How Can You Determine the Number of Rows Deleted in SQLite3?
In the world of database management, SQLite stands out as a lightweight yet powerful option for developers and data enthusiasts alike. Whether you’re building a mobile application or managing a small-scale project, understanding how to efficiently manipulate data is crucial. One common operation that developers encounter is the deletion of rows from a SQLite database. But how can you determine exactly how many rows were deleted after executing a DELETE statement? This question is not just a matter of curiosity; it’s essential for maintaining data integrity and ensuring that your application behaves as expected.
When you execute a DELETE command in SQLite, the database engine performs the operation and returns a result that indicates the success of the command. However, knowing the number of rows affected can provide invaluable insights into the state of your data. This metric can help you verify that your deletion criteria were correctly applied and that no unintended data was removed. Additionally, tracking the number of deleted rows can assist in debugging and optimizing your queries, ensuring that your database remains efficient and responsive.
In this article, we will explore the various methods available in SQLite to determine how many rows have been deleted after executing a DELETE statement. We will delve into the underlying mechanisms, best practices, and tips for effectively managing your SQLite database. By the end, you’ll be equipped with the knowledge to
Understanding Row Deletion in SQLite3
When working with SQLite3, it is essential to determine how many rows have been deleted after executing a DELETE statement. This can be accomplished using the built-in `sqlite3_changes()` function, which returns the number of rows affected by the last INSERT, UPDATE, or DELETE statement.
To effectively utilize this function, consider the following:
- The function must be called immediately after the DELETE operation to ensure it returns accurate results.
- It can be used in conjunction with transactions to maintain data integrity and verify the success of your operations.
Example of Deleting Rows and Tracking Changes
Here is an example of how to delete rows from a table and check how many were deleted:
“`sql
BEGIN TRANSACTION;
DELETE FROM employees WHERE department = ‘Sales’;
SELECT sqlite3_changes();
COMMIT;
“`
In the above example:
- A transaction is initiated to ensure that the deletion is atomic.
- Rows from the `employees` table where the department is ‘Sales’ are deleted.
- The `sqlite3_changes()` function is called to determine the number of rows affected by the DELETE operation.
Using C API for Row Deletion Count
For developers using the C API, the process is similar, but the implementation requires specific function calls. Here’s a brief overview:
- Execute the DELETE statement using `sqlite3_exec()`.
- Immediately call `sqlite3_changes(db)` after the deletion to retrieve the count of affected rows.
Example in C:
“`c
sqlite3 *db;
char *errMsg = 0;
int rc;
rc = sqlite3_open(“mydatabase.db”, &db);
if (rc) {
// Handle error
}
sqlite3_exec(db, “DELETE FROM employees WHERE department = ‘Sales’;”, 0, 0, &errMsg);
int changes = sqlite3_changes(db);
// Now you can use ‘changes’ to know how many rows were deleted
sqlite3_close(db);
“`
Summary of Row Deletion Methods
Here is a summary table of different methods to determine how many rows were deleted:
Method | Description | Use Case |
---|---|---|
sqlite3_changes() | Returns the number of rows affected by the last SQL statement. | After executing DELETE/UPDATE/INSERT. |
Row Count in C API | Use sqlite3_changes() after executing DELETE. | For C programming with SQLite. |
SQL SELECT COUNT() | Count rows before deletion as a comparison. | When tracking changes over time. |
By employing these methods, you can ensure accurate tracking of row deletions and maintain the integrity of your database operations within SQLite3.
Understanding SQLite3 Row Deletion and Affected Rows
When executing a deletion operation in SQLite3, understanding how to determine the number of rows affected is crucial for maintaining data integrity and ensuring that the application behaves as expected.
Using the `DELETE` Statement
The `DELETE` statement in SQLite3 removes rows from a table based on specified conditions. The basic syntax is:
“`sql
DELETE FROM table_name WHERE condition;
“`
To effectively determine how many rows were deleted, you can utilize the following methods:
Checking the Number of Affected Rows
SQLite3 provides a straightforward way to check the number of rows affected by the last executed statement through the `changes()` function. This function returns the number of rows modified by the most recent `INSERT`, `UPDATE`, or `DELETE` statement.
- Example:
“`sql
DELETE FROM employees WHERE department = ‘Sales’;
SELECT changes();
“`
In this example, after executing the `DELETE` statement, the `SELECT changes();` query will return the number of rows that were deleted.
Using the SQLite3 Command-Line Interface
If you are using the SQLite3 command-line interface, you can easily check the number of deleted rows by following these steps:
- Execute the `DELETE` command.
- Immediately after, run the `.changes` command to display the number of affected rows.
– **Example:**
“`sql
sqlite> DELETE FROM employees WHERE department = ‘Sales’;
sqlite> .changes
“`
This will output the number of rows deleted from the `employees` table.
