How Can You Efficiently Use SQL Loops to Iterate Through Each Value in a List?
In the world of database management, SQL (Structured Query Language) serves as the backbone for interacting with relational databases. Among its many capabilities, the ability to iterate over a list of values using loops is a powerful technique that can streamline data processing and enhance the efficiency of queries. Whether you’re a seasoned developer or a newcomer to database programming, understanding how to implement loops in SQL can significantly elevate your data manipulation skills. This article will delve into the nuances of using loops in SQL, particularly focusing on the `FOR EACH` construct, which allows for seamless iteration over lists of values.
SQL loops provide a dynamic way to handle repetitive tasks, enabling developers to execute a series of commands for each item in a specified list. This functionality is particularly useful in scenarios where bulk operations are required, such as updating records, performing calculations, or even generating reports. By mastering the art of looping in SQL, you can automate processes that would otherwise be tedious and time-consuming, thereby improving the overall performance of your database interactions.
As we explore the intricacies of SQL loops, we’ll highlight the syntax and best practices that can help you implement efficient and effective solutions. From understanding the basic structure of a loop to applying it in real-world scenarios, this article aims to equip you with the knowledge needed
Understanding SQL Loops
In SQL, loops are primarily utilized in procedural extensions like PL/SQL (Oracle) or T-SQL (SQL Server). Loops allow you to execute a set of SQL statements repeatedly until a certain condition is met. This can be particularly useful when you need to process multiple rows of data sequentially.
Types of SQL Loops
There are several types of loops available in SQL, including:
- Basic Loop: A simple loop that continues indefinitely until explicitly exited.
- While Loop: Executes a block of statements as long as a specified condition evaluates to true.
- For Loop: Iterates through a defined range or set of values.
Using a For Loop in SQL
In T-SQL, the `FOR` loop is not directly available; however, you can achieve similar functionality using a `WHILE` loop or a cursor. In PL/SQL, you can directly use a `FOR` loop to iterate over a collection or a range. Below is an example of using a `FOR` loop in PL/SQL:
“`sql
DECLARE
TYPE number_list IS TABLE OF NUMBER;
num_list number_list := number_list(1, 2, 3, 4, 5);
BEGIN
FOR i IN 1..num_list.COUNT LOOP
DBMS_OUTPUT.PUT_LINE(‘Number: ‘ || num_list(i));
END LOOP;
END;
“`
This example declares a collection of numbers and iterates through each value, printing it to the output.
Iterating Over a List with a Cursor
When working with T-SQL in SQL Server, you can utilize a cursor to loop through a result set. Here’s a basic example:
“`sql
DECLARE @num INT;
DECLARE num_cursor CURSOR FOR
SELECT number FROM NumbersTable;
OPEN num_cursor;
FETCH NEXT FROM num_cursor INTO @num;
WHILE @@FETCH_STATUS = 0
BEGIN
PRINT ‘Number: ‘ + CAST(@num AS VARCHAR);
FETCH NEXT FROM num_cursor INTO @num;
END
CLOSE num_cursor;
DEALLOCATE num_cursor;
“`
In this example:
- A cursor is declared to select numbers from `NumbersTable`.
- The loop continues until all rows have been processed.
Best Practices for Using Loops in SQL
When implementing loops in SQL, consider the following best practices:
- Limit Loop Usage: Avoid using loops for set-based operations; SQL is optimized for handling sets of data.
- Use Cursors Sparingly: Cursors can lead to performance issues, so use them only when necessary.
- Optimize Queries: Ensure that your SQL queries are optimized to reduce the load during loop execution.
Performance Considerations
Using loops can significantly impact performance, particularly with large datasets. Here’s a comparison of set-based operations versus looping constructs:
Method | Performance | Use Case |
---|---|---|
Set-based Operation | High | Processing large datasets efficiently |
Cursor Loop | Medium to Low | Iterative processing of smaller datasets |
While Loop | Medium | Conditional repetitive tasks |
In summary, while loops can provide flexibility in SQL programming, they should be employed judiciously to maintain optimal performance.
Implementing a Loop in SQL for Each Value in a List
SQL does not have traditional looping constructs like some programming languages, but you can achieve similar functionality using cursors or common table expressions (CTEs). Below are methods to loop through a list of values in SQL.
Using Cursors
Cursors allow you to retrieve rows from a result set one at a time. The following steps illustrate how to use a cursor to loop through a list of values.
- Declare the Cursor: Define the SQL statement that selects the values you want to loop through.
- Open the Cursor: Execute the SQL statement.
- Fetch the Values: Retrieve each row one at a time.
- Process Each Row: Perform the desired operations on the fetched data.
- Close the Cursor: Release the resources associated with the cursor.
“`sql
DECLARE @value INT;
DECLARE cursor_example CURSOR FOR
SELECT column_name FROM table_name WHERE condition;
OPEN cursor_example;
FETCH NEXT FROM cursor_example INTO @value;
WHILE @@FETCH_STATUS = 0
BEGIN
— Perform actions with @value
PRINT @value; — Example action
FETCH NEXT FROM cursor_example INTO @value;
END
CLOSE cursor_example;
DEALLOCATE cursor_example;
“`
Using Common Table Expressions (CTEs)
CTEs can also be used to process data iteratively. This is particularly useful when you want to perform operations on a subset of data without creating temporary tables.
