Why Do I Encounter ‘String Indices Must Be Integers Not ‘Str’ Error in Python?
Understanding the Error: “string indices must be integers not ‘str'”
In the world of programming, encountering errors is part of the journey, but some messages can be particularly perplexing. One such error is the infamous “string indices must be integers not ‘str’.” This cryptic message often leaves developers scratching their heads, especially those who are new to Python or similar programming languages. As you delve into the intricacies of this error, you’ll discover that it serves as a crucial reminder of how data types interact within your code.
At its core, this error arises when a programmer attempts to access a string using a string index instead of the required integer index. Understanding the nature of strings and indices in programming is essential to unraveling this common pitfall. As you explore the nuances of this issue, you’ll learn not only why it occurs but also how to avoid it in your coding practices.
Throughout this article, we will dissect the underlying principles that lead to this error, offering insights and practical examples to illuminate the path forward. Whether you’re troubleshooting your own code or simply looking to deepen your understanding of data types, this exploration will equip you with the knowledge to navigate the complexities of string manipulation with confidence.
Understanding the Error Message
The error message “string indices must be integers, not ‘str'” typically occurs in Python programming when attempting to access elements of a string using string indices. In Python, strings are sequences of characters, and they can only be accessed using integer indices. This error often arises in the following contexts:
- When mistakenly treating a string as a dictionary or list.
- When trying to iterate through a string and using string keys instead of integer indices.
To clarify the issue, consider the following example:
“`python
data = “Hello”
print(data[‘H’]) This will raise the error
“`
In this code, the attempt to access the string `data` with a character key (‘H’) results in an error because only integers are valid indices for strings.
Common Scenarios Leading to the Error
There are several common scenarios where this error can occur:
- Accessing String Elements Incorrectly: When you attempt to index a string using another string as the index.
- Confusion Between Data Types: Mixing up strings with dictionaries or lists, where strings are treated as if they can be indexed with keys.
- Incorrect Looping Constructs: Using a string in a loop where a dictionary is expected, leading to an attempt to access it with string keys.
Example Scenarios
Below are a couple of examples that illustrate the error in different contexts.
- Incorrect Indexing:
“`python
my_string = “Python”
print(my_string[‘0’]) Raises the error
“`
- Dictionary Misuse:
“`python
my_dict = {“name”: “Alice”, “age”: 30}
print(my_dict[“name”]) Correct usage
print(my_dict[“0”]) Raises the error
“`
Debugging the Error
To resolve the “string indices must be integers, not ‘str'” error, follow these debugging steps:
- Review the Data Structure: Ensure you are aware of the type of data you are working with (string, list, dictionary).
- Check Indexing Methods: Verify that you are using integer indices for strings and string keys for dictionaries.
- Utilize Print Statements: Add print statements before the line causing the error to inspect the data types and structures.
Best Practices to Avoid the Error
To mitigate the risk of encountering this error, consider adopting the following best practices:
- Always validate the data type before performing indexing operations.
- Use `type()` function to check the type of the variable if unsure.
- Implement exception handling to gracefully manage errors.
Error Scenario | Correction |
---|---|
Accessing string with a string key | Use an integer index instead |
Confusing dictionary with string | Ensure correct data structure is used |
Using a string in loops | Confirm the expected data type for the loop |
Understanding the Error
The error message “string indices must be integers, not ‘str'” occurs in Python when attempting to access elements of a string using a string index rather than an integer index. This misunderstanding often arises from confusion between string and dictionary data types.
- Strings: In Python, strings are sequences of characters, and their indices must be integers (e.g., `my_string[0]`).
- Dictionaries: These are collections of key-value pairs, where keys can be strings (e.g., `my_dict[‘key’]`).
This error typically indicates that the code is trying to treat a string as if it were a dictionary.
Common Scenarios Leading to the Error
Several common coding practices can trigger this error:
- Incorrect Variable Usage: Using a string variable where a dictionary is expected.
“`python
my_string = “example”
print(my_string[‘key’]) This will raise the error.
“`
- Improper Parsing of Data: When parsing JSON or other data formats, if the data structure is not as expected, it can lead to this error.
“`python
import json
data = json.loads(‘{“key”: “value”}’)
print(data[‘key’]) This is correct; accessing a dictionary.
print(data[‘key’][0]) Correctly accessing a string’s index.
“`
- Looping through Strings Instead of Dictionaries: Looping through strings without properly handling the data type can lead to confusion.
“`python
for char in my_string:
print(char[‘key’]) Incorrect: char is a string.
