Why Am I Getting a ReferenceError: React is Not Defined?
In the dynamic world of web development, few frameworks have captured the attention of developers quite like React. This powerful JavaScript library has revolutionized the way we build user interfaces, enabling the creation of interactive and responsive applications with ease. However, as with any technology, developers often encounter challenges that can hinder their progress. One such common issue is the dreaded `ReferenceError: React is not defined`. This seemingly simple error can lead to frustration and confusion, especially for those new to the React ecosystem. In this article, we will delve into the causes of this error, explore its implications, and provide actionable solutions to help you get back on track.
When working with React, developers may find themselves staring at a blank screen or a console filled with error messages, with `ReferenceError: React is not defined` being one of the most perplexing. This error typically arises when the React library is not properly imported or when there are issues with the project’s configuration. Understanding the underlying reasons for this error is crucial for any developer aiming to build robust applications. By addressing the root causes, you can streamline your development process and enhance your coding skills.
Throughout this article, we will break down the common pitfalls that lead to the `ReferenceError`, offering insights into best practices for importing
Understanding the Error
A `ReferenceError: react is not defined` typically occurs when the React library is not properly imported or recognized within your JavaScript environment. This error indicates that the code is attempting to reference the React variable, but it has not been declared or is out of scope.
Common scenarios that lead to this error include:
- Forgetting to import React at the top of your component file.
- Incorrectly set up bundlers or module loaders.
- Using React in a script without including the necessary library file.
How to Fix the Error
To resolve the `ReferenceError: react is not defined`, follow these steps:
- Ensure Proper Import: Check that you have imported React at the top of your file. For example:
“`javascript
import React from ‘react’;
“`
- Check File Extensions: Make sure that your file has the correct file extension. React components should typically have a `.js` or `.jsx` extension.
- Verify Module Bundler Configuration: If you are using a module bundler like Webpack or Parcel, ensure that it is configured correctly to handle `.js` and `.jsx` files.
- Include React in HTML: If you are not using a module bundler, include the React library directly in your HTML file. This can be done by adding the following script tags:
“`html
“`
- Check for Typos: Ensure there are no typographical errors in your import statements or component definitions.
Best Practices to Avoid the Error
To minimize the chances of encountering this error in the future, consider implementing the following best practices:
- Always import React at the beginning of your files where JSX is used.
- Utilize modern JavaScript practices, such as ES6 modules, to manage imports and exports effectively.
- Maintain a consistent file structure and naming conventions to make it easier to identify components and their dependencies.
Quick Reference Table
Issue | Solution |
---|---|
No React import | Add `import React from ‘react’;` at the top of your file. |
Wrong file type | Use `.js` or `.jsx` file extensions. |
Bundler misconfiguration | Check your bundler settings for file type handling. |
Missing script tags in HTML | Include React scripts in your HTML file. |
Typographical errors | Review your code for any spelling mistakes in imports or JSX. |
Understanding the Cause of “ReferenceError: React is not defined”
The “ReferenceError: React is not defined” typically arises in React applications when the React library has not been correctly imported or is missing in the codebase. This error signifies that the JavaScript engine cannot find the React variable, which is essential for rendering components.
Common Scenarios Leading to the Error
Several situations can lead to this error:
- Missing Import Statement: If the React library is not imported in your component file, the error will occur. Every file that uses React components needs to have the import statement at the top.
- Incorrect Import Path: Specifying an incorrect path in the import statement can result in the library not being found.
- Build Configuration Issues: Issues with build tools or configurations (like Webpack or Babel) can also prevent React from being recognized.
- Global Scope Issues: In cases where React is expected to be available globally (for instance, through a CDN), and it is not loaded, the error will manifest.
