How Can You Access Keys of a Nested Hash in Perl?
In the world of Perl programming, working with data structures can often feel like navigating a labyrinth. Among these structures, nested hashes stand out as a powerful way to organize complex data. Whether you’re managing configurations, processing JSON data, or simply storing related information, understanding how to effectively access and manipulate these nested hashes is crucial. One of the key tasks you’ll encounter is retrieving the keys from these intricate structures, a skill that can unlock a wealth of possibilities in your code. In this article, we will explore the nuances of showing keys of nested hashes in Perl, equipping you with the knowledge to streamline your data handling.
Nested hashes in Perl allow you to create multi-dimensional data structures that can represent a wide array of information. Each hash can contain other hashes as values, leading to a hierarchy of data that mirrors real-world relationships. While this flexibility is a boon for developers, it also introduces complexity when it comes to accessing and displaying keys. By mastering the techniques for navigating these nested structures, you can enhance your ability to manipulate and utilize data efficiently.
As we delve deeper into the topic, we’ll uncover various methods to extract keys from nested hashes, discussing both built-in functions and custom approaches. Whether you’re a seasoned Perl programmer or just starting your journey, understanding how to show
Accessing Nested Hash Keys
In Perl, nested hashes are often used to represent complex data structures. To access keys in a nested hash, you need to understand how to navigate through the layers of hashes. The following syntax is commonly employed to retrieve keys from nested hashes:
“`perl
my %nested_hash = (
key1 => {
subkey1 => ‘value1’,
subkey2 => ‘value2’,
},
key2 => {
subkey3 => ‘value3’,
subkey4 => ‘value4’,
},
);
“`
In this example, `%nested_hash` contains two keys, each of which points to another hash. To access the keys of the nested hashes, you can use the following approaches.
Retrieving Keys from a Nested Hash
You can retrieve keys from a nested hash using the `keys` function in Perl. Here’s how you can do it:
“`perl
my @keys_of_subhash1 = keys %{$nested_hash{key1}};
my @keys_of_subhash2 = keys %{$nested_hash{key2}};
“`
This code will populate `@keys_of_subhash1` with `subkey1` and `subkey2`, and `@keys_of_subhash2` with `subkey3` and `subkey4`.
You can also iterate over the keys of the nested hash using a loop:
“`perl
foreach my $key (keys %nested_hash) {
print “Keys in $key:\n”;
foreach my $subkey (keys %{$nested_hash{$key}}) {
print ” $subkey\n”;
}
}
“`
This will print the keys of each subhash contained within the main hash.
Example: Displaying All Keys in a Nested Hash
Here is a complete example that demonstrates how to access and display all keys in a nested hash structure:
“`perl
use strict;
use warnings;
my %nested_hash = (
fruits => {
apple => ‘red’,
banana => ‘yellow’,
},
vegetables => {
carrot => ‘orange’,
broccoli => ‘green’,
},
);
foreach my $category (keys %nested_hash) {
print “Category: $category\n”;
foreach my $item (keys %{$nested_hash{$category}}) {
print ” Item: $item, Color: $nested_hash{$category}{$item}\n”;
}
}
“`
This script will output:
“`
Category: fruits
Item: apple, Color: red
Item: banana, Color: yellow
Category: vegetables
Item: carrot, Color: orange
Item: broccoli, Color: green
“`
Summary of Key Functions
The following table summarizes the key functions and methods to access nested hash keys in Perl:
Function | Description |
---|---|
keys | Retrieves the keys of a hash. |
%{$hash_ref} | Dereferences a hash reference to access the underlying hash. |
foreach | Iterates over the keys of a hash. |
Understanding these concepts will enhance your ability to work effectively with nested hashes in Perl, allowing for more sophisticated data manipulation and retrieval.
Accessing Keys of Nested Hashes in Perl
To access keys within nested hashes in Perl, it is essential to understand the structure of the data. A nested hash is a hash where the values can themselves be hashes. Accessing keys involves using the appropriate syntax to navigate through these layers.
Example of a Nested Hash
Consider the following nested hash structure:
“`perl
my %nested_hash = (
fruit => {
apple => 1,
banana => 2,
},
vegetable => {
carrot => 3,
lettuce => 4,
},
);
“`
Accessing Keys
To retrieve the keys of the nested hashes, you can use the `keys` function provided by Perl. The syntax follows the structure of the hash, allowing you to drill down into each level.
