How Can You Use an SQL Query to Check If a Table Exists?
In the world of database management, ensuring the integrity and existence of tables is a fundamental task that every developer and database administrator must master. Whether you’re working on a new project or maintaining an existing database, knowing how to check if a table exists can save you time, prevent errors, and streamline your workflow. SQL queries are the backbone of database interactions, and understanding how to utilize them effectively is crucial for anyone looking to harness the full power of relational databases.
When it comes to verifying the existence of a table, SQL provides several methods that can be employed across different database systems. Each database management system has its own nuances, but the core principle remains the same: you want to ensure that your queries run smoothly without encountering issues related to missing tables. This not only enhances the reliability of your applications but also aids in debugging and optimizing your database interactions.
In this article, we will explore the various SQL queries and techniques used to check for table existence. From simple conditional checks to more advanced approaches, you’ll learn how to implement these strategies in your own projects. Whether you’re a seasoned developer or just starting out, mastering this skill will undoubtedly enhance your database management capabilities and contribute to the overall success of your applications.
Checking for Table Existence in SQL
To determine whether a specific table exists within a database, SQL provides several methods depending on the database management system (DBMS) you are using. The following approaches are commonly used in different DBMSs to check for the existence of a table.
Using Information Schema
Most SQL databases support the `INFORMATION_SCHEMA` views, which contain metadata about the database objects. You can query the `TABLES` view to check for a table’s existence.
For example, in MySQL or PostgreSQL, you can use the following SQL query:
“`sql
SELECT *
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = ‘your_database_name’
AND TABLE_NAME = ‘your_table_name’;
“`
This query will return a row if the specified table exists in the specified schema. If no rows are returned, the table does not exist.
Using System Catalogs in PostgreSQL
In PostgreSQL, you can also check for a table’s existence using the `pg_catalog` schema:
“`sql
SELECT EXISTS (
SELECT 1
FROM pg_catalog.pg_tables
WHERE schemaname = ‘public’
AND tablename = ‘your_table_name’
);
“`
This query returns a boolean value (`true` or “) indicating whether the table exists.
Using IF EXISTS Clause in MySQL
In MySQL, you can use the `IF EXISTS` clause when dropping a table, which can help check its existence indirectly:
“`sql
DROP TABLE IF EXISTS your_table_name;
“`
This command will not throw an error if the table does not exist, effectively providing a way to check for existence before performing operations.
Using TRY-CATCH in SQL Server
In SQL Server, you can use a `TRY-CATCH` block to check for table existence:
“`sql
BEGIN TRY
SELECT * FROM your_table_name;
PRINT ‘Table exists.’;
END TRY
BEGIN CATCH
PRINT ‘Table does not exist.’;
END CATCH;
“`
This method attempts to select from the table and catches any errors that occur if the table does not exist.
Summary of Methods
Here’s a concise table summarizing the different methods to check if a table exists in various SQL databases:
DBMS | Query Method | Example |
---|---|---|
MySQL | INFORMATION_SCHEMA | SELECT * FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_NAME = ‘your_table_name’; |
PostgreSQL | pg_catalog | SELECT EXISTS (SELECT 1 FROM pg_catalog.pg_tables WHERE tablename = ‘your_table_name’); |
SQL Server | TRY-CATCH | BEGIN TRY SELECT * FROM your_table_name; END TRY BEGIN CATCH PRINT ‘Table does not exist.’; END CATCH; |
These methods provide a reliable way to check for the existence of tables across different SQL environments, ensuring that you can handle your database operations with confidence.
Checking if a Table Exists in SQL
To determine whether a specific table exists within a database, you can use various SQL queries depending on the database management system (DBMS) you are using. Below are examples for some of the most commonly used DBMSs.
MySQL
In MySQL, you can check for the existence of a table using the `SHOW TABLES` command or by querying the `information_schema`:
“`sql
SELECT COUNT(*)
FROM information_schema.tables
WHERE table_schema = ‘your_database_name’
AND table_name = ‘your_table_name’;
“`
- Replace `your_database_name` with the name of your database.
- Replace `your_table_name` with the name of the table you are checking for.
If the result returns `1`, the table exists; if it returns `0`, it does not.
PostgreSQL
For PostgreSQL, you can use a similar approach with the `pg_catalog` schema:
“`sql
SELECT EXISTS (
SELECT 1
FROM pg_tables
WHERE schemaname = ‘public’
AND tablename = ‘your_table_name’
);
“`
- Adjust `public` if your table resides in a different schema.
- Replace `your_table_name` with the actual table name.
This query returns `true` if the table exists, otherwise “.
