How Can I View the Code of a Function in R?
In the world of programming, understanding the inner workings of functions is crucial for mastering any language, and R is no exception. Whether you’re a novice looking to grasp the basics or an experienced coder seeking to optimize your scripts, knowing how to see the code of a function can unlock a treasure trove of insights. This powerful skill not only enhances your ability to troubleshoot and refine your code but also deepens your comprehension of the language itself. Join us as we explore the various methods to access and analyze function code in R, empowering you to become a more proficient and confident programmer.
When you encounter a function in R, it often comes with a layer of abstraction that can make it challenging to understand exactly what’s happening behind the scenes. Fortunately, R provides several tools that allow you to peek beneath that surface. By examining the source code of built-in functions or user-defined ones, you can gain valuable knowledge about their implementation and behavior. This exploration can lead to improved coding practices, better debugging skills, and a more intuitive grasp of R’s capabilities.
In this article, we will guide you through the essential techniques for accessing function code in R. From utilizing built-in commands to leveraging R’s rich ecosystem of packages, you’ll learn how to demystify functions and enhance
Viewing Function Code in R
In R, you can easily view the code of any function by using several methods. This is particularly useful for understanding how functions work, debugging, or learning from existing code. Here are the primary ways to see the code of a function:
- Using the `get` function: This method retrieves the function object and prints its code.
“`R
get(“function_name”)
“`
- Simply typing the function name: If you enter the name of a function without parentheses, R will display its code in the console.
“`R
function_name
“`
- Using the `body` function: This extracts the body of the function, providing a concise view of its content.
“`R
body(function_name)
“`
- Utilizing the `str` function: This function gives you a structured overview of the function, including its arguments.
“`R
str(function_name)
“`
Accessing Package Functions
When dealing with functions from packages, you can specify the package name to avoid confusion with similarly named functions from other packages. For instance, to view a function from the `dplyr` package, you would use:
“`R
dplyr::function_name
“`
This ensures that you are accessing the correct function, especially in environments where multiple packages may have overlapping function names.
Example Function Code
Here’s an example of how to view the code of the built-in `lm` function in R, which is used for linear modeling:
“`R
lm
“`
Alternatively, you can use:
“`R
get(“lm”)
“`
Both commands will display the code for the `lm` function in the R console.
Understanding Function Structure
R functions typically have a specific structure, which can be summarized as follows:
- Arguments: Inputs the function accepts.
- Body: The core logic of the function that defines its behavior.
- Return Value: The output produced by the function after executing its body.
You can visualize the structure using a simple table:
Component | Description |
---|---|
Arguments | Inputs defined in the function header. |
Body | The set of operations performed on the inputs. |
Return Value | The result returned by the function. |
By understanding these components, you can better interpret the functions you encounter in R.
Additional Resources
For those looking to delve deeper into R programming, consider exploring the following resources:
- R Documentation: Access comprehensive guides and manuals directly from R’s help system using the `?function_name` syntax.
- Online Tutorials: Websites such as R-bloggers and DataCamp offer extensive tutorials and courses.
- Books: Literature such as “R for Data Science” can provide practical insights and examples.
Utilizing these methods and resources will enhance your ability to understand and leverage R functions effectively.
Methods to View Function Code in R
In R, there are several methods to view the code of a function, which can be particularly useful for understanding how functions work or for debugging purposes. Below are the most common approaches:
Using the `print` or `cat` Functions
You can directly print the function to the console to see its code. This is the simplest method for quickly viewing a function’s definition.
“`R
my_function <- function(x) {
return(x^2)
}
print(my_function)
```
This will output the body of `my_function`, providing a straightforward look at its code.
Using the `body` Function
The `body` function extracts the body of a function, which can be useful if you want to see just the code without additional information.
“`R
body(my_function)
“`
This will return the internal code of `my_function` without any other metadata.
Using the `deparse` Function
The `deparse` function converts the function body into a character vector, allowing you to see the code line-by-line.
“`R
deparse(my_function)
“`
The output will display each line of the function as a separate string element in a vector.
