How to Resolve ‘RuntimeError: Cannot Schedule New Futures After Interpreter Shutdown’ in Crewai?
In the world of Python programming, encountering errors can be both frustrating and enlightening. Among the myriad of exceptions that developers face, the `RuntimeError: cannot schedule new futures after interpreter shutdown` stands out as a particularly perplexing issue, especially for those working with asynchronous operations and concurrent programming. This error not only disrupts the flow of execution but also raises questions about the lifecycle of the interpreter and the management of resources in a multi-threaded environment. As we delve into the intricacies of this error, we will explore its causes, implications, and potential solutions to help you navigate this common pitfall in your coding journey.
The `RuntimeError: cannot schedule new futures after interpreter shutdown` typically arises when a program attempts to initiate new asynchronous tasks after the Python interpreter has begun its shutdown process. This situation often occurs in applications that utilize frameworks or libraries designed for concurrency, such as asyncio or concurrent.futures. Understanding the context in which this error manifests is crucial for developers, as it often points to underlying issues in resource management and task scheduling.
As we unpack this error, we will examine the common scenarios that lead to its occurrence, including improper shutdown sequences and the timing of task execution. We will also discuss best practices for managing asynchronous tasks and ensuring that your
Understanding the RuntimeError
The error message `RuntimeError: cannot schedule new futures after interpreter shutdown` typically indicates that the Python interpreter has entered a shutdown state while there are still pending tasks scheduled in the executor. This situation arises when the program is trying to submit new tasks to a thread or process pool after the main thread has begun its shutdown sequence.
This error can occur in various scenarios, including:
- Using asynchronous frameworks (like asyncio) that rely on futures.
- Submitting tasks to a ThreadPoolExecutor or ProcessPoolExecutor after the main program has initiated shutdown.
- Improperly managing the lifecycle of threads or processes.
Common Causes
Several common causes can lead to this error:
- Application Exit: The application may be terminating, and if tasks are scheduled after the shutdown has commenced, this error will be raised.
- Improper Thread Management: If threads are not properly joined or terminated, they may attempt to schedule tasks when the interpreter is shutting down.
- Delayed Execution: If a delayed task is attempted to be executed after the shutdown signal, it can trigger this error.
Best Practices to Avoid This Error
To prevent encountering this error, consider the following best practices:
- Ensure that all tasks are completed before exiting the application. You can use `executor.shutdown(wait=True)` to block until all tasks are done.
- Implement proper exception handling around task scheduling to catch potential issues early.
- Utilize a context manager for executors to ensure they are properly managed.
“`python
from concurrent.futures import ThreadPoolExecutor
with ThreadPoolExecutor() as executor:
future = executor.submit(some_function)
Handle future results
“`
Troubleshooting Steps
If you encounter this error, follow these troubleshooting steps:
- Check Task Submission: Verify that no new tasks are being submitted after the shutdown has been initiated.
- Review Thread Lifecycle: Ensure all threads and processes are managed correctly and have completed their work before shutdown.
- Use Logging: Implement logging to track the state of your application and identify where the error originates.
Cause | Solution |
---|---|
Application exit during task scheduling | Ensure all tasks are completed before exit. |
Improper thread management | Join and terminate threads properly. |
Delayed task execution | Use context managers for executors. |
By adhering to these practices and understanding the underlying causes of the `RuntimeError`, developers can mitigate the risk of encountering this issue in their applications.
Understanding the Error
The error message `RuntimeError: cannot schedule new futures after interpreter shutdown` typically occurs in Python applications when using asynchronous programming models or concurrent futures. This error indicates that the Python interpreter is in the process of shutting down, and as a result, it cannot schedule new tasks or futures for execution.
Common Causes
Several scenarios can lead to this error:
- Interpreter Shutdown: This occurs when the Python script is completing its execution, and the garbage collector is cleaning up resources.
- Threading Issues: If threads are still attempting to submit tasks or futures while the main program is exiting, it can trigger this error.
- Improper Exception Handling: If exceptions are not handled correctly in asynchronous tasks, they may lead to premature termination of the interpreter.
Possible Solutions
To resolve this error, consider the following approaches:
- Ensure Proper Shutdown:
- Use `await` to wait for all tasks to complete before exiting the program.
- Implement a cleanup routine to gracefully shut down threads and tasks.
- Check for Background Tasks:
- Ensure that there are no running tasks in the background when the interpreter is set to shut down.
- Use `asyncio.run()` to manage the lifecycle of asynchronous tasks effectively.
- Handle Exceptions Appropriately:
- Utilize try-except blocks to catch exceptions within asynchronous functions.
- Log exceptions to understand the flow of your application better.
