How Can I Use Regular Expressions to Validate Email Addresses Effectively?
In our increasingly digital world, email remains a cornerstone of communication, serving as a vital link between individuals and organizations alike. Whether you’re crafting a newsletter, managing user registrations, or simply reaching out to friends, ensuring that the email addresses you collect are valid is crucial. Enter the regular expression—a powerful tool that can help you sift through the chaos of potential typos and formatting errors to ensure that every email address adheres to the established standards. In this article, we will delve into the intricacies of crafting a regular expression specifically designed to validate email addresses, equipping you with the knowledge to enhance your data integrity and streamline your communication processes.
Understanding the structure of an email address is the first step in building an effective validation mechanism. At its core, an email address consists of a local part, an “@” symbol, and a domain part. However, the nuances of what constitutes a valid email address can be complex, with various rules governing permissible characters and formats. Regular expressions provide a flexible and efficient way to encapsulate these rules, allowing developers to create patterns that can accurately match valid email addresses while filtering out invalid ones.
As we explore the world of regular expressions for email validation, we will highlight common pitfalls and best practices to ensure your patterns are both robust and efficient. From
Understanding Email Validation with Regular Expressions
To validate an email address using regular expressions, it is essential to understand the structure of a valid email. An email typically consists of a local part, an “@” symbol, and a domain part. The local part can include letters, numbers, dots, hyphens, and underscores, while the domain part usually includes the domain name followed by a top-level domain (TLD).
A commonly used regular expression for email validation is:
“`
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
“`
Breakdown of the Regular Expression
- `^`: Asserts the start of the string.
- `[a-zA-Z0-9._%+-]+`: Matches one or more characters that can be letters (both uppercase and lowercase), numbers, and special characters such as dot, underscore, percentage, plus, and hyphen.
- `@`: Matches the “@” symbol, which separates the local part from the domain.
- `[a-zA-Z0-9.-]+`: Matches one or more characters for the domain name, which can also include dots and hyphens.
- `\.`: Escapes the dot character to match it literally.
- `[a-zA-Z]{2,}`: Ensures that the top-level domain consists of at least two letters.
- `$`: Asserts the end of the string.
Common Email Validation Scenarios
While the above regex covers many valid cases, there are some scenarios to consider:
- Internationalized Domain Names (IDNs): Support for non-ASCII characters in domain names requires additional handling.
- Specific TLD Requirements: Certain domains may have specific formats that need to be considered.
- Length Restrictions: The overall length of the email address must not exceed 254 characters.
Example Email Validation Patterns
Here’s a summary of various patterns for email validation, including some common formats:
Email Format | Example | Valid? |
---|---|---|
Basic email | [email protected] | Yes |
Email with subdomain | [email protected] | Yes |
Email with special chars | [email protected] | Yes |
Invalid email (missing @) | userexample.com | No |
Invalid email (invalid TLD) | [email protected] | No |
Best Practices for Email Validation
- Use Regex Sparingly: Regular expressions can become complex and difficult to maintain. Consider using built-in libraries or functions for email validation when available.
- User Feedback: Provide clear error messages to users when their input fails validation, indicating what part of their email is incorrect.
- Test Rigorously: Validate against a diverse set of email formats to ensure robustness.
By utilizing a comprehensive regular expression and adhering to best practices, developers can efficiently validate email addresses, enhancing user experience and reducing errors in data collection.
Regular Expression for Email Validation
The following regular expression is commonly used to validate email addresses. It checks for a basic structure that includes a local part, an “@” symbol, and a domain part.
“`regex
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
“`
Breakdown of the Regex Components
Component | Description |
---|---|
`^` | Asserts the start of the string. |
`[a-zA-Z0-9._%+-]+` | Matches one or more characters from the set: letters (both cases), digits, and special characters (., _, %, +, -). This represents the local part of the email. |
`@` | Matches the “@” symbol, which separates the local part from the domain. |
`[a-zA-Z0-9.-]+` | Matches one or more characters for the domain name, allowing letters, digits, dots, and hyphens. |
`\.` | Escapes the dot (.) to ensure it is treated as a literal character. |
`[a-zA-Z]{2,}` | Matches the top-level domain, which must consist of at least two letters. |
`$` | Asserts the end of the string. |
Usage Considerations
While the above regex covers many valid email formats, it may not capture all edge cases specified in the official specifications (RFC 5322). Some considerations include:
- Unicode Characters: The regex does not account for internationalized email addresses (those with characters outside the ASCII range).
