How Can You Use SQL to Update Multiple Columns Efficiently?
In the world of database management, SQL (Structured Query Language) serves as the backbone for interacting with relational databases. As data continues to grow in complexity and size, the need for efficient data manipulation becomes increasingly vital. One common task that database administrators and developers face is the need to update multiple columns in a single query. This capability not only streamlines the process but also enhances performance by reducing the number of queries sent to the database. Whether you’re refining user information, adjusting product details, or correcting errors across multiple fields, mastering the art of updating multiple columns in SQL is an essential skill for anyone working with data.
Updating multiple columns in SQL can seem daunting at first, especially for those new to the language. However, with a clear understanding of the syntax and structure, this task becomes straightforward. The SQL UPDATE statement allows users to modify existing records in a table, and when combined with the proper conditions, it can efficiently target specific rows and columns for updates. This not only saves time but also minimizes the risk of errors that could arise from executing multiple individual update statements.
As we delve deeper into the mechanics of updating multiple columns, we will explore various techniques and best practices. From understanding the foundational syntax to examining real-world examples, this article will equip you with the knowledge needed to
Updating Multiple Columns in SQL
Updating multiple columns in SQL can streamline data management and enhance database efficiency. To perform this operation, you can utilize the `UPDATE` statement effectively, specifying the target table, the columns to be updated, and the new values.
The basic syntax for updating multiple columns is as follows:
“`sql
UPDATE table_name
SET column1 = value1,
column2 = value2,
column3 = value3
WHERE condition;
“`
In this syntax:
- `table_name` refers to the table containing the data.
- `column1`, `column2`, and `column3` are the columns to be updated.
- `value1`, `value2`, and `value3` are the new values to be assigned to these columns.
- The `WHERE` clause specifies which records should be updated. Omitting this clause will update all records in the table.
Example of Updating Multiple Columns
Consider a scenario where you have a `Employees` table with columns for `FirstName`, `LastName`, and `Salary`. If you need to update the salary and last name of an employee with a specific `EmployeeID`, you can execute the following SQL command:
“`sql
UPDATE Employees
SET LastName = ‘Smith’,
Salary = 75000
WHERE EmployeeID = 1234;
“`
This command updates the `LastName` to ‘Smith’ and the `Salary` to 75000 for the employee whose `EmployeeID` is 1234.
Best Practices for Updating Multiple Columns
When updating multiple columns, adhere to these best practices:
- Always use a WHERE clause: To prevent unintended updates, ensure that the `WHERE` clause accurately identifies the records to be modified.
- Backup data before updates: Regularly back up your data to safeguard against accidental loss during updates.
- Use transactions: If your database supports transactions, consider wrapping your updates in a transaction to maintain data integrity. For example:
“`sql
BEGIN TRANSACTION;
UPDATE Employees
SET LastName = ‘Smith’,
Salary = 75000
WHERE EmployeeID = 1234;
COMMIT;
“`
- Test your queries: Before executing updates on production data, test your SQL queries in a development environment.
Performance Considerations
Updating multiple columns in a table can affect performance, especially with large datasets. Consider the following:
- Indexing: Ensure that columns used in the `WHERE` clause are indexed to enhance query performance.
- Batch updates: If updating many records, consider batching updates to minimize locking and enhance performance.
Column Name | Data Type | Example Value |
---|---|---|
FirstName | VARCHAR(50) | John |
LastName | VARCHAR(50) | Doe |
Salary | DECIMAL(10, 2) | 65000.00 |
By following these guidelines and understanding the syntax, you can effectively manage updates across multiple columns in your SQL databases, ensuring both efficiency and data integrity.
Updating Multiple Columns in SQL
To update multiple columns in a SQL table, the `UPDATE` statement is utilized, allowing you to modify one or more columns simultaneously. This is particularly useful for maintaining data integrity and ensuring that related information is updated consistently across the database.
Basic Syntax
The basic syntax for updating multiple columns in SQL is as follows:
“`sql
UPDATE table_name
SET column1 = value1,
column2 = value2,
column3 = value3
WHERE condition;
“`
- table_name: The name of the table containing the columns you wish to update.
- column1, column2, column3: The columns to be updated.
- value1, value2, value3: The new values assigned to each column.
- condition: A condition to specify which rows should be updated. If omitted, all rows will be affected.
Example of Updating Multiple Columns
Assume you have a table named `employees` with the following columns: `first_name`, `last_name`, `department`, and `salary`. To update the department and salary of an employee with the last name ‘Smith’, you would write:
“`sql
UPDATE employees
SET department = ‘Marketing’,
salary = 60000
WHERE last_name = ‘Smith’;
“`
This command updates the department to ‘Marketing’ and the salary to 60,000 for all employees whose last name is ‘Smith’.
