How Can I Use Python’s urllib to Make Requests with Custom Headers?
In the ever-evolving world of web development and data retrieval, Python has emerged as a powerhouse language, offering a myriad of libraries and tools to streamline the process. One such tool, `urllib`, stands out for its simplicity and effectiveness when it comes to handling URLs and making HTTP requests. But what happens when you need to send specific information along with your requests, such as authentication tokens or content types? This is where the ability to include custom headers becomes essential. In this article, we will delve into the nuances of using Python’s `urllib` to make requests that are not only functional but also tailored to meet the demands of modern web APIs.
Understanding how to manipulate headers in your HTTP requests can significantly enhance your interaction with web services. Headers are crucial for conveying metadata about the request or response, such as the type of content being sent or received, authentication credentials, and more. With `urllib`, you can easily customize these headers to ensure that your requests are recognized and processed correctly by the server. This capability opens up a world of possibilities for developers looking to integrate with various APIs or scrape data from websites that require specific header information.
As we explore the intricacies of using `urllib` for making requests with custom headers, we will cover essential concepts, practical examples, and
Using Headers with urllib.request
When making HTTP requests using the `urllib.request` module in Python, it is often necessary to include headers to provide additional information to the server. Headers can specify the type of content being sent, the type of content expected in response, authentication tokens, and more. Here’s how to include headers in your requests.
To include headers in a request, you can use the `Request` object from the `urllib.request` module. This object allows you to specify not only the URL and data but also any headers you want to include.
Here’s an example of how to create a request with custom headers:
“`python
import urllib.request
url = ‘http://example.com/api/resource’
headers = {
‘User-Agent’: ‘Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3’,
‘Authorization’: ‘Bearer your_token_here’,
‘Accept’: ‘application/json’,
}
request = urllib.request.Request(url, headers=headers)
Sending the request and getting the response
with urllib.request.urlopen(request) as response:
response_data = response.read()
print(response_data)
“`
In this code snippet:
- The `headers` dictionary contains key-value pairs that represent the header names and their respective values.
- The `Request` object is initialized with the target URL and the headers.
- The request is sent using `urlopen`, and the response is read and printed.
Common HTTP Headers
When working with headers, certain ones are frequently used. Here is a brief overview:
Header Name | Description |
---|---|
`User-Agent` | Identifies the client software making the request. |
`Authorization` | Contains credentials for authenticating the client. |
`Accept` | Specifies the media types that are acceptable for the response. |
`Content-Type` | Indicates the media type of the resource being sent. |
`Cache-Control` | Directives for caching mechanisms in both requests and responses. |
Handling Different Content Types
When sending data to a server, the `Content-Type` header is particularly important. It tells the server how to interpret the data being sent. Common content types include:
- `application/json`: Used when sending JSON data.
- `application/x-www-form-urlencoded`: The default content type for form submissions.
- `multipart/form-data`: Used for file uploads.
To send JSON data, you can modify the request as follows:
“`python
import json
data = json.dumps({‘key’: ‘value’}).encode(‘utf-8’)
headers[‘Content-Type’] = ‘application/json’
request = urllib.request.Request(url, data=data, headers=headers)
“`
In this example:
- The data is serialized to JSON and encoded as bytes.
- The `Content-Type` is set to `application/json` to inform the server of the data format.
By incorporating headers effectively, you can ensure that your HTTP requests are more informative and compatible with various APIs and web services.
Using `urllib.request` to Make HTTP Requests with Headers
In Python, the `urllib` module provides a powerful way to handle HTTP requests, including the ability to specify custom headers. Headers can be crucial for tasks such as API authentication, content negotiation, and more. Below is a guide on how to effectively use `urllib.request` with custom headers.
Setting Up Your Environment
Before you begin, ensure you have Python installed. The `urllib` module is part of the standard library, so no additional installation is required. You can use any text editor or IDE to write your Python scripts.
Basic Structure for HTTP Requests with Headers
To make a request with custom headers, follow this basic structure:
“`python
import urllib.request
url = ‘https://api.example.com/data’
headers = {
‘User-Agent’: ‘Mozilla/5.0’,
‘Authorization’: ‘Bearer YOUR_ACCESS_TOKEN’,
‘Accept’: ‘application/json’
}
request = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(request)
Read the response
data = response.read()
print(data)
“`
Key Components Explained
- Importing the Module: The `urllib.request` module is imported to handle the HTTP requests.
- URL: This is the endpoint you want to reach.
- Headers Dictionary: Here, you define the headers you want to include in your request. Common headers include:
- `User-Agent`: Identifies the client software making the request.
- `Authorization`: Often used for API authentication.
- `Accept`: Specifies the media types that are acceptable for the response.