“`sql
WITH ValueList AS (
SELECT value FROM (VALUES (1), (2), (3), (4)) AS T(value)
)
SELECT * FROM ValueList;
“`
You can combine CTEs with other SQL operations to manipulate data. For example, to perform an operation for each value in the CTE:
“`sql
WITH ValueList AS (
SELECT value FROM (VALUES (1), (2), (3), (4)) AS T(value)
)
SELECT value, value * 2 AS DoubleValue FROM ValueList;
“`
Using Temporary Tables
Another approach involves using temporary tables. This method allows you to store the list of values and iterate through them:
- Create a Temporary Table: Store the list of values.
- Insert Values: Populate the temporary table with the desired data.
- Select and Process: Use a loop or cursor to process the data.
“`sql
CREATE TABLE TempValues (Value INT);
INSERT INTO TempValues (Value)
VALUES (1), (2), (3), (4);
DECLARE @value INT;
DECLARE temp_cursor CURSOR FOR
SELECT Value FROM TempValues;
OPEN temp_cursor;
FETCH NEXT FROM temp_cursor INTO @value;
WHILE @@FETCH_STATUS = 0
BEGIN
— Perform actions with @value
PRINT @value; — Example action
FETCH NEXT FROM temp_cursor INTO @value;
END
CLOSE temp_cursor;
DEALLOCATE temp_cursor;
DROP TABLE TempValues;
“`
Performance Considerations
When implementing loops in SQL, consider the following to optimize performance:
- Minimize the Use of Cursors: Cursors can be slow; consider set-based operations whenever possible.
- Batch Processing: Process data in batches instead of row-by-row to enhance efficiency.
- Indexing: Ensure that the tables involved are properly indexed to speed up data retrieval.
Utilizing these methods effectively will allow you to loop through lists of values in SQL, enabling more dynamic and flexible data manipulation.
Expert Insights on SQL Loops for Iterating Through Lists
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When working with SQL loops, particularly for iterating through lists, it is essential to understand the performance implications. Using a cursor is common, but for larger datasets, consider set-based operations to enhance efficiency.”
Michael Chen (Senior Software Engineer, Data Dynamics). “In SQL, looping through each value in a list can be achieved using a WHILE loop or a CURSOR. However, developers should be cautious, as excessive looping can lead to performance bottlenecks. Always evaluate if a set-based approach could achieve the same result more efficiently.”
Lisa Patel (SQL Consultant, Query Masters). “Utilizing loops in SQL can simplify certain tasks, but they should be used judiciously. For each value in a list, leveraging temporary tables or table-valued parameters can often yield better performance than traditional looping constructs.”
Frequently Asked Questions (FAQs)
What is a loop in SQL?
A loop in SQL is a control structure that allows for the repeated execution of a block of SQL statements. It is commonly used in procedural extensions of SQL, such as PL/SQL or T-SQL, to perform operations on multiple rows or to execute a set of commands multiple times.
How can I loop through each value in a list in SQL?
To loop through each value in a list in SQL, you typically use a cursor or a WHILE loop in procedural SQL. A cursor allows you to fetch rows one at a time, while a WHILE loop can iterate based on a condition, executing the SQL statements for each iteration.
What are the differences between a cursor and a WHILE loop in SQL?
A cursor is designed to retrieve rows one at a time and is suitable for row-by-row processing, while a WHILE loop executes a block of code repeatedly until a specified condition is met. Cursors can be more memory-intensive, whereas WHILE loops can be simpler for certain tasks.
Can I use a FOR EACH loop in SQL?
SQL itself does not have a built-in FOR EACH loop construct. However, in procedural SQL languages like PL/SQL or T-SQL, you can achieve similar functionality using cursors or by iterating through collections or temporary tables.
What is the best practice for using loops in SQL?
Best practices for using loops in SQL include minimizing their use for performance reasons, opting for set-based operations whenever possible, and ensuring that the loop does not lead to excessive resource consumption. Always consider the impact on performance and scalability.
Are there performance implications when using loops in SQL?
Yes, using loops in SQL can lead to performance issues, especially with large datasets. Loops can result in increased execution time and resource utilization. It is generally advisable to use set-based operations instead of loops for better performance.
SQL loops, particularly when iterating through each value in a list, are essential for executing repetitive tasks within database operations. These loops enable developers to process multiple entries efficiently, allowing for operations such as updates, inserts, or deletions based on a dynamic set of data. Utilizing loops can significantly streamline workflows, especially in scenarios where batch processing is required, thereby enhancing overall performance and reducing the likelihood of errors in manual data manipulation.
One of the key insights regarding SQL loops is the distinction between different looping constructs available in SQL, such as cursors, while loops, and for-each loops. Each construct has its own advantages and use cases. For example, cursors provide a way to handle row-by-row processing, while while loops are beneficial for situations where the number of iterations is not predetermined. Understanding these differences is crucial for selecting the most appropriate method for a given task.
Moreover, it is important to consider the potential performance implications of using loops in SQL. While they can simplify complex operations, excessive or poorly optimized loops can lead to increased execution time and resource consumption. Therefore, best practices should be followed, such as minimizing the use of loops when set-based operations can achieve the same result more efficiently. This approach not only enhances
Author Profile
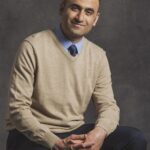
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?