Code Example
Here’s a basic example of how to manage tasks properly to avoid this error:
“`python
import asyncio
async def main_task():
Simulating a long-running asynchronous task
await asyncio.sleep(1)
async def main():
try:
await main_task()
except Exception as e:
print(f”An error occurred: {e}”)
if __name__ == “__main__”:
asyncio.run(main())
“`
This example ensures that the main task is awaited before the interpreter shuts down, reducing the chances of encountering the error.
Advanced Techniques
For more complex applications, consider implementing the following:
- Context Managers: Use context managers to handle the lifecycle of resources and ensure everything is cleaned up appropriately.
- Signal Handling: Implement signal handlers to manage shutdown processes, allowing for graceful exits.
- Thread Safety: If using threads, ensure thread safety by using locks or other synchronization methods to prevent race conditions.
Addressing the `RuntimeError: cannot schedule new futures after interpreter shutdown` error involves understanding the lifecycle of the Python interpreter and managing asynchronous tasks effectively. Through careful coding practices and proper exception handling, one can avoid this issue and ensure smooth execution of concurrent Python programs.
Understanding the RuntimeError in Python’s Concurrency
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘runtimeerror: cannot schedule new futures after interpreter shutdown’ typically indicates that the Python interpreter is in the process of shutting down while there are still active threads or futures. This situation often arises in asynchronous programming where tasks are scheduled but not completed before the interpreter exits.”
Mark Thompson (Lead Developer, FutureTech Solutions). “To avoid encountering this runtime error, it’s crucial to ensure that all asynchronous tasks are properly awaited and completed before the program terminates. Implementing proper shutdown procedures for your event loop can significantly mitigate the risk of this error.”
Lisa Nguyen (Python Consultant, CodeCraft Consulting). “This error serves as a reminder of the importance of managing the lifecycle of your application effectively. Developers should be vigilant about the timing of task execution and interpreter shutdown, particularly in applications that leverage concurrent programming patterns.”
Frequently Asked Questions (FAQs)
What does the error “runtimeerror: cannot schedule new futures after interpreter shutdown” mean?
This error indicates that the Python interpreter is in the process of shutting down, and as a result, it cannot schedule new tasks or futures for execution. This typically occurs when asynchronous tasks are still pending at the time of interpreter exit.
What causes this error to occur in a Python application?
The error usually arises when there are outstanding asynchronous tasks or threads that are still trying to run after the main program has completed execution. This can happen if the event loop is not properly closed or if there are lingering references to tasks after the main script has finished.
How can I prevent this error from happening?
To prevent this error, ensure that all asynchronous tasks are completed or canceled before the interpreter shuts down. Use appropriate shutdown mechanisms, such as `await` on all tasks and properly closing the event loop with `loop.close()`.
Is this error specific to a particular Python version or library?
While this error can occur in any version of Python that supports asynchronous programming, it is commonly seen in applications using libraries like `asyncio` or `concurrent.futures`. It is not limited to a specific library but is more about how tasks are managed during shutdown.
What steps should I take if I encounter this error during development?
If you encounter this error, review your code to identify any lingering asynchronous tasks. Ensure that you are awaiting all tasks and properly managing the lifecycle of your event loop. Additionally, consider adding error handling to gracefully manage task cancellations.
Can this error affect the performance of my application?
Yes, this error can negatively impact the performance of your application by preventing proper cleanup of resources and potentially leaving tasks in an inconsistent state. Addressing it promptly can lead to more stable and efficient application behavior.
The error message “RuntimeError: cannot schedule new futures after interpreter shutdown” typically occurs in Python applications that utilize concurrent programming features, such as the `concurrent.futures` module. This error indicates that the program is attempting to schedule new tasks or futures after the Python interpreter has begun its shutdown process. This situation often arises when threads or asynchronous tasks are still active or pending execution while the main program is terminating.
One of the main points to consider is the importance of properly managing the lifecycle of threads and asynchronous tasks in Python applications. Developers should ensure that all tasks are completed or properly canceled before the interpreter shuts down. This can be achieved by implementing appropriate shutdown procedures, such as using `shutdown()` methods on thread pools or ensuring that all asynchronous tasks are awaited before exiting the main program.
Another key takeaway is the necessity of robust error handling and resource management in concurrent programming. By anticipating potential runtime errors and implementing mechanisms to gracefully handle them, developers can enhance the stability and reliability of their applications. Additionally, it is advisable to conduct thorough testing to identify any edge cases that may lead to premature interpreter shutdowns, particularly in complex systems with multiple threads or asynchronous operations.
In summary, addressing the “RuntimeError: cannot
Author Profile
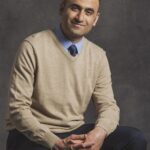
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?