Using Multiple Conditions
You can specify multiple conditions in the `WHERE` clause to narrow down which records to update. For example:
“`sql
UPDATE employees
SET department = ‘Sales’,
salary = 70000
WHERE last_name = ‘Doe’ AND first_name = ‘John’;
“`
This updates the department and salary only for John Doe.
Updating with Subqueries
Sometimes, you may need to update a column based on values from another table. This can be accomplished using a subquery. Here’s an example:
“`sql
UPDATE employees
SET salary = (SELECT AVG(salary) FROM employees WHERE department = ‘Sales’)
WHERE department = ‘Marketing’;
“`
In this example, the salary for all employees in the Marketing department is updated to the average salary of employees in the Sales department.
Transaction Control
When updating multiple rows, it’s prudent to use transaction control commands to maintain data integrity. This can be achieved through the following commands:
- BEGIN TRANSACTION: Starts a new transaction.
- COMMIT: Saves all changes made during the transaction.
- ROLLBACK: Undoes changes made during the transaction if an error occurs.
Example:
“`sql
BEGIN TRANSACTION;
UPDATE employees
SET salary = salary * 1.10
WHERE department = ‘Engineering’;
COMMIT;
“`
If an error occurs, you can use `ROLLBACK` to revert changes.
Updating multiple columns in SQL is a straightforward process that enhances data management efficiency. By utilizing the correct syntax and understanding how to implement conditions and transactions, users can effectively maintain their databases.
Expert Insights on Updating Multiple Columns in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When updating multiple columns in SQL, it is crucial to ensure that your SET clause is properly structured. Using a single UPDATE statement with multiple column assignments not only enhances performance but also maintains data integrity across related fields.”
James Patel (Senior Database Administrator, Data Solutions Group). “Utilizing transactions when updating multiple columns is essential. This approach allows you to roll back changes if any part of the update fails, thus preserving the consistency of your database.”
Lisa Tran (SQL Consultant, OptimizeDB). “Incorporating conditional logic within your UPDATE statement can significantly improve the efficiency of updating multiple columns. By using CASE statements, you can apply different values based on specific conditions, which reduces the need for multiple queries.”
Frequently Asked Questions (FAQs)
How can I update multiple columns in a single SQL statement?
You can update multiple columns in a single SQL statement by specifying each column and its new value in the SET clause of the UPDATE statement. For example:
“`sql
UPDATE table_name
SET column1 = value1, column2 = value2
WHERE condition;
“`
Is it possible to update multiple rows at once in SQL?
Yes, you can update multiple rows at once by using a WHERE clause that matches multiple records. For example:
“`sql
UPDATE table_name
SET column1 = value1
WHERE condition;
“`
The condition can be based on a specific criterion that applies to multiple rows.
Can I use a subquery to update multiple columns in SQL?
Yes, you can use a subquery to update multiple columns. The subquery can return values that are used to set the new values for the columns. For example:
“`sql
UPDATE table_name
SET column1 = (SELECT value FROM another_table WHERE condition),
column2 = (SELECT value FROM another_table WHERE condition)
WHERE condition;
“`
What is the syntax for updating multiple columns with different values in SQL?
The syntax for updating multiple columns with different values is as follows:
“`sql
UPDATE table_name
SET column1 = value1,
column2 = value2,
column3 = value3
WHERE condition;
“`
Each column can be assigned a unique value.
Are there any performance considerations when updating multiple columns in SQL?
Yes, updating multiple columns can impact performance, especially if the table has a large number of rows or if the update involves complex conditions. It is advisable to ensure that appropriate indexes are in place and to limit the number of rows affected by the update.
What happens if I forget to include a WHERE clause in my update statement?
If you forget to include a WHERE clause in your update statement, all rows in the table will be updated with the specified values. This can lead to unintended data changes, so it is crucial to always verify the condition before executing the update.
In summary, updating multiple columns in SQL is a fundamental operation that allows users to modify existing records efficiently. The SQL UPDATE statement is utilized for this purpose, enabling the specification of multiple column-value pairs within a single command. This approach not only streamlines the update process but also enhances performance by reducing the number of database transactions required, which is particularly beneficial in scenarios involving large datasets.
When constructing an SQL UPDATE query to modify multiple columns, it is essential to follow the correct syntax. The basic structure involves the UPDATE statement, followed by the table name, the SET clause with the column-value pairs, and an optional WHERE clause to specify which records should be updated. This ensures that only the intended records are affected, thereby maintaining data integrity.
Key takeaways from this discussion include the importance of using the WHERE clause judiciously to prevent unintended updates to all records in a table. Additionally, understanding the implications of updating multiple columns simultaneously can lead to more efficient database management practices. By mastering the SQL UPDATE statement, users can enhance their ability to maintain and manipulate data effectively within relational databases.
Author Profile
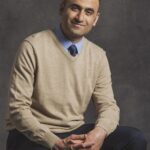
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?