Handling Different Request Methods
While the example above demonstrates a GET request, you can also make POST requests using `urllib.request`. Here’s how to do that:
“`python
import urllib.request
import json
url = ‘https://api.example.com/data’
headers = {
‘Content-Type’: ‘application/json’,
‘Authorization’: ‘Bearer YOUR_ACCESS_TOKEN’
}
data = json.dumps({‘key’: ‘value’}).encode(‘utf-8′) Payload for POST
request = urllib.request.Request(url, data=data, headers=headers, method=’POST’)
response = urllib.request.urlopen(request)
Read the response
response_data = response.read()
print(response_data)
“`
In this example:
- Data Payload: The data to be sent with the request is serialized into JSON format and encoded to bytes.
- Method Parameter: Specifying `method=’POST’` indicates that this is a POST request.
Error Handling
It’s important to handle potential errors during your HTTP requests. You can use a try-except block to catch exceptions:
“`python
try:
response = urllib.request.urlopen(request)
data = response.read()
except urllib.error.HTTPError as e:
print(f’HTTP error: {e.code} – {e.reason}’)
except urllib.error.URLError as e:
print(f’URL error: {e.reason}’)
“`
- HTTPError: Catches errors related to the HTTP protocol (e.g., 404 Not Found).
- URLError: Catches errors related to the URL itself (e.g., network issues).
Using `urllib.request` to send HTTP requests with custom headers is straightforward. By following the examples provided, you can effectively interact with web APIs and handle various scenarios such as GET and POST requests, along with error handling mechanisms. This allows for robust application development in Python that can leverage web services effectively.
Expert Insights on Using Python’s urllib with Custom Headers
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When working with Python’s urllib, setting custom headers is crucial for interacting with APIs that require authentication or specific content types. Utilizing the `Request` class allows developers to easily add headers, ensuring that the requests are processed correctly by the server.”
Mark Thompson (Lead Developer, Open Source Projects). “Incorporating headers in urllib requests is essential for maintaining security and functionality. For instance, when sending a JSON payload, it is important to set the ‘Content-Type’ header to ‘application/json’ to inform the server about the data format being sent.”
Linda Nguyen (Python Developer Advocate, CodeCraft). “Understanding how to manipulate headers in urllib requests can significantly enhance the performance of your applications. By customizing headers, you can manage caching, control content delivery, and improve user experience by ensuring that the right data is sent and received.”
Frequently Asked Questions (FAQs)
How do I make a GET request with headers using Python’s urllib?
To make a GET request with headers using Python’s urllib, you can utilize `urllib.request.Request` to create a request object with your desired headers, then use `urllib.request.urlopen()` to send the request.
Can I add multiple headers to my request in Python’s urllib?
Yes, you can add multiple headers by including them in a dictionary format when creating the request object. Each key-value pair in the dictionary represents a header name and its corresponding value.
What is the correct way to set the User-Agent header in a urllib request?
You can set the User-Agent header by including it in the headers dictionary when creating the request object. For example: `headers = {‘User-Agent’: ‘Your User Agent’}`.
How do I handle exceptions when making requests with urllib in Python?
You can handle exceptions by wrapping your request code in a try-except block. Catch specific exceptions like `urllib.error.HTTPError` and `urllib.error.URLError` to manage errors effectively.
Is it possible to send a POST request with headers using Python’s urllib?
Yes, you can send a POST request by using `urllib.request.Request` with the method set to ‘POST’ and including the data you want to send, along with your headers.
What libraries can I use as alternatives to urllib for making HTTP requests in Python?
Alternatives to urllib include the `requests` library, which offers a simpler and more flexible API for making HTTP requests with headers and handling responses.
In summary, utilizing the Python `urllib` library for making HTTP requests with custom headers is a straightforward process that enhances the flexibility and functionality of web interactions. The `urllib.request` module allows developers to create requests that can include various headers, such as User-Agent, Authorization, and Content-Type, which are essential for interacting with APIs and web servers that require specific header information for proper request handling.
One of the key takeaways is the importance of understanding how to construct and send requests with headers. By using the `Request` class from `urllib.request`, developers can easily set headers by passing a dictionary of key-value pairs. This capability is crucial for ensuring that the server correctly interprets the request and responds appropriately, especially when dealing with RESTful APIs that often require authentication and specific content types.
Additionally, error handling and response management are vital components of working with `urllib`. Developers should implement robust error handling to manage potential exceptions that may arise during the request process. Furthermore, examining the response headers and status codes can provide valuable insights into the success of the request and the nature of the returned data.
mastering the use of `urllib.request` with headers in Python is an essential skill for developers looking
Author Profile
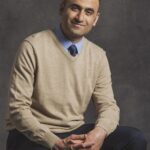
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?