SQL Server
In SQL Server, you can check for a table’s existence using the `OBJECT_ID` function:
“`sql
IF OBJECT_ID(‘schema_name.your_table_name’, ‘U’) IS NOT NULL
PRINT ‘Table exists’
ELSE
PRINT ‘Table does not exist’;
“`
- Replace `schema_name` with the appropriate schema (like `dbo`) and `your_table_name` with the name of the table.
This method will print a message indicating whether the table exists.
Oracle
In Oracle, you can query the `USER_TABLES` or `ALL_TABLES` views:
“`sql
SELECT COUNT(*)
FROM user_tables
WHERE table_name = ‘YOUR_TABLE_NAME’;
“`
- Ensure that you use uppercase for the `YOUR_TABLE_NAME` since Oracle stores table names in uppercase by default.
The result will indicate how many tables match the name provided, where `0` indicates non-existence.
SQLite
SQLite allows you to check for a table’s existence using the `sqlite_master` table:
“`sql
SELECT name
FROM sqlite_master
WHERE type=’table’
AND name=’your_table_name’;
“`
If the query returns a row, the table exists; if it returns no rows, the table does not exist.
Checking for the existence of a table is essential for database management tasks. The above queries are tailored for different SQL dialects, allowing you to seamlessly integrate this functionality into your database scripts. Always ensure to adapt the schema and table names as necessary for your specific use case.
Expert Insights on SQL Query for Table Existence
Dr. Emily Carter (Database Architect, Data Solutions Inc.). “To determine if a table exists in a SQL database, one can utilize the information_schema.tables view. A simple query can check for the presence of the table by filtering on the table name and schema, ensuring efficient verification without unnecessary overhead.”
James Liu (Senior SQL Developer, Tech Innovations). “Using the IF EXISTS clause in conjunction with a SELECT statement can provide a straightforward approach to check for table existence. This method is not only concise but also enhances readability, making it easier for developers to understand the intent of the query.”
Maria Gonzalez (Lead Data Analyst, Insight Analytics). “Incorporating error handling in your SQL queries when checking for table existence is crucial. This practice can prevent runtime errors and ensure that your application behaves predictably, especially in dynamic environments where table structures may change frequently.”
Frequently Asked Questions (FAQs)
How can I check if a table exists in SQL Server?
You can check if a table exists in SQL Server by using the following query:
“`sql
IF OBJECT_ID(‘schema_name.table_name’, ‘U’) IS NOT NULL
BEGIN
PRINT ‘Table exists.’
END
ELSE
BEGIN
PRINT ‘Table does not exist.’
END
“`
What SQL query can I use to verify a table’s existence in MySQL?
In MySQL, you can use the following query to check if a table exists:
“`sql
SHOW TABLES LIKE ‘table_name’;
“`
If the table exists, it will return the table name; otherwise, it will return an empty result.
Is there a way to check for table existence in PostgreSQL?
Yes, in PostgreSQL, you can use the following query:
“`sql
SELECT EXISTS (SELECT 1 FROM information_schema.tables
WHERE table_schema = ‘schema_name’ AND table_name = ‘table_name’);
“`
This will return a boolean indicating the presence of the table.
Can I check for table existence in SQLite?
Yes, in SQLite, you can use the following query to check for a table’s existence:
“`sql
SELECT name FROM sqlite_master WHERE type=’table’ AND name=’table_name’;
“`
If the table exists, it will return the table name; otherwise, it will return an empty result.
What is the purpose of checking if a table exists before creating it?
Checking if a table exists before creating it prevents errors and ensures that the database schema remains consistent. It avoids attempts to create duplicate tables, which can lead to runtime exceptions.
Are there any performance implications of checking for table existence?
While checking for table existence is generally a lightweight operation, excessive checks in performance-critical applications can introduce overhead. It’s advisable to minimize such checks in high-frequency operations.
In SQL, checking whether a table exists is a common requirement for database management and development. This process typically involves querying the system catalog or information schema, which contains metadata about the database objects. Different database management systems (DBMS) offer various methods to achieve this, such as using the `INFORMATION_SCHEMA` views in MySQL and SQL Server or leveraging system tables in PostgreSQL and Oracle.
One of the most widely used approaches is to execute a query that checks for the presence of the table in the relevant schema. For instance, in SQL Server, the query might look like: `SELECT * FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_NAME = ‘your_table_name’`. This method is efficient and provides a straightforward way to confirm the existence of a table before performing further operations, such as inserting data or altering the structure.
Additionally, utilizing conditional statements can enhance the robustness of your SQL scripts. By incorporating checks for table existence, developers can prevent errors that arise from attempting to access or modify non-existent tables. This practice not only streamlines the code but also contributes to better error handling and overall database integrity.
verifying the existence of a table in SQL is a fundamental task that can be accomplished through
Author Profile
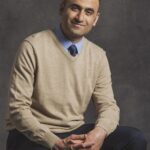
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?