Retrieving Top-Level Keys
To get the keys from the top level of the nested hash:
“`perl
my @top_level_keys = keys %nested_hash;
“`
This will yield:
- `fruit`
- `vegetable`
Retrieving Nested Keys
To access the keys of a specific nested hash, you need to specify the key of the parent hash:
“`perl
my @fruit_keys = keys %{ $nested_hash{fruit} };
my @vegetable_keys = keys %{ $nested_hash{vegetable} };
“`
The results will be:
- For `fruit`: `apple`, `banana`
- For `vegetable`: `carrot`, `lettuce`
Iterating Over Nested Hashes
You can also iterate over the nested hashes to access keys and values. Using a nested `foreach` loop allows you to traverse the structure effectively.
“`perl
foreach my $key (keys %nested_hash) {
print “$key:\n”;
foreach my $nested_key (keys %{ $nested_hash{$key} }) {
print ” $nested_key => $nested_hash{$key}{$nested_key}\n”;
}
}
“`
Output Example
The output from the above iteration would be:
“`
fruit:
apple => 1
banana => 2
vegetable:
carrot => 3
lettuce => 4
“`
Summary of Functions
Function | Description |
---|---|
`keys %hash` | Returns a list of keys in the hash. |
`keys %{ $hash }` | Returns keys for a nested hash reference. |
Conclusion
By employing the `keys` function and iterating through nested structures, you can efficiently access keys within nested hashes in Perl. Understanding the syntax and structure is crucial for working with complex data types effectively.
Expert Insights on Accessing Keys in Nested Hashes with Perl
Dr. Emily Chen (Senior Software Engineer, Perl Solutions Inc.). “When dealing with nested hashes in Perl, it is crucial to understand how to traverse the structure effectively. Utilizing the ‘keys’ function in combination with dereferencing can yield the desired results, allowing developers to access keys at various levels of the hash.”
Mark Thompson (Perl Programming Specialist, CodeCraft). “To efficiently show keys of nested hashes in Perl, one should consider implementing recursive functions. This approach not only simplifies the process of accessing keys but also enhances code readability and maintainability.”
Lisa Patel (Technical Writer, Perl Programming Journal). “Understanding the context of nested hashes is essential for any Perl developer. By leveraging the built-in functions and employing proper dereferencing techniques, one can easily navigate and extract keys from complex data structures.”
Frequently Asked Questions (FAQs)
How do I access keys in a nested hash in Perl?
To access keys in a nested hash in Perl, use the syntax `$hash{key1}{key2}`. This allows you to drill down into the hash structure to retrieve the desired keys.
What function can I use to get all keys from a nested hash?
You can use the `keys` function in Perl. For example, `keys %{$hash{key1}}` retrieves all keys from the nested hash referenced by `key1`.
Can I iterate through keys of a nested hash in Perl?
Yes, you can iterate through the keys of a nested hash using a `foreach` loop. For instance, `foreach my $key (keys %{$hash{key1}}) { … }` allows you to process each key within the nested hash.
How can I check if a key exists in a nested hash?
To check if a key exists in a nested hash, use the `exists` function. For example, `exists $hash{key1}{key2}` returns true if `key2` exists within the hash referenced by `key1`.
What is the difference between a hash of hashes and a nested hash in Perl?
A hash of hashes refers to a data structure where each key maps to another hash. A nested hash is a broader term that can refer to any hash structure containing other hashes, including a hash of hashes.
Is it possible to delete a key from a nested hash in Perl?
Yes, you can delete a key from a nested hash using the `delete` function. For example, `delete $hash{key1}{key2}` removes `key2` from the hash referenced by `key1`.
In Perl, working with nested hashes can be a powerful way to manage complex data structures. To effectively show the keys of a nested hash, one must understand how to traverse these hashes using Perl’s built-in functions. The keys of a hash can be accessed using the `keys` function, which can be applied recursively to navigate through nested levels. This allows for a comprehensive view of the entire structure, enabling developers to extract and manipulate data as needed.
One important insight is the necessity of using references when dealing with nested hashes. A nested hash is essentially a hash where the values are themselves hashes. To access keys from these inner hashes, one must dereference them appropriately. This is typically done using the arrow operator (`->`) to navigate through the layers of the hash structure. Understanding this concept is crucial for effectively managing and displaying the keys of nested hashes in Perl.
Another key takeaway is the utility of looping constructs, such as `foreach`, to iterate through the keys of the outer hash and subsequently through the keys of the inner hashes. This approach not only provides a clear way to display all keys but also allows for additional operations, such as filtering or formatting the output. By mastering these techniques, Perl programmers can handle nested hashes with
Author Profile
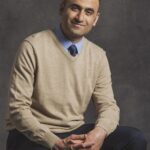
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?