“`
Debugging Techniques
To troubleshoot this error effectively, consider the following approaches:
- Check Data Types: Use the `type()` function to verify the data type of variables.
“`python
print(type(my_variable))
“`
- Print Intermediate Values: Before the line causing the error, print out the variable to understand its content.
“`python
print(my_variable) Helps to ensure it’s the expected type.
“`
- Review Data Structures: Ensure that the data structure being accessed is indeed a dictionary if string keys are being used.
Examples of Correct Usage
Here are some corrected examples demonstrating proper access:
- Accessing a String:
“`python
my_string = “Hello”
print(my_string[1]) Outputs: e
“`
- Accessing a Dictionary:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
print(my_dict[‘name’]) Outputs: Alice
“`
- Combining Both:
“`python
data = {‘greeting’: ‘Hello’}
print(data[‘greeting’][0]) Outputs: H
“`
Preventative Measures
To prevent encountering the “string indices must be integers, not ‘str'” error, consider implementing these practices:
- Type Checking: Before accessing elements, verify the expected data type.
- Use Descriptive Variable Names: This helps clarify the intended data structure.
- Implement Exception Handling: Use try-except blocks to catch and handle errors gracefully.
“`python
try:
print(my_variable[‘key’])
except TypeError:
print(“Caught a TypeError: Ensure my_variable is a dictionary.”)
“`
By understanding the nature of this error and applying the suggested debugging techniques, developers can avoid common pitfalls associated with data type mismanagement in Python.
Understanding the Error: “string indices must be integers not ‘str'”
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘string indices must be integers not ‘str” typically arises in Python when attempting to access a character in a string using a string key instead of an integer index. This highlights the importance of understanding data types and their appropriate usage in programming.”
Michael Thompson (Lead Data Scientist, Data Solutions Group). “In data manipulation, especially when dealing with JSON objects, this error can occur if one mistakenly treats a string as a dictionary. Properly parsing the data structure before accessing its elements is crucial to avoid such issues.”
Sarah Jenkins (Python Programming Instructor, Code Academy). “Students often encounter the ‘string indices must be integers not ‘str” error when they are new to Python. It serves as a valuable lesson in understanding the difference between mutable and immutable types, and the significance of correct indexing.”
Frequently Asked Questions (FAQs)
What does the error “string indices must be integers not ‘str'” mean?
This error occurs in Python when attempting to access a character in a string using a string index instead of an integer. Strings can only be indexed with integers representing the position of the character.
In which scenarios might I encounter this error?
You may encounter this error when mistakenly trying to index a string using another string variable or when trying to access dictionary values without properly referencing the dictionary structure.
How can I fix the “string indices must be integers not ‘str'” error?
To fix this error, ensure that you are using integer indices when accessing string elements. If you are working with a dictionary, confirm that you are using the correct key to access its values.
Can this error occur when working with JSON data?
Yes, this error can occur when parsing JSON data. If you attempt to access a string within a JSON object using a string key instead of accessing the object correctly, it will lead to this error.
What are some common coding mistakes that lead to this error?
Common mistakes include confusing strings with dictionaries, incorrectly nesting data structures, or failing to convert data types when necessary. Always verify the data type of the variable before accessing its elements.
Is there a way to prevent this error in my code?
To prevent this error, implement type checks before accessing indices. Use functions like `isinstance()` to ensure that you are working with the expected data types, and consider using exception handling to manage potential errors gracefully.
The error message “string indices must be integers, not ‘str'” typically arises in Python programming when a developer attempts to access a character in a string using a string index rather than an integer. This indicates a fundamental misunderstanding of how string indexing works in Python, as strings are indexed by integers that represent the position of characters within the string, starting from zero. This error often surfaces in the context of manipulating data structures, particularly when dealing with dictionaries and lists where keys and indices can be confused.
To resolve this issue, it is essential to ensure that the correct data type is being used for indexing. When accessing elements in a string, one must utilize integer indices. Conversely, if the intention is to access elements in a dictionary, string keys should be applied. This distinction is crucial in preventing type errors and ensuring that the program functions as intended. Debugging techniques, such as printing variable types and values, can be beneficial in identifying where the confusion may lie.
In summary, the “string indices must be integers, not ‘str'” error serves as a reminder of the importance of understanding data types and their respective operations in Python. Developers should take care to familiarize themselves with the nuances of strings, lists, and dictionaries to avoid such errors
Author Profile
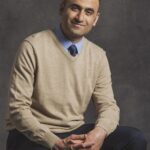
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?