- Special Cases: Email addresses with special formats, such as quoted strings or comments, may not be validated correctly.
Example Validations
Email Address | Valid? |
---|---|
`[email protected]` | Yes |
`[email protected]` | Yes |
`[email protected]` | Yes |
`[email protected]` | No |
`[email protected]` | No |
Implementation in Programming Languages
Here are examples of how to implement this regular expression in various programming languages:
Python Example:
“`python
import re
email_regex = r’^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$’
email = “[email protected]”
if re.match(email_regex, email):
print(“Valid email”)
else:
print(“Invalid email”)
“`
JavaScript Example:
“`javascript
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
const email = “[email protected]”;
if (emailRegex.test(email)) {
console.log(“Valid email”);
} else {
console.log(“Invalid email”);
}
“`
Conclusion
Utilizing a regular expression for email validation can help filter out incorrect formats. However, developers should be aware of its limitations and consider additional checks or libraries for comprehensive validation, especially when dealing with internationalization or specialized email formats.
Expert Insights on Email Validation Using Regular Expressions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A well-crafted regular expression for email validation should account for the various formats emails can take. It is essential to balance strictness with flexibility to accommodate legitimate email addresses without inadvertently rejecting valid ones.”
James Liu (Lead Developer, SecureNet Solutions). “While regular expressions can effectively validate the syntax of an email address, they should not be the sole method of verification. Implementing a two-step verification process enhances security and reliability beyond what regex can provide.”
Maria Gonzalez (Data Privacy Consultant, CyberSafe Advisory). “Using regular expressions for email validation is a common practice, but developers must be cautious of overly complex patterns that can lead to maintenance challenges. Simplicity often yields better long-term results in code readability and performance.”
Frequently Asked Questions (FAQs)
What is a regular expression for validating email addresses?
A common regular expression for validating email addresses is: `^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$`. This pattern checks for a valid format, including characters before and after the “@” symbol and ensuring a proper domain structure.
Why is it important to validate email addresses using regular expressions?
Validating email addresses helps ensure that the input conforms to standard email formats, reducing the risk of errors in communication, preventing spam, and ensuring data integrity in databases.
Are there any limitations to using regular expressions for email validation?
Yes, regular expressions can only validate the format of an email address, not its existence. They may also not cover all valid email formats as defined by the official standards, potentially leading to negatives.
Can I modify the regular expression to allow specific characters in the email?
Yes, you can modify the regular expression to include or exclude specific characters by adjusting the character classes within the brackets. For instance, to allow additional symbols, you would add them to the character set.
How can I test a regular expression for email validation?
You can test a regular expression using various online regex testers, programming language environments, or text editors that support regex. Input sample email addresses to see if they match the pattern.
Is it necessary to validate email addresses on both client-side and server-side?
Yes, it is essential to validate email addresses on both client-side and server-side. Client-side validation provides immediate feedback to users, while server-side validation ensures data integrity and security before processing the information.
In summary, crafting a regular expression to validate email addresses is a complex task due to the diverse formats that emails can take. A well-structured regular expression must account for various components of an email, including the local part, the “@” symbol, and the domain part. While there are simplified regex patterns available, they may not cover all valid email formats as specified by the standards, such as RFC 5321 and RFC 5322. Therefore, it is essential to strike a balance between validation accuracy and practical usability.
Key takeaways from the discussion include the recognition that while regex can effectively filter out many invalid email formats, it is not infallible. Overly strict patterns may inadvertently reject legitimate email addresses, while overly permissive patterns may allow invalid ones. It is advisable to use regex as a preliminary validation step, followed by additional checks, such as sending a verification email, to ensure the authenticity of the address.
Furthermore, developers should remain aware of the evolving nature of email standards and the importance of keeping their validation patterns updated. As email usage continues to grow and change, maintaining an adaptable approach to email validation will enhance user experience and data integrity. Ultimately, while regex is a powerful tool in email validation, it
Author Profile
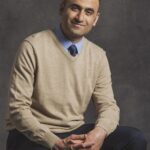
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?