrieve. For nested dictionaries, use multiple keys.
- Lists: Use integer indexing to access elements. For nested lists, combine multiple indices.
For example, consider the following nested data structure:
“`python
data = {
“user”: {
“name”: “Alice”,
“age”: 30,
“contacts”: {
“email”: “[email protected]”,
“phone”: [“123-456-7890”, “987-654-3210”]
}
}
}
“`
To access Alice’s email, you would use:
“`python
email = data[“user”][“contacts”][“email”]
“`
To access the first phone number, use:
“`python
phone_number = data[“user”][“contacts”][“phone”][0]
“`
Path Retrieval for Nested Structures
When dealing with deeply nested structures, constructing a path can help simplify the retrieval process. A path is essentially a sequence of keys and indices that lead to the desired value.
Consider the following path structure:
Path | Description |
---|---|
`[“user”, “contacts”, “email”]` | Directly retrieves the email. |
`[“user”, “contacts”, “phone”, 0]` | Retrieves the first phone number. |
To implement path retrieval programmatically, a function can be created that navigates through the nested structure based on a provided path:
“`python
def retrieve_value(data, path):
for key in path:
data = data[key]
return data
email = retrieve_value(data, [“user”, “contacts”, “email”])
phone_number = retrieve_value(data, [“user”, “contacts”, “phone”, 0])
“`
This function iterates through each element in the path, updating the `data` variable at each step until it reaches the desired value.
Best Practices for Working with Nested Data Structures
When dealing with nested data structures, consider the following best practices:
- Keep Structure Simple: Overly complex nested structures can lead to difficult-to-maintain code. Strive for clarity.
- Error Handling: Implement error handling to manage potential KeyErrors or IndexErrors when accessing values.
- Document Your Data: Maintain documentation on the structure of your data. This aids in understanding the hierarchy and accessing values appropriately.
- Use Libraries: Consider using libraries such as `json` for parsing JSON data or `pandas` for handling tabular data with nested structures.
Implementing these practices not only improves code readability but also enhances maintainability and usability.
Navigating Nested Data Structures in Python
In Python, nested data structures commonly include lists, dictionaries, or combinations thereof. These structures can be complex, requiring specific methods to navigate and retrieve values efficiently.
Common Nested Data Structures
- Lists of Dictionaries: A list containing multiple dictionaries, often representing a collection of records.
- Dictionaries of Lists: A dictionary where each key maps to a list of values, suitable for grouping data.
- Nested Dictionaries: A dictionary that contains other dictionaries as values, allowing hierarchical data representation.
Accessing Values in Nested Structures
To retrieve values from nested data structures, you can use indexing and key references. Below are examples demonstrating how to access data:
“`python
Example of a list of dictionaries
data = [
{‘name’: ‘Alice’, ‘age’: 30, ‘address’: {‘city’: ‘New York’, ‘zip’: ‘10001’}},
{‘name’: ‘Bob’, ‘age’: 25, ‘address’: {‘city’: ‘Los Angeles’, ‘zip’: ‘90001’}}
]
Accessing values
alice_city = data[0][‘address’][‘city’] Outputs ‘New York’
bob_age = data[1][‘age’] Outputs 25
“`
Function to Retrieve Values
You can create a recursive function to retrieve values from nested structures based on a specified path. This path can be a list of keys or indices that defines how to navigate through the structure.
“`python
def retrieve_value(data, path):
for key in path:
data = data[key]
return data
Example usage
path_to_alice_city = [0, ‘address’, ‘city’]
alice_city = retrieve_value(data, path_to_alice_city) Outputs ‘New York’
“`
Handling Missing Keys or Indices
When accessing values, it is crucial to handle potential missing keys or indices gracefully. You can use the `get` method for dictionaries or a try-except block for lists.