Solutions to Resolve the Error
To resolve the “ReferenceError: React is not defined,” consider the following solutions:
- Check Import Statements: Ensure that you have the correct import statement at the top of your file:
“`javascript
import React from ‘react’;
“`
- Verify the React Installation: Confirm that React is installed in your project. You can check this by looking at your `package.json` file or running:
“`bash
npm list react
“`
- Correct Import Path: If using a relative path, ensure it points to the correct location of the React library:
“`javascript
import React from ‘./path/to/react’;
“`
- Global React Configuration: If using a CDN, ensure that the script is included before your script files. For example:
“`html
“`
- Check Build Configuration: If using tools like Webpack, ensure that the configuration is set to properly handle React files. This includes ensuring Babel is configured to transpile JSX.
Debugging Tips
Here are some tips to help debug the issue effectively:
- Browser Console: Check the console for additional error messages that may provide more context.
- Rebuild the Project: Sometimes a simple rebuild can resolve configuration or caching issues.
- Inspect Node Modules: Look inside the `node_modules` directory to confirm that React is properly installed.
- Use ESLint: Integrate ESLint with React rules to catch import errors and other potential issues early in the development process.
- Version Conflicts: Ensure that the version of React being used is compatible with other libraries in your project.
Addressing the “ReferenceError: React is not defined” requires a systematic approach to identifying the root cause. By ensuring proper imports, verifying installations, and checking configurations, developers can swiftly resolve this common issue and continue building robust React applications.
Understanding the ‘ReferenceError: React is not defined’ Issue
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘ReferenceError: React is not defined’ typically arises when the React library is not properly imported into your JavaScript file. It is crucial to ensure that the import statement is correctly placed at the top of your component file to avoid this error.”
Michael Chen (Front-End Developer, CodeCraft Solutions). “This error can also occur if the React library is not included in your project dependencies. Double-check your package.json file to confirm that React is listed and that you have installed it correctly using npm or yarn.”
Sarah Johnson (React Specialist, Web Development Hub). “In some cases, this error may be due to an incorrect build configuration. If you are using a module bundler like Webpack, ensure that your configuration is set up to handle JSX and that React is included in the build process.”
Frequently Asked Questions (FAQs)
What does the error “ReferenceError: React is not defined” mean?
This error indicates that the React library is not properly imported or defined in your JavaScript file, preventing the use of React components and functions.
How can I fix the “ReferenceError: React is not defined” error?
To resolve this error, ensure that you have imported React at the top of your file using `import React from ‘react’;` or include the React script in your HTML file if you are not using a module bundler.
Is it necessary to import React in every file that uses JSX?
Yes, in versions prior to React 17, it is required to import React in every file that uses JSX. However, starting with React 17, it is no longer mandatory due to the new JSX transform.
What are common mistakes that lead to this error?
Common mistakes include forgetting to import React, misspelling the import statement, or using React components in files without the necessary import.
Can this error occur in a React project created with Create React App?
Yes, this error can still occur in a Create React App project if you accidentally remove the import statement for React or if you are using a file that does not have React imported.
What should I check if I still see the error after importing React?
If the error persists after importing React, check for typos in your import statement, ensure that your project dependencies are correctly installed, and verify that your build configuration supports JSX.
The error message “ReferenceError: React is not defined” typically indicates that the React library has not been properly imported or is not available in the current scope of your JavaScript file. This is a common issue encountered by developers, especially those who are new to React or are working in environments where module imports are required. To resolve this error, it is essential to ensure that React is imported correctly at the beginning of your component file. This can be done by adding the line `import React from ‘react’;` at the top of your file.
Another aspect to consider is the environment in which you are working. If you are using a build tool such as Webpack or a framework like Create React App, make sure that your project is set up correctly and that React is included in your project’s dependencies. If React is not listed in your package.json file, you will need to install it using npm or yarn. Running the command `npm install react` or `yarn add react` in your project directory will ensure that the library is available for use.
In addition to proper imports and installations, it is also crucial to check for typos or syntax errors that may lead to this reference error. A simple mistake, such as miss
Author Profile
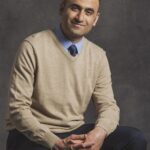
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?