Using the `str` Function
The `str` function can provide a structured view of the function, showing its components and attributes.
“`R
str(my_function)
“`
This gives insight into the function’s structure and can help identify arguments and other attributes.
Accessing Source Code for Built-in Functions
For built-in functions, you can also use the `get` function to retrieve the function object before printing it. This is particularly useful for functions that may be masked by other packages.
“`R
get(“mean”)
“`
You can then apply any of the methods above to view its code.
Viewing Code in Packages
When dealing with functions from loaded packages, you can directly access the function using the `::` operator followed by the function name. For example:
“`R
stats::lm
“`
This command allows you to see the code for the `lm` function from the `stats` package.
Using the `help` Function
The `help` function provides documentation for functions, which may include code snippets. While it does not show the complete function code, it can be useful for understanding the function’s purpose and usage.
“`R
help(“lm”)
“`
This will provide a detailed description along with examples of how to use `lm`.
Example of Viewing a Function’s Code
Here is a complete example demonstrating how to view a custom function:
“`R
my_function <- function(x) {
return(x^3 + x)
}
Print the function
print(my_function)
View the body
body(my_function)
Deparse the function
deparse(my_function)
```
Each of these methods will help you to effectively view and understand the code behind R functions, whether they are user-defined or part of a package.
Understanding Function Code Visibility in R Programming
Dr. Emily Carter (Senior Data Scientist, Analytics Innovations). “In R, the ability to view the code of a function is crucial for understanding its underlying mechanics. This transparency allows developers to modify or extend functionalities effectively, ensuring that the codebase remains adaptable and maintainable.”
Michael Chen (Lead Software Engineer, CodeInsight Solutions). “Accessing the source code of functions in R is not just a matter of curiosity; it is an essential practice for debugging and optimizing performance. By examining the code, developers can identify bottlenecks and implement improvements that enhance efficiency.”
Laura Henderson (Professor of Computer Science, University of Tech). “The R programming environment encourages users to explore function definitions as a way to deepen their understanding of programming paradigms. This exploration fosters better coding practices and promotes a culture of learning within the data science community.”
Frequently Asked Questions (FAQs)
How can I view the code of a function in R?
You can view the code of a function in R by using the `get` function or simply typing the function name without parentheses. For example, `get(“function_name”)` or just `function_name` will display the function’s source code.
Can I see the code for built-in R functions?
Yes, you can view the code for built-in R functions using the same methods. For example, to see the code for the `mean` function, type `mean` in the console.
What if the function is part of a package?
If the function is part of a package, ensure the package is loaded using `library(package_name)`, then you can view the function’s code as described above.
Are there any functions specifically for examining function code?
Yes, the `body()` function can be used to extract the body of a function, and `formals()` can be used to see the arguments of a function.
Can I see the code of anonymous functions?
Yes, you can view the code of anonymous functions by assigning them to a variable and then using the same methods to inspect them.
Is it possible to modify the code of a function in R?
Yes, you can modify the code of a function by reassigning it with new code. However, be cautious as this can affect the function’s behavior and any dependent code.
The ability to see the code of a function in R is fundamental for understanding how the function operates, debugging, and enhancing one’s programming skills. R provides several methods to access the source code of functions, which can be particularly useful for users who want to learn from existing code or modify it for their specific needs. Users can utilize the `getAnywhere()` function, the `body()` function, or simply type the function name without parentheses to view the code, depending on the function’s environment and scope.
Moreover, examining the code of functions can offer insights into the underlying algorithms and logic that drive the functionality. This practice not only aids in grasping the intricacies of R programming but also fosters a deeper appreciation for the language’s capabilities. Understanding how functions are constructed can lead to better coding practices and more efficient problem-solving approaches.
In summary, accessing the code of functions in R is a valuable skill that enhances one’s programming acumen. By leveraging the various methods available, users can deepen their understanding of R’s functionalities and improve their overall coding proficiency. This practice encourages a culture of learning and adaptation within the R programming community.
Author Profile
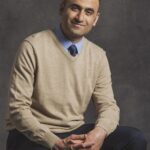
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?