“`python
def safe_retrieve(data, path):
try:
for key in path:
data = data[key]
return data
except (KeyError, IndexError):
return None or an alternative value
Example usage
missing_value = safe_retrieve(data, [0, ‘address’, ‘state’]) Outputs None
“`
Example of Complex Nested Structure
Here is an example illustrating a more complex nested data structure and how to navigate it:
“`python
complex_data = {
’employees’: [
{‘name’: ‘Alice’, ‘details’: {‘age’: 30, ‘skills’: [‘Python’, ‘Django’]}},
{‘name’: ‘Bob’, ‘details’: {‘age’: 25, ‘skills’: [‘JavaScript’, ‘React’]}}
]
}
Retrieve Bob’s skills
bobs_skills = complex_data[’employees’][1][‘details’][‘skills’] Outputs [‘JavaScript’, ‘React’]
“`
Best Practices for Working with Nested Data Structures
- Use Descriptive Names: Ensure your variable names and keys are descriptive to enhance readability.
- Implement Error Handling: Always consider the possibility of missing data and handle exceptions appropriately.
- Consider Using Libraries: For very complex data structures, consider using libraries like `pandas` for data manipulation or `json` for structured data handling.
By applying these methods and practices, you can efficiently navigate and retrieve data from nested structures in Python.
Expert Insights on Navigating Nested Data Structures in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When dealing with nested data structures in Python, it is crucial to understand the hierarchy of the data. Utilizing libraries such as `json` or `pandas` can significantly simplify the process of accessing and retrieving values, allowing for efficient data manipulation and analysis.”
Michael Chen (Software Architect, CodeCraft Solutions). “To effectively retrieve values from nested data structures, employing recursive functions can be a powerful approach. This method allows you to traverse complex structures without losing sight of the data’s organization, ensuring that you can access the desired values seamlessly.”
Sarah Thompson (Senior Python Developer, DevMasters). “It’s essential to leverage dictionary methods and list comprehensions when working with nested data in Python. These tools not only enhance code readability but also improve performance when searching for and retrieving values within deeply nested structures.”
Frequently Asked Questions (FAQs)
What are nested data structures in Python?
Nested data structures in Python refer to data types that contain other data types within them, such as lists, dictionaries, or tuples. For example, a list can contain dictionaries, and a dictionary can contain lists, allowing for complex data organization.
How can I access a value in a nested dictionary?
To access a value in a nested dictionary, use a series of keys corresponding to the hierarchy of the dictionary. For example, if you have `data = {‘key1’: {‘key2’: ‘value’}}`, you can retrieve the value using `data[‘key1’][‘key2’]`.
What is the best way to retrieve a value from a nested list?
To retrieve a value from a nested list, use index notation for each level of the list. For instance, if you have `data = [[1, 2], [3, 4]]`, you can access the value `4` with `data[1][1]`.
How can I safely retrieve a value from a nested data structure without causing errors?
To safely retrieve a value, use the `get()` method for dictionaries, which returns `None` if the key does not exist, or use try-except blocks to handle potential `IndexError` or `KeyError` exceptions when accessing lists or dictionaries.
What libraries can assist with managing nested data structures in Python?
Libraries such as `pandas` and `json` can assist with managing nested data structures. `pandas` provides data manipulation capabilities, while `json` allows for easy parsing and manipulation of JSON-like nested structures.
Can I modify values in a nested data structure in Python?
Yes, you can modify values in a nested data structure by accessing the specific key or index and assigning a new value. For example, to change a value in a nested dictionary, use `data[‘key1’][‘key2’] = ‘new_value’`.
In Python, nested data structures such as dictionaries and lists are commonly used to store complex data records. These structures allow for the organization of data in a hierarchical manner, enabling the representation of relationships between different data points. To retrieve values from these nested structures, one must understand the paths that lead to the desired data, which typically involves indexing and key access. This process can be straightforward for simple structures but may require more intricate navigation for deeply nested ones.
When working with nested data structures, it is essential to adopt a systematic approach for value retrieval. Utilizing loops, recursion, or built-in functions can facilitate the extraction of data from these structures. Additionally, leveraging Python’s powerful libraries, such as `json` for parsing JSON data or `pandas` for handling tabular data, can significantly streamline the process. Understanding how to traverse these structures effectively is crucial for data manipulation and analysis in various applications.
Key takeaways include the importance of mastering the syntax for accessing nested data, as well as the utility of Python’s built-in functions and libraries that can simplify the task. Furthermore, developers should be aware of the potential complexities that arise with deeply nested structures and plan their data access strategies accordingly. By honing these skills, one can enhance
Author Profile
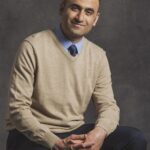
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?