Using SQLite3 with Programming Languages
When interfacing SQLite3 with programming languages such as Python, you can retrieve the number of deleted rows using the cursor object’s `rowcount` property.
- Python Example:
“`python
import sqlite3
connection = sqlite3.connect(‘example.db’)
cursor = connection.cursor()
cursor.execute(“DELETE FROM employees WHERE department = ‘Sales'”)
print(“Rows deleted:”, cursor.rowcount)
connection.commit()
connection.close()
“`
In this snippet, `cursor.rowcount` provides the number of rows deleted after the execution of the `DELETE` statement.
Considerations When Deleting Rows
- No Condition Specified: If you execute a `DELETE` statement without a `WHERE` clause, all rows in the table will be deleted, and `changes()` will return the total number of rows in that table.
- Transactions: When performing multiple deletions, consider using transactions to ensure that all operations are executed as a single unit. Use `BEGIN TRANSACTION` and `COMMIT` to wrap your delete operations.
Action | Affected Rows |
---|---|
DELETE with condition | Specific rows deleted |
DELETE without condition | All rows deleted |
Using `SELECT changes();` | Shows deleted rows |
Using `cursor.rowcount` | Rows deleted in program context |
By employing these techniques, you can effectively track the number of rows deleted in your SQLite3 database operations, ensuring your data management processes remain reliable and efficient.
Understanding Row Deletion in SQLite3
Dr. Emily Chen (Database Systems Researcher, Tech Innovations Journal). “To determine how many rows have been deleted in SQLite3, one can utilize the built-in `changes()` function, which returns the number of rows affected by the last SQL statement. This function is particularly useful for tracking changes in a database after a delete operation.”
Mark Thompson (Senior Database Administrator, Data Solutions Inc.). “In SQLite3, after executing a delete command, you can also check the result of the command itself. The SQL statement will return the number of rows deleted, which can be captured and logged for auditing purposes. This approach ensures that you have a clear record of the database’s state.”
Lisa Patel (Software Engineer, Open Source Database Community). “For applications that require tracking deletions over time, implementing a trigger that logs deletions to a separate table can be beneficial. This way, you can maintain a history of deleted rows and analyze the data as needed, rather than relying solely on the immediate output of the delete command.”
Frequently Asked Questions (FAQs)
How can I determine the number of rows deleted in SQLite3?
You can determine the number of rows deleted in SQLite3 by using the `sqlite3_changes()` function immediately after executing a DELETE statement. This function returns the number of rows that were changed by the last SQL statement executed.
Is there a way to get the number of deleted rows in a single SQL query?
No, SQLite3 does not provide a built-in way to return the number of deleted rows directly in a single DELETE statement. However, you can use a combination of a SELECT statement to count rows before deletion and then execute the DELETE statement.
What is the purpose of the `DELETE` statement in SQLite3?
The `DELETE` statement in SQLite3 is used to remove one or more rows from a table based on specified conditions. It is essential for managing data integrity and ensuring that obsolete or unnecessary data is removed.
Can I log deleted rows in SQLite3 for future reference?
Yes, you can log deleted rows by creating a trigger that inserts the deleted rows into a separate logging table before they are removed. This way, you can keep a record of all deletions for auditing or recovery purposes.
What happens if I try to delete rows that do not exist?
If you attempt to delete rows that do not exist, SQLite3 will simply not affect any rows, and the operation will still succeed without generating an error. The return value from `sqlite3_changes()` will indicate that zero rows were deleted.
How can I check if a DELETE operation was successful in SQLite3?
You can check the success of a DELETE operation by examining the return value of the `sqlite3_changes()` function. If it returns a value greater than zero, the operation was successful and rows were deleted. If it returns zero, no rows matched the deletion criteria.
In SQLite, determining how many rows have been deleted after executing a DELETE statement is straightforward. After the execution of a DELETE command, the SQLite database engine provides a way to retrieve the number of affected rows using the `changes()` function. This function returns the count of rows that were modified by the last executed statement, which includes deletions, updates, and insertions. This feature is particularly useful for applications that require confirmation of the number of records impacted by a database operation.
Moreover, utilizing the `sqlite3` command-line interface or the SQLite C API allows developers to access this information easily. For instance, in the command-line interface, after performing a DELETE operation, executing the command `SELECT changes();` will yield the number of rows deleted. This capability can be instrumental in scenarios where data integrity and auditing are critical, as it provides immediate feedback on the operation’s impact.
In summary, the ability to determine how many rows have been deleted in SQLite enhances the database management experience. By leveraging the `changes()` function, developers can efficiently track modifications and ensure that their applications respond appropriately to data changes. This feature not only aids in debugging but also supports the overall reliability of data operations within SQLite databases.
Author Profile
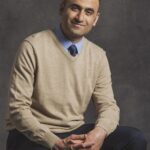
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?